Using the Sugar Market API to Create Or Update Contacts (with PHP examples)
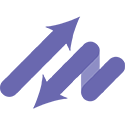
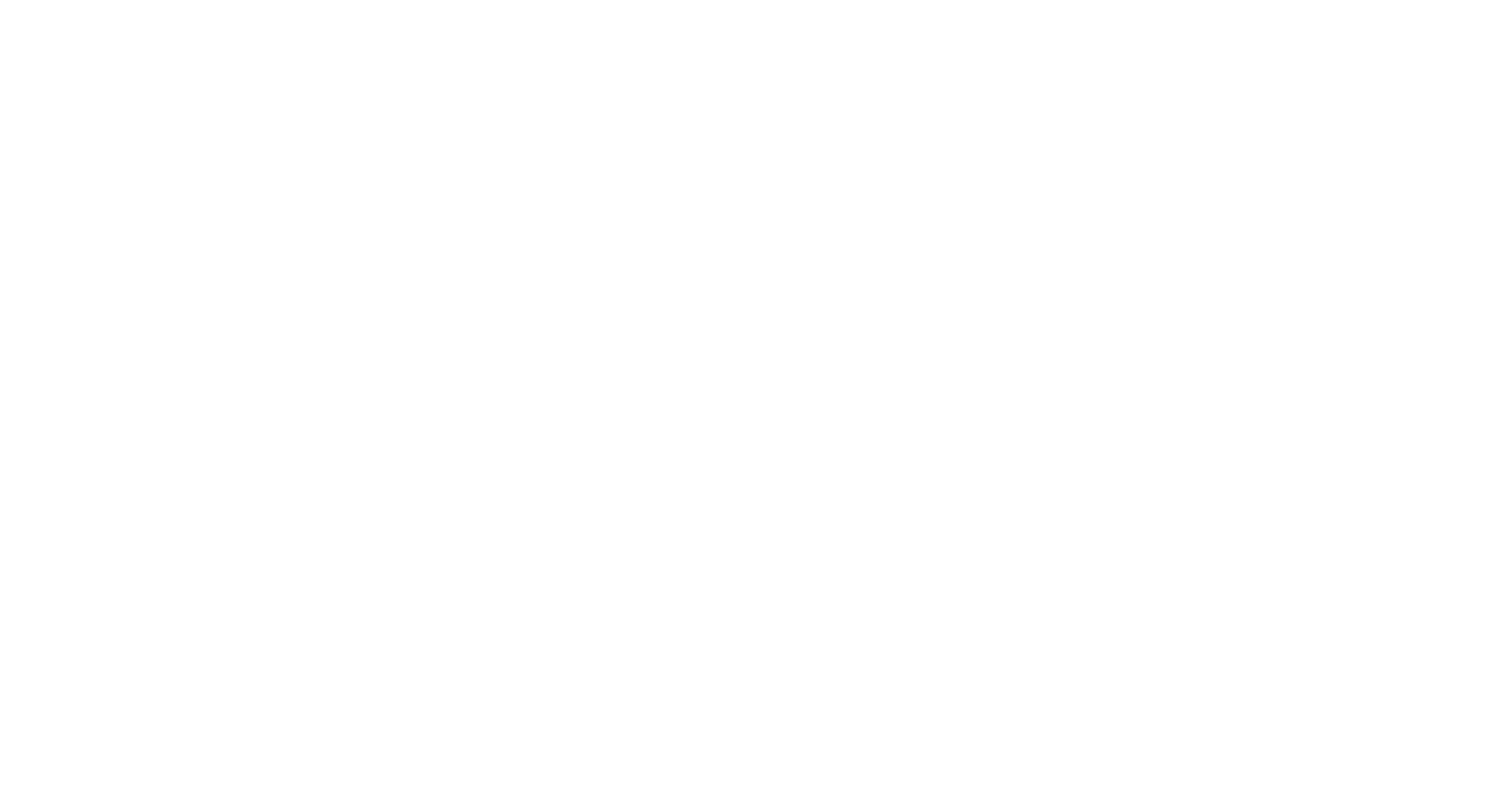
Introduction to Sugar Market
Sugar Market is a powerful marketing automation platform designed to enhance the marketing efforts of B2B businesses. It offers a comprehensive suite of tools for email marketing, lead nurturing, and campaign management, enabling companies to streamline their marketing processes and drive better results.
Developers may want to integrate with the Sugar Market API to automate the management of contact data, such as creating or updating contact information. For example, a developer could use the Sugar Market API to synchronize contact details from an external CRM system, ensuring that marketing campaigns are always targeting the most up-to-date audience.
Setting Up Your Sugar Market Test or Sandbox Account
Before you can start using the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data. Follow these steps to get started:
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the platform's features and allow you to test API interactions.
- Visit the Sugar Market website and navigate to the sign-up page.
- Fill in the required information to create your account.
- Once your account is created, log in to access the Sugar Market dashboard.
Generating API Credentials for Sugar Market
To interact with the Sugar Market API, you'll need to generate API credentials. Sugar Market uses a custom authentication method, so follow these steps to obtain the necessary credentials:
- Log in to your Sugar Market account and navigate to the API settings section.
- Locate the option to create a new API application or token.
- Follow the prompts to generate your client ID and client secret. These will be used to authenticate your API requests.
- Store these credentials securely, as you'll need them for making API calls.
Configuring OAuth for Sugar Market API
Although Sugar Market uses custom authentication, you may need to configure OAuth settings for certain integrations. Here's how to set it up:
- In the API settings, find the OAuth configuration section.
- Register your application by providing necessary details such as redirect URI and application name.
- Save the configuration to obtain your OAuth client ID and secret.
With your Sugar Market account and API credentials set up, you're ready to start making API calls to create or update contacts using PHP. In the next section, we'll dive into the specifics of making these API requests.
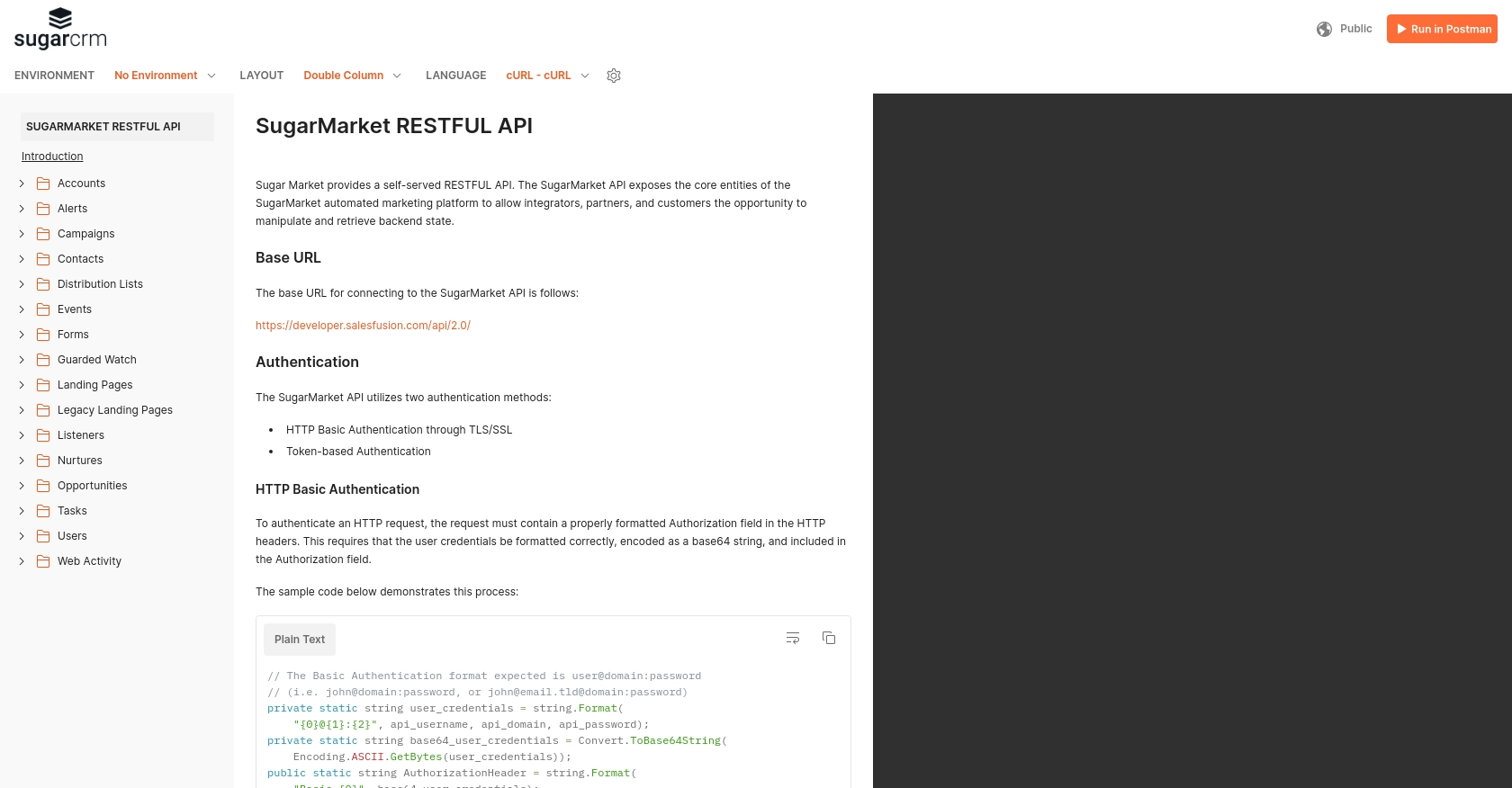
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Sugar Market Using PHP
With your Sugar Market account and API credentials ready, you can now proceed to make API calls to create or update contacts. This section will guide you through the process using PHP, ensuring you have the right setup and code to interact with the Sugar Market API effectively.
Setting Up Your PHP Environment for Sugar Market API Integration
Before diving into the code, ensure your PHP environment is properly configured. You'll need the following:
- PHP 7.4 or later
- cURL extension enabled
To check if cURL is enabled, you can create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL section in the output to confirm it's enabled.
Creating or Updating Contacts with Sugar Market API Using PHP
Now, let's write the PHP code to create or update contacts in Sugar Market. You'll use the cURL library to make HTTP requests to the API.
<?php
// Define your API endpoint
$endpoint = "https://api.sugarmarket.com/contacts";
// Set your API credentials
$client_id = "Your_Client_ID";
$client_secret = "Your_Client_Secret";
// Prepare the contact data
$contactData = [
"email" => "example@domain.com",
"firstName" => "John",
"lastName" => "Doe"
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Authorization: Basic " . base64_encode("$client_id:$client_secret")
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($contactData));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse the response
$responseData = json_decode($response, true);
if (isset($responseData['success']) && $responseData['success']) {
echo "Contact created/updated successfully.";
} else {
echo "Failed to create/update contact.";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Client_ID
and Your_Client_Secret
with your actual Sugar Market API credentials. This script initializes a cURL session, sets the necessary headers and data, and executes the request to create or update a contact.
Verifying API Call Success in Sugar Market
After running the script, you can verify the success of your API call by checking the Sugar Market dashboard. Navigate to the contacts section to see if the contact has been created or updated as expected.
Handling Errors and Troubleshooting API Calls
If you encounter errors, ensure that:
- Your API credentials are correct and have the necessary permissions.
- The API endpoint URL is accurate.
- Your PHP environment meets the requirements.
Refer to the Sugar Market API documentation for detailed error codes and troubleshooting tips.
Conclusion and Best Practices for Sugar Market API Integration
Integrating with the Sugar Market API allows developers to efficiently manage contact data, enhancing marketing automation efforts. By following the steps outlined in this guide, you can successfully create or update contacts using PHP, ensuring your marketing campaigns are always targeting the right audience.
Best Practices for Secure and Efficient Sugar Market API Usage
- Securely Store Credentials: Always store your API credentials securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sugar Market API to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Standardize Data Fields: Ensure that contact data fields are standardized to maintain consistency across different systems and integrations.
- Monitor API Usage: Regularly monitor your API usage and logs to quickly identify and resolve any issues or anomalies.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Sugar Market can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
By leveraging Endgrate, you can build once for each use case and connect to multiple platforms effortlessly. This not only saves time and resources but also provides an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?