Using the Nutshell API to Get Users in PHP
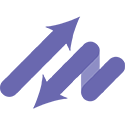
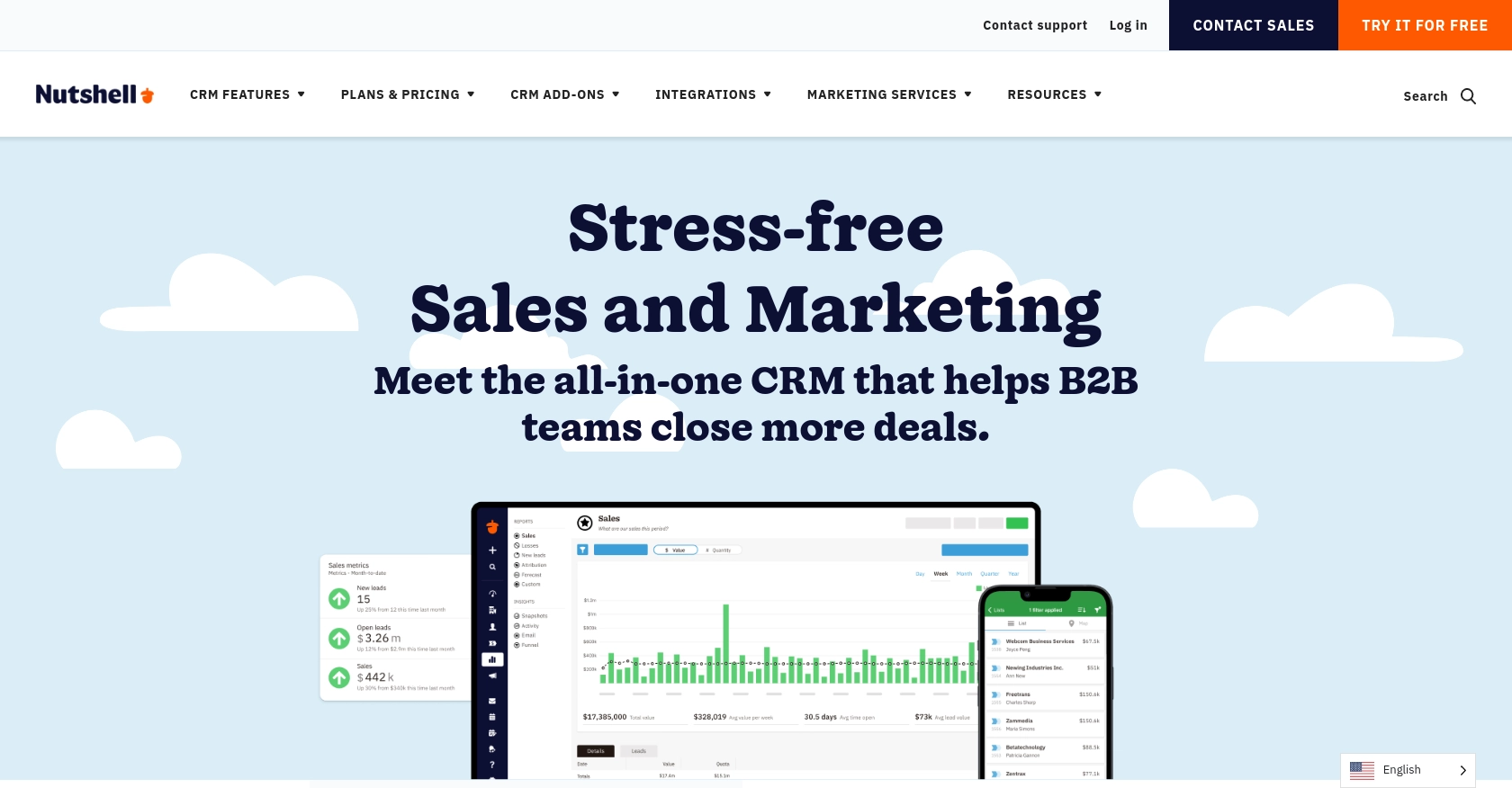
Introduction to Nutshell CRM
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. With its user-friendly interface and robust features, Nutshell enables companies to track leads, manage contacts, and streamline their sales operations.
Integrating with the Nutshell API allows developers to access and manipulate user data programmatically. For example, you might want to retrieve a list of users within your organization to synchronize with another system or to generate custom reports. This capability can significantly enhance productivity and data management across platforms.
Setting Up a Nutshell Test Account for API Integration
Before you can start using the Nutshell API to retrieve user data, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. Follow the on-screen instructions to create your account. Once your account is set up, log in to access the dashboard.
Generating an API Key for Nutshell
To interact with the Nutshell API, you need an API key. Follow these steps to generate one:
- Log in to your Nutshell account.
- Navigate to the "Setup" tab in the top navigation bar.
- Select "API" from the sidebar menu.
- Click on "Create New API Key" and fill in the necessary details.
- Ensure that the API key has the appropriate permissions for accessing user data.
- Save the API key securely, as you will need it for authentication in your API requests.
Understanding Nutshell API Authentication
The Nutshell API uses HTTP Basic authentication. You'll need to include the API key in the Authentication header of your requests. The username for authentication is your company's domain or a specific user's email address, and the password is the API key.
Here's an example of how to structure your authentication header:
$headers = [
'Authorization: Basic ' . base64_encode('your_domain_or_email:your_api_key')
];
Testing Your API Setup
Once you've set up your account and generated an API key, it's a good idea to test your setup by making a simple API call. This will ensure that your authentication is working correctly and that you can successfully connect to the Nutshell API.
Refer to the Nutshell API documentation for more details on available endpoints and methods.
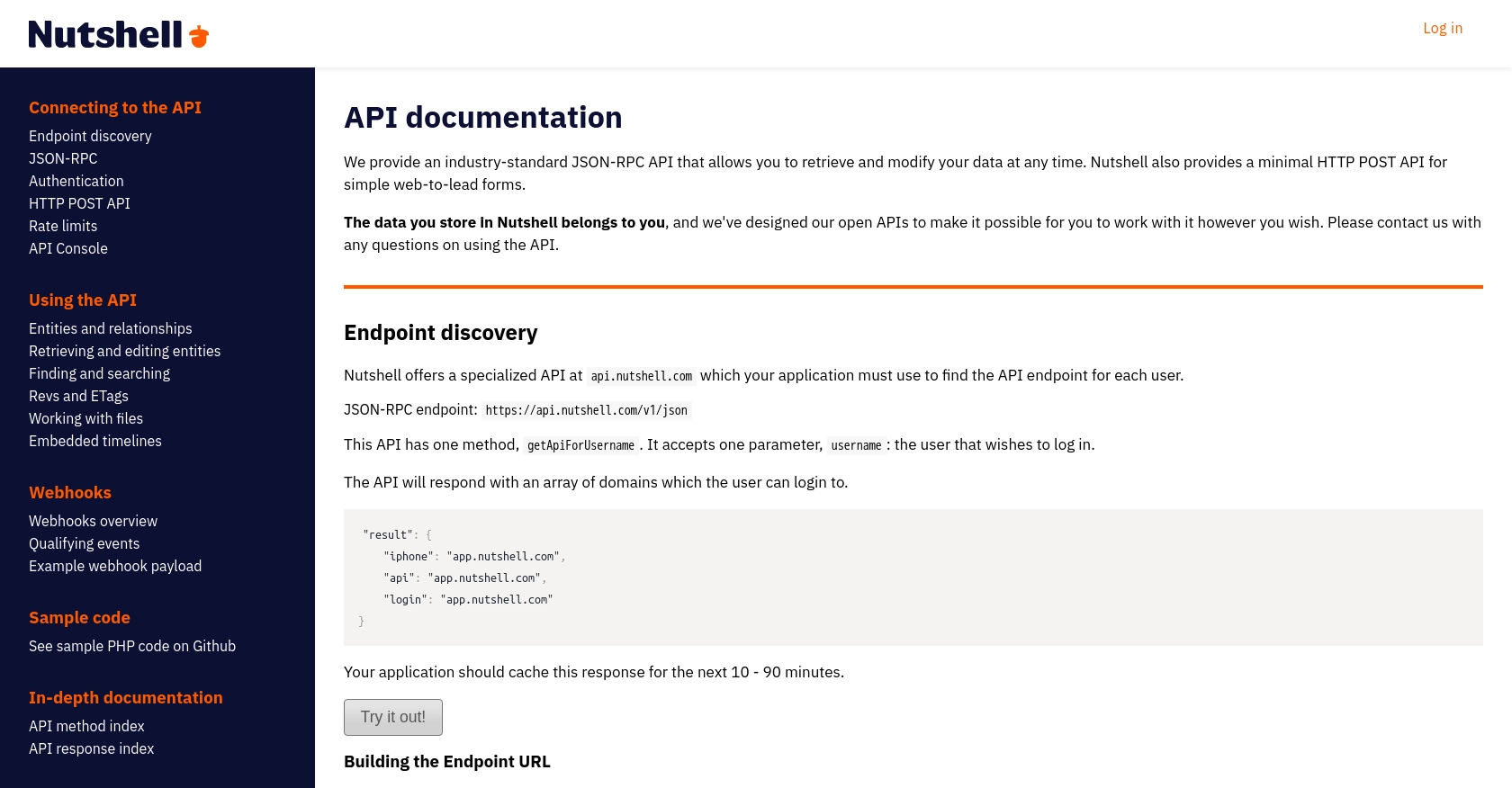
sbb-itb-96038d7
Making API Calls to Retrieve Users from Nutshell Using PHP
To interact with the Nutshell API and retrieve user data, you need to set up your PHP environment correctly. This section will guide you through the process of making API calls to Nutshell to fetch user information.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- cURL extension for PHP
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Writing the PHP Code to Fetch Users from Nutshell
Now that your environment is ready, you can write the PHP code to interact with the Nutshell API. The following example demonstrates how to retrieve a list of users:
<?php
// Set the API endpoint
$endpoint = "https://app.nutshell.com/api/v1/json";
// Set the authentication details
$username = "your_domain_or_email";
$apiKey = "your_api_key";
// Prepare the headers
$headers = [
'Content-Type: application/json',
'Authorization: Basic ' . base64_encode("$username:$apiKey")
];
// Prepare the JSON-RPC request payload
$data = [
'method' => 'findUsers',
'params' => [],
'id' => 1
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$result = json_decode($response, true);
if (isset($result['result'])) {
// Loop through the users and print their information
foreach ($result['result'] as $user) {
echo "User ID: " . $user['id'] . "\n";
echo "Name: " . $user['name'] . "\n";
echo "Email: " . implode(', ', $user['emails']) . "\n\n";
}
} else {
echo "Failed to retrieve users.";
}
}
// Close the cURL session
curl_close($ch);
?>
Verifying the API Call Success
After running the PHP script, you should see a list of users printed in the console. This indicates that the API call was successful. You can verify the retrieved data by cross-referencing it with the user data in your Nutshell dashboard.
Handling Errors and Common Issues
If you encounter errors, check the following:
- Ensure your API key and username are correct.
- Verify that the cURL extension is installed and enabled.
- Check for any network connectivity issues.
Refer to the Nutshell API documentation for more information on error codes and troubleshooting.
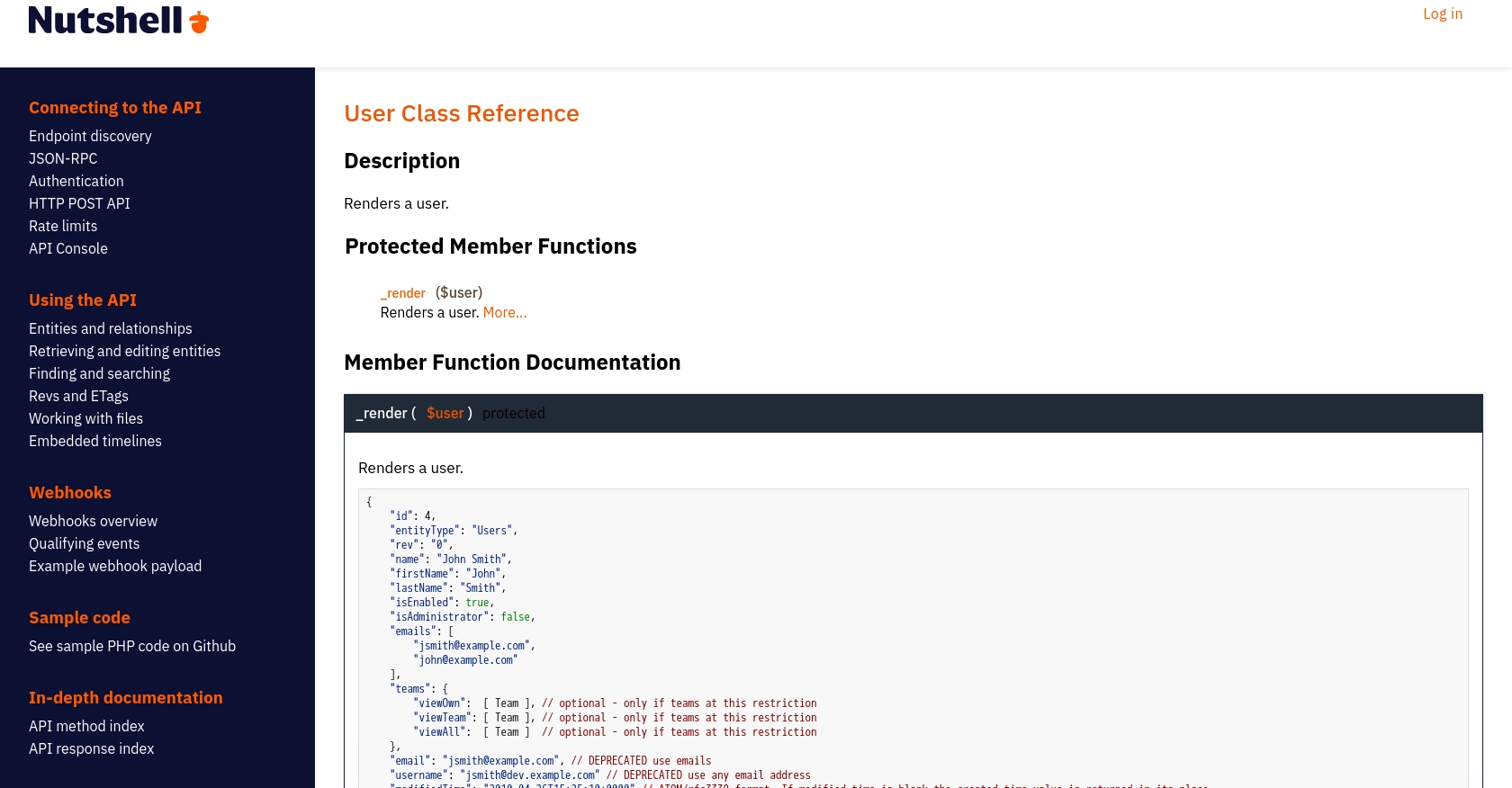
Best Practices for Using the Nutshell API in PHP
When working with the Nutshell API, it's important to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Nutshell API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Nutshell is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API errors and exceptions. Log errors for troubleshooting and monitoring purposes.
Enhancing Integration with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Nutshell. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
Ready to get started?