Using the Zoho Books API to Create or Update Items in Javascript
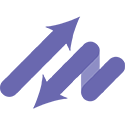
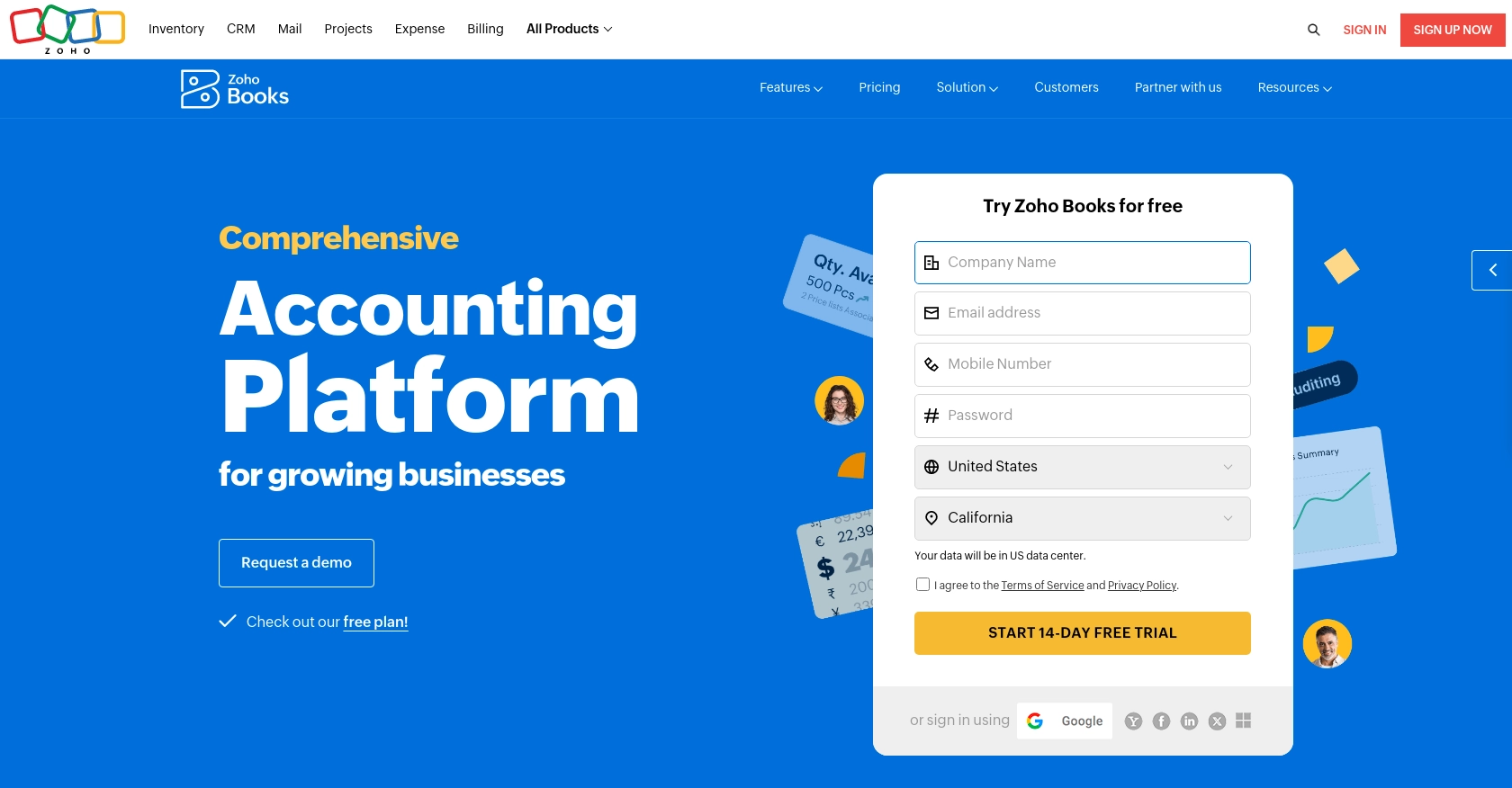
Introduction to Zoho Books API
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial management for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses seeking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to enhance their applications by automating tasks such as creating or updating items in the inventory. For example, a developer might use the API to automatically update product details in Zoho Books whenever changes are made in an external inventory management system, ensuring data consistency across platforms.
In this article, we will explore how to use JavaScript to interact with the Zoho Books API, focusing on creating and updating items. This integration can significantly improve efficiency by reducing manual data entry and minimizing errors.
Setting Up Your Zoho Books Test Account for API Integration
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Step 1: Register for a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. This will give you access to all the features you need to test your API integration.
Step 2: Access the Zoho Developer Console
To interact with the Zoho Books API, you'll need to register your application in the Zoho Developer Console. Visit the Zoho Developer Console and click on "Add Client ID" to create a new application.
Step 3: Obtain OAuth Credentials
- Provide the necessary details to register your application, such as the application name and redirect URI.
- Once registered, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Step 4: Generate an OAuth Grant Token
To generate a grant token, redirect users to the following authorization URL with the required parameters:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.CREATE,ZohoBooks.settings.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URI
with your actual Client ID and redirect URI.
Step 5: Exchange the Grant Token for Access and Refresh Tokens
After obtaining the grant token, make a POST request to exchange it for access and refresh tokens:
https://accounts.zoho.com/oauth/v2/token?code=YOUR_GRANT_TOKEN&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Ensure you replace placeholders with your actual values. The response will include both access and refresh tokens.
Step 6: Securely Store Your Tokens
Store the access and refresh tokens securely in your application. The access token will expire, but you can use the refresh token to obtain a new access token when needed.
With your Zoho Books test account and OAuth credentials set up, you're now ready to start making API calls to create or update items using JavaScript.
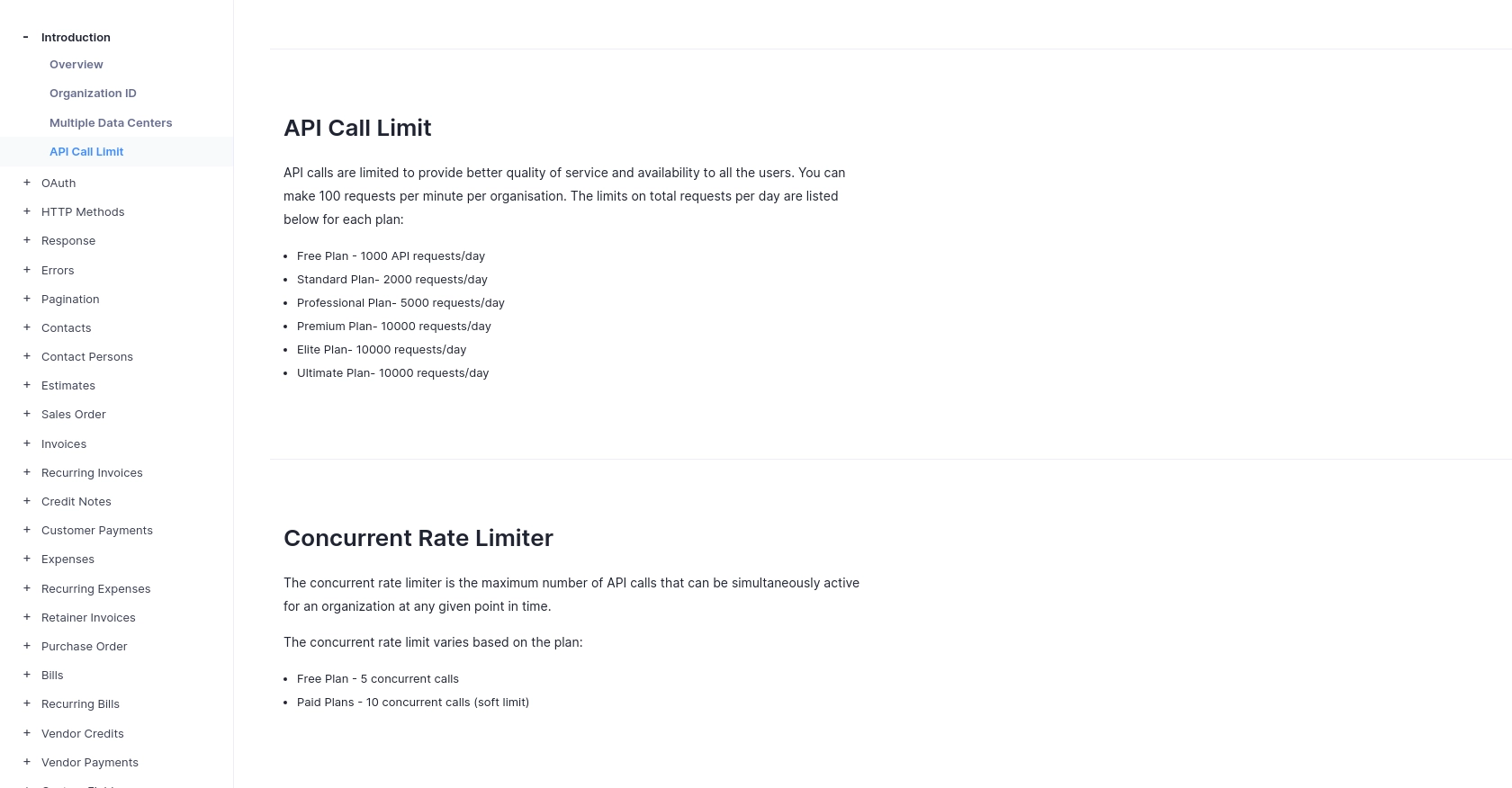
sbb-itb-96038d7
Making API Calls to Zoho Books for Creating or Updating Items Using JavaScript
Now that you have set up your Zoho Books account and obtained the necessary OAuth credentials, it's time to make API calls to create or update items. In this section, we'll guide you through the process using JavaScript, ensuring a seamless integration with Zoho Books.
Prerequisites for JavaScript Integration with Zoho Books API
Before proceeding, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
node-fetch
package for making HTTP requests. You can install it using the following command:
npm install node-fetch
Creating an Item in Zoho Books Using JavaScript
To create an item in Zoho Books, you'll need to make a POST request to the API endpoint. Here's a step-by-step guide:
const fetch = require('node-fetch');
const createItem = async () => {
const url = 'https://www.zohoapis.com/books/v3/items?organization_id=YOUR_ORG_ID';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
const body = JSON.stringify({
name: 'New Product',
rate: 100,
description: 'A new product description',
sku: 'NP12345',
product_type: 'goods'
});
try {
const response = await fetch(url, {
method: 'POST',
headers: headers,
body: body
});
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error creating item:', error);
}
};
createItem();
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. This code will create a new item in your Zoho Books account.
Updating an Item in Zoho Books Using JavaScript
To update an existing item, you'll need to make a PUT request. Here's how you can do it:
const updateItem = async (itemId) => {
const url = `https://www.zohoapis.com/books/v3/items/${itemId}?organization_id=YOUR_ORG_ID`;
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
const body = JSON.stringify({
name: 'Updated Product Name',
rate: 150,
description: 'Updated product description'
});
try {
const response = await fetch(url, {
method: 'PUT',
headers: headers,
body: body
});
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error updating item:', error);
}
};
updateItem('ITEM_ID');
Replace YOUR_ORG_ID
, YOUR_ACCESS_TOKEN
, and ITEM_ID
with your actual organization ID, access token, and the ID of the item you wish to update. This code will update the specified item in your Zoho Books account.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. Zoho Books API uses standard HTTP status codes:
- 200 OK: The request was successful.
- 201 Created: The item was successfully created.
- 400 Bad Request: The request was invalid. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified item was not found.
- 429 Rate Limit Exceeded: Too many requests. Respect the rate limits.
- 500 Internal Server Error: A server error occurred. Try again later.
For more details on error handling, refer to the Zoho Books API documentation.
Verifying API Call Success in Zoho Books
After making API calls, verify the success by checking the Zoho Books dashboard. Newly created items should appear in the inventory, and updated items should reflect the changes.
By following these steps, you can efficiently integrate Zoho Books API into your JavaScript applications, automating item management and enhancing your business processes.
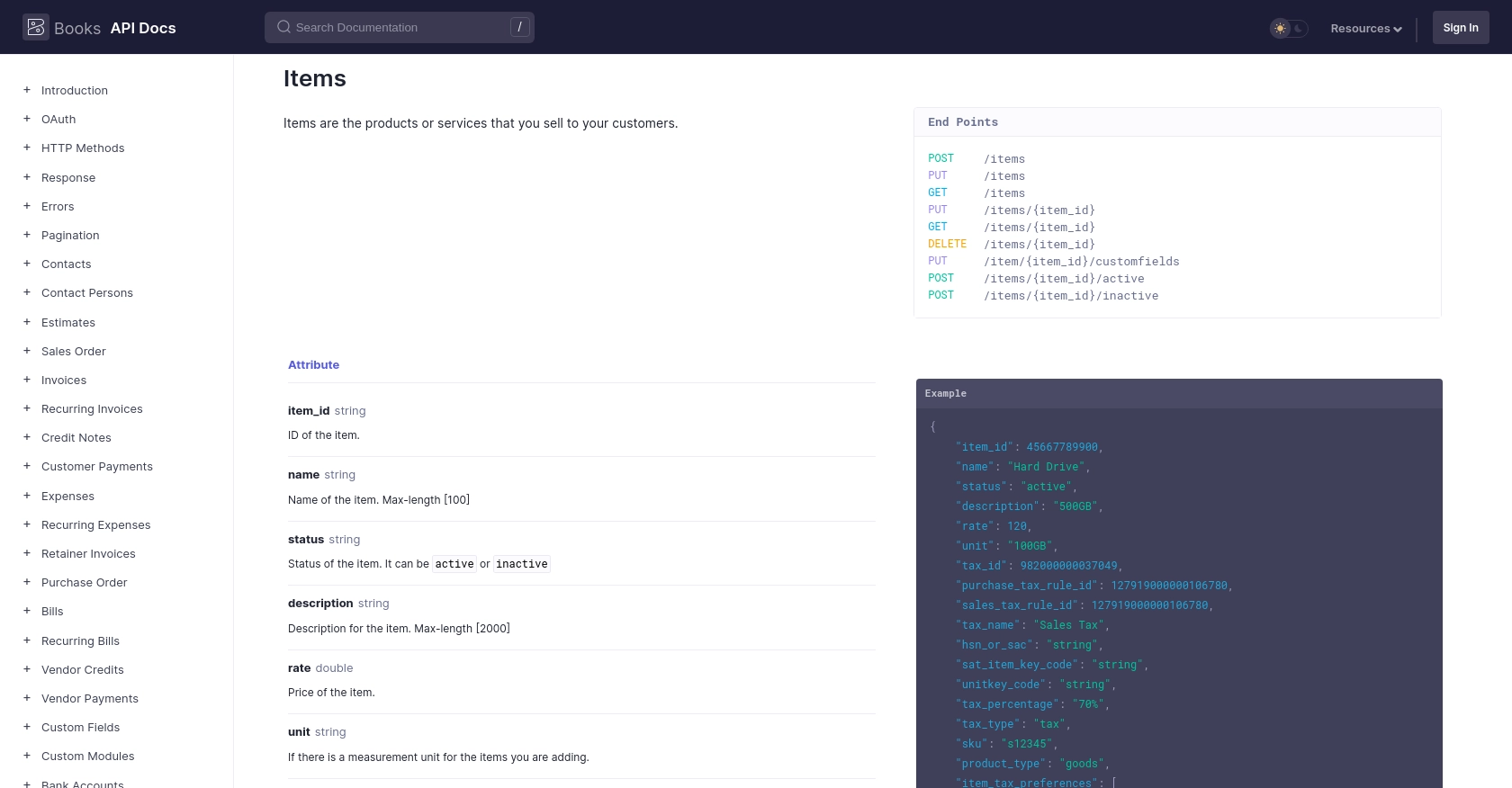
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using JavaScript can significantly enhance your application's capabilities by automating item management tasks. By following the steps outlined in this guide, you can create and update items efficiently, reducing manual effort and minimizing errors.
Best Practices for Secure and Efficient Zoho Books API Usage
- Secure Token Storage: Always store your OAuth tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Zoho Books API has a rate limit of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the API call limit documentation.
- Error Handling: Implement robust error handling to manage different HTTP status codes. This ensures your application can respond appropriately to various scenarios.
- Data Standardization: Ensure consistent data formats across your systems to maintain data integrity when syncing with Zoho Books.
Enhance Your Integration Strategy with Endgrate
While integrating with Zoho Books API can streamline your processes, managing multiple integrations can be complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product. By using Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/items/#overview
Ready to get started?