How to Get Contacts with the Cloze API in Javascript
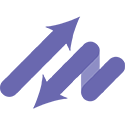
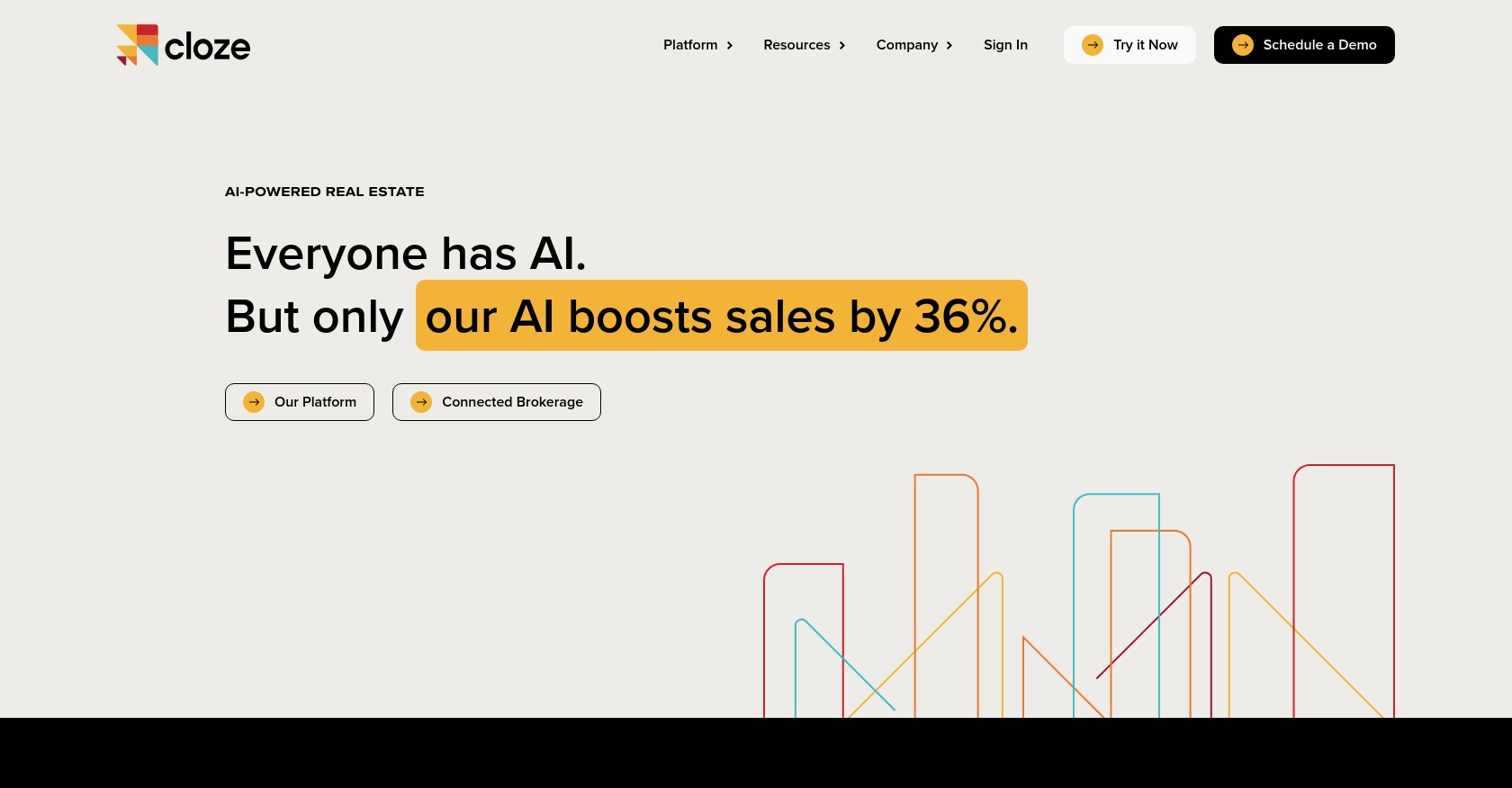
Introduction to Cloze API Integration
Cloze is a powerful relationship management platform that helps businesses manage their contacts, projects, and communications seamlessly. It offers a comprehensive suite of tools designed to enhance productivity and streamline workflows by integrating various aspects of customer relationship management.
Developers may want to integrate with the Cloze API to automate and enhance their contact management processes. For example, using the Cloze API, you can retrieve contact information to keep your CRM data synchronized with other business applications, ensuring that your team always has access to the most up-to-date information.
This article will guide you through the process of using JavaScript to interact with the Cloze API to retrieve contact data efficiently. By following this tutorial, you'll learn how to set up your environment, authenticate with the Cloze API, and execute API calls to manage your contacts effectively.
Setting Up Your Cloze API Test Account
Before you can begin integrating with the Cloze API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Cloze offers a straightforward process for creating a test environment, ensuring you have all the necessary credentials to access their API.
Creating a Cloze Account
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. This trial will give you access to the platform's features, allowing you to explore its capabilities and set up your test environment.
- Visit the Cloze website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access the Cloze dashboard.
Generating Your Cloze API Key
To authenticate your API requests, you'll need an API key. Follow these steps to generate one:
- Log in to your Cloze account and navigate to the settings page.
- Under the "Integrations" section, find the "API Keys" option.
- Click on "Create API Key" and provide a name for your key to identify it later.
- Copy the generated API key and keep it secure, as you'll need it for authentication in your API requests.
For more information on creating an API key, refer to the Cloze API documentation.
Configuring OAuth for Public Integrations
If you're developing a public integration intended for use by other Cloze users, you'll need to use OAuth for authentication. This involves setting up an OAuth app within Cloze:
- Contact Cloze support at support@cloze.com to get started with OAuth integration.
- Follow the instructions provided by Cloze support to configure your OAuth app, including setting up redirect URIs and obtaining client credentials.
With your test account and API key ready, you're now set to begin making API calls to the Cloze platform. In the next section, we'll explore how to use JavaScript to interact with the Cloze API and retrieve contact information.
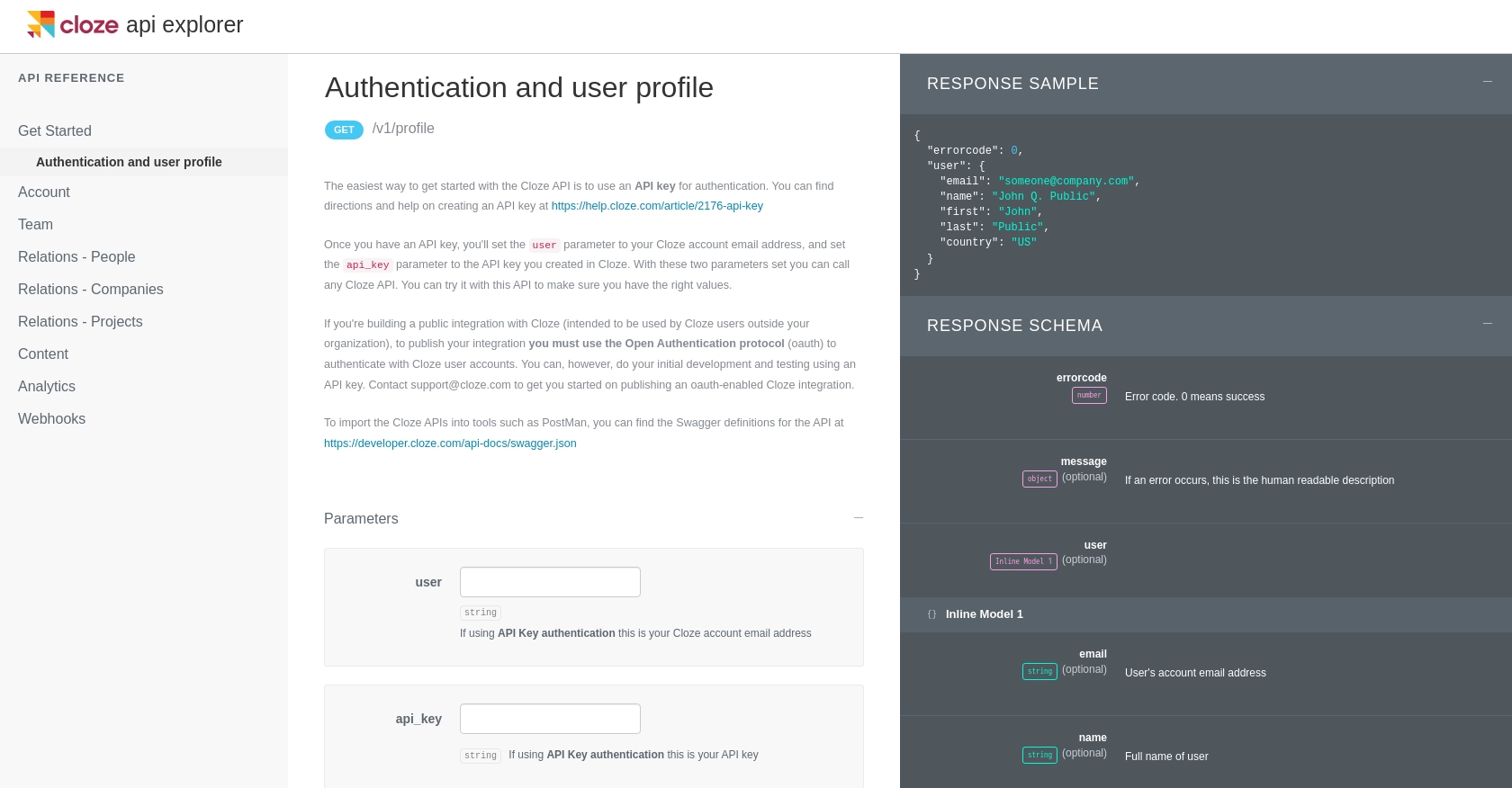
sbb-itb-96038d7
Making API Calls to Retrieve Contacts with Cloze API Using JavaScript
Now that you have your Cloze API key, you can start making API calls to retrieve contact information. In this section, we'll guide you through the process of setting up your JavaScript environment and executing API requests to interact with the Cloze API.
Setting Up Your JavaScript Environment for Cloze API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal.
Installing Required Dependencies for Cloze API Interaction
To make HTTP requests, you'll need the axios
library. Install it using npm:
npm install axios
Executing API Calls to Retrieve Contacts from Cloze
With your environment set up, you can now write JavaScript code to interact with the Cloze API. Below is an example of how to retrieve contacts using the API key for authentication.
const axios = require('axios');
// Define the API endpoint and your API key
const endpoint = 'https://api.cloze.com/v1/people/get';
const apiKey = 'Your_API_Key';
const userEmail = 'Your_Cloze_Email';
// Function to retrieve contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, {
params: {
user: userEmail,
api_key: apiKey
}
});
// Check if the request was successful
if (response.data.errorcode === 0) {
console.log('Contacts retrieved successfully:', response.data.people);
} else {
console.error('Error retrieving contacts:', response.data.message);
}
} catch (error) {
console.error('An error occurred:', error.message);
}
}
// Execute the function
getContacts();
Replace Your_API_Key
and Your_Cloze_Email
with your actual Cloze API key and email address.
Verifying Successful API Requests in Cloze
After executing the code, you should see a list of contacts printed in the console. To verify the request's success, check your Cloze dashboard to ensure the data matches the retrieved contacts.
Handling Errors and Understanding Cloze API Response Codes
It's crucial to handle errors gracefully. The Cloze API returns an errorcode
in the response. A value of 0 indicates success, while other values indicate errors. Always check this code to determine the request's outcome.
For more detailed error information, refer to the Cloze API documentation.
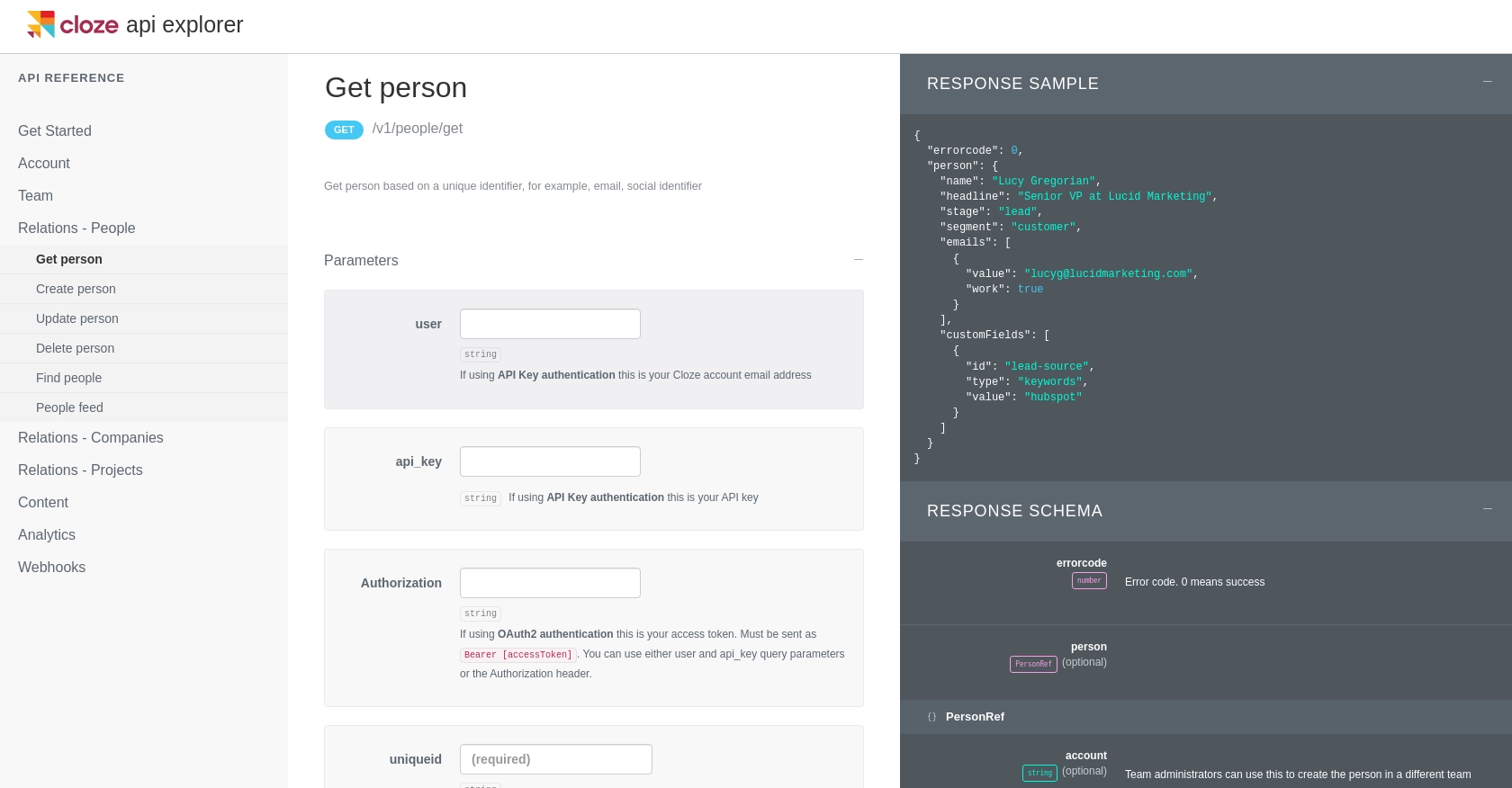
Conclusion and Best Practices for Cloze API Integration
Integrating with the Cloze API using JavaScript can significantly enhance your contact management processes by automating data synchronization and ensuring your team has access to the latest information. By following the steps outlined in this guide, you can efficiently retrieve contact data and integrate it with your existing systems.
Best Practices for Secure and Efficient Cloze API Usage
- Secure Storage of API Credentials: Always store your API key securely and avoid hardcoding it in your source files. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the Cloze API is transformed and standardized to match your application's data model.
Enhancing Your Integration Experience with Endgrate
While integrating with the Cloze API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Cloze. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?