Using the Zoho Books API to Create or Update Accounts in PHP
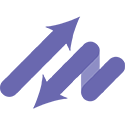
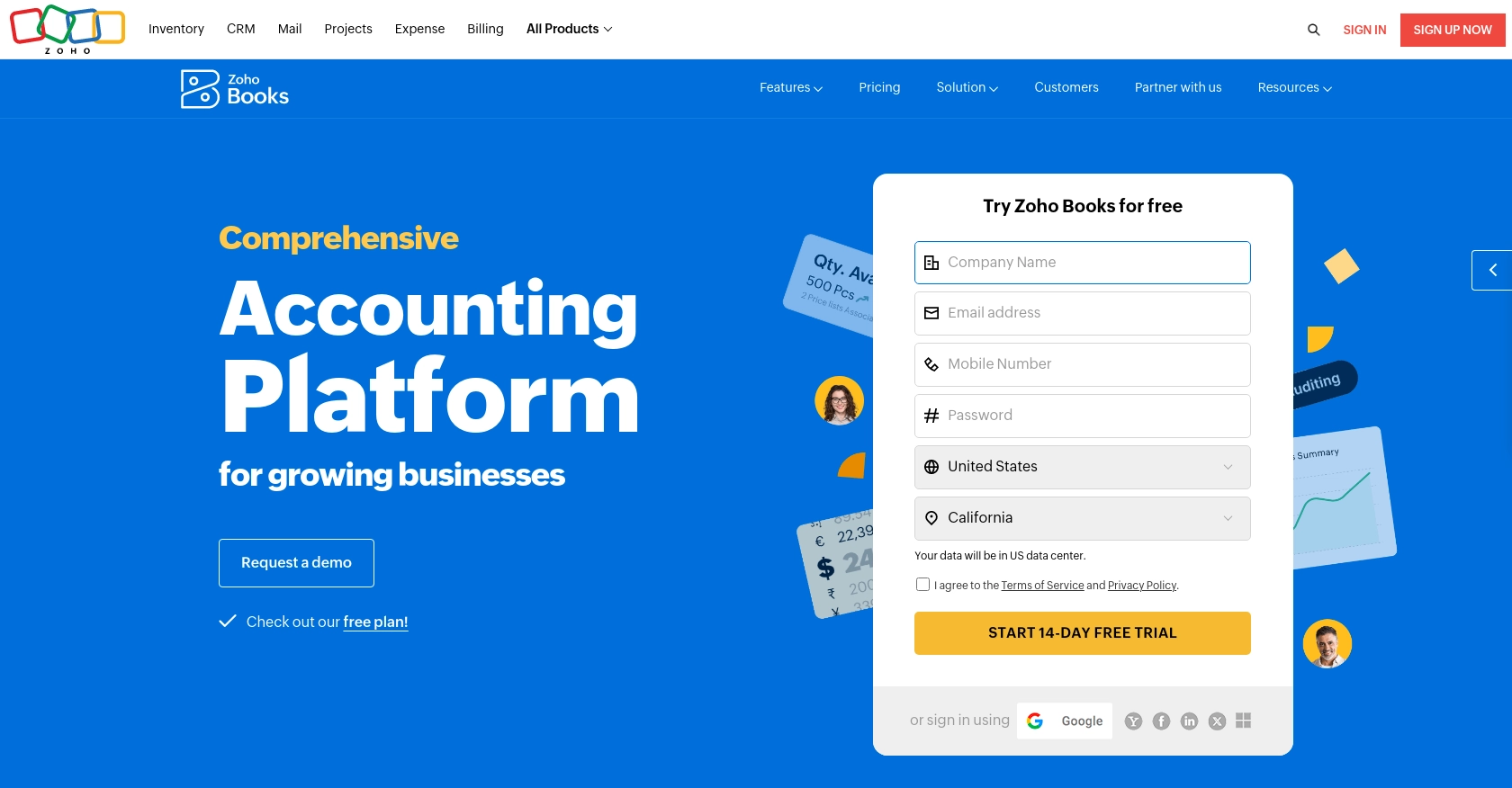
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive cloud-based accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it an ideal choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate and streamline accounting processes, such as creating or updating accounts programmatically. For example, you can use the API to automatically update account details in Zoho Books whenever changes occur in your internal systems, ensuring that your financial data remains accurate and up-to-date.
This article will guide you through using the Zoho Books API with PHP to create or update accounts, providing a step-by-step approach to setting up and executing API calls efficiently.
Setting Up a Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API using PHP, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Zoho Books provides a free trial that you can use for this purpose.
Step-by-Step Guide to Creating a Zoho Books Account
- Visit the Zoho Books website and click on the "Free Trial" button.
- Fill out the registration form with the required details, including your email address and password.
- Once registered, you'll receive a confirmation email. Click the link in the email to verify your account.
- Log in to your Zoho Books account and navigate to the dashboard.
Configuring OAuth for Zoho Books API Access
The Zoho Books API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth and obtain the necessary credentials:
- Go to the Zoho Developer Console and click on "Add Client ID".
- Fill in the required details, such as the client name and redirect URI, and submit the form.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Generating OAuth Tokens for Zoho Books API
With your Client ID and Client Secret, you can now generate OAuth tokens:
- Redirect users to the following URL to obtain a grant token:
- After user consent, you'll receive a code in the redirect URI. Use this code to request an access token:
- Store the access token securely. It will be used in the Authorization header for API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
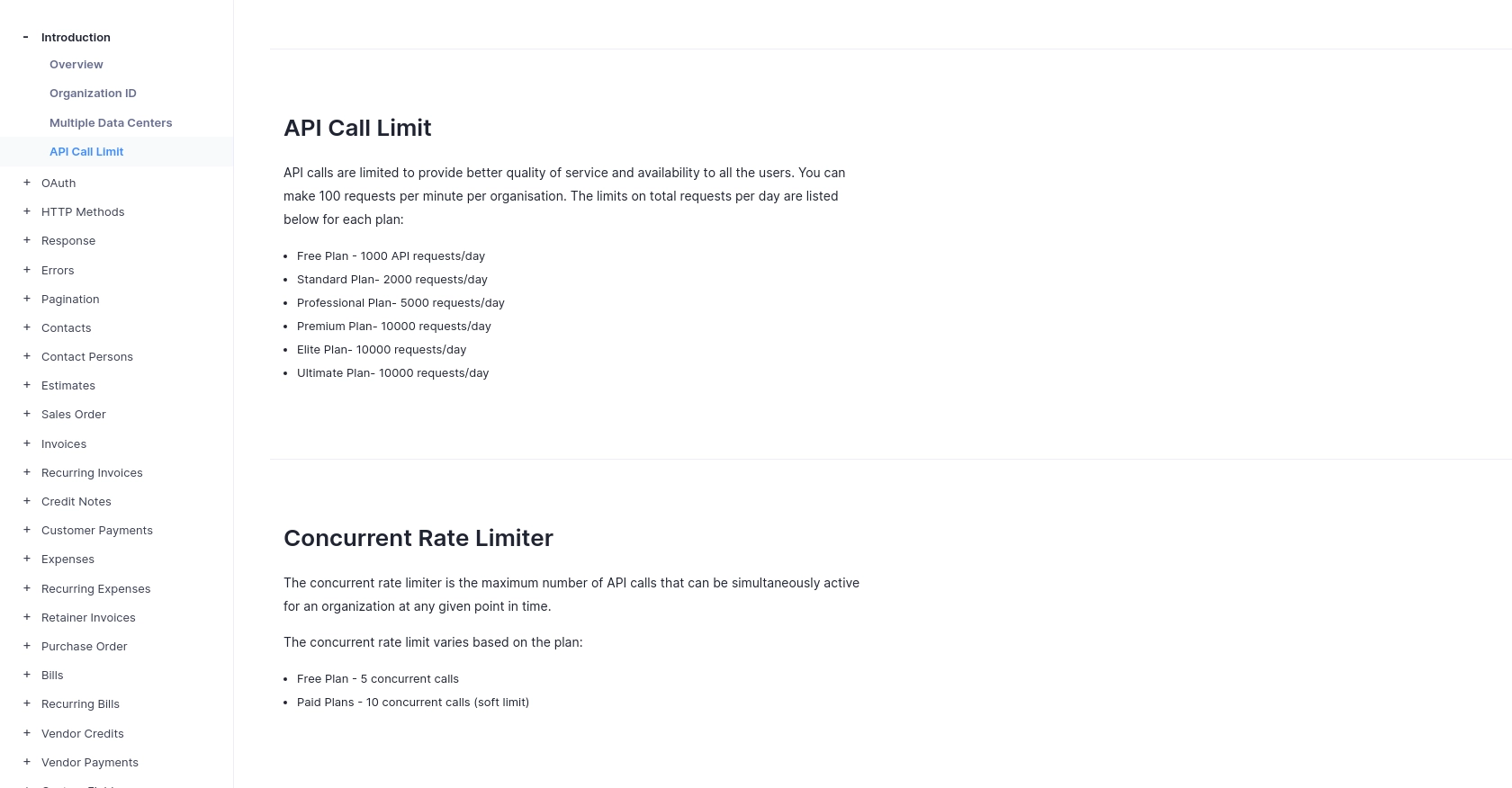
sbb-itb-96038d7
Making API Calls to Zoho Books Using PHP
To interact with the Zoho Books API and perform operations such as creating or updating accounts, you'll need to set up your PHP environment and execute API calls. This section will guide you through the necessary steps to achieve this.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled. You can check your PHP version and extensions using the following command:
php -v
php -m | grep curl
Installing Required PHP Dependencies
To make HTTP requests, you'll use the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Creating a New Account in Zoho Books Using PHP
Here's how to create a new account in Zoho Books using the API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://www.zohoapis.com/books/v3/chartofaccounts', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'query' => [
'organization_id' => 'YOUR_ORGANIZATION_ID'
],
'json' => [
'account_name' => 'New Account',
'account_type' => 'income',
'currency_id' => '460000000000097',
'description' => 'Description of the new account'
]
]);
echo $response->getBody();
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID.
Updating an Existing Account in Zoho Books Using PHP
To update an existing account, use the following code:
$response = $client->put('https://www.zohoapis.com/books/v3/chartofaccounts/{account_id}', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'query' => [
'organization_id' => 'YOUR_ORGANIZATION_ID'
],
'json' => [
'account_name' => 'Updated Account Name',
'description' => 'Updated description'
]
]);
echo $response->getBody();
Replace {account_id}
with the ID of the account you wish to update.
Handling API Responses and Errors
Zoho Books API uses HTTP status codes to indicate success or failure. Here are some common codes:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 429 Rate Limit Exceeded: Too many requests.
For more details, refer to the Zoho Books Error Documentation.
Verifying API Call Success in Zoho Books
After making API calls, verify the changes in your Zoho Books account. For account creation, check the Chart of Accounts section to see the new account. For updates, ensure the account details reflect the changes.
By following these steps, you can efficiently integrate with the Zoho Books API using PHP, automating your accounting processes and ensuring data accuracy.
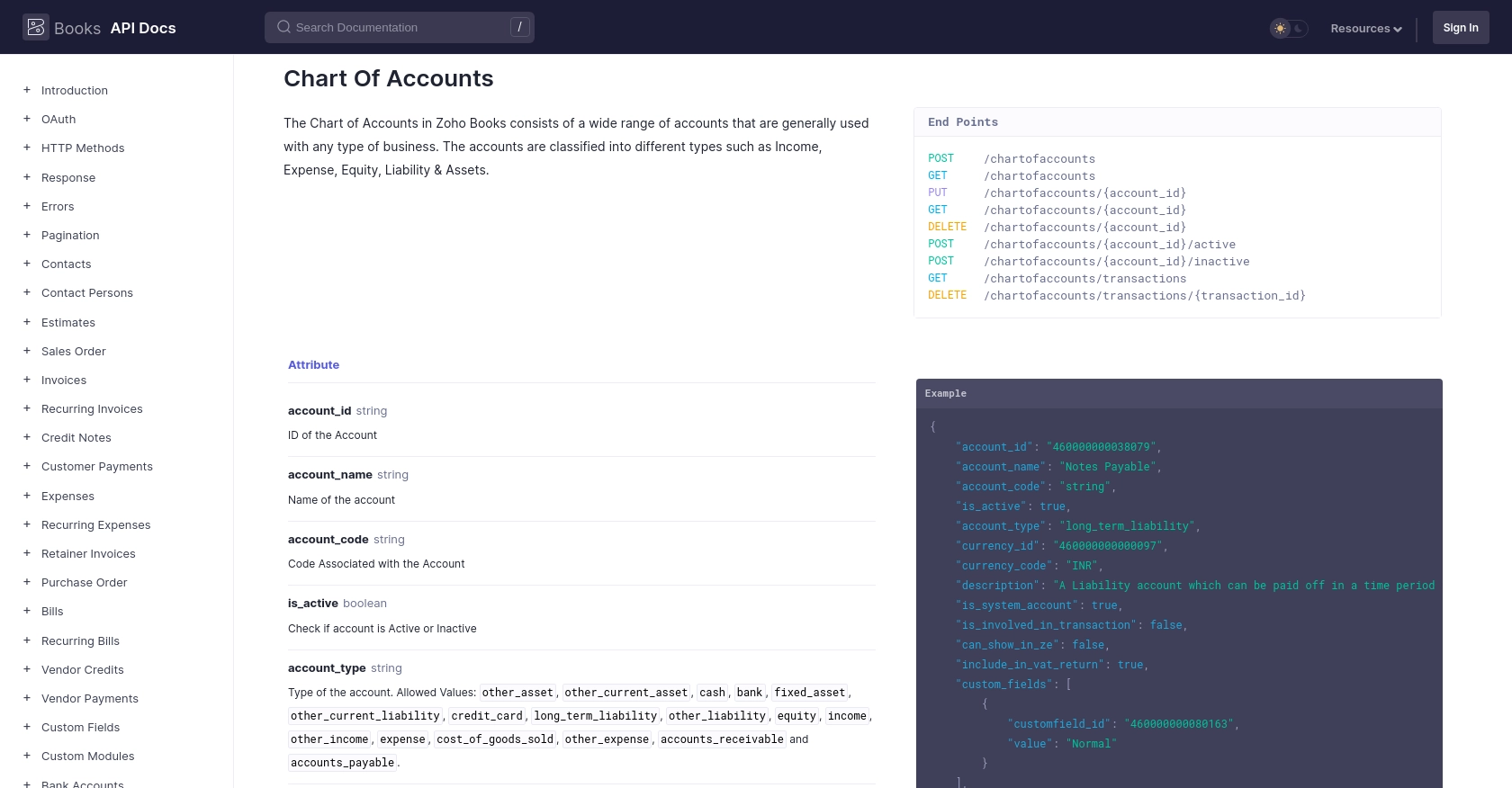
Best Practices for Zoho Books API Integration in PHP
When integrating with the Zoho Books API using PHP, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and access tokens, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho Books API has rate limits, such as 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Zoho Books API Call Limit documentation.
- Implement Error Handling: Properly handle API errors by checking HTTP status codes and response messages. Implement retry logic for transient errors and log errors for debugging purposes. Refer to the Zoho Books Error Documentation for more information.
- Data Transformation and Standardization: Ensure that data sent to and received from the API is correctly transformed and standardized to match your internal systems. This helps maintain data consistency across platforms.
Streamline Your Integration Process with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify the process by leveraging a unified API endpoint that connects to multiple platforms, including Zoho Books. This allows you to focus on your core product while outsourcing the integration work.
Endgrate offers an intuitive integration experience, enabling you to build once for each use case instead of multiple times for different integrations. This not only saves time and resources but also enhances the integration experience for your customers.
Explore how Endgrate can help you streamline your integration process by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?