Using the Postgres API to Get Records in Javascript
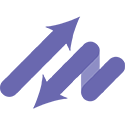
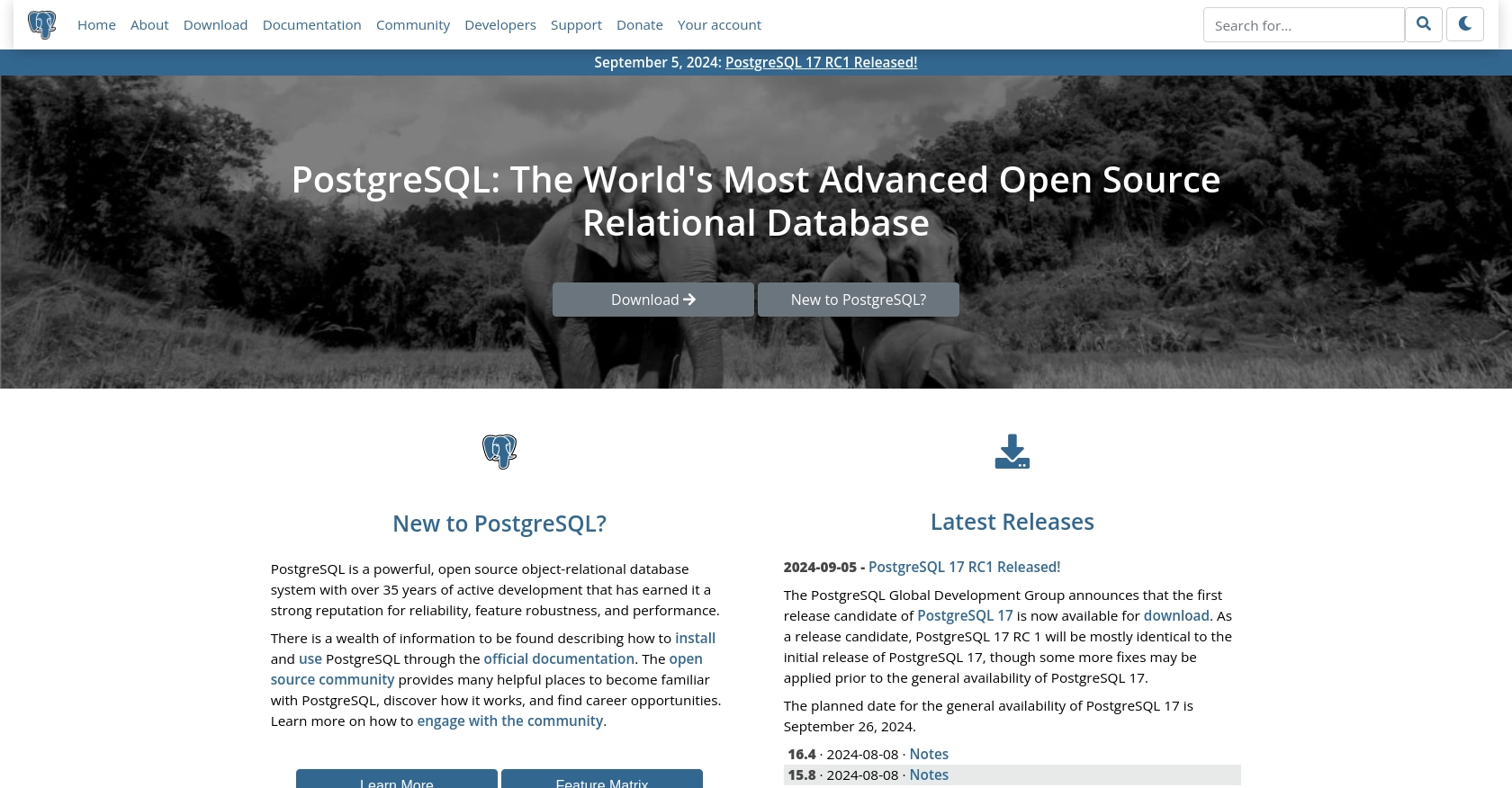
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and extensive feature set. It supports a wide range of data types and offers advanced functionalities such as full-text search, custom data types, and more. PostgreSQL is widely used by developers and organizations to manage and store data efficiently.
Integrating with the PostgreSQL API allows developers to interact programmatically with the database, enabling operations such as retrieving, updating, and managing records. For example, a developer might use the PostgreSQL API to fetch records for data analysis or to synchronize data between different systems. This capability is crucial for applications that require real-time data access and manipulation.
Setting Up a PostgreSQL Test Environment for API Integration
Before you can start interacting with the PostgreSQL API using JavaScript, it's essential to set up a test environment. This will allow you to experiment with API calls without affecting your production data. PostgreSQL provides a robust platform for creating and managing databases, making it ideal for testing purposes.
Creating a PostgreSQL Test Database
To begin, you'll need to create a test database in PostgreSQL. Follow these steps to set up your environment:
- Install PostgreSQL on your local machine or use a cloud-based service like AWS RDS or Heroku Postgres.
- Open the PostgreSQL command-line interface (psql) or a GUI tool like pgAdmin.
- Create a new database by executing the following command:
CREATE DATABASE test_db;
This command creates a new database named test_db
that you can use for testing API interactions.
Configuring User Roles and Permissions
Next, configure user roles and permissions to ensure secure access to your test database:
- Create a new user with limited permissions:
CREATE USER test_user WITH PASSWORD 'securepassword';
- Grant the necessary privileges to the user:
GRANT CONNECT ON DATABASE test_db TO test_user;
GRANT USAGE ON SCHEMA public TO test_user;
GRANT SELECT, INSERT, UPDATE, DELETE ON ALL TABLES IN SCHEMA public TO test_user;
These commands ensure that test_user
can connect to the database and perform basic operations.
Setting Up API Access with Custom Authentication
PostgreSQL does not have a built-in API, but you can create one using server-side languages like Node.js or frameworks like Express.js. Here's a basic setup:
- Install Node.js and Express.js on your machine.
- Create a new project and install the necessary dependencies:
mkdir postgres-api
cd postgres-api
npm init -y
npm install express pg
- Create a basic Express.js server to handle API requests:
const express = require('express');
const { Pool } = require('pg');
const app = express();
const pool = new Pool({
user: 'test_user',
host: 'localhost',
database: 'test_db',
password: 'securepassword',
port: 5432,
});
app.get('/records', async (req, res) => {
try {
const result = await pool.query('SELECT * FROM your_table');
res.json(result.rows);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This code sets up a simple API endpoint to fetch records from your test database. Replace your_table
with the actual table name you want to query.
With this setup, you can now test API calls to your PostgreSQL database using JavaScript. This environment provides a safe space to develop and refine your integration logic.
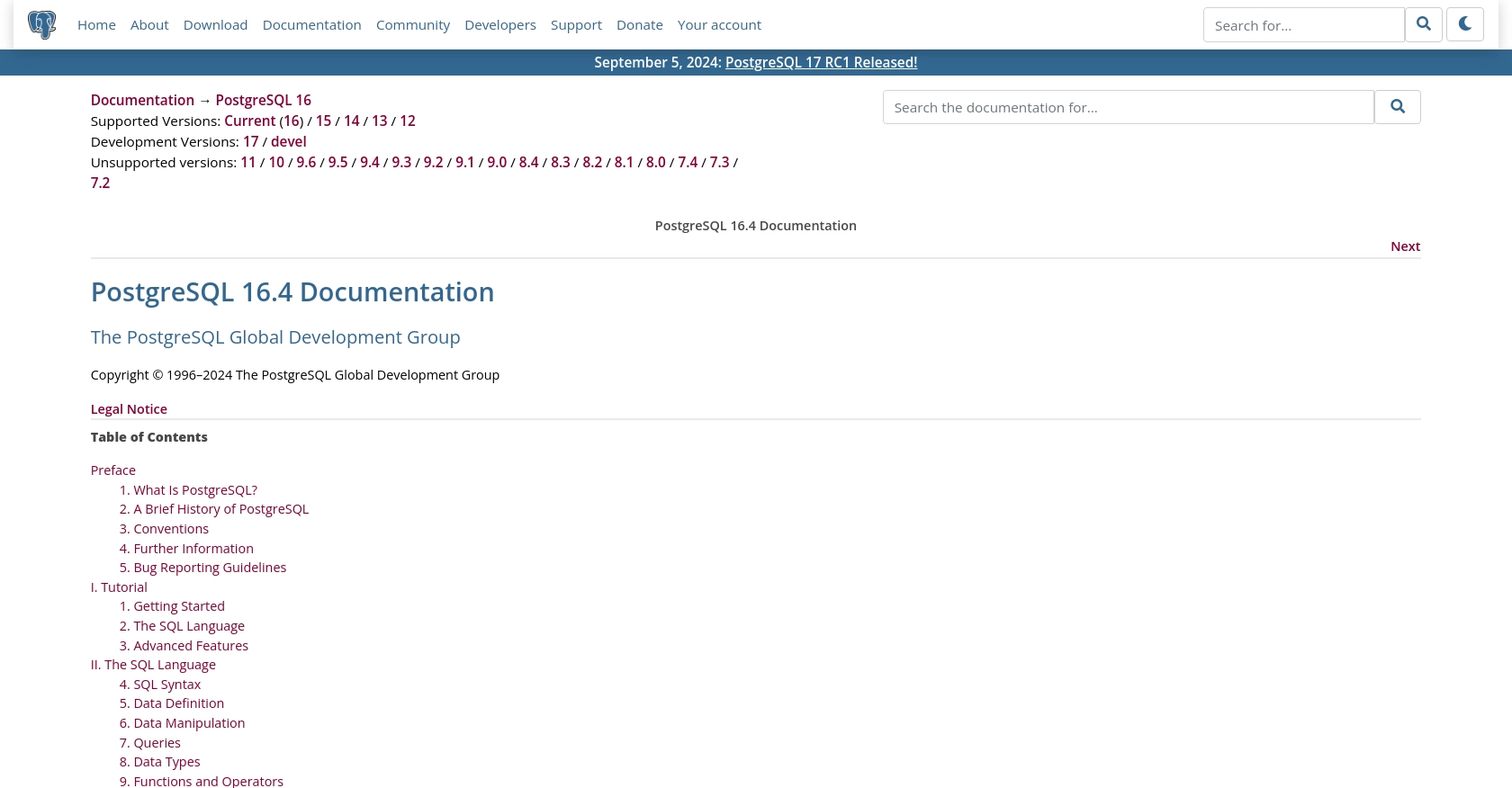
sbb-itb-96038d7
Executing API Calls to Retrieve Records from PostgreSQL Using JavaScript
To interact with PostgreSQL and retrieve records using JavaScript, you'll need to set up your environment and write code that communicates with the database. This section will guide you through the process of making API calls to PostgreSQL using Node.js and Express.js.
Setting Up Your JavaScript Environment for PostgreSQL API Calls
Before making API calls, ensure you have the necessary tools and libraries installed:
- Node.js: Ensure you have Node.js installed on your machine. You can download it from the official website.
- Express.js: This framework will help you create a server to handle API requests.
- pg: This is a PostgreSQL client for Node.js, which allows you to interact with your PostgreSQL database.
Install the required packages by running the following command in your project directory:
npm install express pg
Writing JavaScript Code to Fetch Records from PostgreSQL
With your environment set up, you can now write the code to fetch records from your PostgreSQL database. Follow these steps:
- Create a new file named
app.js
in your project directory. - Add the following code to set up a basic Express.js server and connect to PostgreSQL:
const express = require('express');
const { Pool } = require('pg');
const app = express();
const pool = new Pool({
user: 'test_user',
host: 'localhost',
database: 'test_db',
password: 'securepassword',
port: 5432,
});
app.get('/records', async (req, res) => {
try {
const result = await pool.query('SELECT * FROM your_table');
res.json(result.rows);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Replace your_table
with the name of the table you wish to query. This code sets up an endpoint at /records
that fetches all records from the specified table.
Running Your JavaScript Application and Testing API Calls
To run your application and test the API call, follow these steps:
- Start your server by running the following command in your terminal:
node app.js
- Open your browser or a tool like Postman and navigate to
http://localhost:3000/records
.
You should see a JSON response containing the records from your PostgreSQL table.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors and verify the success of your API calls:
- Check the server logs for any error messages if the API call fails.
- Ensure that the database connection details are correct and that the PostgreSQL server is running.
- Verify the output by comparing it with the expected data in your test database.
By following these steps, you can efficiently retrieve records from PostgreSQL using JavaScript, enabling seamless data integration and manipulation.
Conclusion: Best Practices for Integrating with PostgreSQL Using JavaScript
Integrating with PostgreSQL using JavaScript provides developers with a powerful way to manage and manipulate data efficiently. By following the steps outlined in this article, you can set up a robust environment for API interactions and ensure seamless data retrieval and management.
Best Practices for Secure and Efficient PostgreSQL API Integration
- Secure User Credentials: Always store user credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Although PostgreSQL itself does not impose rate limits, consider implementing your own limits to prevent overwhelming your server with requests.
- Data Standardization: Ensure that data fields are standardized and transformed as needed to maintain consistency across different systems.
- Error Handling: Implement comprehensive error handling to manage potential issues gracefully and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While building integrations manually can be effective, it can also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including PostgreSQL. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate today.
Read More
Ready to get started?