Using the Zendesk Sell API to Get Leads in Python
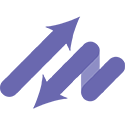
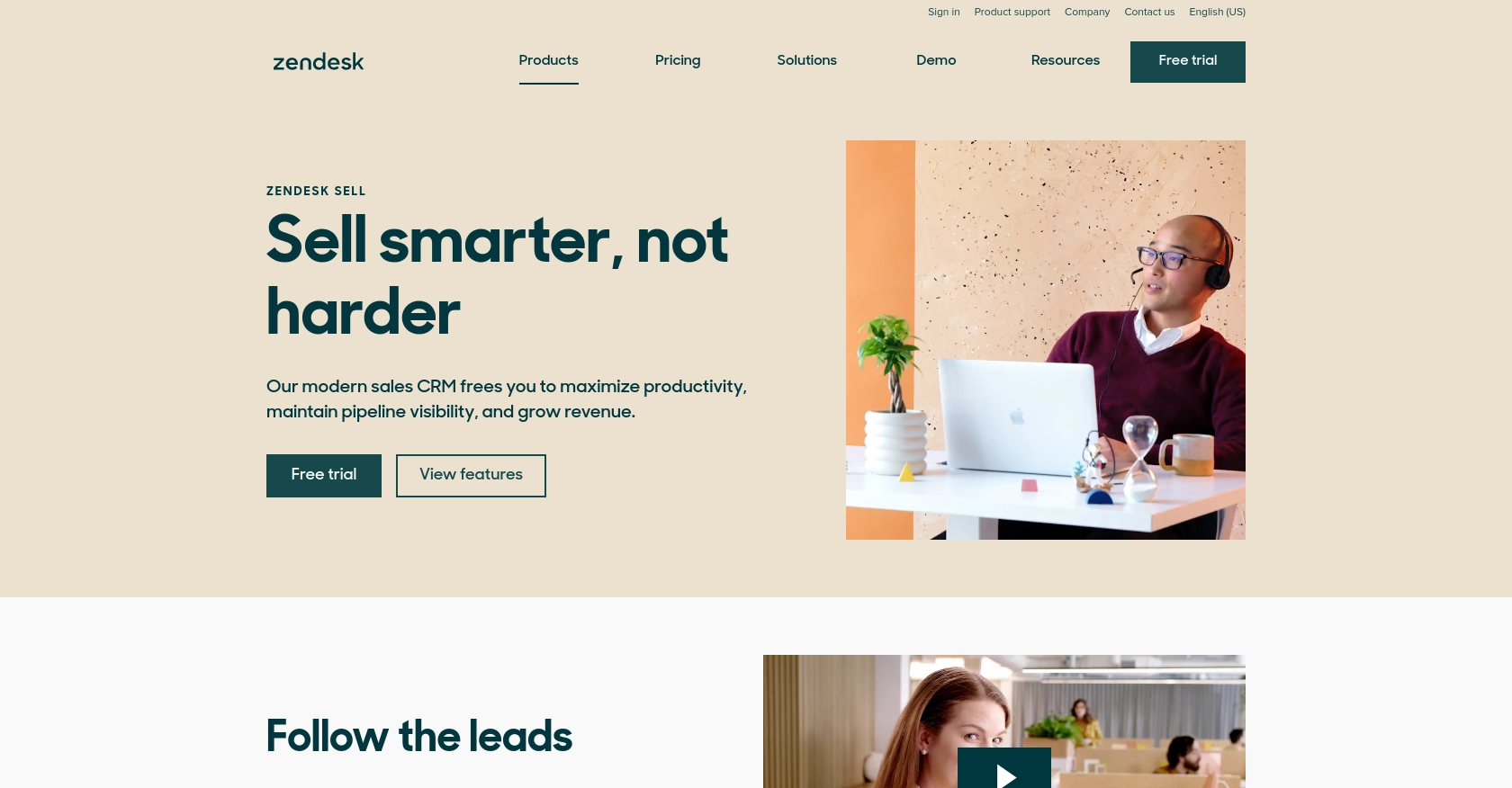
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools to manage leads, contacts, and deals, making it an essential tool for sales teams aiming to improve their efficiency and close more deals.
Integrating with the Zendesk Sell API allows developers to automate and optimize sales workflows. For example, by using the API, developers can programmatically retrieve leads and integrate them into other systems, such as marketing automation platforms, to create targeted campaigns and improve lead nurturing.
This article will guide you through the process of using Python to interact with the Zendesk Sell API, specifically focusing on retrieving leads. By following this tutorial, you will learn how to set up your environment, authenticate using OAuth 2.0, and make API calls to access lead data.
Setting Up Your Zendesk Sell Test Account
Before you can start interacting with the Zendesk Sell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Zendesk Sell offers a free trial that you can use to access the API and test your integrations.
Creating a Zendesk Sell Free Trial Account
To begin, visit the Zendesk Sell website and sign up for a free trial. Follow the on-screen instructions to create your account. Once your account is set up, you will have access to the Zendesk Sell dashboard where you can manage your leads, contacts, and other sales data.
Registering Your Application for OAuth 2.0 Authentication
Zendesk Sell uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials. Follow these steps to register your application:
- Log in to your Zendesk Sell account and navigate to the Settings section.
- Under Integrations, select API & Apps.
- Click on Create App and fill in the required details such as the app name and description.
- Once your app is created, you will receive a Client ID and Client Secret. Make sure to store these securely as they will be used for authentication.
Configuring OAuth 2.0 for Zendesk Sell API Access
With your application registered, you can now configure OAuth 2.0 to authenticate API requests. Use the following steps to set up OAuth 2.0:
- Direct users to the authorization URL to obtain an authorization code:
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate your API requests.
https://api.getbase.com/oauth2/authorize?client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
curl -X POST https://api.getbase.com/oauth2/token \
-u "YOUR_CLIENT_ID:YOUR_CLIENT_SECRET" \
-d "grant_type=authorization_code" \
-d "code=AUTHORIZATION_CODE" \
-d "redirect_uri=YOUR_REDIRECT_URI"
By completing these steps, you will have a fully configured test environment to interact with the Zendesk Sell API using OAuth 2.0 authentication. This setup ensures that you can safely develop and test your integrations with Zendesk Sell.
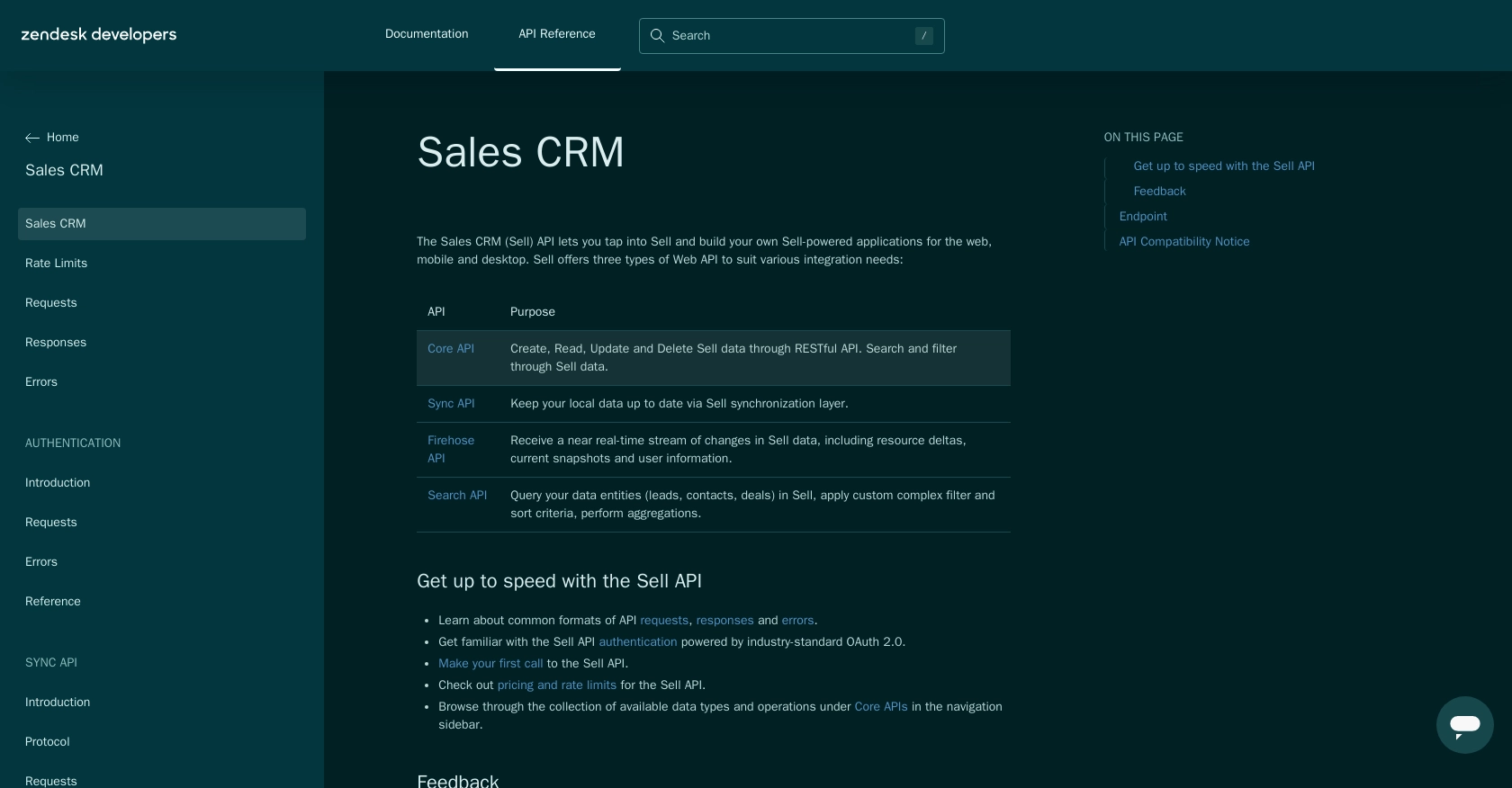
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Zendesk Sell Using Python
To interact with the Zendesk Sell API and retrieve leads, you'll need to set up your Python environment and make authenticated API requests. This section will guide you through the necessary steps to achieve this.
Setting Up Your Python Environment for Zendesk Sell API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.x
- The Python package manager
pip
Install the requests
library, which will be used to make HTTP requests to the Zendesk Sell API:
pip install requests
Writing Python Code to Retrieve Leads from Zendesk Sell API
Create a Python script named get_zendesk_leads.py
and add the following code to it:
import requests
# Set the API endpoint and headers
endpoint = "https://api.getbase.com/v2/leads"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
leads = response.json()
for lead in leads["items"]:
print(f"Lead ID: {lead['data']['id']}, Name: {lead['data']['first_name']} {lead['data']['last_name']}")
else:
print(f"Failed to retrieve leads: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authentication process.
Running the Python Script to Access Zendesk Sell Leads
Execute the script from your terminal or command line:
python get_zendesk_leads.py
If successful, the script will output a list of leads with their IDs and names. If there is an error, it will display the error code and message.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. The Zendesk Sell API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: The access token is missing or invalid.
- 429 Too Many Requests: The rate limit has been exceeded. You can make up to 36,000 requests per hour (10 requests/token/second). See Zendesk Sell Rate Limits for more details.
For a complete list of error codes, refer to the Zendesk Sell API Errors Documentation.
By following these steps, you can successfully retrieve leads from the Zendesk Sell API using Python, allowing you to integrate this data into your applications and enhance your sales processes.
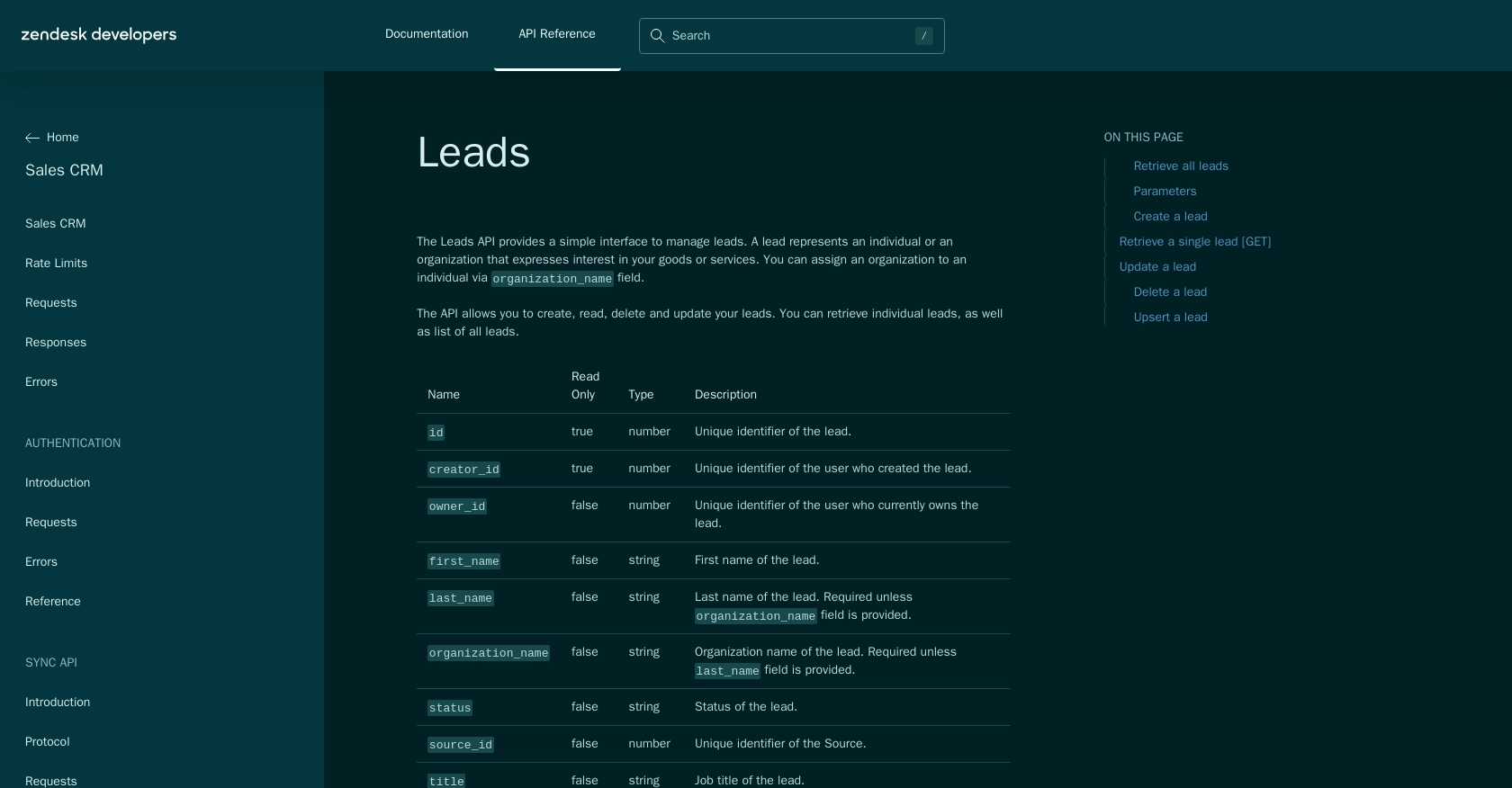
Best Practices for Using Zendesk Sell API in Python
When integrating with the Zendesk Sell API, it's crucial to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as the Client ID, Client Secret, and access tokens, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the rate limits imposed by Zendesk Sell. You can make up to 36,000 requests per hour (10 requests/token/second). Implement logic to handle the
429 Too Many Requests
error by retrying after a delay. For more information, refer to the Zendesk Sell Rate Limits documentation. - Data Transformation and Standardization: When retrieving leads, ensure that the data is transformed and standardized to fit your application's requirements. This may involve mapping fields or converting data types.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Log errors for further analysis and provide meaningful feedback to users when issues arise.
Enhance Your Integration Strategy with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including Zendesk Sell. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/leads/
Ready to get started?