Using the Asana API to Get Tasks (with Javascript examples)
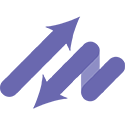
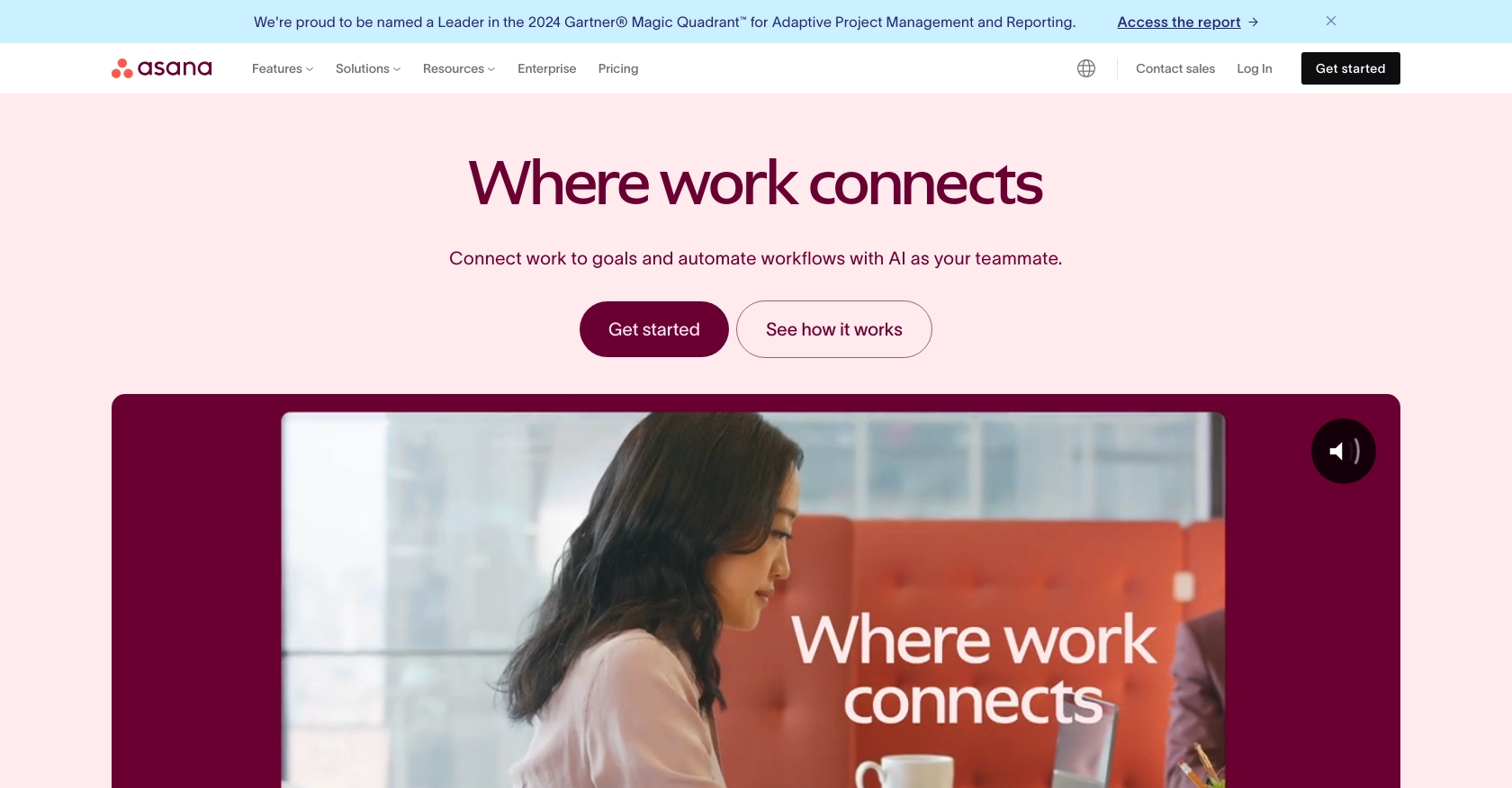
Introduction to Asana API Integration
Asana is a powerful project management tool that helps teams organize, track, and manage their work. With its user-friendly interface and robust features, Asana is widely used by businesses to enhance productivity and collaboration.
Integrating with the Asana API allows developers to automate and streamline workflows by interacting with tasks, projects, and other resources programmatically. For example, a developer might use the Asana API to retrieve tasks and automate reporting or synchronize tasks with another system, enhancing efficiency and reducing manual effort.
Setting Up Your Asana Developer Account and Sandbox Environment
Before you can start interacting with the Asana API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integrations without affecting live data.
Creating an Asana Developer Account
If you don't already have an Asana account, you can sign up for a free account on the Asana website. Once you have an account, you'll need to access the developer console to create your app and manage authentication.
Setting Up OAuth Authentication for Asana API
Asana uses OAuth for authentication, which is ideal for applications that require multiple users to sign in. Follow these steps to set up OAuth:
- Navigate to the Asana Developer Console.
- Click on "Create App" to start a new application.
- Fill in the required details such as the app name and redirect URL.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating a Personal Access Token (PAT) for Testing
For initial testing, you can use a Personal Access Token (PAT) to authenticate API requests. This is a simpler method for development purposes:
- In the Asana Developer Console, navigate to the "My Apps" section.
- Select "Create a Personal Access Token" and follow the prompts.
- Copy the generated token and store it securely. This token will allow you to make API calls on behalf of your account.
Using the Asana Developer Sandbox
To test premium features or ensure your integration works as expected, consider using the Asana Developer Sandbox:
- Request access to the sandbox environment through the Asana Developer Console.
- Use the sandbox to experiment with API calls without impacting your live data.
With your developer account, OAuth setup, and sandbox environment ready, you're all set to start making API calls to Asana. In the next section, we'll explore how to use JavaScript to interact with the Asana API and retrieve tasks.
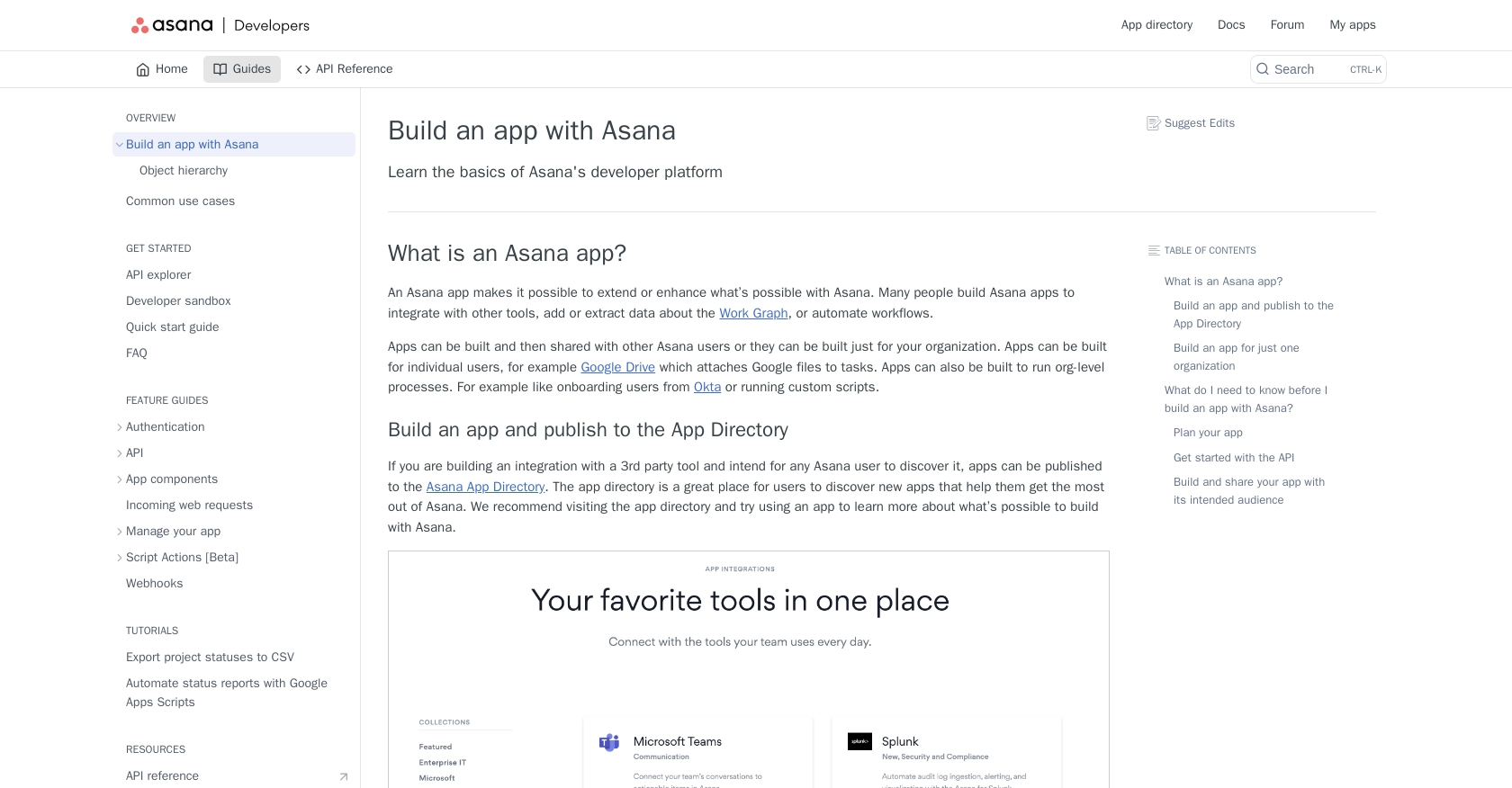
sbb-itb-96038d7
Making API Calls to Retrieve Asana Tasks Using JavaScript
To interact with the Asana API and retrieve tasks, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Asana API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Retrieve Tasks from Asana
With your environment set up, you can now write the JavaScript code to make an API call to Asana and retrieve tasks. Here's a step-by-step guide:
const axios = require('axios');
// Set your Asana Personal Access Token
const token = 'Your_Personal_Access_Token';
// Define the API endpoint for retrieving tasks
const endpoint = 'https://app.asana.com/api/1.0/tasks';
// Configure the request headers
const headers = {
'Authorization': `Bearer ${token}`
};
// Make a GET request to the Asana API
axios.get(endpoint, { headers })
.then(response => {
// Handle successful response
console.log('Tasks retrieved successfully:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error retrieving tasks:', error.response.data);
});
Replace Your_Personal_Access_Token
with the token you generated earlier. This script uses the axios
library to send a GET request to the Asana API, retrieving tasks associated with your account.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of tasks printed to the console. This confirms that the API call was successful. If you encounter errors, check the following:
- Ensure your Personal Access Token is correct and has the necessary permissions.
- Verify that the API endpoint URL is accurate.
- Check for network connectivity issues.
For more detailed error information, refer to the Asana API documentation on error handling.
Handling Asana API Rate Limits
Asana imposes rate limits on API requests to ensure fair usage. If you exceed these limits, you'll receive a 429 status code. To handle rate limiting:
- Implement exponential backoff to retry requests after a delay.
- Monitor your application's request rate and adjust as needed.
For more information on rate limits, consult the Asana API documentation.
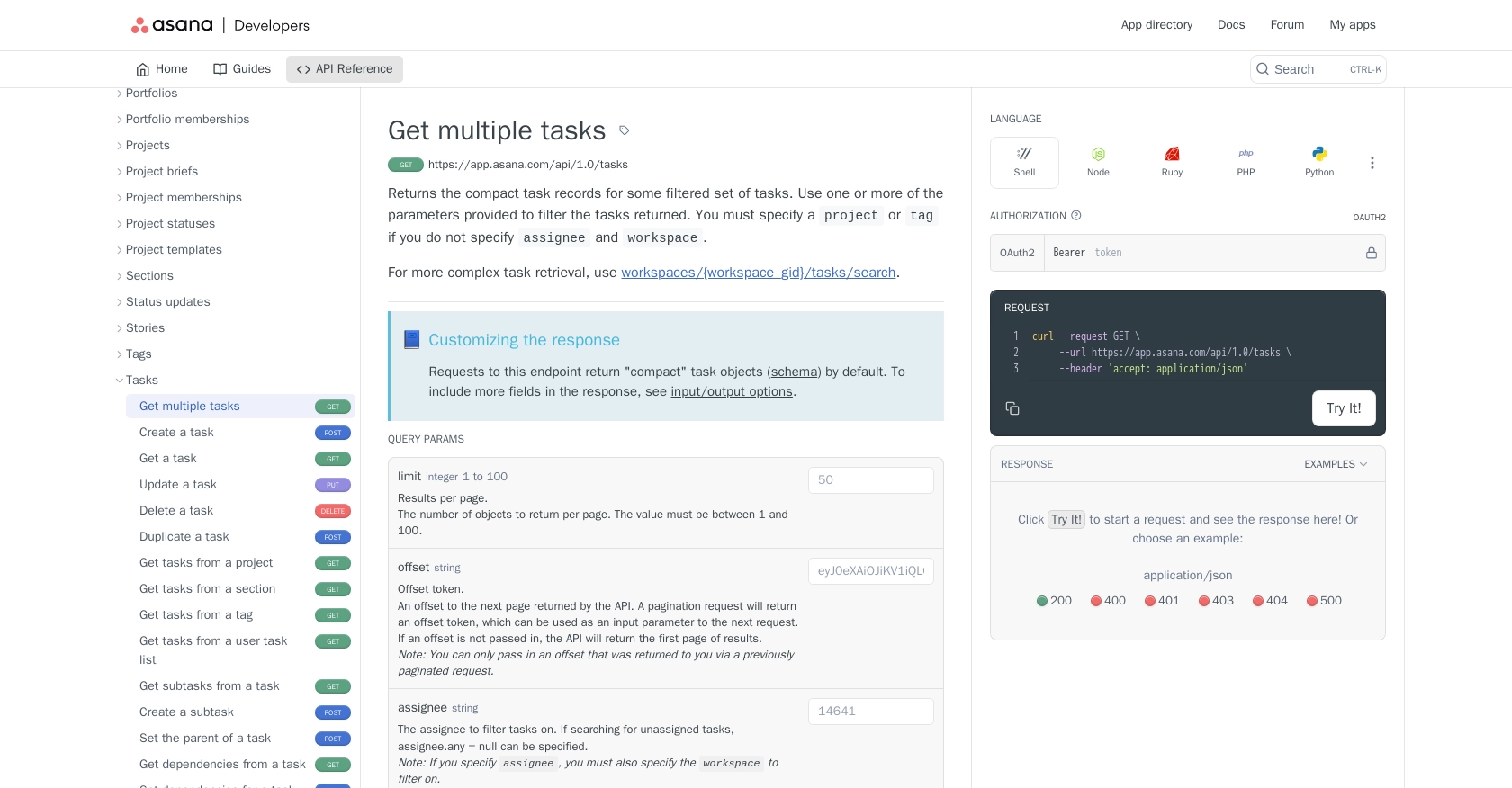
Conclusion and Best Practices for Asana API Integration
Integrating with the Asana API using JavaScript offers a powerful way to automate and enhance your project management workflows. By retrieving tasks programmatically, you can streamline reporting, synchronize data with other systems, and reduce manual effort, ultimately boosting productivity and efficiency.
Best Practices for Secure and Efficient Asana API Usage
- Secure Storage of Credentials: Always store your Personal Access Tokens and OAuth credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits Gracefully: Implement strategies like exponential backoff to manage rate limits effectively. This ensures your application remains responsive even when limits are reached.
- Data Standardization: When integrating with multiple systems, standardize data fields to maintain consistency and avoid discrepancies across platforms.
Leverage Endgrate for Seamless Integration
While building integrations with Asana can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint for multiple platforms, including Asana. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover how you can build once and deploy across various platforms effortlessly.
Read More
Ready to get started?