How to Get Organization with the Pipedrive API in Python
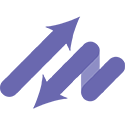
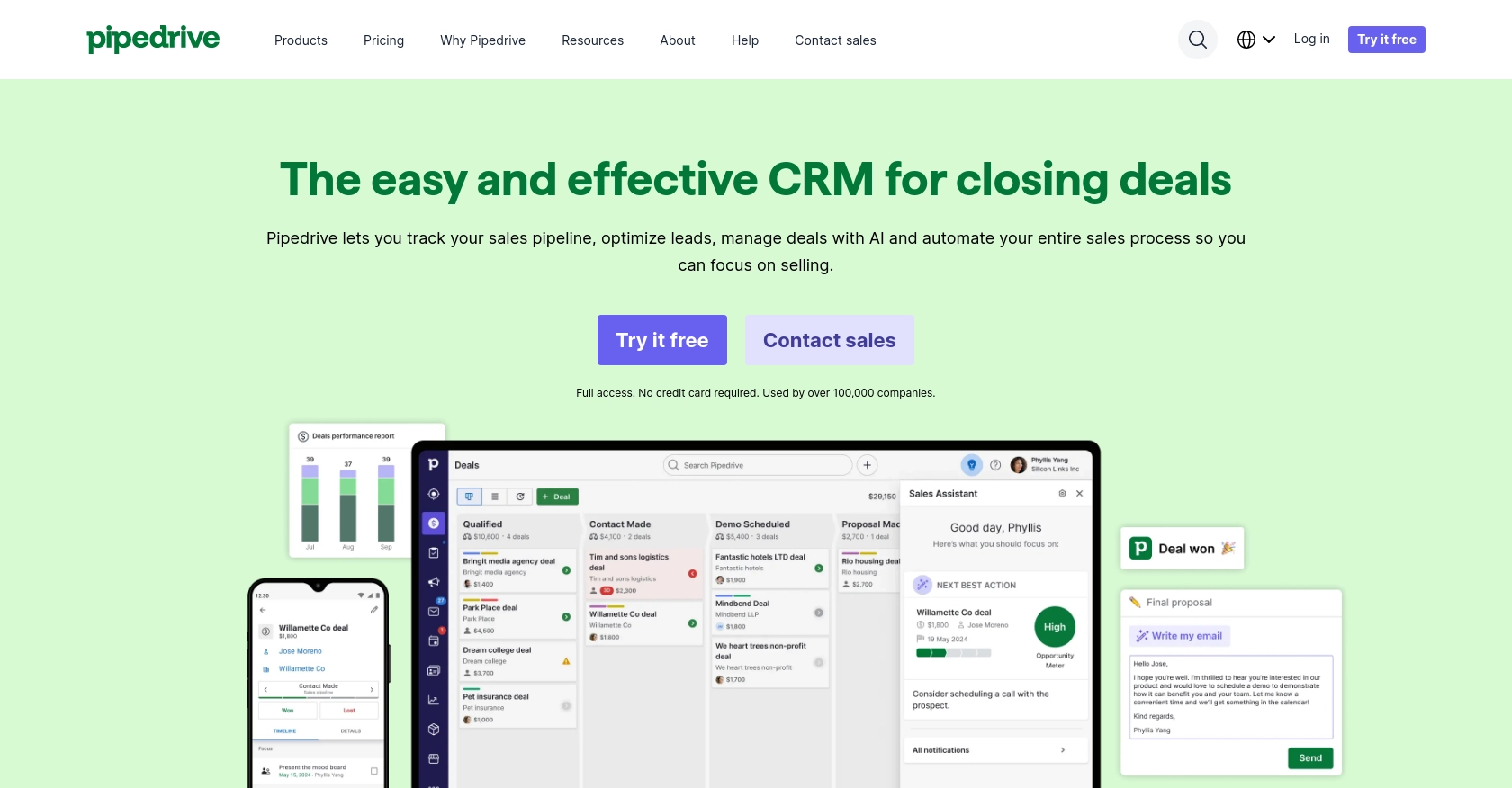
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. Known for its intuitive interface and robust features, Pipedrive enables sales teams to track deals, manage contacts, and automate workflows, making it a popular choice for organizations looking to enhance their sales operations.
Integrating with Pipedrive's API allows developers to access and manipulate data within the CRM, providing opportunities to automate tasks and streamline sales processes. For example, developers can use the Pipedrive API to retrieve organization data, enabling them to sync CRM information with other business systems or generate detailed sales reports.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start interacting with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a risk-free environment where you can test and develop your integrations without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox page.
- Fill out the form to request access to a sandbox account.
- Once your request is approved, you'll receive an email with instructions to access your sandbox account.
The sandbox account functions like a regular Pipedrive account but is limited to five seats, allowing you to safely test your applications.
Generating OAuth Credentials for Pipedrive API
Since Pipedrive uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- Log in to your Pipedrive Developer Hub using your sandbox account credentials.
- Navigate to the "Apps" section and click on "Create an App."
- Fill in the required details for your app, such as the name and description.
- After creating the app, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for OAuth authentication.
Configuring OAuth Scopes and Permissions
To ensure your app has the necessary permissions to access organization data, configure the OAuth scopes:
- In the app settings, navigate to the "Scopes" section.
- Select the appropriate scopes that your app requires, such as "read" and "write" permissions for organizations.
- Save your changes to update the app's permissions.
Importing Sample Data into Your Pipedrive Sandbox
To simulate real-world scenarios, you can import sample data into your sandbox account:
- Download the sample data spreadsheets from the Pipedrive documentation.
- In your sandbox account, go to the "More" menu and select "Import data."
- Choose "From a spreadsheet" and follow the prompts to upload your sample data.
This data will help you test your API interactions effectively.
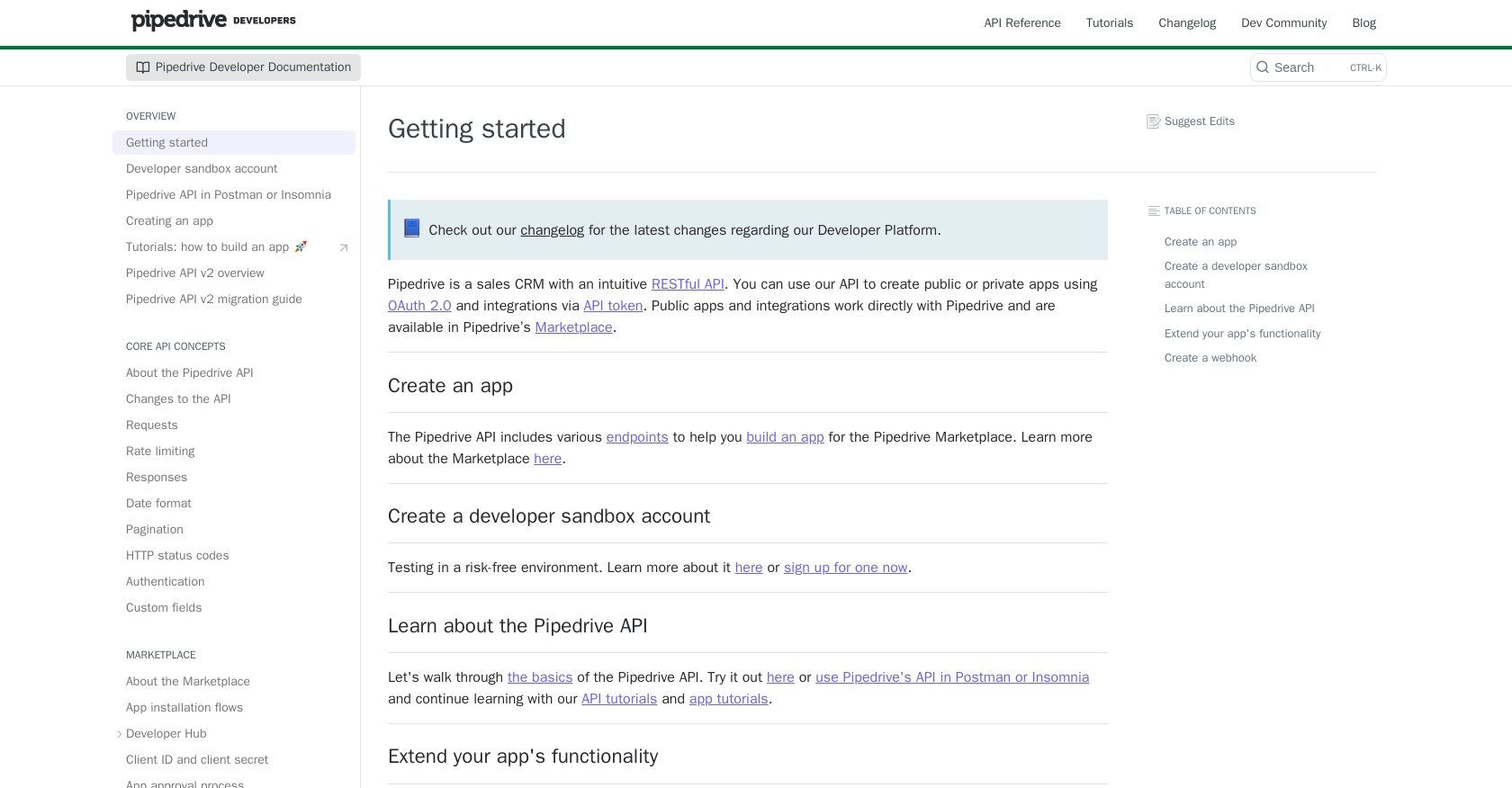
sbb-itb-96038d7
Making API Calls to Retrieve Organizations with Pipedrive API in Python
To interact with the Pipedrive API using Python, you'll need to ensure you have the correct environment set up. This section will guide you through the necessary steps to make API calls to retrieve organization data from Pipedrive.
Setting Up Your Python Environment for Pipedrive API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Organizations from Pipedrive
With your environment ready, you can now write the Python code to interact with the Pipedrive API. The following example demonstrates how to retrieve all organizations:
import requests
# Define the API endpoint and headers
endpoint = "https://api.pipedrive.com/v1/organizations"
headers = {
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for organization in data["data"]:
print(f"Organization Name: {organization['name']}")
else:
print(f"Failed to retrieve organizations: {response.status_code}")
Replace Your_Access_Token
with the access token obtained from your OAuth setup.
Understanding the API Response and Handling Errors
After executing the code, you should see the names of the organizations printed in your terminal. If the request fails, the status code will help you diagnose the issue. Common status codes include:
- 200 OK: The request was successful.
- 401 Unauthorized: The access token is invalid or expired.
- 429 Too Many Requests: The rate limit has been exceeded. Refer to the rate limiting documentation for more details.
Verifying API Call Success in Pipedrive Sandbox
To confirm that your API call was successful, log in to your Pipedrive sandbox account and navigate to the Organizations section. You should see the organizations listed as returned by your API call.
Handling Pipedrive API Rate Limits
Pipedrive enforces rate limits on API calls. For OAuth apps, the limit is 80 requests per 2 seconds per access token. If you exceed this limit, you'll receive an HTTP 429 error. To avoid this, consider implementing strategies such as:
- Batching requests to reduce the number of API calls.
- Using webhooks to receive updates instead of polling the API.
- Upgrading your plan for higher rate limits if necessary.
For more information, refer to the Pipedrive rate limiting documentation.
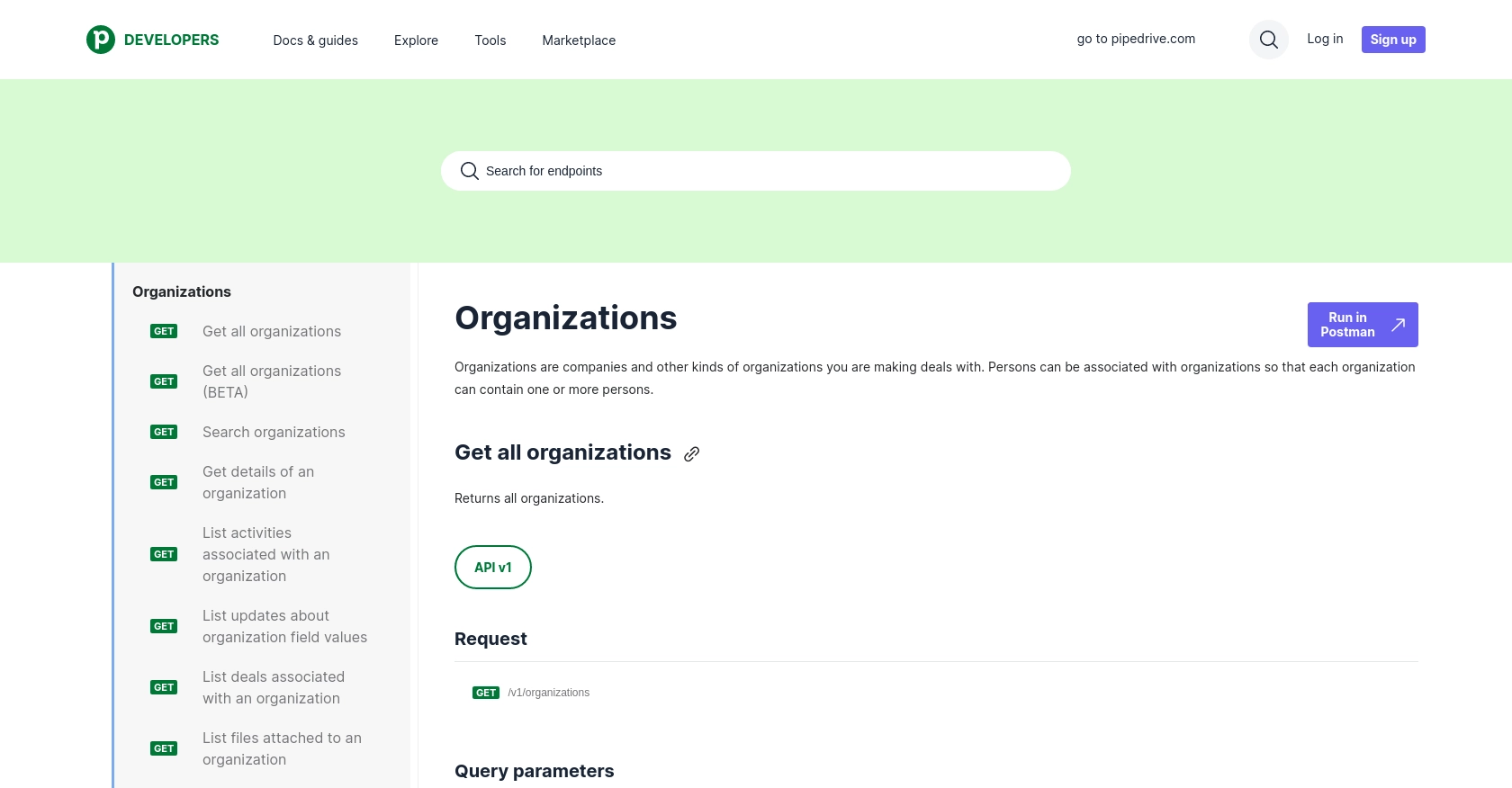
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API using Python provides a powerful way to automate and enhance your sales processes. By following the steps outlined in this guide, you can efficiently retrieve organization data and incorporate it into your business workflows.
Best Practices for Storing Pipedrive User Credentials Securely
When handling OAuth credentials, ensure they are stored securely. Consider using environment variables or secure vaults to keep your Client ID and Client Secret safe. Avoid hardcoding these credentials in your source code.
Managing Pipedrive API Rate Limits Effectively
To prevent hitting rate limits, implement strategies such as batching requests and using webhooks. Monitor your API usage and adjust your integration to optimize performance and stay within the allowed limits.
Standardizing and Transforming Data Fields from Pipedrive
When integrating Pipedrive data with other systems, ensure consistent data formats. Transform and standardize fields as needed to maintain data integrity across platforms.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate. With Endgrate, you can streamline your integration process, saving time and resources. Focus on your core product while Endgrate handles the complexities of connecting with platforms like Pipedrive.
By leveraging tools like Endgrate, you can provide a seamless integration experience for your customers, allowing you to build once for each use case and scale efficiently.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Organizations
- https://developers.pipedrive.com/docs/api/v1/OrganizationFields
- https://developers.pipedrive.com/docs/api/v1/OrganizationRelationships
Ready to get started?