Using the PipelineCRM API to Get People in PHP
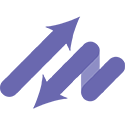
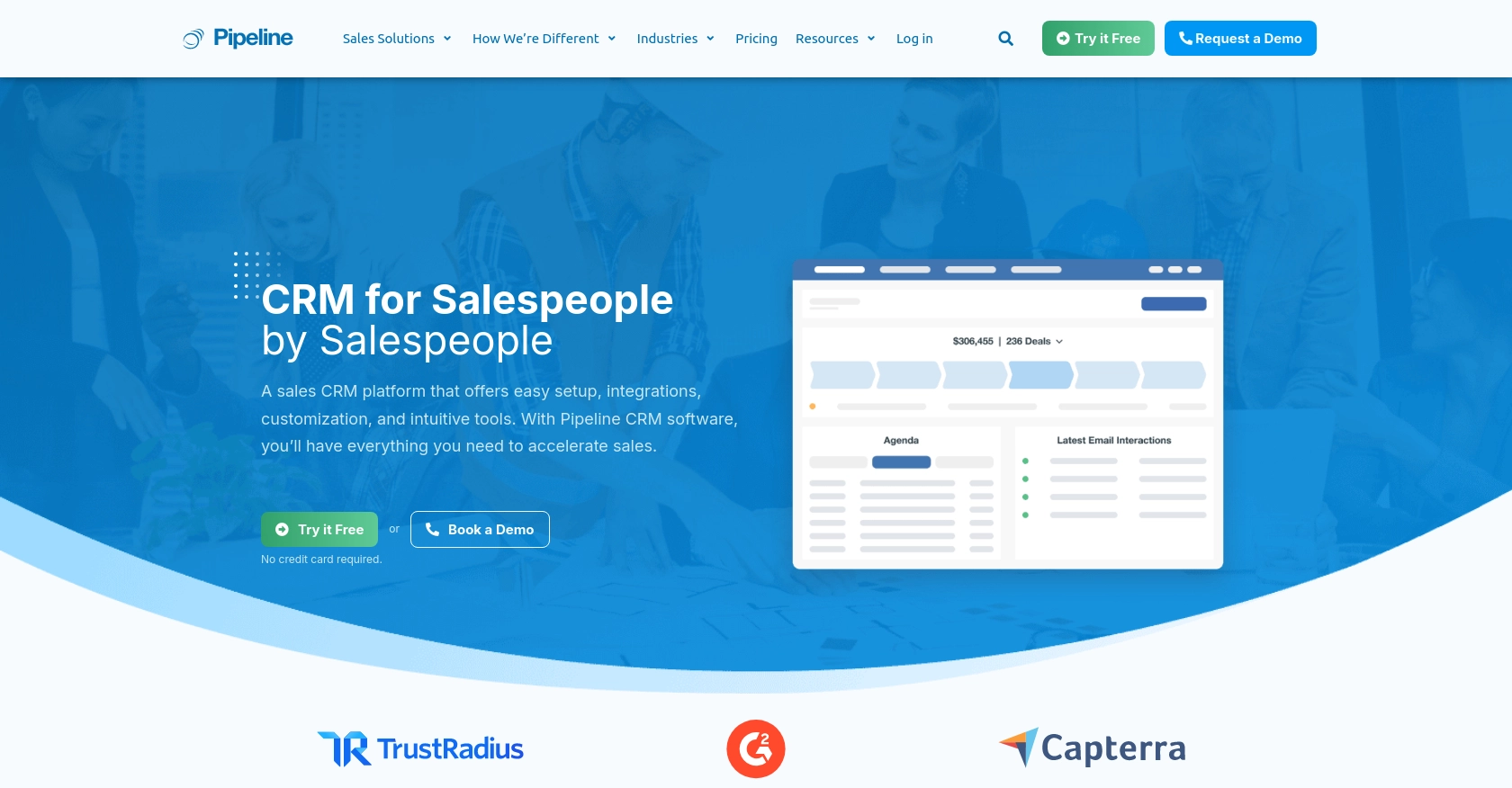
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes more effectively. With its user-friendly interface and powerful features, PipelineCRM enables companies to track leads, manage contacts, and streamline their sales pipeline.
Developers may want to integrate with PipelineCRM's API to automate and enhance their sales operations. For example, using the PipelineCRM API, a developer can retrieve and manage contact information, allowing for seamless synchronization of customer data across different platforms.
This article will guide you through the process of using PHP to interact with the PipelineCRM API, specifically focusing on retrieving people data. By following this tutorial, you'll be able to efficiently access and manage contact information within the PipelineCRM platform.
Setting Up Your PipelineCRM Test Account
Before you can start interacting with the PipelineCRM API using PHP, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting any live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test the API integration.
- Visit the PipelineCRM website.
- Follow the instructions to create a new account.
- Once your account is set up, log in to access the dashboard.
Generating an API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the settings section, usually found in the top-right corner of the dashboard.
- Look for the API settings or integrations tab.
- Generate a new API key and make sure to copy it. You'll need this key to authenticate your API requests.
Keep your API key secure and do not share it publicly, as it provides access to your account's data.
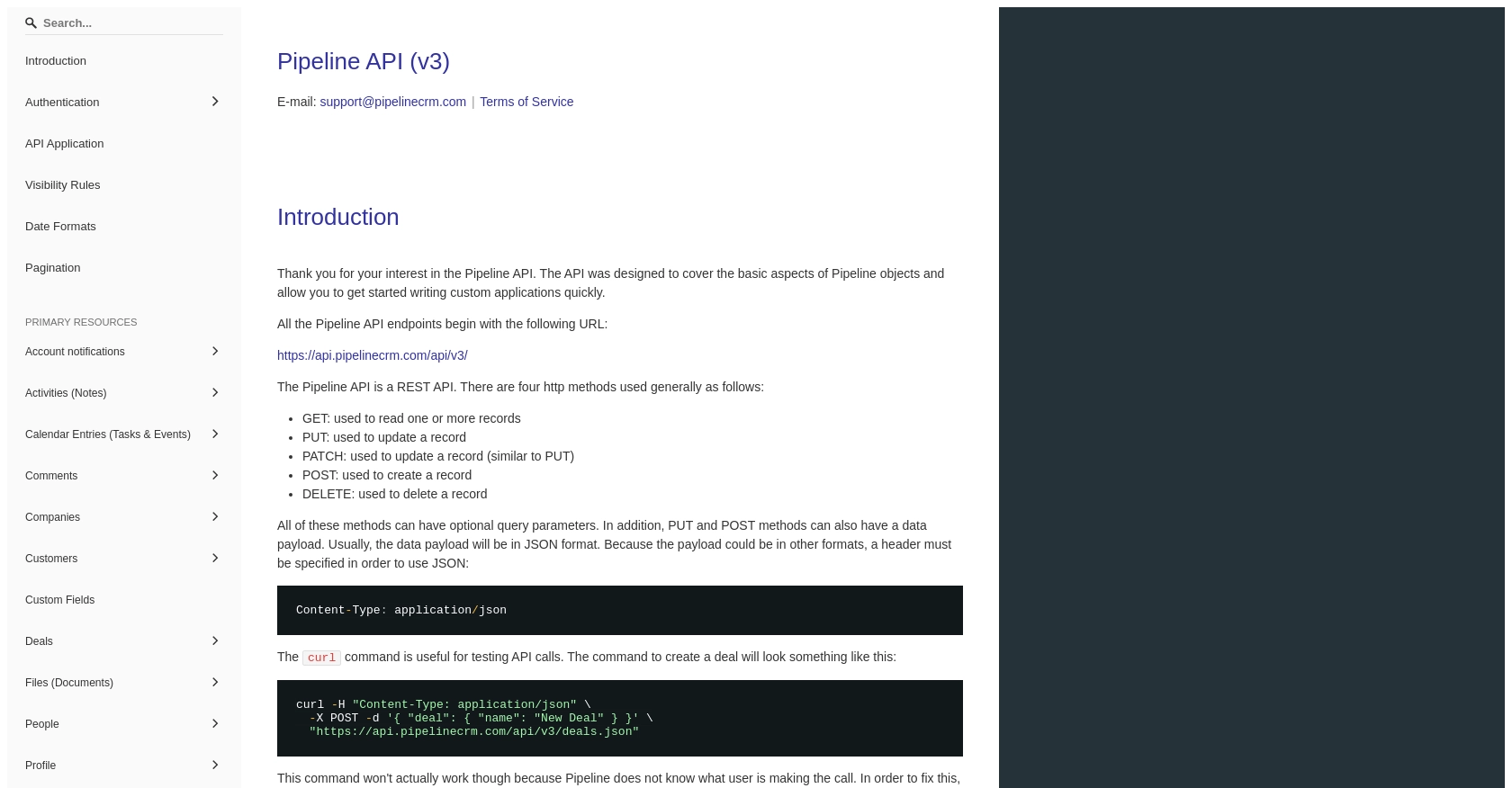
sbb-itb-96038d7
Making API Calls to Retrieve People Data from PipelineCRM Using PHP
To interact with the PipelineCRM API using PHP, you'll need to set up your environment and write code to make the necessary API calls. This section will guide you through the process of retrieving people data from PipelineCRM.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer (for managing dependencies)
You'll also need to install the GuzzleHTTP
library, a popular PHP HTTP client, to handle API requests. You can install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve People Data from PipelineCRM
Once your environment is set up, you can write a PHP script to make a GET request to the PipelineCRM API and retrieve people data. Create a file named get_pipelinecrm_people.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$apiKey = 'Your_API_Key'; // Replace with your actual API key
$client = new Client();
try {
$response = $client->request('GET', 'https://api.pipelinecrm.com/api/v3/people', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $person) {
echo 'Name: ' . $person['first_name'] . ' ' . $person['last_name'] . "<br>";
echo 'Email: ' . $person['email'] . "<br><br>";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script uses Guzzle to send a GET request to the PipelineCRM API endpoint for people data. It then decodes the JSON response and prints out the names and emails of the people retrieved.
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_pipelinecrm_people.php
If successful, you should see a list of people with their names and emails printed in the terminal. This confirms that the API call was successful and the data was retrieved correctly.
Handling Errors and Troubleshooting
While making API calls, you may encounter errors. Common issues include:
- Invalid API key: Ensure your API key is correct and has the necessary permissions.
- Network issues: Check your internet connection and ensure the API endpoint is accessible.
- Rate limiting: PipelineCRM may impose rate limits on API requests. If you receive a rate limit error, consider implementing a retry mechanism or reducing the frequency of requests.
For more detailed error information, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for Using PipelineCRM API with PHP
Integrating with the PipelineCRM API using PHP can significantly enhance your ability to manage and automate customer relationship processes. By following the steps outlined in this guide, you can efficiently retrieve and handle people data within the PipelineCRM platform.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits. Implement retry mechanisms or exponential backoff strategies to handle rate limit errors gracefully. This ensures your application remains robust and responsive.
- Data Standardization: When retrieving data from the API, consider transforming and standardizing it to fit your application's data model. This can simplify data handling and integration with other systems.
Streamlining Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including PipelineCRM.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing a seamless experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?