How to Create or Update Customers with the Customer.io Track API in Python
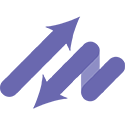
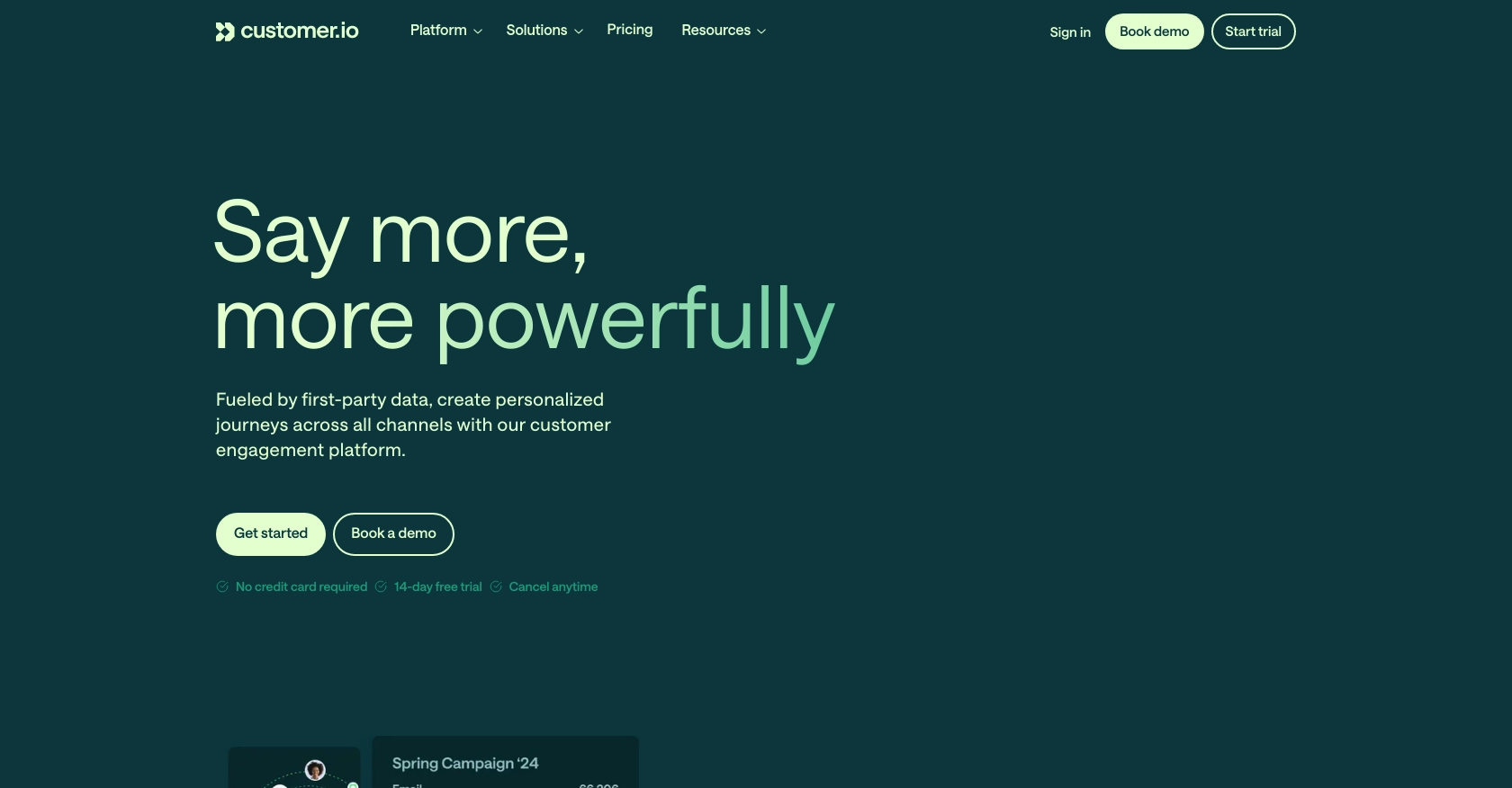
Introduction to Customer.io Track API
Customer.io is a powerful marketing automation platform that helps businesses send targeted messages to their customers. It provides tools for creating personalized email campaigns, managing customer data, and tracking user interactions.
Integrating with the Customer.io Track API allows developers to efficiently manage customer data and automate communication workflows. For example, you can use the API to create or update customer profiles in real-time, ensuring that your marketing campaigns are always based on the most current information.
Setting Up Your Customer.io Track API Test Account
Before you begin integrating with the Customer.io Track API, it's essential to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Customer.io Account
If you don't already have a Customer.io account, you can sign up for a free trial on the Customer.io website. This will give you access to the platform's features and allow you to test API integrations.
- Visit the Customer.io website and click on "Start Trial."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generate API Credentials
To interact with the Customer.io Track API, you'll need to generate API credentials. These credentials will include your Site ID and API key, which are necessary for authentication.
- Navigate to your account settings in the Customer.io dashboard.
- Locate the "API Keys" section and click on "Generate API Key."
- Copy your Site ID and API key. You'll need these for authenticating your API requests.
Understanding Customer.io Track API Authentication
The Customer.io Track API uses HTTP basic authentication. Your credentials are your Site ID and API key, Base-64 encoded in the format site_id:api_key
. This ensures secure communication between your application and the API.
Set Up a Test Environment
It's crucial to test your API calls in a controlled environment. You can use tools like Postman to simulate API requests and verify responses.
- Download and install Postman if you haven't already.
- Create a new request and set the method to POST or PUT, depending on your operation.
- Enter the Customer.io Track API endpoint URL.
- In the "Authorization" tab, select "Basic Auth" and enter your Site ID and API key.
- Test your API calls to ensure they work as expected.
By following these steps, you'll be ready to start integrating with the Customer.io Track API, allowing you to create or update customer profiles seamlessly.
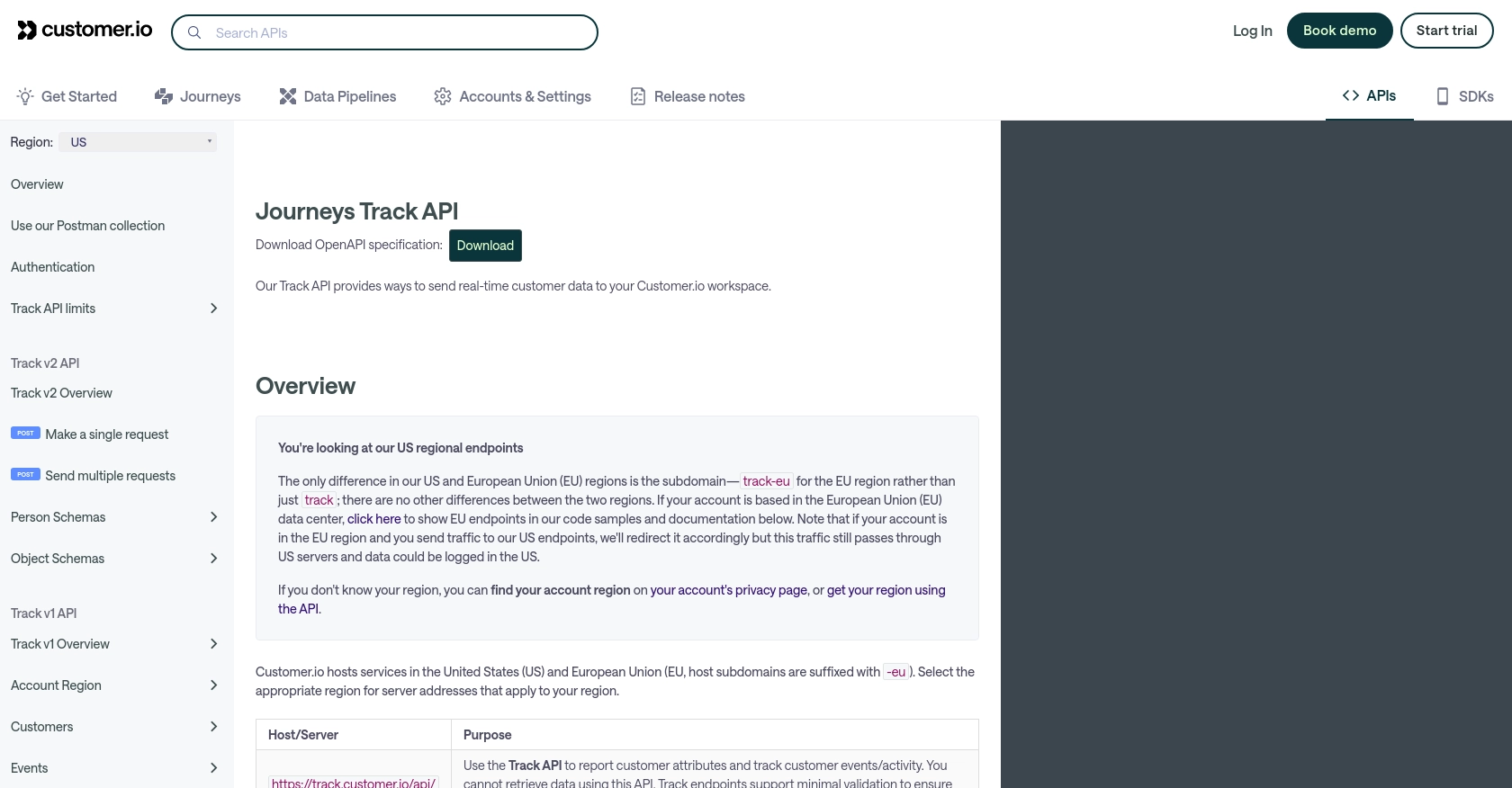
sbb-itb-96038d7
Making API Calls to Create or Update Customers with Customer.io Track API in Python
To interact with the Customer.io Track API using Python, you'll need to set up your environment and write code to make API requests. This section will guide you through the process of creating or updating customer profiles using Python.
Setting Up Your Python Environment for Customer.io Track API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Writing Python Code to Create or Update Customers
Now, let's write a Python script to create or update a customer profile using the Customer.io Track API. The following code demonstrates how to make a PUT request to the API endpoint.
import requests
import base64
# Set your Site ID and API Key
site_id = 'your_site_id'
api_key = 'your_api_key'
# Encode credentials for HTTP Basic Authentication
credentials = f"{site_id}:{api_key}"
encoded_credentials = base64.b64encode(credentials.encode()).decode()
# Define the API endpoint and headers
url = "https://track.customer.io/api/v1/customers/{identifier}"
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json"
}
# Define the customer data
customer_data = {
"email": "customer@example.com",
"created_at": 1361205308,
"first_name": "John",
"last_name": "Doe"
}
# Make the PUT request to create or update the customer
response = requests.put(url, json=customer_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Customer created or updated successfully.")
else:
print(f"Failed to create or update customer: {response.status_code} - {response.text}")
Verifying API Call Success in Customer.io Track
After running the script, verify that the customer profile was created or updated successfully by checking your Customer.io dashboard. If the request was successful, the customer data should reflect the changes.
Handling Errors and Understanding Response Codes
It's crucial to handle potential errors when making API calls. The Customer.io Track API may return various status codes:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: Authentication failed. Check your credentials.
Ensure you handle these responses appropriately in your application to provide a robust integration.
By following these steps, you can efficiently manage customer data using the Customer.io Track API in Python. This integration allows you to automate and streamline your marketing workflows with up-to-date customer information.
Conclusion and Best Practices for Using Customer.io Track API in Python
Integrating with the Customer.io Track API using Python provides a powerful way to manage customer data and automate marketing workflows. By following the steps outlined in this guide, you can create or update customer profiles efficiently, ensuring your marketing campaigns are always based on the most current information.
Best Practices for Secure and Efficient Customer.io Track API Integration
- Securely Store Credentials: Always store your Site ID and API key securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: The Customer.io Track API has a fair-use rate limit of 100 requests per second. Implement retry logic and exponential backoff to handle rate limiting gracefully.
- Validate API Responses: Always check the response status codes and handle errors appropriately. This ensures a robust integration that can handle unexpected issues.
- Standardize Data Fields: Ensure that customer data fields are standardized and validated before making API calls. This minimizes errors and ensures data consistency.
Streamline Your Integrations with Endgrate
While integrating with Customer.io Track API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Customer.io. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?