How to Send Messages with the SMTP API in PHP
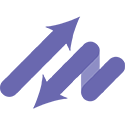
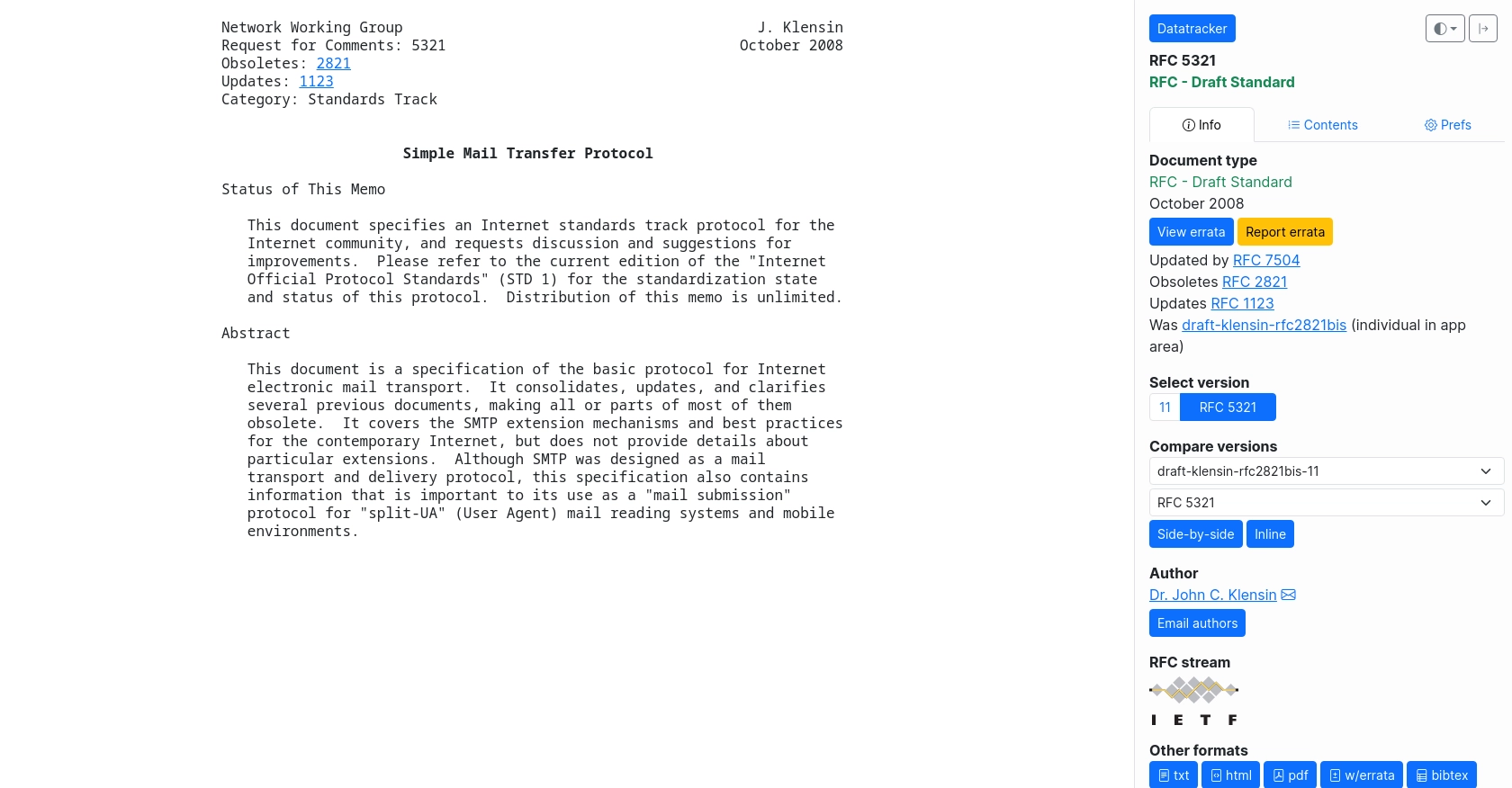
Introduction to SMTP and Its Importance in PHP Development
SMTP, or Simple Mail Transfer Protocol, is a fundamental protocol used for sending emails across the internet. It is widely adopted by developers to facilitate the transmission of messages between servers and email clients. SMTP's reliability and efficiency make it an essential tool for businesses and developers looking to implement email functionalities in their applications.
For PHP developers, integrating with the SMTP API can streamline the process of sending automated emails, newsletters, and notifications directly from web applications. This integration is particularly beneficial for applications that require robust email capabilities, such as e-commerce platforms, customer relationship management systems, and content management systems.
In this article, we will explore how to send messages using the SMTP API in PHP, providing you with the necessary steps and code examples to implement this integration effectively.
Setting Up a Test Environment for SMTP Integration in PHP
Before you can start sending messages using the SMTP API in PHP, it's crucial to set up a test environment. This allows you to safely experiment with the integration without affecting your production environment. Here's how you can get started:
Create a Test Email Account
To begin, you'll need a test email account. This can be created using any email service provider that supports SMTP. Ensure that the account is configured to allow SMTP access, as this will be necessary for sending emails programmatically.
Configure SMTP Settings
Once you have your test email account, gather the following SMTP settings from your email provider:
- SMTP Server: The address of the SMTP server (e.g., smtp.example.com).
- Port: The port number used for SMTP communication (commonly 587 or 465 for secure connections).
- Username: Your email address or username for authentication.
- Password: The password associated with your email account.
- Encryption: The type of encryption used (e.g., TLS or SSL).
Install PHP Mailer Library
To interact with the SMTP server in PHP, you'll need to use a library like PHPMailer. This library simplifies the process of sending emails via SMTP. You can install it using Composer:
composer require phpmailer/phpmailer
Configure PHPMailer for SMTP
With PHPMailer installed, you can now configure it to use your SMTP settings. Create a PHP script and include the following code:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
// SMTP server configuration
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = 'your-email@example.com';
$mail->Password = 'your-password';
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
// Sender and recipient settings
$mail->setFrom('your-email@example.com', 'Your Name');
$mail->addAddress('recipient@example.com', 'Recipient Name');
// Email content
$mail->isHTML(true);
$mail->Subject = 'Test Email';
$mail->Body = 'This is a test email sent using PHPMailer.';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
Replace the placeholders with your actual SMTP settings and email addresses. This script will send a test email to verify that your SMTP configuration is working correctly.
Verify the Test Email
After running the script, check the recipient's inbox to ensure the test email was delivered successfully. If you encounter any errors, double-check your SMTP settings and ensure that your email account allows SMTP access.
Executing SMTP API Calls in PHP: Step-by-Step Guide
To effectively send messages using the SMTP API in PHP, you'll need to ensure your development environment is properly configured. This section will guide you through the process of making SMTP API calls using PHP, focusing on the necessary code and configurations.
Understanding PHP Version and Dependencies for SMTP Integration
Before diving into the code, ensure that your PHP environment is up-to-date. It's recommended to use PHP 7.4 or later for optimal performance and compatibility with libraries like PHPMailer. Additionally, ensure that Composer is installed to manage dependencies efficiently.
Installing and Configuring PHPMailer for SMTP API Calls
PHPMailer is a popular library for sending emails via SMTP in PHP. If you haven't already installed it, use Composer to add PHPMailer to your project:
composer require phpmailer/phpmailer
Once installed, you can configure PHPMailer with your SMTP settings. Below is a sample PHP script to send an email using the SMTP API:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
// SMTP server configuration
$mail->isSMTP();
$mail->Host = 'smtp.example.com'; // Replace with your SMTP server
$mail->SMTPAuth = true;
$mail->Username = 'your-email@example.com'; // Replace with your SMTP username
$mail->Password = 'your-password'; // Replace with your SMTP password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587; // Common SMTP port
// Sender and recipient settings
$mail->setFrom('your-email@example.com', 'Your Name');
$mail->addAddress('recipient@example.com', 'Recipient Name');
// Email content
$mail->isHTML(true);
$mail->Subject = 'Test Email';
$mail->Body = 'This is a test email sent using PHPMailer.';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
Replace the placeholders with your actual SMTP server details and email addresses. This script demonstrates how to configure PHPMailer to send an email through your SMTP server.
Verifying Successful SMTP API Calls in PHP
After executing the script, check the recipient's inbox to confirm the delivery of the test email. If the email is not received, review the error message provided by PHPMailer and ensure that your SMTP settings are correct.
Handling Errors and Debugging SMTP API Calls
Errors during SMTP API calls can occur due to incorrect configurations or network issues. PHPMailer provides detailed error messages that can help you diagnose and resolve issues. Common errors include authentication failures and incorrect server addresses. Ensure your SMTP credentials are accurate and that your server allows SMTP connections.
Conclusion: Best Practices for SMTP API Integration in PHP
Successfully integrating the SMTP API in PHP requires careful attention to configuration details and best practices. Here are some recommendations to ensure a smooth and efficient integration:
Securely Storing SMTP Credentials
Always store your SMTP credentials securely. Use environment variables or secure vaults to keep sensitive information like usernames and passwords out of your codebase. This practice helps prevent unauthorized access and protects your application from security breaches.
Handling SMTP Rate Limiting
Be mindful of any rate limits imposed by your SMTP provider. Sending too many emails in a short period can lead to throttling or temporary bans. Implement logic to manage email sending rates, such as queuing messages or using exponential backoff strategies.
Transforming and Standardizing Email Data
Ensure that the data you send via SMTP is properly formatted and standardized. This includes using consistent email templates, encoding special characters, and validating email addresses before sending. Proper data handling improves deliverability and reduces the risk of errors.
Utilizing Endgrate for Simplified Integration
For developers looking to streamline their integration processes, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including SMTP services. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while ensuring a seamless integration experience for your users.
Visit Endgrate to learn more about how you can optimize your integration efforts and enhance your application's capabilities.
Read More
Ready to get started?