Using the Microsoft Dynamics 365 API to Get Custom Objects (with PHP examples)
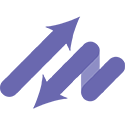
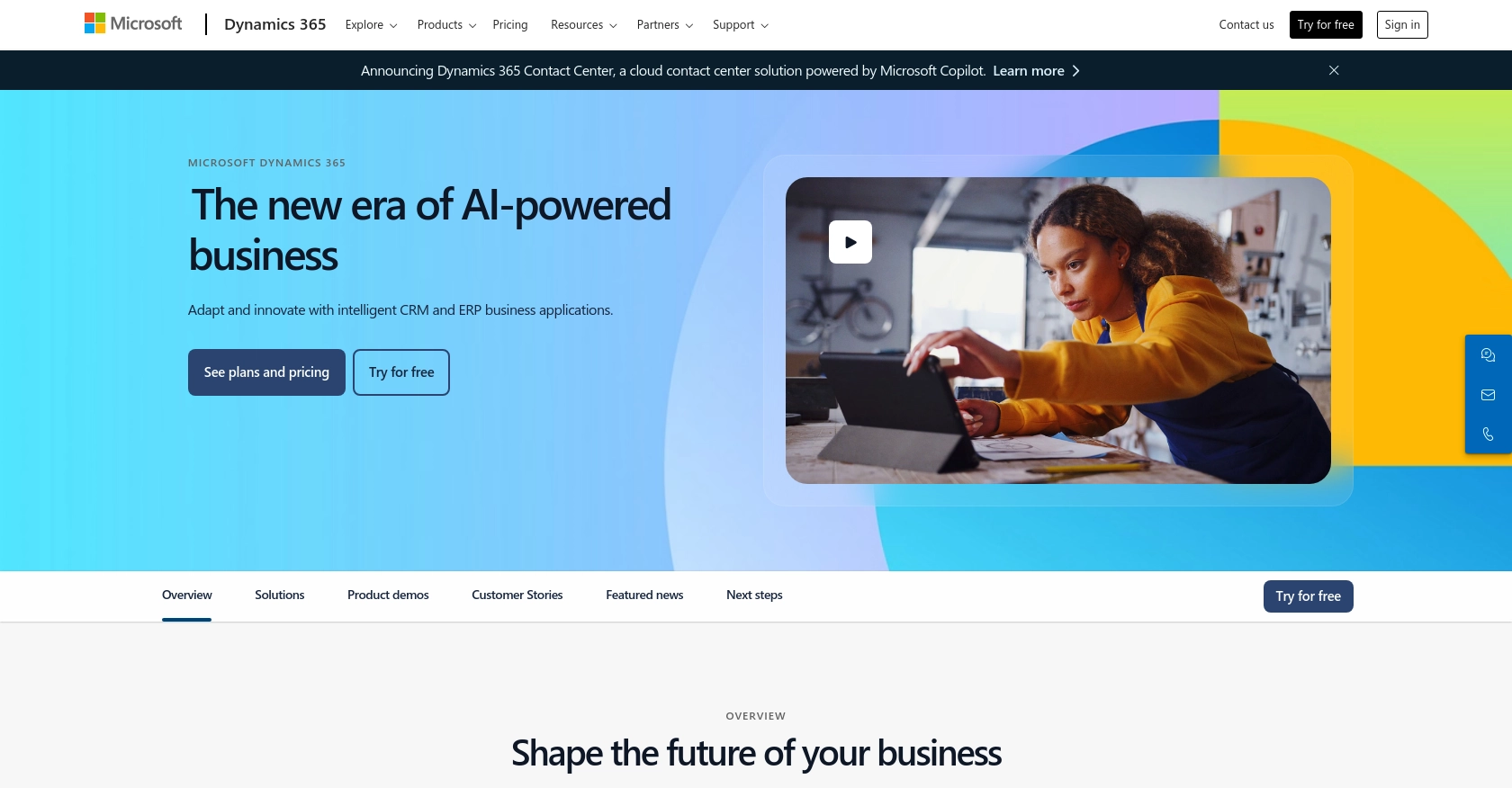
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a powerful suite of business applications that combine CRM and ERP capabilities to streamline operations and drive business growth. It offers a comprehensive platform for managing customer relationships, financials, operations, and more, making it an essential tool for businesses looking to enhance their digital transformation efforts.
Integrating with the Microsoft Dynamics 365 API allows developers to access and manipulate data within the platform, enabling custom solutions tailored to specific business needs. For example, a developer might use the API to retrieve custom objects, such as unique customer records or sales data, to integrate with other business systems or to generate detailed reports.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely experiment and test your API interactions without affecting live data.
Creating a Microsoft Dynamics 365 Free Trial Account
To begin, sign up for a free trial of Microsoft Dynamics 365. This trial provides access to the full suite of features, allowing you to explore and test the API capabilities.
- Visit the Microsoft Dynamics 365 website.
- Click on the "Try for free" button to start the registration process.
- Fill in the required information, including your email address and company details.
- Follow the instructions to complete the setup and access your trial account.
Registering an App for OAuth Authentication
Microsoft Dynamics 365 uses OAuth 2.0 for authentication, which requires you to register an application in Microsoft Entra ID. This process provides you with the necessary credentials to authenticate API requests.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to "Azure Active Directory" and select "App registrations".
- Click on "New registration" and fill in the application details:
- Name: Enter a name for your application.
- Supported account types: Choose "Accounts in this organizational directory only".
- Redirect URI: Set this to a URL where you will handle authentication responses, such as
https://localhost
for local development. - Click "Register" to create the application.
Generating Client ID and Client Secret
Once your app is registered, you need to generate the client ID and client secret, which are essential for OAuth authentication.
- In the app registration overview, note down the "Application (client) ID". This is your client ID.
- Navigate to "Certificates & secrets" and click on "New client secret".
- Provide a description and set an expiration period for the secret.
- Click "Add" and copy the generated client secret. Store it securely as it will not be shown again.
Configuring API Permissions
To interact with Microsoft Dynamics 365 data, you must configure the necessary API permissions for your app.
- Go to "API permissions" in your app registration.
- Click "Add a permission" and select "Dynamics CRM".
- Choose the permissions your app requires, such as "user_impersonation" for accessing data on behalf of a user.
- Click "Add permissions" to apply the changes.
With your test account and app registration set up, you're now ready to authenticate and interact with the Microsoft Dynamics 365 API using PHP.
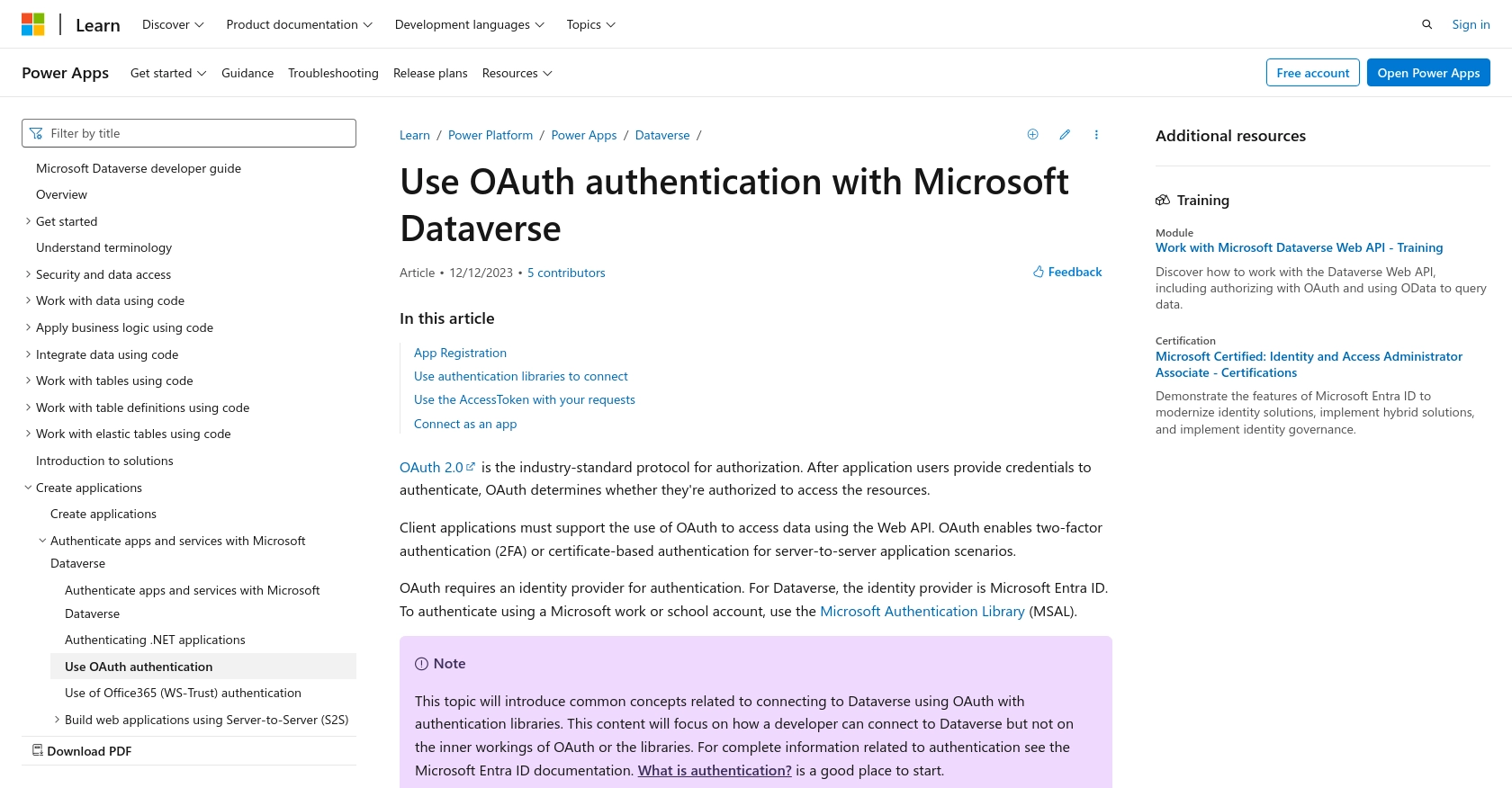
sbb-itb-96038d7
Making API Calls to Retrieve Custom Objects from Microsoft Dynamics 365 Using PHP
To interact with the Microsoft Dynamics 365 API and retrieve custom objects, you'll need to make authenticated API calls using PHP. This section will guide you through setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Authenticating with Microsoft Dynamics 365 API Using OAuth
To authenticate, you'll use the client ID and client secret obtained during the app registration process. You'll need to acquire an access token from Microsoft Entra ID.
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://login.microsoftonline.com/{tenant_id}/oauth2/v2.0/token', [
'form_params' => [
'grant_type' => 'client_credentials',
'client_id' => 'Your_Client_ID',
'client_secret' => 'Your_Client_Secret',
'scope' => 'https://yourorg.crm.dynamics.com/.default'
]
]);
$tokenData = json_decode($response->getBody(), true);
$accessToken = $tokenData['access_token'];
Replace {tenant_id}
, Your_Client_ID
, and Your_Client_Secret
with your actual values.
Retrieving Custom Objects from Microsoft Dynamics 365
With the access token, you can now make API calls to retrieve custom objects. Here's an example of how to fetch entities:
$response = $client->get('https://yourorg.crm.dynamics.com/api/data/v9.2/entities', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'OData-MaxVersion' => '4.0',
'OData-Version' => '4.0',
'Accept' => 'application/json'
]
]);
$entities = json_decode($response->getBody(), true);
foreach ($entities['value'] as $entity) {
echo 'Entity Name: ' . $entity['name'] . '<br>';
}
Ensure you replace yourorg.crm.dynamics.com
with your organization's specific URL.
Handling API Response and Errors
After making the API call, you should verify the response to ensure it succeeded. Check the HTTP status code and handle any errors appropriately.
if ($response->getStatusCode() === 200) {
// Process the response
echo 'Custom objects retrieved successfully.';
} else {
// Handle errors
echo 'Failed to retrieve custom objects. Status code: ' . $response->getStatusCode();
}
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
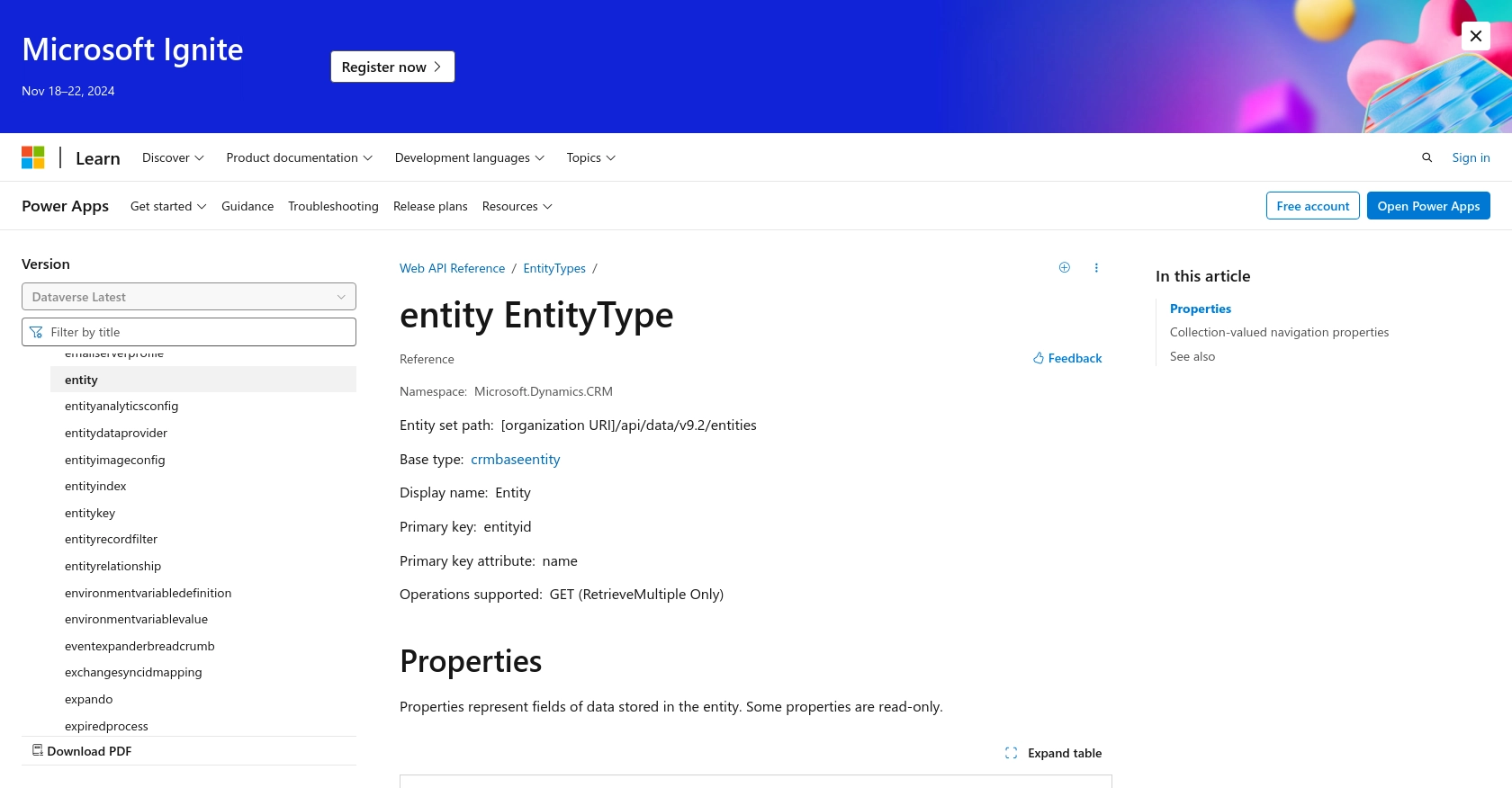
Best Practices for Using Microsoft Dynamics 365 API in PHP
When working with the Microsoft Dynamics 365 API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Microsoft Dynamics 365 API documentation.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to fit your application's requirements. This may involve mapping fields and converting data types.
- Monitor and Log API Requests: Implement logging to track API requests and responses. This will help in debugging and monitoring the performance of your integration.
Streamlining Integrations with Endgrate
Building and maintaining integrations with platforms like Microsoft Dynamics 365 can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365.
With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/entity?view=dataverse-latest
Ready to get started?