Using the Webhook API to Push Data in PHP
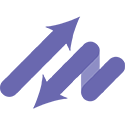
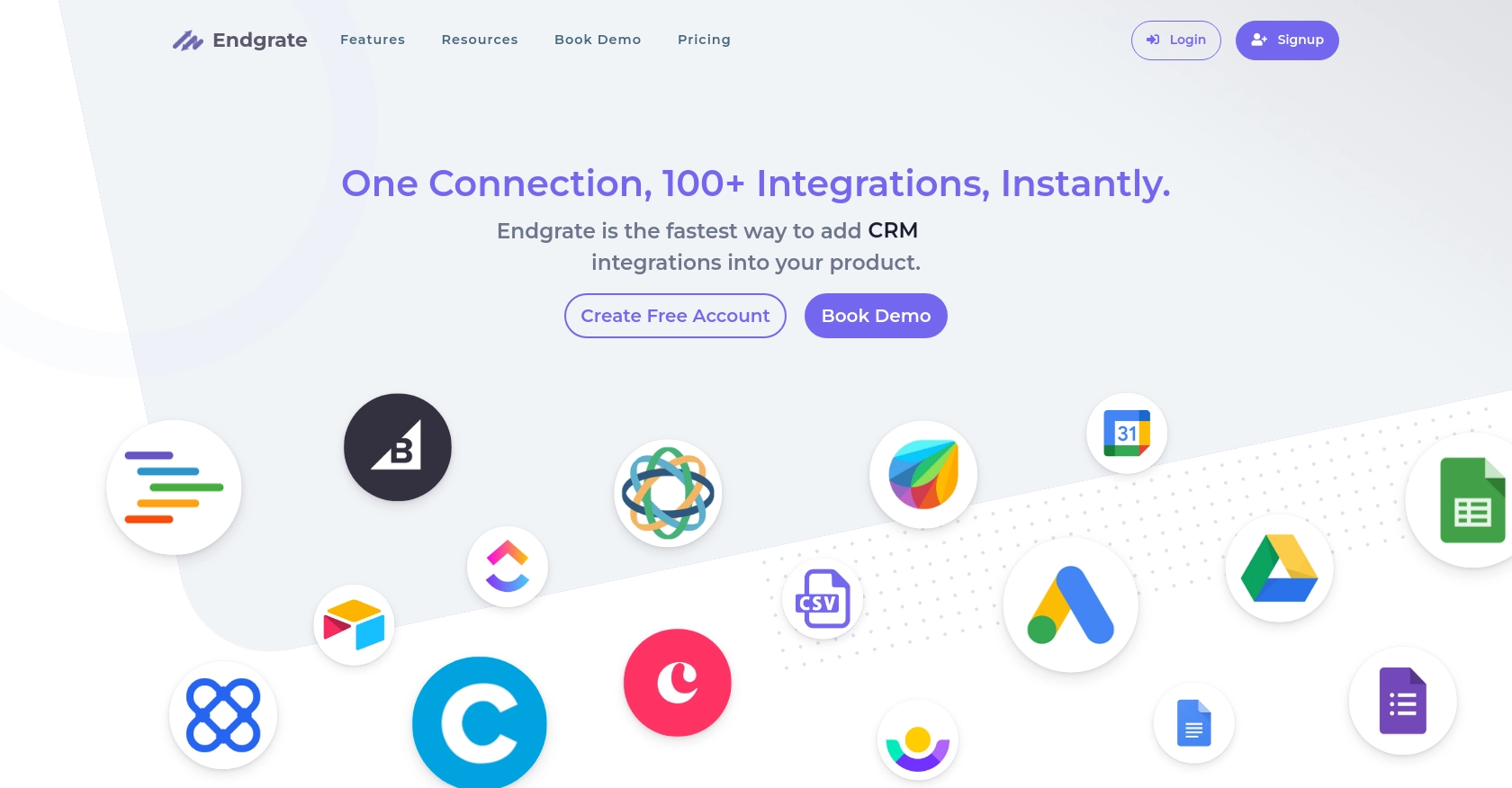
Introduction to Webhook API Integration
The Webhook API is a powerful tool that allows developers to automate data transmission between different web services. By utilizing webhooks, developers can push data to specific URLs, enabling real-time communication and integration between applications. This is particularly useful for B2B SaaS products that require seamless data exchange across platforms.
Integrating with the Webhook API can significantly enhance a developer's ability to manage data flows efficiently. For example, a developer might use the Webhook API to push customer data from an e-commerce platform to a CRM system, ensuring that sales teams have up-to-date information for personalized customer interactions.
Setting Up a Test or Sandbox Account for Webhook API Integration
Before you can start pushing data using the Webhook API, it's essential to set up a test or sandbox environment. This allows you to experiment with the API without affecting live data, ensuring that your integration is both functional and secure.
Creating a Webhook Test Environment
To begin, you'll need to establish a test environment where you can simulate API interactions. This typically involves setting up a sandbox account or using a testing tool that mimics the behavior of the Webhook API.
- Choose a Testing Tool: Select a tool that supports webhook testing, such as Webhook.site or RequestBin. These platforms allow you to create temporary URLs to receive webhook data.
- Generate a Test URL: Use the chosen tool to generate a unique URL. This URL will act as the endpoint to which you will send data during testing.
Configuring Webhook API for Testing
Once you have your test URL, configure your application to send data to this endpoint. This involves setting up the necessary parameters and ensuring that your application can communicate with the Webhook API.
- Define the Endpoint: In your application, specify the test URL as the endpoint for your webhook data.
- Set Data Format: Ensure that the data you plan to send is in a format accepted by the Webhook API, typically JSON.
Validating Webhook Configuration
Before proceeding with full-scale testing, it's crucial to validate your webhook configuration. This step ensures that your setup is correct and that data can be transmitted successfully.
- Send a Test Request: Use your application to send a sample data payload to the test URL. This helps verify that the endpoint is correctly receiving data.
- Check the Response: Review the server's response to confirm that the data was received and processed without errors. Look for HTTP status codes in the 200 range to indicate success.
By following these steps, you can confidently set up a test environment for your Webhook API integration, paving the way for seamless data transmission in your applications.
Making API Calls with PHP for Webhook Integration
To effectively push data using the Webhook API in PHP, you need to understand the process of making HTTP requests. PHP offers several libraries and built-in functions that simplify this task, allowing you to send data to a specified URL efficiently.
Setting Up Your PHP Environment for Webhook API Calls
Before making API calls, ensure your PHP environment is correctly configured. This includes having the necessary PHP version and libraries installed.
- PHP Version: Ensure you are using PHP 7.4 or higher for optimal performance and security.
- Install cURL: PHP's cURL library is a powerful tool for making HTTP requests. Ensure it's enabled in your PHP configuration.
Writing PHP Code to Send Data to Webhook API
With your environment set up, you can now write the PHP code to send data to the Webhook API. This involves using cURL to make a POST request to the specified URL.
<?php
// Define the webhook URL
$webhookUrl = "https://your-webhook-url.com/endpoint";
// Prepare the data to be sent
$data = [
"name" => "John Doe",
"email" => "john.doe@example.com"
];
// Convert data to JSON format
$jsonData = json_encode($data);
// Initialize cURL session
$ch = curl_init($webhookUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Content-Length: ' . strlen($jsonData)
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response:' . $response;
}
// Close cURL session
curl_close($ch);
?>
Handling Responses and Errors in Webhook API Calls
After sending data, it's crucial to handle the server's response correctly. This ensures that your integration is robust and can handle any issues that arise.
- Check HTTP Status Codes: A status code in the 200 range indicates success. Handle other codes by logging errors and implementing retry mechanisms if necessary.
- Log Responses: Always log the server's response for debugging and auditing purposes.
Verifying Successful Data Transmission to Webhook API
Once your PHP script executes, verify that the data was successfully transmitted to the Webhook API. This can be done by checking the response and ensuring the data appears in the intended application or service.
- Review Logs: Check your application logs to confirm that the data was sent and received without errors.
- Test with Sample Data: Use sample data to test the integration before deploying it with live data.
By following these steps, you can effectively push data using the Webhook API in PHP, ensuring seamless integration and real-time data exchange between your applications.
Conclusion and Best Practices for Webhook API Integration in PHP
Integrating with the Webhook API using PHP can significantly enhance your application's ability to communicate and exchange data in real-time. By following the steps outlined in this guide, you can ensure a seamless and efficient integration process.
Best Practices for Secure and Efficient Webhook API Integration
- Secure Data Transmission: Always use HTTPS to encrypt data transmitted to the webhook endpoint, ensuring data security and integrity.
- Handle Rate Limits: Be mindful of any rate limits imposed by the API provider. Implement mechanisms to handle rate limiting gracefully, such as exponential backoff or queuing requests.
- Store Credentials Safely: If your integration requires storing sensitive information, such as API keys or tokens, ensure they are stored securely, using environment variables or secure vaults.
- Implement Error Handling: Robust error handling is crucial. Log errors and implement retry mechanisms to handle transient issues effectively.
- Validate Data: Before sending data, validate it to ensure it meets the expected format and requirements of the Webhook API.
Leverage Endgrate for Streamlined Integration Management
While building integrations manually can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies integration management across multiple platforms, including webhooks. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?