How to Get Companies with the Nimble API in PHP
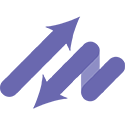
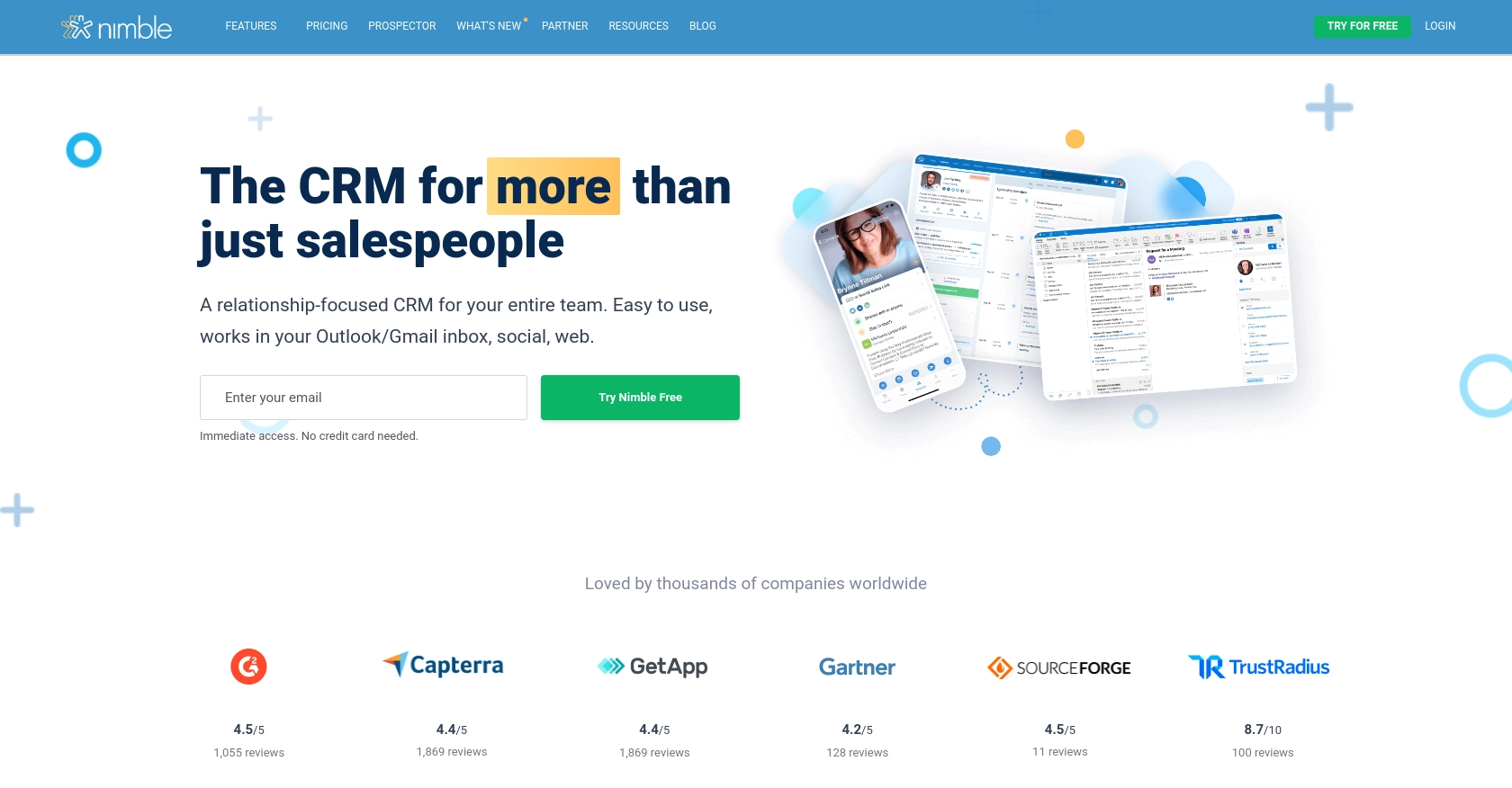
Introduction to Nimble CRM API
Nimble is a relationship-focused CRM platform that integrates seamlessly with Microsoft 365 and Google Workspace, allowing businesses to manage contacts, leads, and deals efficiently. With its intuitive interface and powerful automation tools, Nimble helps teams focus on building and nurturing customer relationships.
Developers may want to integrate with Nimble's API to access and manage company data, enabling them to automate workflows and enhance business processes. For example, using the Nimble API, a developer can retrieve a list of companies and synchronize this data with other business applications, ensuring that all systems have up-to-date information.
Setting Up Your Nimble API Test Account
Before you begin integrating with the Nimble API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. This trial will give you access to the necessary features to test the API integration.
Generate an API Key for Nimble
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps to obtain your API key:
- Log in to your Nimble account.
- Navigate to Settings and select API Token.
- Click on Generate New Token.
- Provide a description for your token and select the necessary scopes for accessing company data.
- Click Generate to create your API key.
- Copy the generated API key and store it securely, as you'll need it for API requests.
Note: Only Nimble account administrators can generate API keys. Ensure you have the necessary permissions or contact your account admin for assistance.
Authorize API Requests with the Nimble API Key
To authenticate your API requests, include the API key in the HTTP header. Use the following format:
"Authorization: Bearer YOUR_API_KEY"
Replace YOUR_API_KEY
with the API key you generated. This header must be included in all API requests to access Nimble data.
For more detailed instructions, refer to the Nimble API documentation at Generate an API key to access the Nimble API.
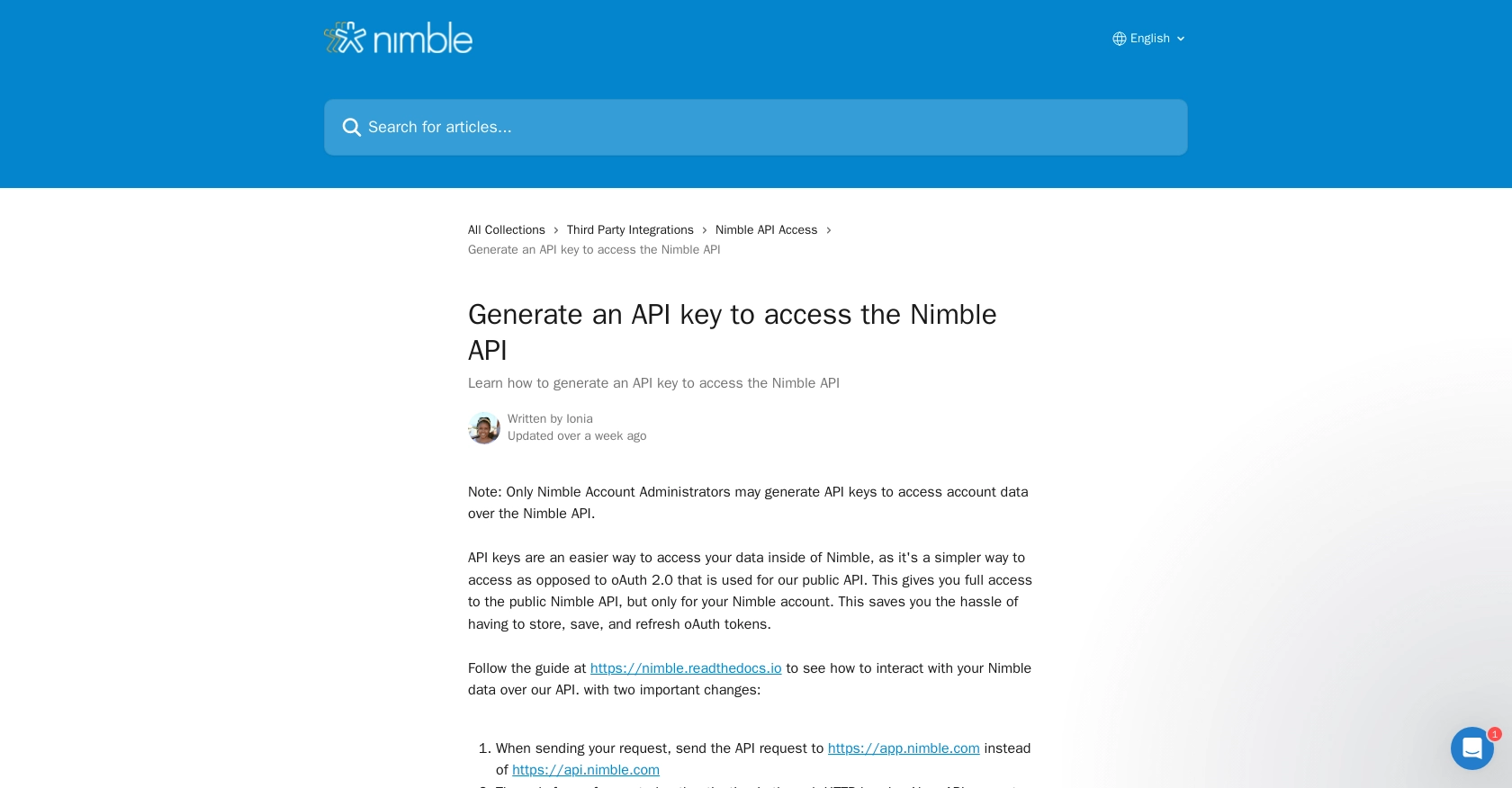
sbb-itb-96038d7
Making API Calls to Retrieve Companies with Nimble API in PHP
To interact with the Nimble API using PHP, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls to retrieve company data from Nimble.
Prerequisites for Using PHP with Nimble API
Before proceeding, ensure you have the following installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Example Code to Retrieve Companies from Nimble API
Once you have the prerequisites set up, you can use the following PHP code to make a GET request to the Nimble API and retrieve a list of companies:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'YOUR_API_KEY';
$endpoint = 'https://app.nimble.com/api/v1/companies';
try {
$response = $client->request('GET', $endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['resources'] as $company) {
echo 'Company Name: ' . $company['fields']['company name'][0]['value'] . "\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace YOUR_API_KEY
with the API key you generated earlier. This code initializes a Guzzle client, sets up the necessary headers, and makes a GET request to the Nimble API to fetch company data.
Handling API Responses and Errors
After executing the API call, the response will contain the company data. You can iterate over the data to extract and display the information you need. If the request fails, the catch block will handle the error, and you can inspect the error message for troubleshooting.
For more information on handling errors, refer to the Nimble API documentation at Nimble API Access.
Verifying API Call Success in Nimble
To verify that your API call was successful, you can log into your Nimble account and check the company data. The retrieved data should match the information displayed in your Nimble dashboard.
Conclusion and Best Practices for Using Nimble API in PHP
Integrating with the Nimble API using PHP allows developers to efficiently manage company data, automate workflows, and enhance business processes. By following the steps outlined in this guide, you can successfully retrieve and interact with company information from Nimble.
Best Practices for Storing Nimble API Credentials
- Store your API key securely and avoid hardcoding it in your source code. Consider using environment variables or a secure vault.
- Regularly rotate your API keys to enhance security.
Handling Nimble API Rate Limits
While interacting with the Nimble API, be mindful of any rate limits that may apply. Implement error handling to gracefully manage rate limit errors and consider implementing exponential backoff strategies to retry requests.
Data Transformation and Standardization
When integrating data from Nimble with other systems, ensure that data fields are transformed and standardized to maintain consistency across platforms. This will help in seamless data synchronization and reporting.
Leverage Endgrate for Simplified Integrations
If managing multiple integrations becomes complex, consider using Endgrate to streamline your integration processes. Endgrate offers a unified API endpoint that connects to various platforms, including Nimble, allowing you to focus on your core product while outsourcing integration tasks.
Visit Endgrate to learn more about how you can save time and resources by simplifying your integration efforts.
Read More
Ready to get started?