Using the Zoho CRM API to Create or Update Companies in Javascript
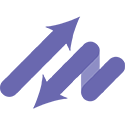
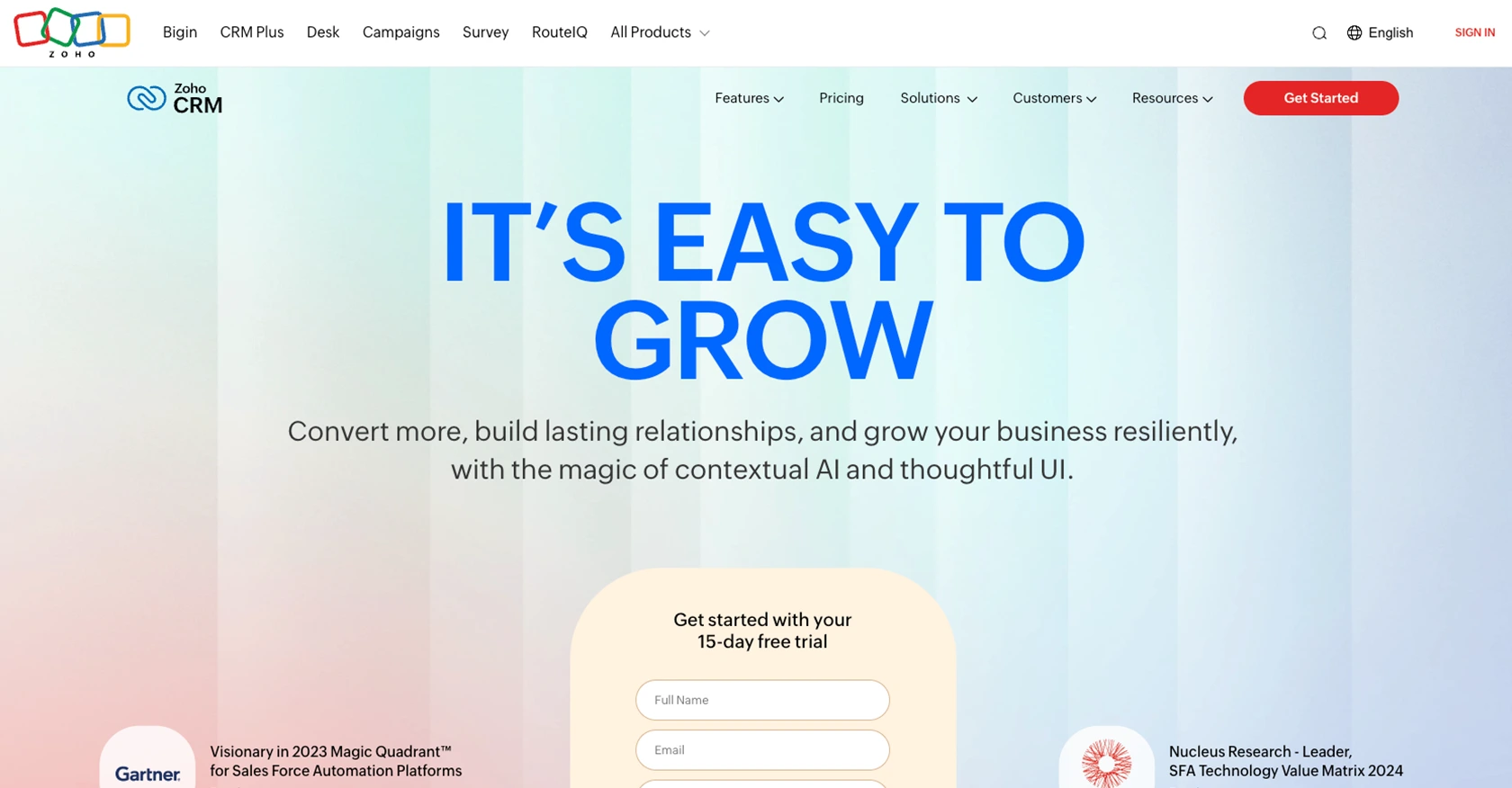
Introduction to Zoho CRM API for Company Management
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in one place. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Developers often integrate with Zoho CRM to automate and optimize various business processes. By leveraging the Zoho CRM API, developers can efficiently manage company data, such as creating or updating company records. This integration can be particularly useful for synchronizing data between Zoho CRM and other business applications, ensuring that company information is always up-to-date and consistent across platforms.
For example, a developer might use the Zoho CRM API to automatically update company details in Zoho CRM whenever changes are made in an external database, reducing manual data entry and minimizing errors.
Setting Up Your Zoho CRM Sandbox Account for API Integration
Before you can start integrating with the Zoho CRM API, you'll need to set up a sandbox account. This allows you to test your API interactions without affecting your live data. Zoho CRM provides a sandbox environment that mirrors your production setup, ensuring a seamless transition from testing to deployment.
Steps to Create a Zoho CRM Sandbox Account
- Sign Up for Zoho CRM: If you don't have a Zoho CRM account, visit the Zoho CRM website and sign up for a free trial or a demo account.
- Access the Developer Console: Log in to your Zoho CRM account and navigate to the Developer Console. This is where you can manage your API integrations and sandbox settings.
- Create a Sandbox: In the Developer Console, select the option to create a new sandbox. Follow the prompts to configure your sandbox environment, ensuring it reflects your production setup.
Configuring OAuth Authentication for Zoho CRM API
Zoho CRM uses OAuth 2.0 for secure API authentication. Follow these steps to set up OAuth for your application:
- Register Your Application: In the Developer Console, register your application by providing details such as the application name, homepage URL, and authorized redirect URIs. This will generate a Client ID and Client Secret.
-
Define Scopes: Specify the scopes your application needs. For managing company data, you might need scopes like
ZohoCRM.modules.accounts.ALL
. - Generate Access Tokens: Use the Client ID and Client Secret to request an access token. This token will be used to authenticate API requests. Refer to the Zoho CRM OAuth documentation for detailed instructions.
Testing API Calls in the Zoho CRM Sandbox
With your sandbox and OAuth setup complete, you can begin testing API calls. Use the sandbox environment to ensure your integration works as expected before deploying to production.
For more information on API limits and error handling, refer to the Zoho CRM API limits documentation.
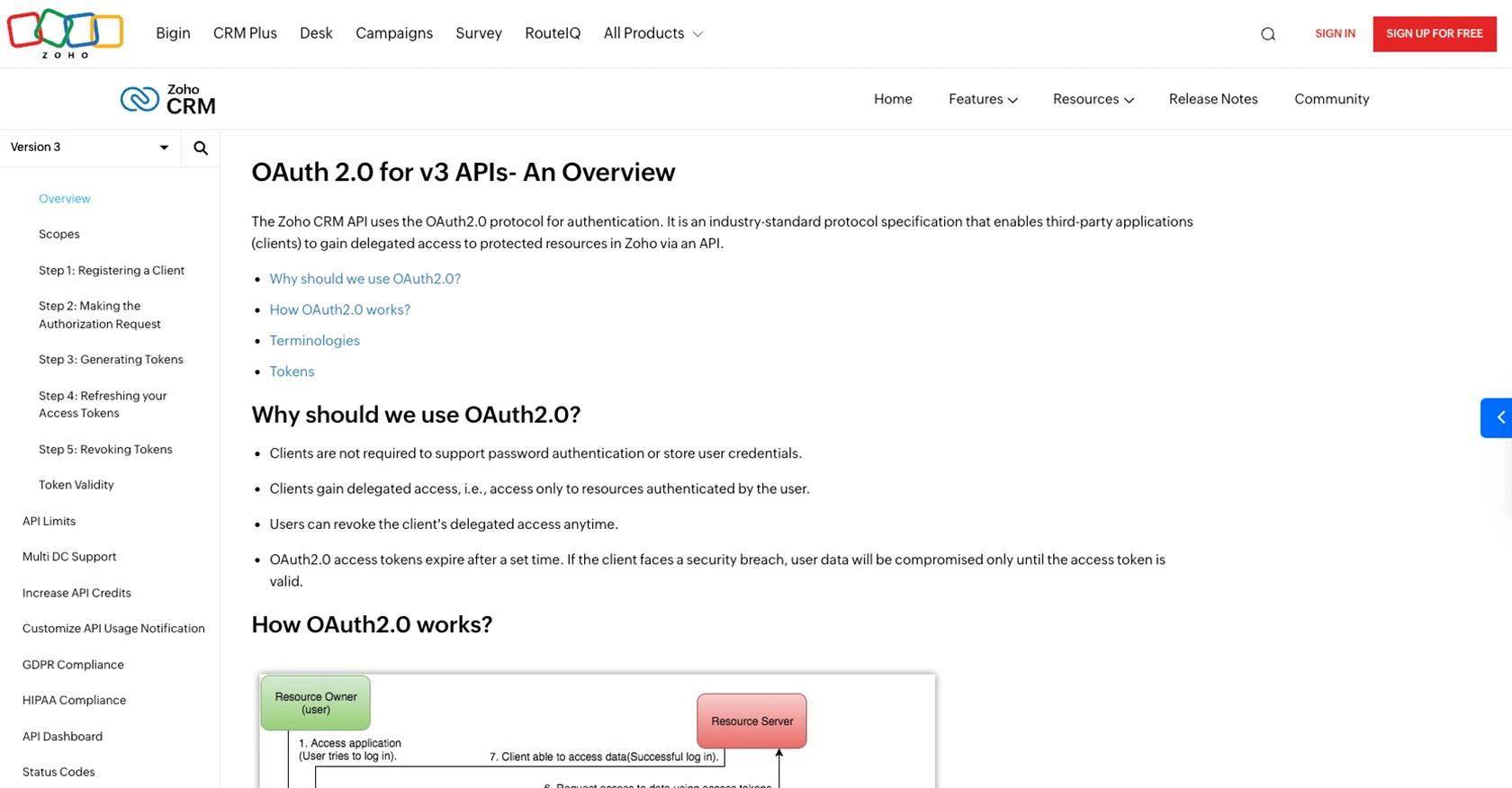
sbb-itb-96038d7
How to Make API Calls to Zoho CRM for Creating or Updating Companies Using JavaScript
To interact with the Zoho CRM API for creating or updating company records, you need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your JavaScript environment, installing necessary dependencies, and executing API calls to manage company data in Zoho CRM.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js: Install Node.js from the official website if you haven't already.
- npm: Node.js comes with npm, which you'll use to install packages.
Once Node.js is installed, create a new project directory and initialize it:
mkdir zoho-crm-integration
cd zoho-crm-integration
npm init -y
Installing Required Packages for Zoho CRM API Calls
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Making API Calls to Zoho CRM to Create or Update Company Records
With your environment set up, you can now write JavaScript code to interact with the Zoho CRM API. Below is an example of how to create or update a company record:
const axios = require('axios');
// Set your access token and API endpoint
const accessToken = 'your_access_token';
const endpoint = 'https://www.zohoapis.com/crm/v3/Accounts';
// Function to create or update a company
async function upsertCompany(companyData) {
try {
const response = await axios.post(endpoint, {
data: [companyData]
}, {
headers: {
'Authorization': `Zoho-oauthtoken ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Response:', response.data);
} catch (error) {
console.error('Error:', error.response ? error.response.data : error.message);
}
}
// Example company data
const companyData = {
Account_Name: 'Example Company',
Website: 'https://example.com',
Industry: 'Technology'
};
// Call the function to create or update the company
upsertCompany(companyData);
Replace your_access_token
with the access token you generated during the OAuth setup. The companyData
object should contain the fields you wish to update or create in Zoho CRM.
Verifying Successful API Requests in Zoho CRM Sandbox
After executing the API call, verify the changes in your Zoho CRM sandbox account. Navigate to the Accounts module to check if the company record has been created or updated as expected.
For error handling, refer to the Zoho CRM API status codes documentation to understand the response codes and troubleshoot any issues.
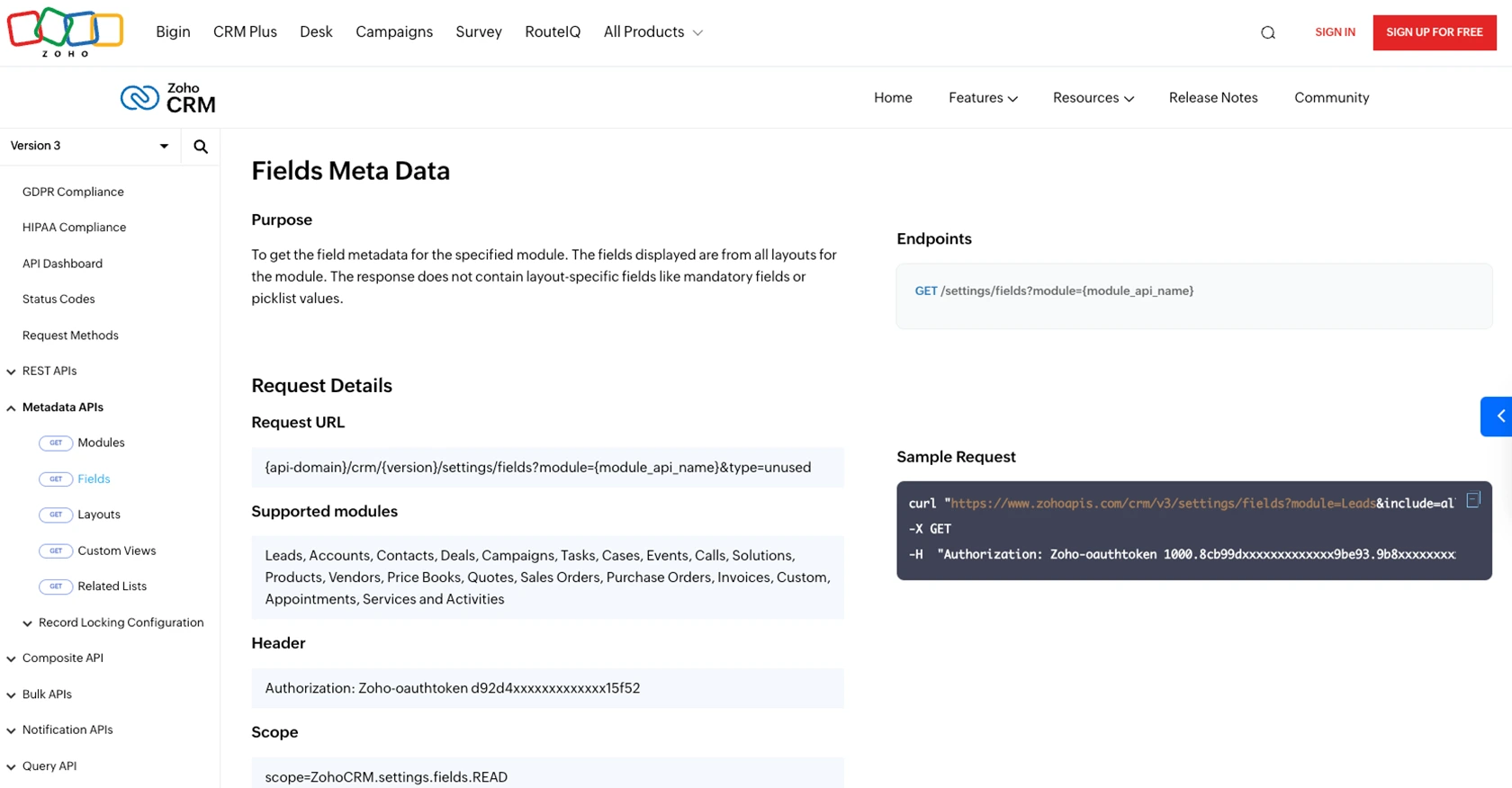
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API to manage company records can significantly enhance your business operations by ensuring data consistency and reducing manual entry. By following the steps outlined in this guide, you can efficiently create or update company information using JavaScript, leveraging the power of Zoho CRM's comprehensive platform.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Zoho CRM's API rate limits to avoid disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the API limits documentation.
- Data Standardization: Ensure that the data you send to Zoho CRM is standardized and validated to prevent errors and maintain data integrity across systems.
- Error Handling: Implement robust error handling by checking response codes and using the status codes documentation to troubleshoot issues.
Streamline Your Integration Process with Endgrate
While integrating with Zoho CRM can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can build once for each use case, saving time and resources while offering an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of outsourcing integrations to focus on your core product development.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?