How to Get Sales Orders with the Quickbooks API in PHP
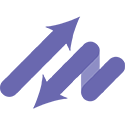
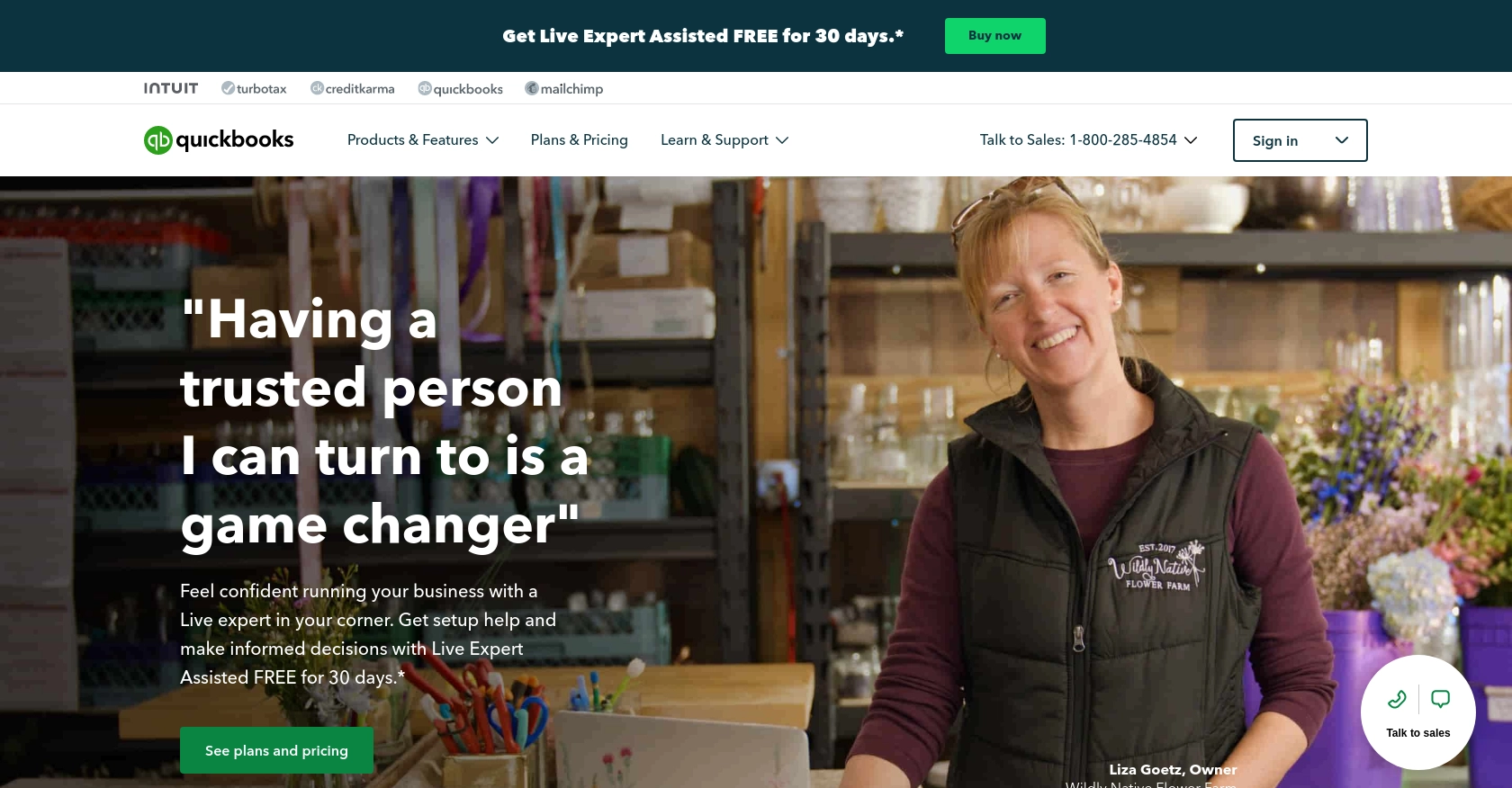
Introduction to QuickBooks API for Sales Orders
QuickBooks is a widely-used accounting software that helps businesses manage their financial operations efficiently. It offers a range of features, including invoicing, payroll, expense tracking, and sales order management, making it a popular choice for businesses of all sizes.
Integrating with the QuickBooks API allows developers to automate and streamline various accounting tasks. For example, retrieving sales orders using the QuickBooks API in PHP can help businesses keep their sales data synchronized with other systems, ensuring accurate and up-to-date financial records.
This article will guide you through the process of using PHP to interact with the QuickBooks API, specifically focusing on how to retrieve sales orders. By following this tutorial, developers can enhance their applications with seamless QuickBooks integration.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start retrieving sales orders using the QuickBooks API in PHP, you'll need to set up a QuickBooks sandbox account. This sandbox environment allows developers to test their applications without affecting live data, providing a safe space to experiment with API calls.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on the "Sign Up" button and fill out the necessary information to create your account.
- Once registered, log in to your QuickBooks Developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, set up a sandbox company to test your API integrations:
- Navigate to the "Sandbox" section in your QuickBooks Developer dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Choose the type of company you want to create and follow the prompts to complete the setup.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- Go to the "My Apps" section in your QuickBooks Developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details for your app, such as name and description.
- Once your app is created, navigate to the "Keys & OAuth" tab to find your Client ID and Client Secret.
Ensure you save these credentials securely, as they will be used to authenticate your API requests.
Configuring OAuth 2.0 Redirect URIs
To complete the OAuth setup, configure the redirect URIs for your app:
- In the "Keys & OAuth" tab, scroll down to the "Redirect URIs" section.
- Add the URI where users will be redirected after authentication. This is typically a URL on your server that handles OAuth responses.
- Save your changes.
With your QuickBooks sandbox account and app set up, you're now ready to start making API calls to retrieve sales orders using PHP.
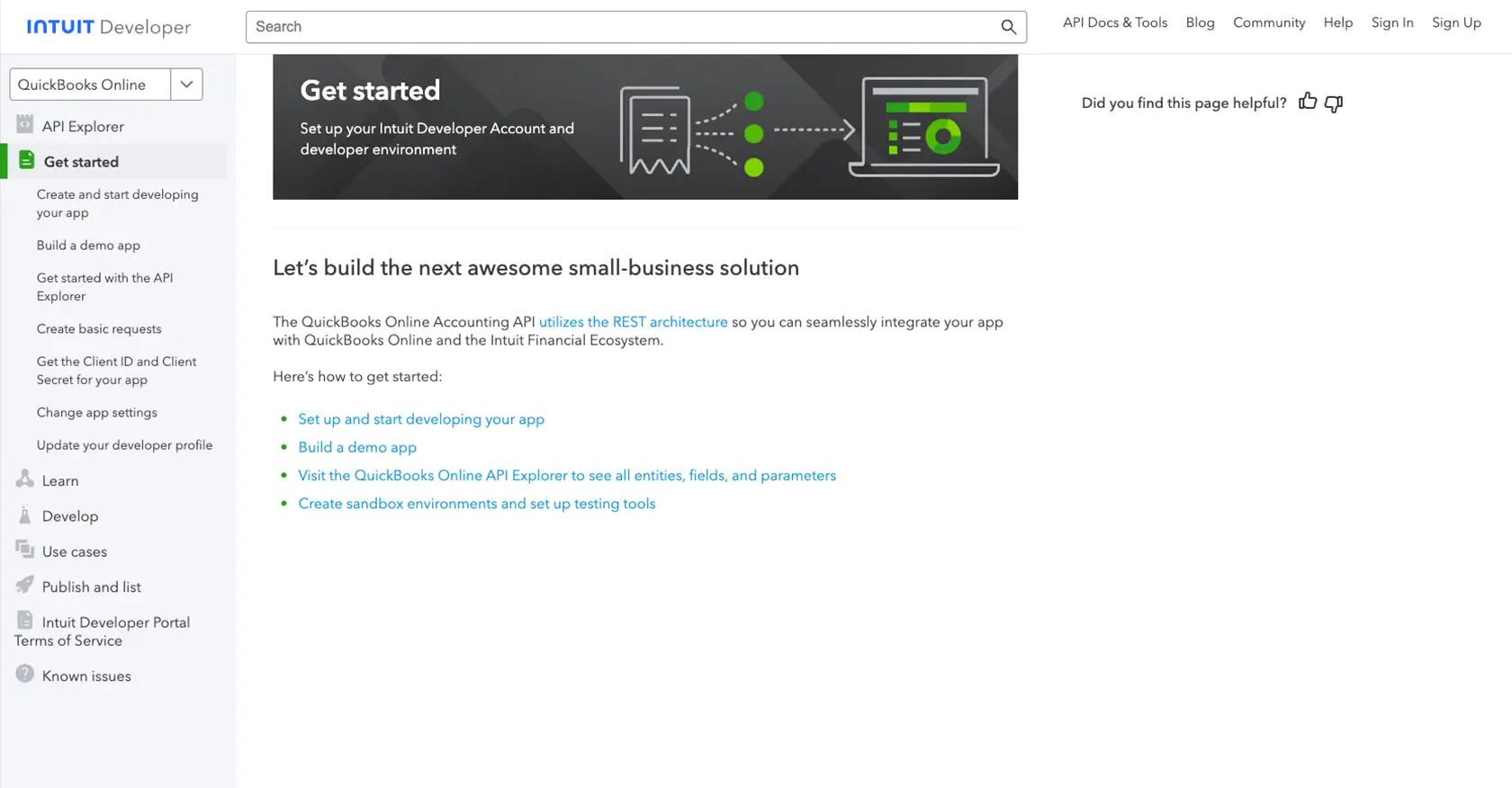
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders with QuickBooks API in PHP
To interact with the QuickBooks API and retrieve sales orders using PHP, you'll need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to fetch sales orders.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need the following:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Once you have PHP and Composer installed, create a new project directory and navigate to it in your terminal. Run the following command to initialize a new Composer project:
composer init
Next, install the guzzlehttp/guzzle
package, which will help you make HTTP requests to the QuickBooks API:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Sales Orders from QuickBooks
With your environment set up, you can now write the PHP code to interact with the QuickBooks API. Create a new PHP file named get_sales_orders.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$realmId = 'Your_Realm_ID';
$url = "https://sandbox-quickbooks.api.intuit.com/v3/company/$realmId/query?query=SELECT * FROM SalesOrder";
$response = $client->request('GET', $url, [
'headers' => [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
],
]);
if ($response->getStatusCode() === 200) {
$data = json_decode($response->getBody(), true);
foreach ($data['QueryResponse']['SalesOrder'] as $order) {
echo "Sales Order ID: " . $order['Id'] . "\n";
echo "Total Amount: " . $order['TotalAmt'] . "\n";
}
} else {
echo "Failed to retrieve sales orders. Status code: " . $response->getStatusCode();
}
Replace Your_Access_Token
and Your_Realm_ID
with your actual QuickBooks OAuth 2.0 access token and company ID, respectively.
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_sales_orders.php
If successful, the script will output the sales order IDs and their total amounts. Verify the results by checking your QuickBooks sandbox account to ensure the data matches.
Handling Errors and QuickBooks API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The QuickBooks API may return various HTTP status codes indicating different outcomes:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 403 Forbidden: You don't have permission to access the resource.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Implement error handling in your code to manage these responses appropriately and ensure a robust integration.
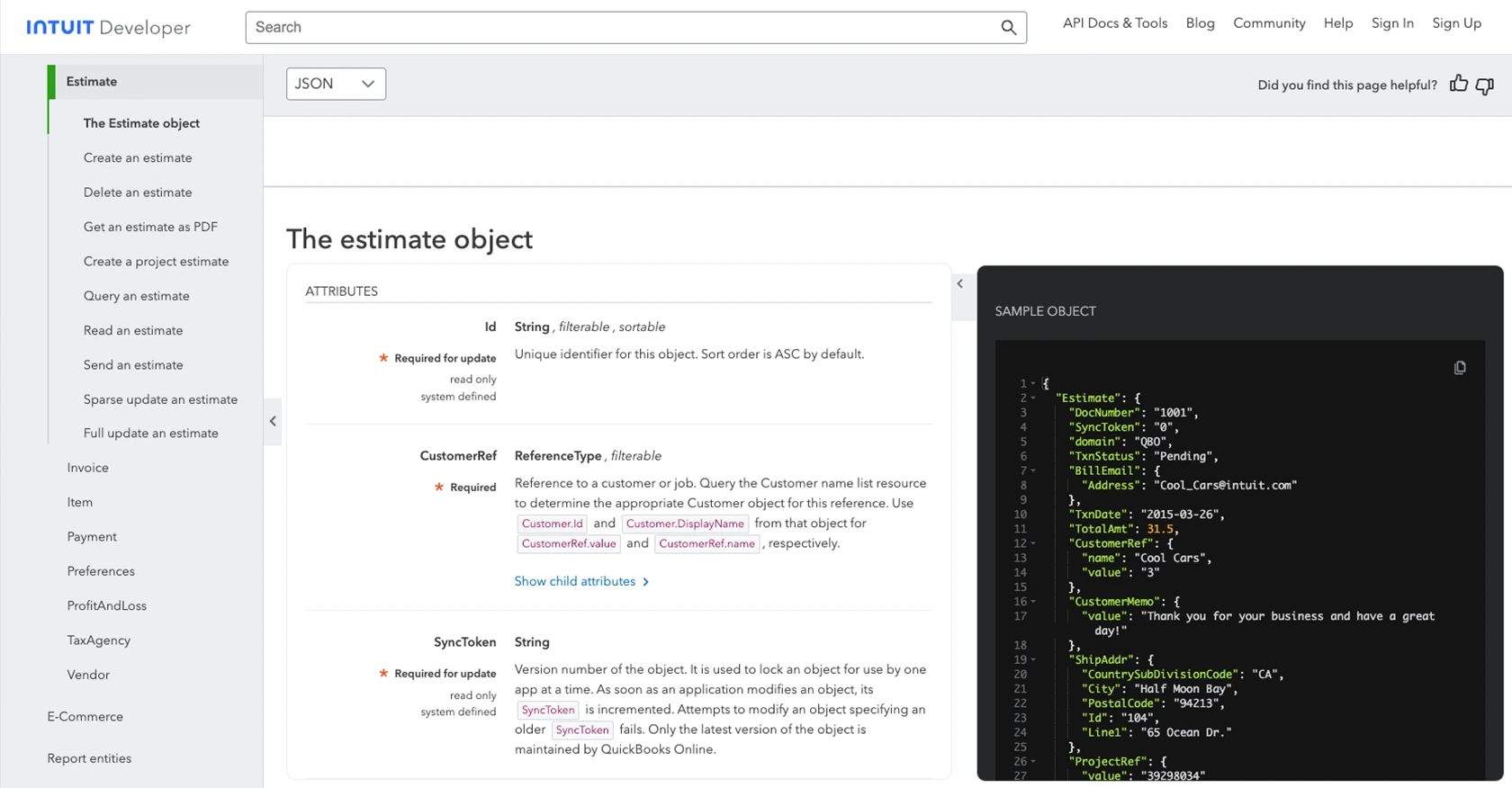
Conclusion and Best Practices for QuickBooks API Integration in PHP
Integrating with the QuickBooks API using PHP can significantly enhance your application's ability to manage financial data efficiently. By following the steps outlined in this guide, you can successfully retrieve sales orders and ensure seamless synchronization with QuickBooks.
Best Practices for Secure and Efficient QuickBooks API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: QuickBooks API has rate limits, so ensure your application handles these limits gracefully. Implement retry logic with exponential backoff to manage rate limit errors effectively.
- Standardize Data Fields: When integrating with multiple systems, standardize data fields to ensure consistency across platforms. This will help in maintaining data integrity and simplifying data transformations.
- Error Handling: Implement robust error handling to manage various API response codes. This ensures your application can gracefully handle issues like authentication failures or server errors.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including QuickBooks. Focus on your core product while Endgrate handles the intricacies of integration, saving you time and resources.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?