How to Create or Update organizations with the Capsule API in PHP
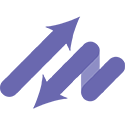
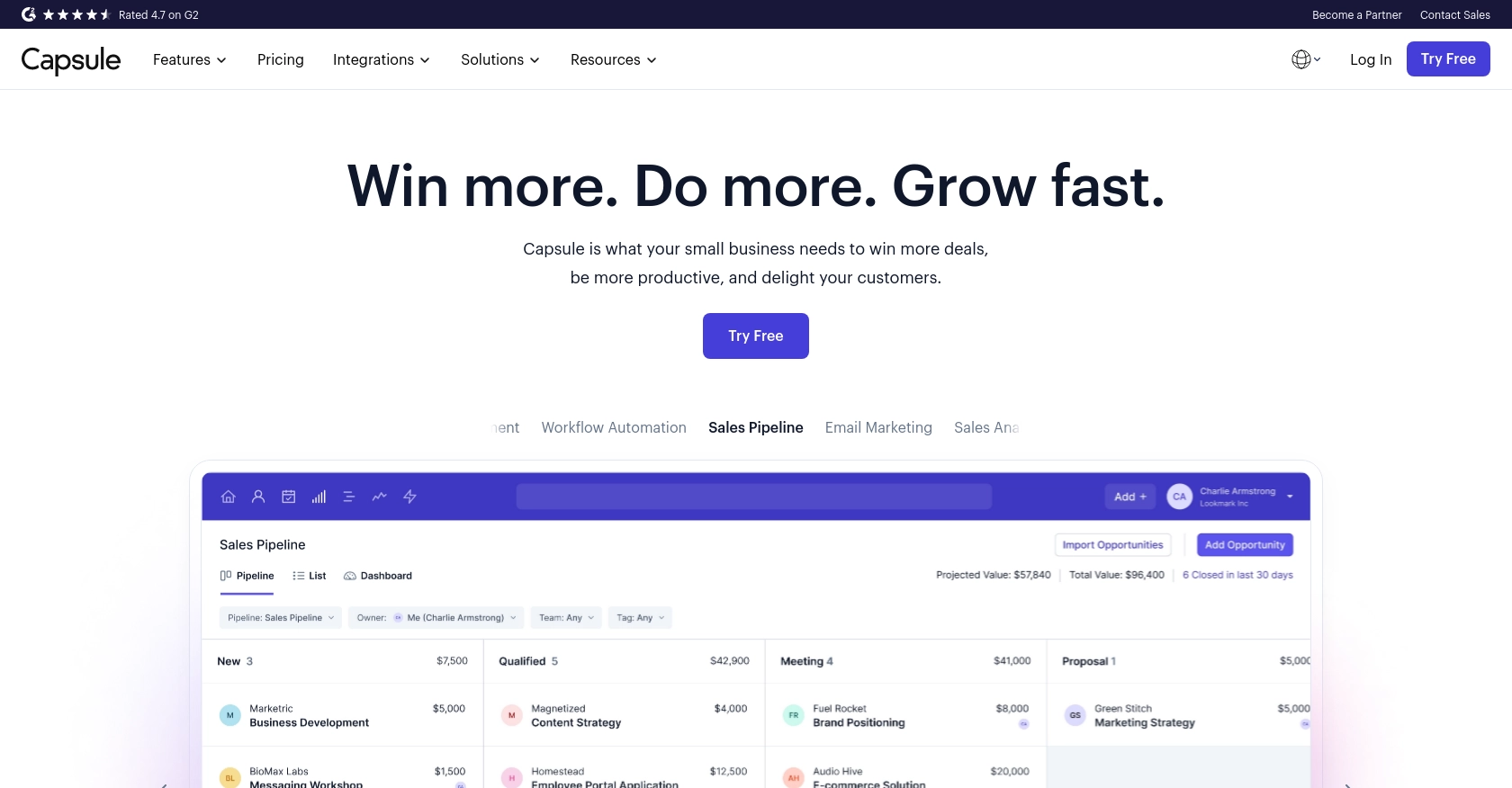
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions efficiently. With its user-friendly interface and powerful features, Capsule CRM is an ideal choice for businesses looking to streamline their CRM processes.
Integrating with Capsule's API allows developers to automate and enhance CRM functionalities. For example, you can create or update organization records directly from your application, ensuring that your CRM data is always up-to-date and accurate. This integration can be particularly useful for syncing data between Capsule and other business applications, reducing manual data entry and improving data consistency.
Setting Up a Capsule CRM Test or Sandbox Account
Before you can start integrating with the Capsule API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Capsule provides a straightforward way to create a sandbox environment through their free trial or demo accounts.
Creating a Capsule CRM Account
To begin, visit the Capsule CRM signup page and register for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you'll have access to the Capsule dashboard where you can manage your CRM data.
Configuring OAuth Authentication for Capsule API
Capsule API uses OAuth 2.0 for authentication, which requires you to create an application within your Capsule account. This process will provide you with a client ID and client secret necessary for API access.
- Log in to your Capsule account and navigate to My Preferences.
- Select API Authentication Tokens from the menu.
- Click on Create New Application and fill in the required details, such as the application name and redirect URI.
- Once the application is created, note down the client ID and client secret. These will be used to authenticate API requests.
Generating a Bearer Token
With your client ID and client secret, you can now generate a bearer token to authenticate your API requests. Follow these steps:
- Redirect users to the Capsule authorization URL to obtain an authorization code:
- After the user authorizes your application, Capsule will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the returned access token securely, as it will be used in the Authorization header for API requests.
GET https://api.capsulecrm.com/oauth/authorise?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=read%20write
POST https://api.capsulecrm.com/oauth/token
{
"code": "AUTHORIZATION_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"grant_type": "authorization_code"
}
For more details on authentication, refer to the Capsule API Authentication documentation.
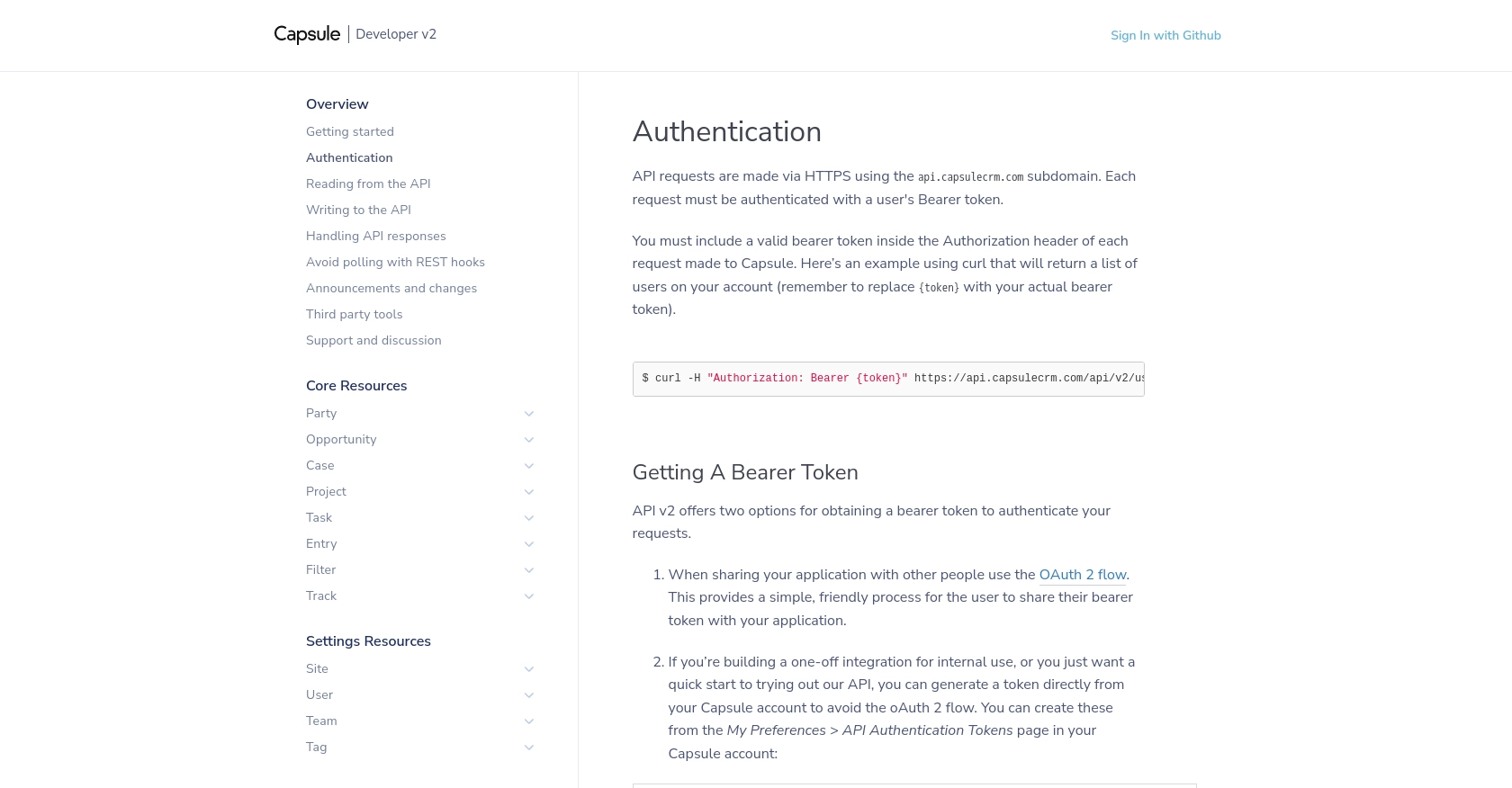
sbb-itb-96038d7
Making API Calls to Create or Update Organizations with Capsule API in PHP
Setting Up Your PHP Environment for Capsule API Integration
To interact with the Capsule API using PHP, ensure you have PHP 7.4 or later installed on your system. Additionally, you'll need the cURL
extension enabled to make HTTP requests. You can verify this by checking your php.ini
file or using phpinfo()
.
Install any necessary dependencies using Composer, the PHP package manager. For this tutorial, we'll use the guzzlehttp/guzzle
library for making HTTP requests:
composer require guzzlehttp/guzzle
Creating an Organization with Capsule API
To create an organization in Capsule CRM, you'll need to make a POST request to the Capsule API's /parties
endpoint. The request must include the organization details in JSON format.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.capsulecrm.com/api/v2/parties', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'party' => [
'type' => 'organisation',
'name' => 'New Organization',
'about' => 'Description of the organization',
'emailAddresses' => [
['type' => 'Work', 'address' => 'info@neworganization.com']
],
'addresses' => [
['type' => 'Office', 'city' => 'New York', 'country' => 'USA']
]
]
]
]);
echo $response->getBody();
Replace YOUR_ACCESS_TOKEN
with the token you obtained during the authentication setup. This code creates a new organization with specified details. Upon success, the API returns the created organization's data.
Updating an Existing Organization in Capsule CRM
To update an existing organization, use the PUT method on the /parties/{partyId}
endpoint. You'll need the organization's ID and the updated data.
$response = $client->put('https://api.capsulecrm.com/api/v2/parties/{partyId}', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'party' => [
'about' => 'Updated description of the organization',
'emailAddresses' => [
['id' => 12345, 'type' => 'Work', 'address' => 'contact@neworganization.com']
]
]
]
]);
echo $response->getBody();
Replace {partyId}
with the actual ID of the organization you wish to update. The API will return the updated organization's details upon a successful request.
Handling API Responses and Errors
Capsule API responses include HTTP status codes to indicate success or failure. A 2xx status code means the request was successful, while 4xx or 5xx codes indicate errors. Here's how you can handle these responses:
try {
$response = $client->post('https://api.capsulecrm.com/api/v2/parties', [/* request options */]);
if ($response->getStatusCode() === 201) {
echo "Organization created successfully!";
}
} catch (GuzzleHttp\Exception\ClientException $e) {
echo "Request failed: " . $e->getResponse()->getBody();
}
For more detailed error handling, refer to the Capsule API Handling API Responses documentation.
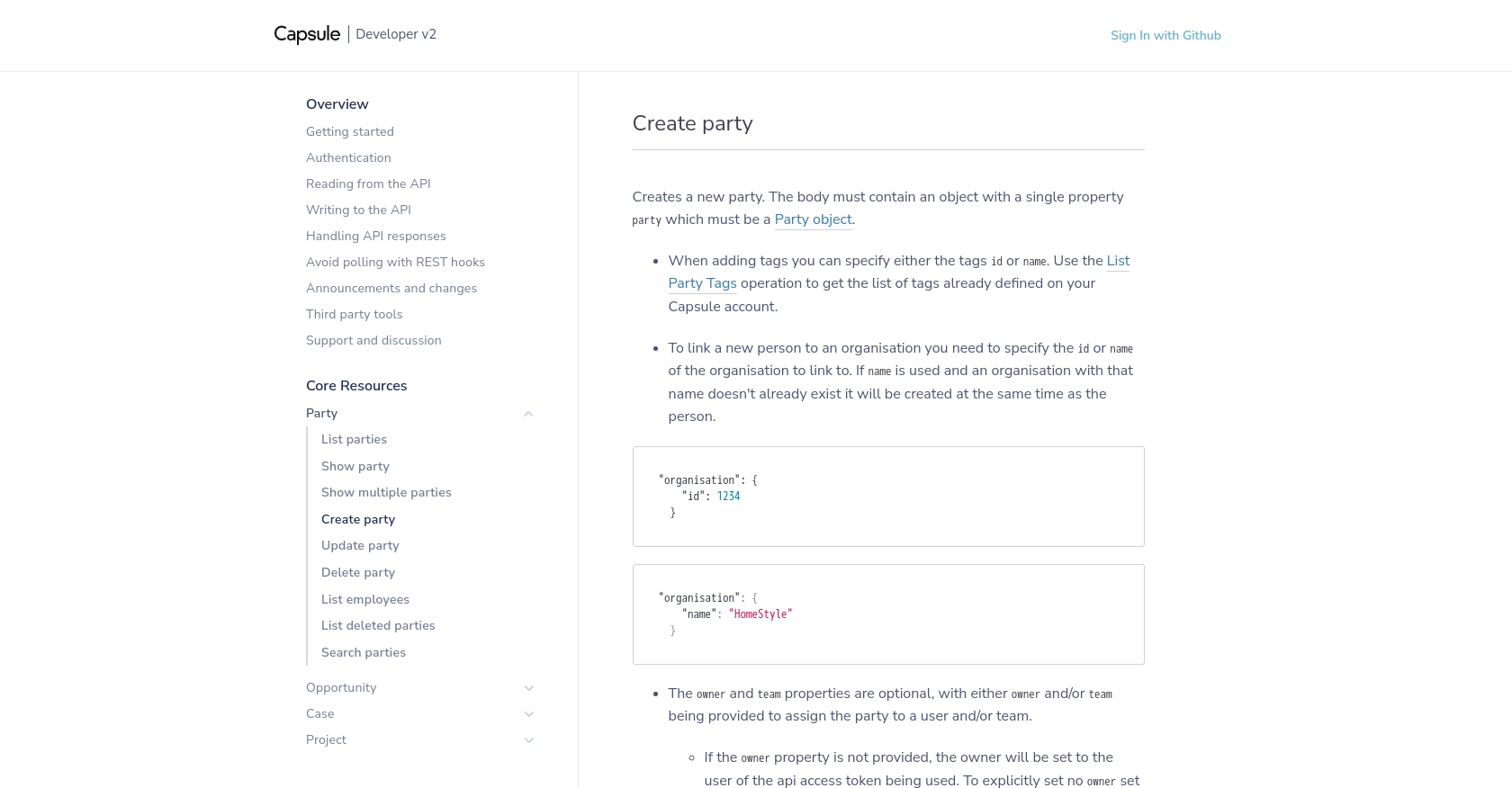
Best Practices for Capsule API Integration in PHP
When integrating with the Capsule API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Secure Storage of Credentials: Store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Capsule API allows up to 4,000 requests per hour. Monitor the
X-RateLimit-Remaining
header to avoid exceeding this limit. Implement exponential backoff strategies if you encounter rate limit errors. For more details, refer to the Capsule API Handling API Responses documentation. - Data Transformation and Standardization: Ensure that data fields are consistent and standardized across different systems. This helps maintain data integrity and simplifies data processing.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API response errors. Log errors for debugging and provide user-friendly messages where applicable.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Capsule CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#createParty
Ready to get started?