How to Get Customers with the BigCommerce API in PHP
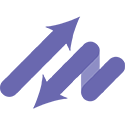
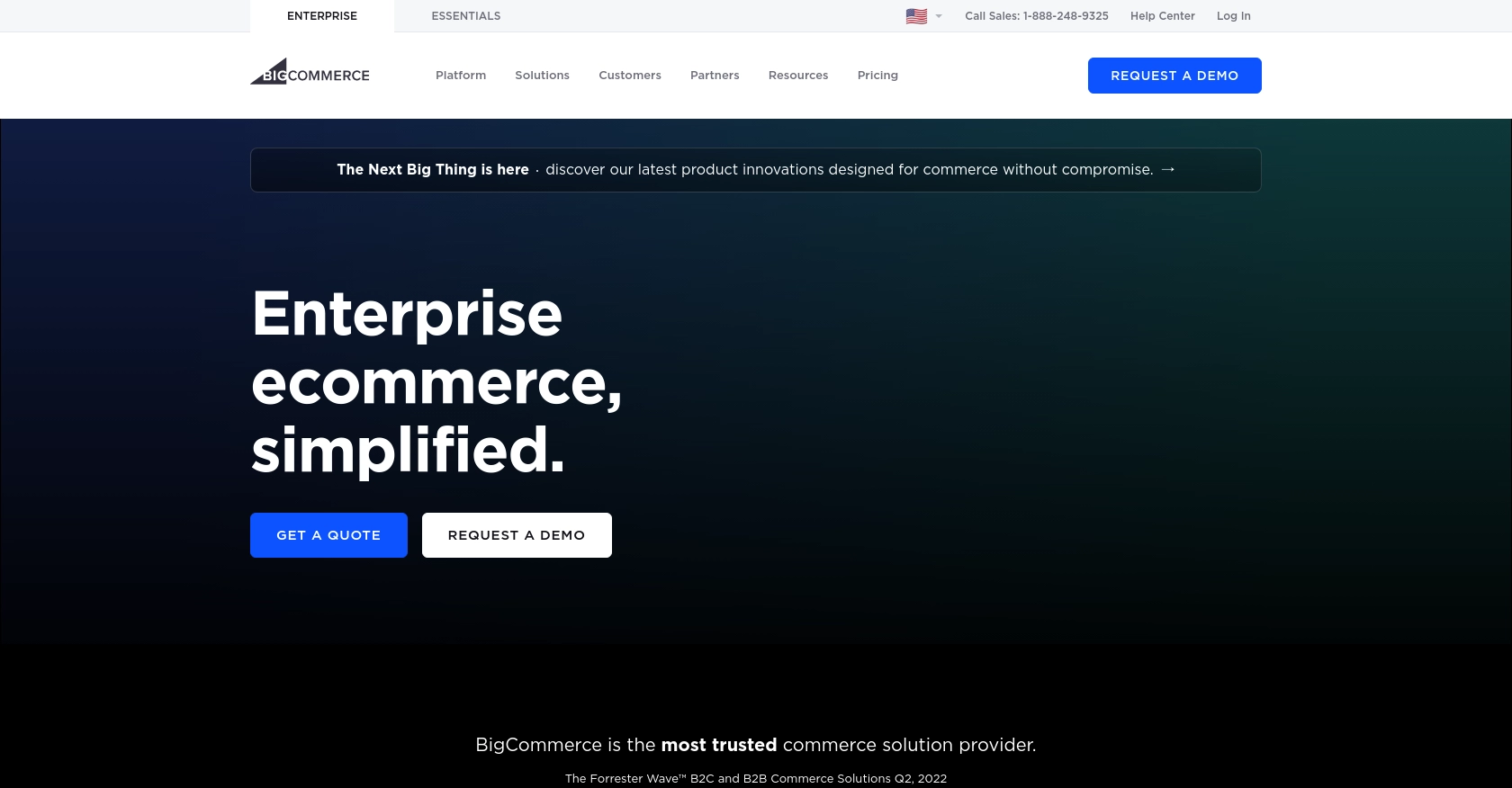
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customers, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically. This can be particularly useful for automating tasks such as retrieving customer information, which can help in personalizing marketing efforts or enhancing customer service. For example, a developer might use the BigCommerce API to fetch customer data and analyze purchasing patterns to tailor marketing campaigns effectively.
Setting Up a BigCommerce Test or Sandbox Account
Before you begin integrating with the BigCommerce API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live store data. BigCommerce provides developers with the tools needed to create a sandbox environment for testing purposes.
Creating a BigCommerce Sandbox Account
To get started, follow these steps to create a BigCommerce sandbox account:
- Visit the BigCommerce Developer Portal and sign up for a developer account if you haven't already.
- Once logged in, navigate to the "Sandbox Stores" section.
- Click on "Create a Sandbox Store" and follow the prompts to set up your sandbox environment.
- After creation, you'll have access to a sandbox store where you can test API interactions.
Generating API Credentials for BigCommerce
To interact with the BigCommerce API, you'll need to generate API credentials. Follow these steps to obtain them:
- In your BigCommerce control panel, go to "Advanced Settings" and select "API Accounts."
- Click on "Create API Account" and choose "Create V2/V3 API Token."
- Provide a name for your API account and select the necessary OAuth scopes that your application requires.
- Once configured, click "Save" to generate your API credentials, including the Client ID, Client Secret, and Access Token.
- Store these credentials securely, as they will be used to authenticate your API requests.
Understanding BigCommerce API Authentication
BigCommerce uses OAuth-based authentication for API requests. The primary method involves using the X-Auth-Token
header to pass the access token. Ensure your API account has the correct permissions by configuring the OAuth scopes appropriately. For more details, refer to the BigCommerce Authentication Documentation.
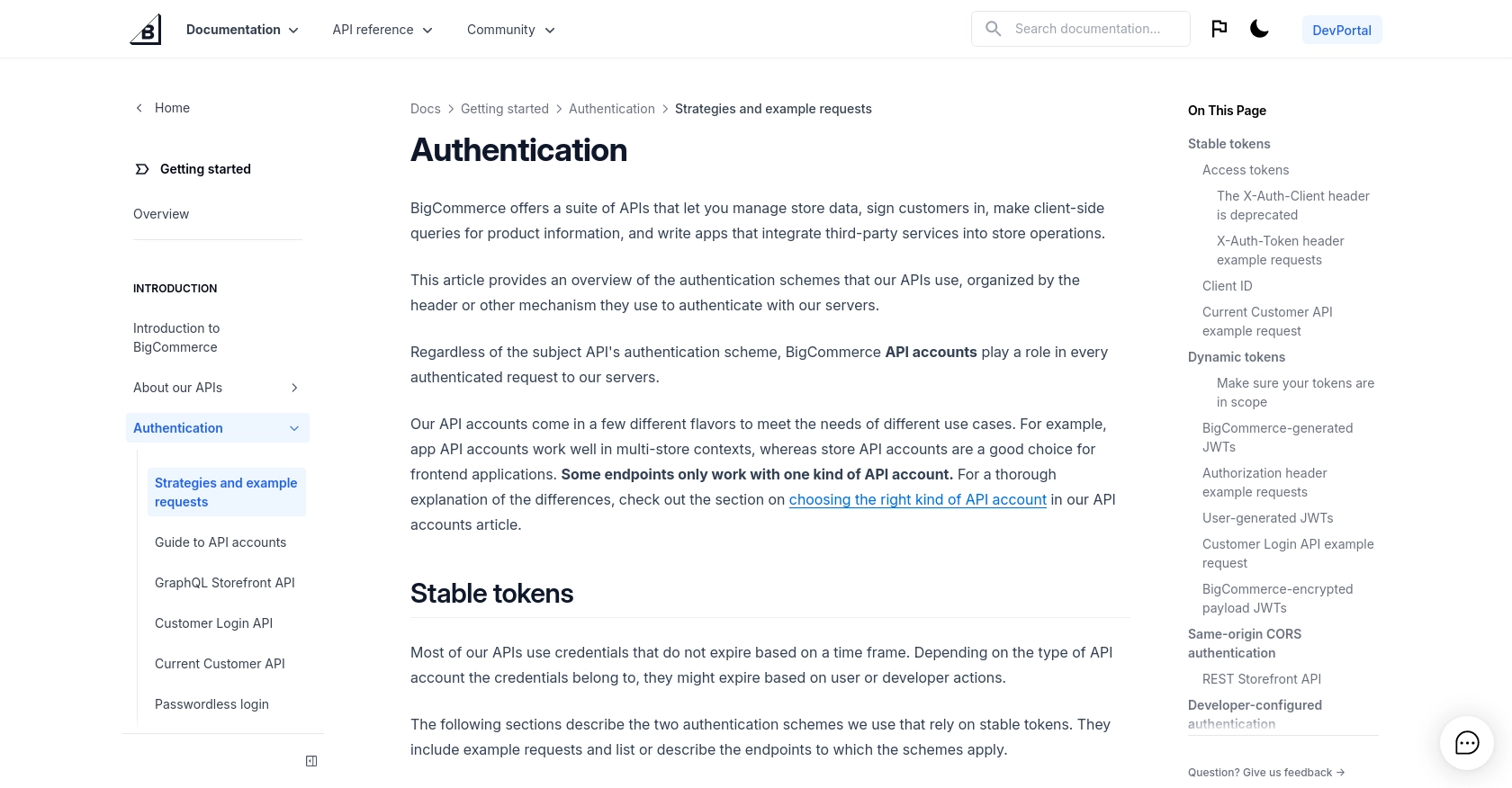
sbb-itb-96038d7
Making API Calls to Retrieve Customers with BigCommerce API in PHP
To interact with the BigCommerce API using PHP, you need to ensure that your development environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls to retrieve customer data from BigCommerce.
Setting Up Your PHP Environment for BigCommerce API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, to handle dependencies.
To install Composer, follow the instructions on the Composer download page.
Installing Required PHP Dependencies for BigCommerce API
You'll need the Guzzle HTTP client to make HTTP requests in PHP. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Customers from BigCommerce
Now that your environment is set up, you can write the PHP code to interact with the BigCommerce API. Create a file named get_customers.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$storeHash = 'your_store_hash';
$accessToken = 'your_access_token';
$response = $client->request('GET', "https://api.bigcommerce.com/stores/{$storeHash}/v3/customers", [
'headers' => [
'X-Auth-Token' => $accessToken,
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$customers = json_decode($response->getBody(), true);
foreach ($customers['data'] as $customer) {
echo "Customer ID: " . $customer['id'] . " - Name: " . $customer['first_name'] . " " . $customer['last_name'] . "\n";
}
} else {
echo "Failed to retrieve customers. Status code: " . $response->getStatusCode();
}
Replace your_store_hash
and your_access_token
with your actual BigCommerce store hash and access token.
Running the PHP Script to Retrieve BigCommerce Customers
Execute the script from the command line using the following command:
php get_customers.php
If successful, the script will output a list of customers from your BigCommerce store.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the HTTP status code returned by the API. A status code of 200 indicates success. If you encounter errors, refer to the BigCommerce API documentation for troubleshooting tips and error code explanations.
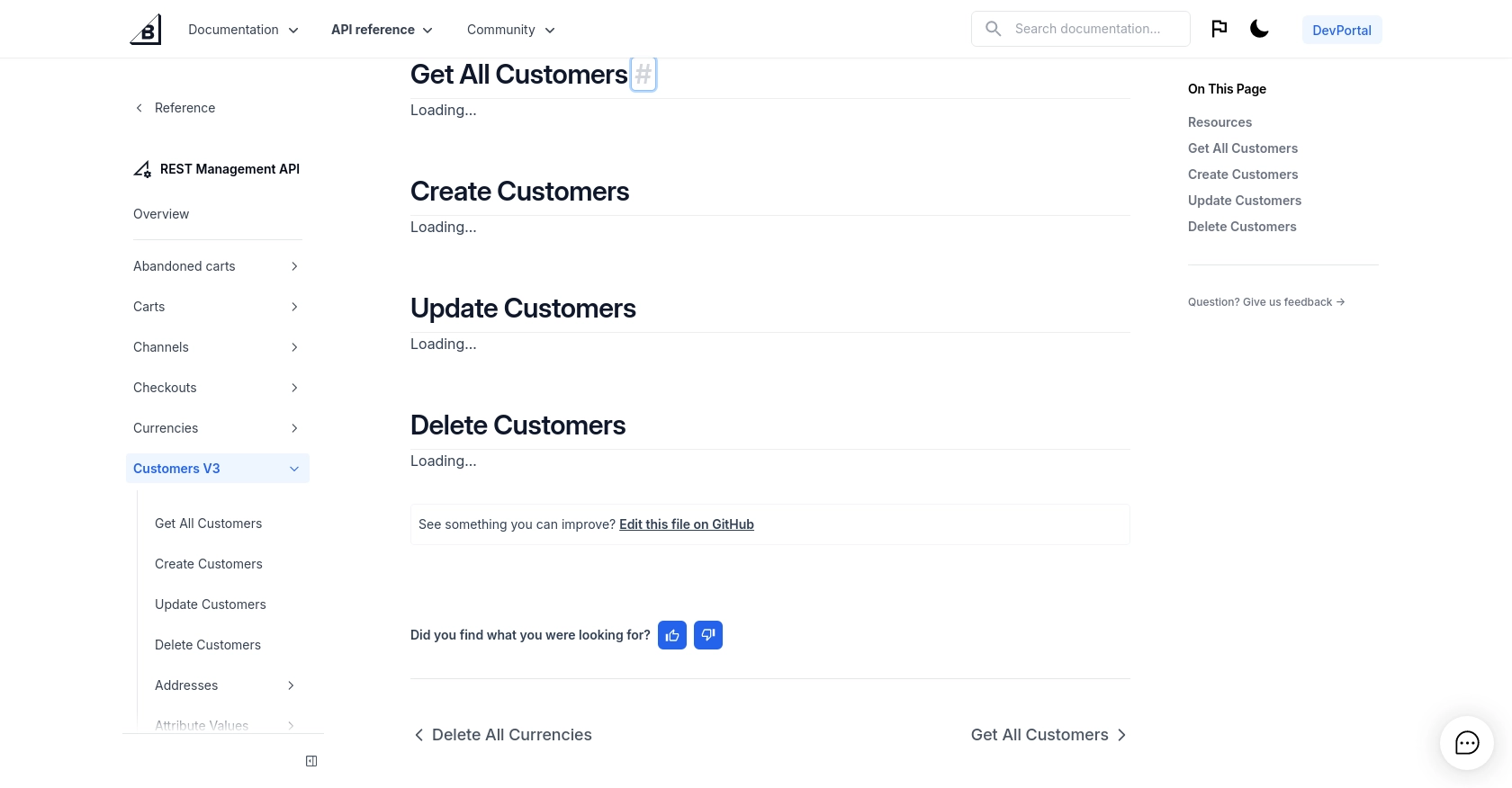
Conclusion and Best Practices for BigCommerce API Integration in PHP
Integrating with the BigCommerce API using PHP provides a powerful way to automate and enhance your e-commerce operations. By retrieving customer data programmatically, you can personalize marketing strategies, improve customer service, and streamline various business processes.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store API Credentials: Always store your API credentials, such as the access token, securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: BigCommerce APIs have rate limits. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. For specific rate limit details, refer to the BigCommerce Authentication Documentation.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with BigCommerce's terms and to optimize your application's performance.
- Standardize Data: When retrieving customer data, consider transforming and standardizing it to fit your application's data model, ensuring consistency across different systems.
Streamline Your Integration Process with Endgrate
While building integrations manually can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including BigCommerce. This allows you to focus on your core product while outsourcing the intricacies of integration development.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, saving both time and resources. Explore how Endgrate can simplify your integration experience by visiting Endgrate's website.
Read More
Ready to get started?