Using the Quickbooks API to Get Products in Javascript
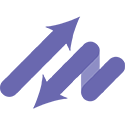
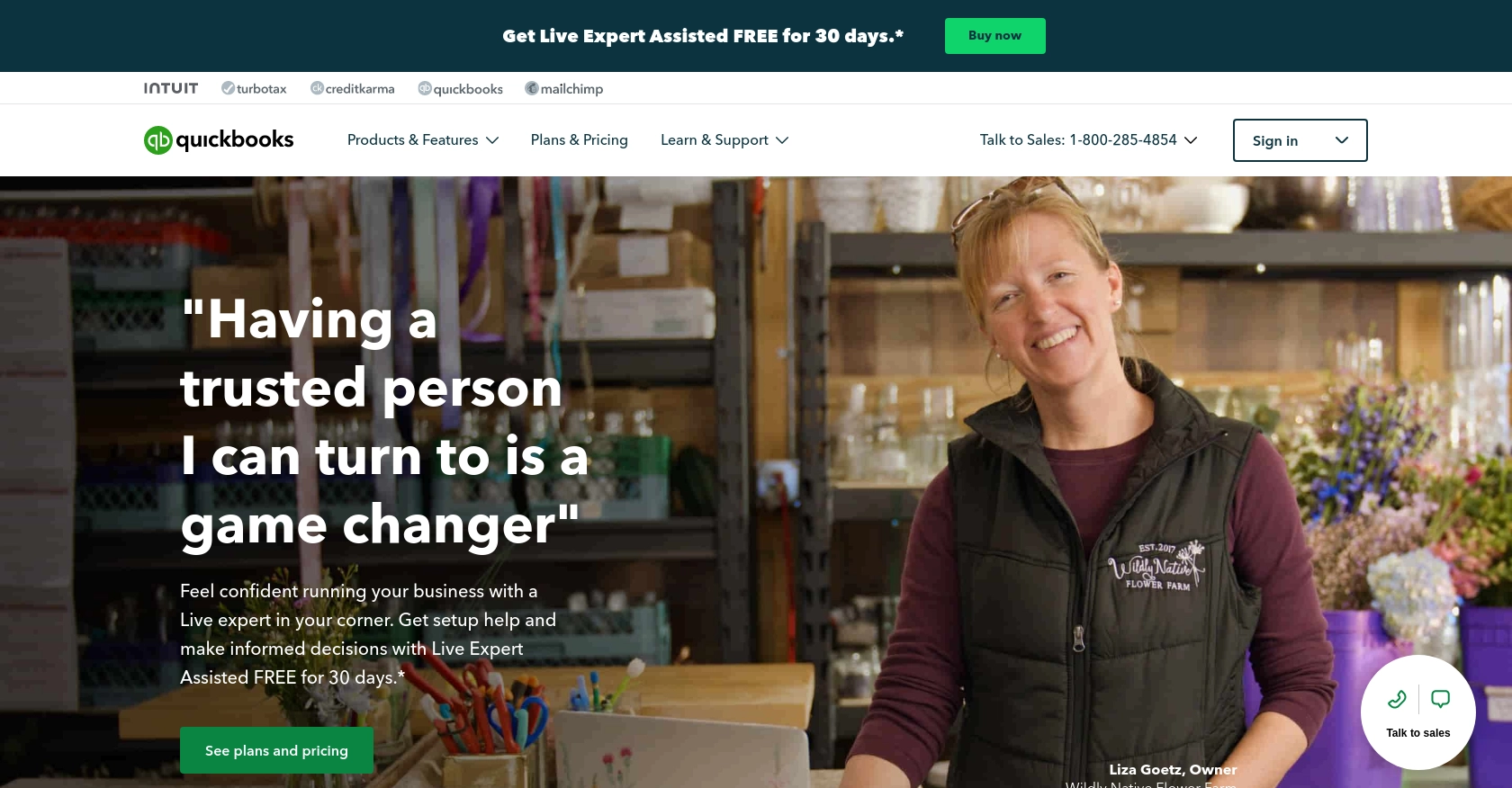
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a range of features including invoicing, expense tracking, payroll, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Developers often integrate with QuickBooks API to automate financial processes and enhance business operations. For example, accessing product data through the QuickBooks API can enable developers to synchronize inventory systems, ensuring that product information is always up-to-date across platforms.
This article will guide you through using JavaScript to interact with the QuickBooks API, specifically focusing on retrieving product information. By the end of this tutorial, you'll be able to efficiently access and manage product data within QuickBooks using JavaScript.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before diving into the QuickBooks API, you'll need to set up a sandbox account. This environment allows you to test API interactions without affecting live data, making it an essential step for developers looking to integrate with QuickBooks.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company to test API calls:
- Navigate to the "Sandbox" section in your QuickBooks Developer dashboard.
- Select "Create a Sandbox Company" and follow the prompts to set up your test environment.
Generating OAuth Credentials for QuickBooks API
The QuickBooks API uses OAuth 2.0 for authentication. Here's how to generate the necessary credentials:
- Go to the App Management section in your developer account.
- Click on "Create an App" and provide the required details.
- Once your app is created, navigate to the "Keys & OAuth" tab to find your Client ID and Client Secret.
- Make sure to save these credentials securely, as you'll need them to authenticate API requests.
With your sandbox account and OAuth credentials ready, you're all set to start interacting with the QuickBooks API using JavaScript. In the next section, we'll explore how to make API calls to retrieve product information.
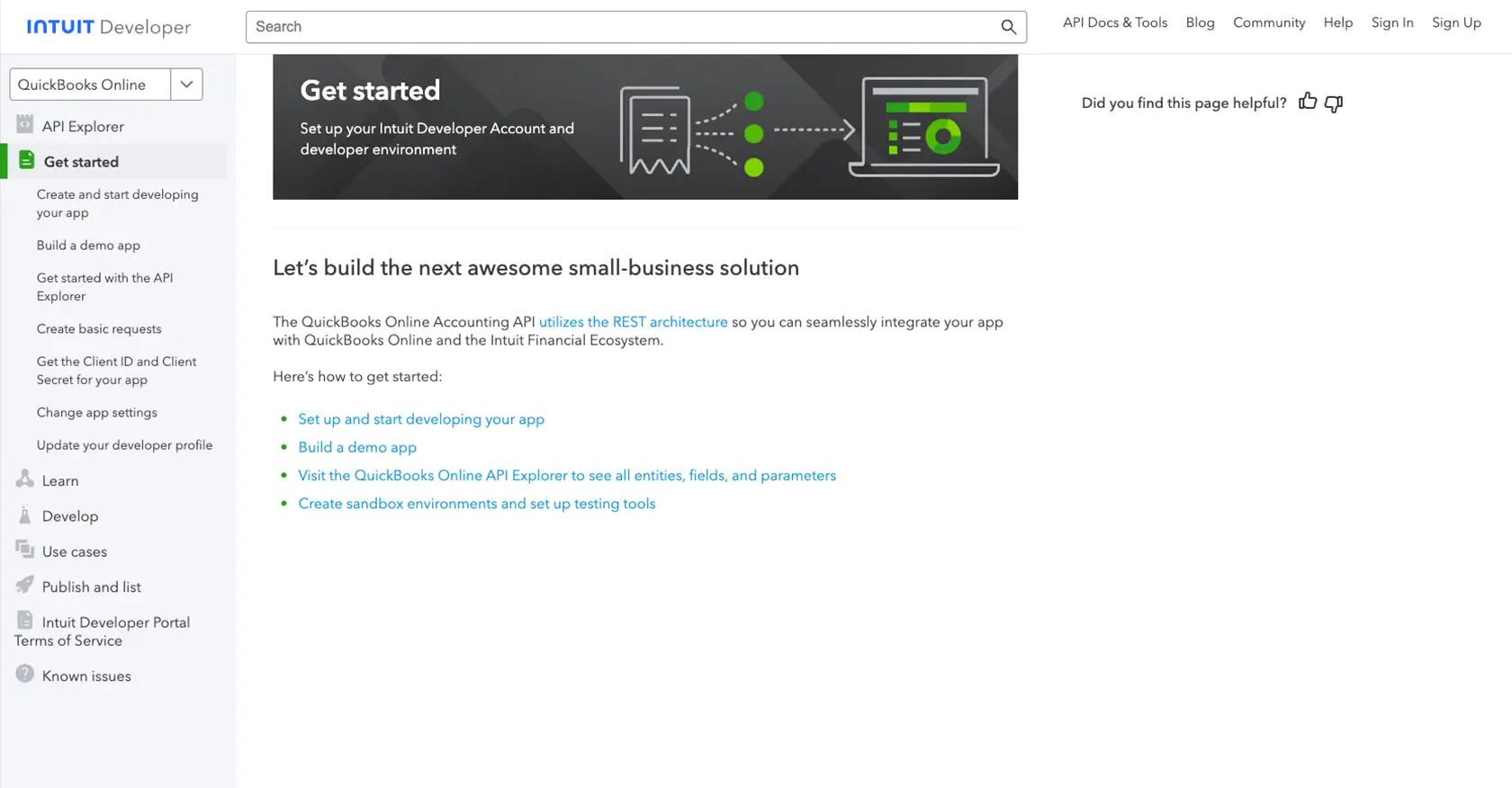
sbb-itb-96038d7
Making API Calls to Retrieve Products from QuickBooks Using JavaScript
Now that you have your QuickBooks sandbox account and OAuth credentials set up, it's time to dive into making API calls to retrieve product information. This section will guide you through the necessary steps to interact with the QuickBooks API using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
Start by creating a new project directory and initializing it:
mkdir quickbooks-api-integration
cd quickbooks-api-integration
npm init -y
Installing Required Dependencies
You'll need the axios
library to make HTTP requests and dotenv
to manage environment variables securely. Install them using the following command:
npm install axios dotenv
Writing the JavaScript Code to Fetch Products
Create a file named getProducts.js
and add the following code:
require('dotenv').config();
const axios = require('axios');
// QuickBooks API endpoint for retrieving products
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/item';
// Function to get products
async function getProducts() {
try {
const response = await axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${process.env.ACCESS_TOKEN}`,
'Accept': 'application/json'
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching products:', error.response ? error.response.data : error.message);
}
}
getProducts();
Replace YOUR_COMPANY_ID
with your actual sandbox company ID and ensure your .env
file contains your ACCESS_TOKEN
.
Running the Code and Verifying Results
Run the script using the following command:
node getProducts.js
If successful, you should see the product data from your QuickBooks sandbox account printed in the console. Verify the data by checking your sandbox environment to ensure it matches the retrieved information.
Handling Errors and Common Error Codes
When interacting with the QuickBooks API, you might encounter errors. Here are some common error codes and their meanings:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your app has the necessary permissions to access the requested resource.
- 404 Not Found: Verify the endpoint URL and company ID are correct.
For more detailed error handling, refer to the QuickBooks API documentation.
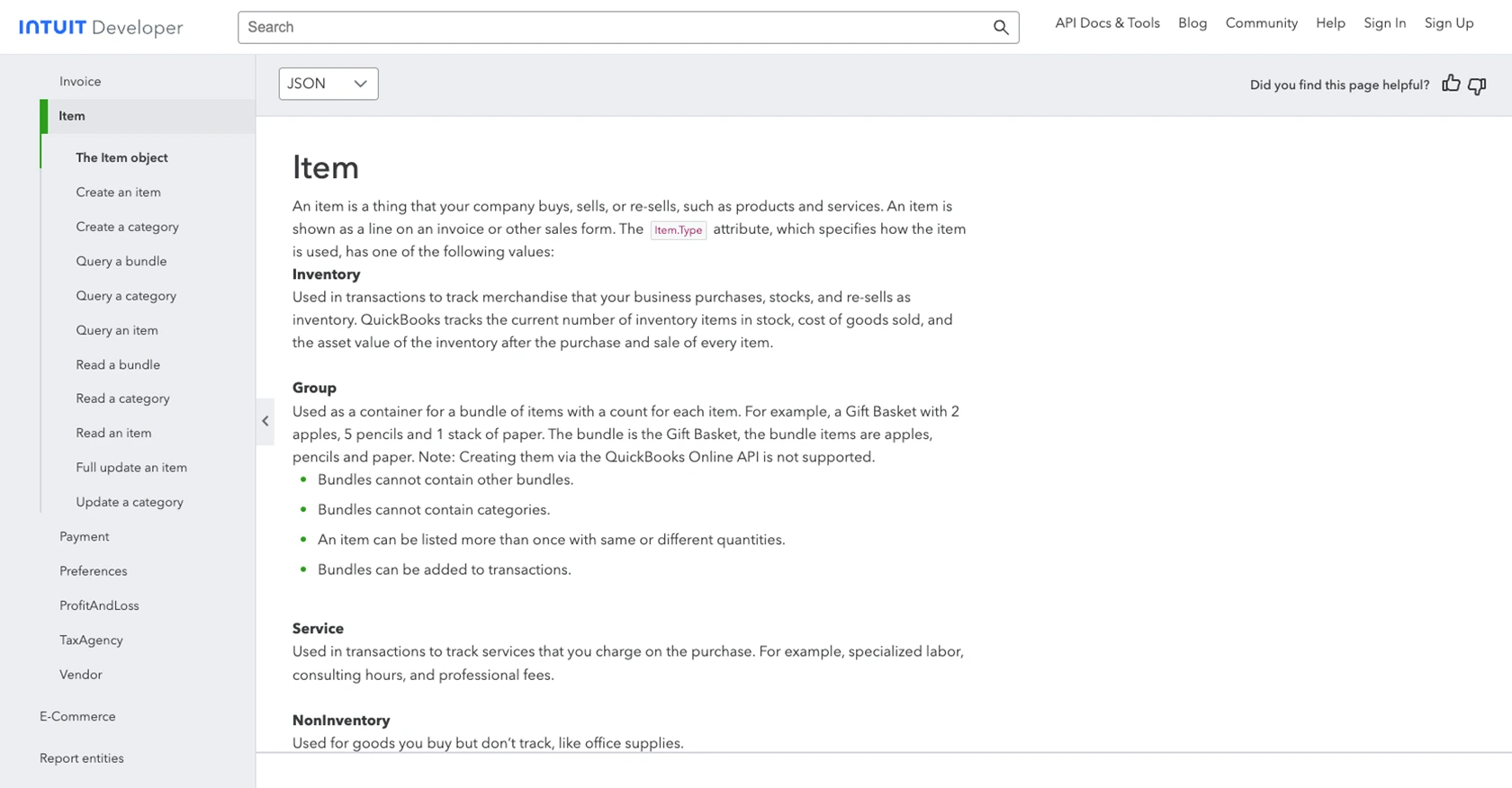
Conclusion: Best Practices for QuickBooks API Integration Using JavaScript
Integrating with the QuickBooks API using JavaScript can significantly enhance your application's ability to manage financial data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve product information and ensure your inventory systems are synchronized with QuickBooks.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and Access Tokens securely. Use environment variables and avoid hardcoding sensitive information in your codebase.
- Handle Rate Limiting: QuickBooks API has rate limits in place. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from QuickBooks is standardized and transformed as needed to fit your application's data model.
Enhance Your Integration Strategy with Endgrate
While integrating with QuickBooks API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various services, including QuickBooks.
With Endgrate, you can save time and resources by building integrations once and reusing them across different platforms. This allows you to focus on your core product while offering a seamless integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/item
Ready to get started?