How to Get Users with the Hubspot API in PHP
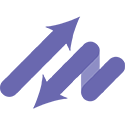
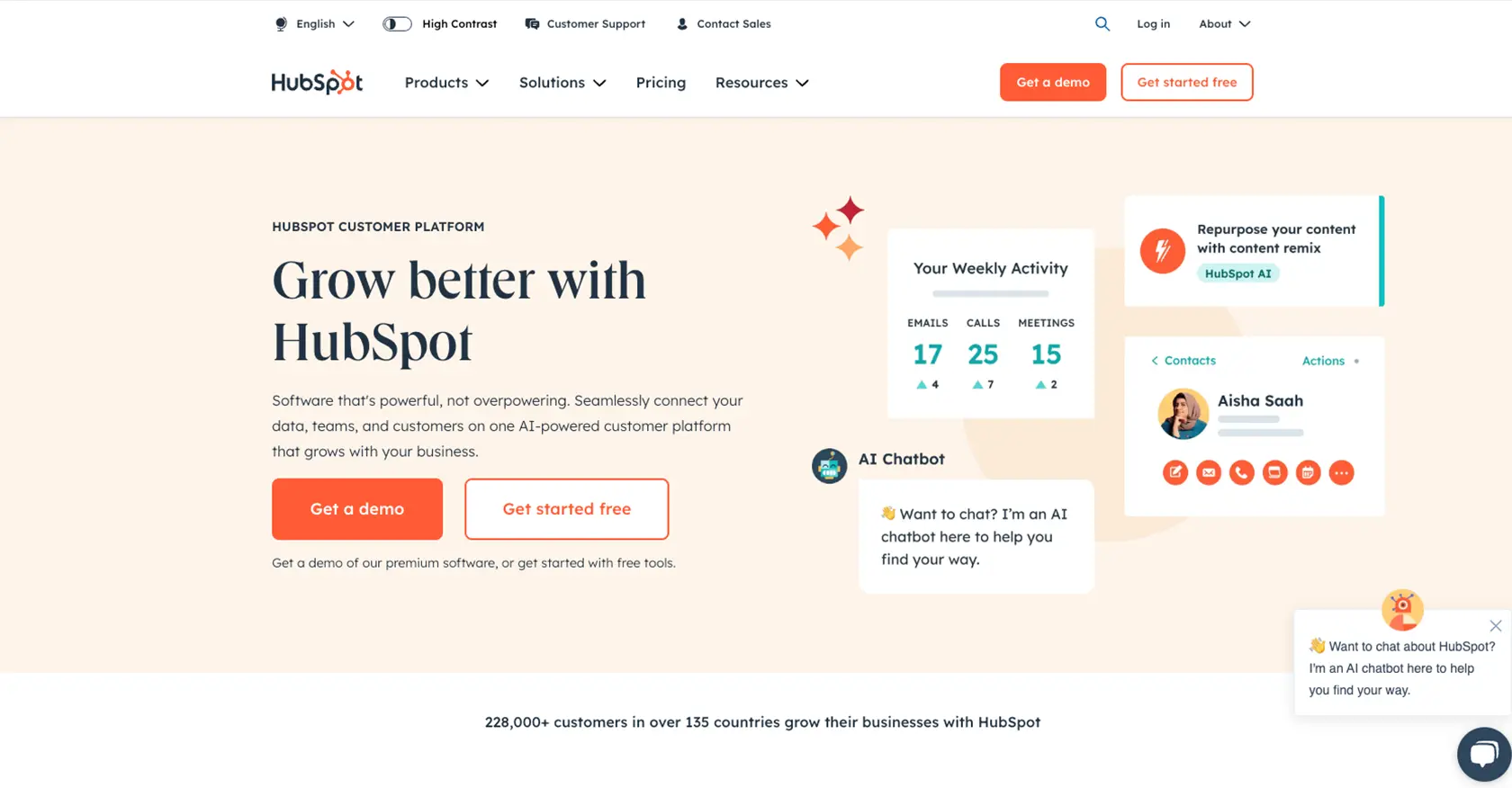
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for businesses looking to streamline their operations and improve customer relationships.
Developers often seek to integrate with HubSpot's API to access and manage user data, enabling them to enhance their applications with powerful CRM functionalities. For example, using the HubSpot API, a developer can retrieve user information to synchronize it with an external workforce management system, ensuring that user data is consistent and up-to-date across platforms.
This article will guide you through the process of using PHP to interact with the HubSpot API, specifically focusing on retrieving user data. By following these steps, you can efficiently integrate HubSpot's capabilities into your own applications, enhancing their functionality and value.
Setting Up Your HubSpot Developer Account for API Integration
Before you can start integrating with the HubSpot API using PHP, you'll need to set up a HubSpot developer account. This account will allow you to create a private app and obtain the necessary credentials for OAuth-based authentication.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
To test your API integration without affecting live data, you can use a HubSpot sandbox account. This environment allows you to safely experiment with API calls and data manipulation.
- In your developer dashboard, navigate to "Test Accounts" and create a new sandbox account.
- Follow the prompts to set up your sandbox environment, which will mimic a real HubSpot account.
Creating a HubSpot Private App for OAuth Authentication
HubSpot uses OAuth for secure API authentication. To interact with the API, you'll need to create a private app and obtain an access token.
- In your HubSpot account, go to "Settings" and select "Integrations" from the sidebar.
- Click on "Private Apps" and then "Create a private app."
- Provide a name and description for your app, then configure the necessary scopes for user data access.
- Once the app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for OAuth authentication.
Generating an OAuth Access Token
With your private app set up, you can now generate an OAuth access token:
- Use the client ID and client secret to request an access token from HubSpot's OAuth server.
- Include the access token in the authorization header of your API requests to authenticate successfully.
For more detailed information on OAuth authentication, refer to the HubSpot OAuth Documentation.
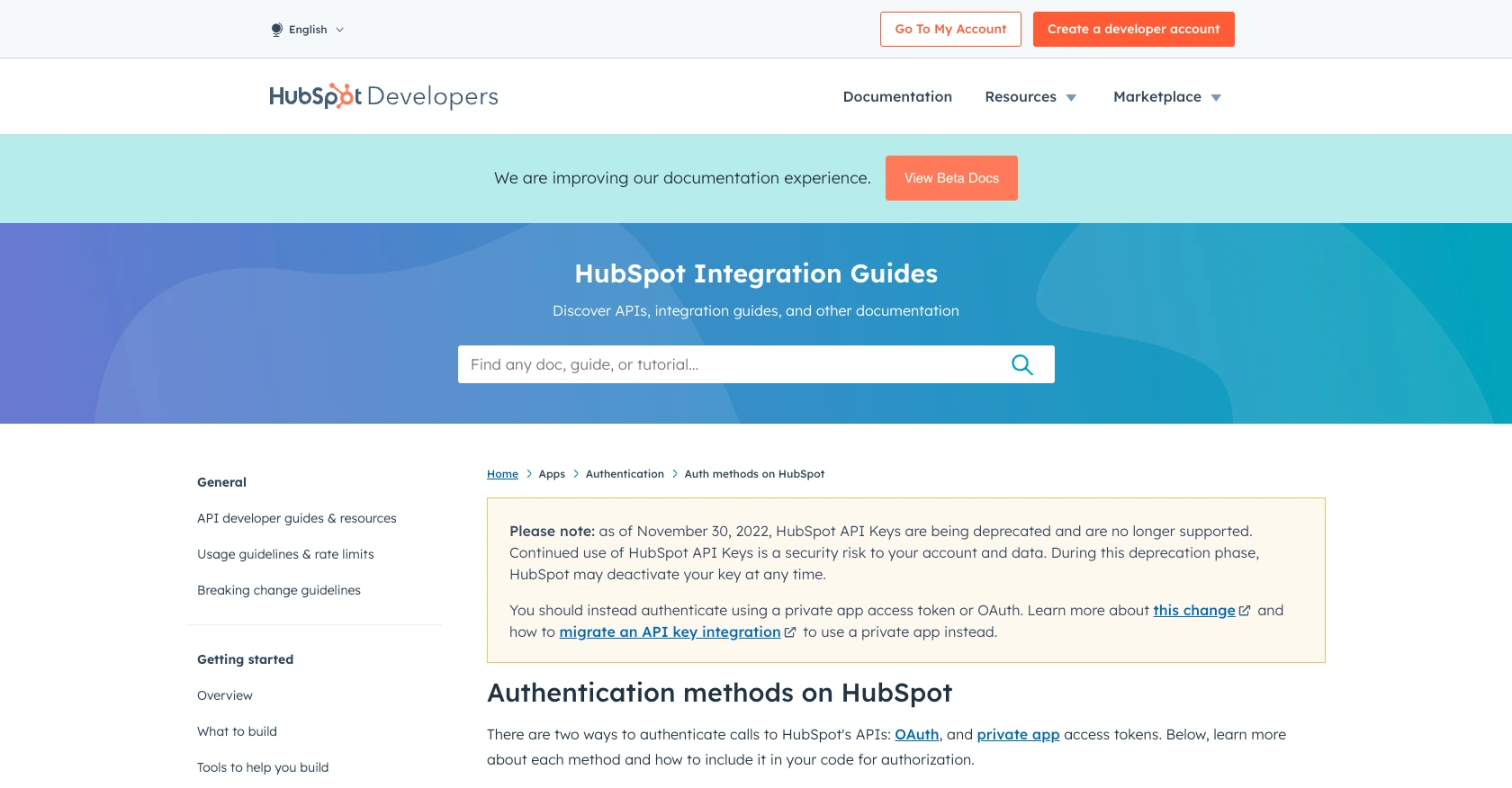
sbb-itb-96038d7
Making API Calls to Retrieve Users with HubSpot API in PHP
To interact with the HubSpot API using PHP, you'll need to set up your environment and write the necessary code to make API calls. This section will guide you through the process of retrieving user data from HubSpot using PHP.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP installed on your system, along with the Composer package manager to handle dependencies.
- Ensure PHP is installed by running
php -v
in your terminal. - Install Composer by following the instructions on the Composer website.
- Use Composer to install the HubSpot API client for PHP by running the command:
composer require hubspot/api-client
.
Writing PHP Code to Retrieve Users from HubSpot
With your environment set up, you can now write the PHP code to retrieve users from HubSpot. The following example demonstrates how to make a GET request to the HubSpot API to fetch user data.
require 'vendor/autoload.php';
use HubSpot\Factory;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('YOUR_ACCESS_TOKEN');
// Define the endpoint to retrieve users
$endpoint = '/crm/v3/objects/users';
// Make the API call
$response = $client->apiRequest()->get($endpoint);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
$users = json_decode($response->getBody(), true);
foreach ($users['results'] as $user) {
echo 'User ID: ' . $user['id'] . "\n";
echo 'Created At: ' . $user['createdAt'] . "\n";
echo 'Updated At: ' . $user['updatedAt'] . "\n";
}
} else {
echo 'Failed to retrieve users. Status Code: ' . $response->getStatusCode();
}
Replace 'YOUR_ACCESS_TOKEN'
with the OAuth access token you generated earlier. This code initializes the HubSpot client, defines the endpoint for retrieving users, and makes the API call. If successful, it will print out user details such as ID, creation date, and last updated date.
Verifying API Call Success and Handling Errors
After running the code, verify the output to ensure the API call was successful. The user data should match the information in your HubSpot sandbox account. If the request fails, the code will output the status code, which can help diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 429 Too Many Requests: You have exceeded the rate limit. HubSpot allows 100 requests every 10 seconds for OAuth apps. Consider implementing request throttling.
For more detailed error handling, refer to the HubSpot Users API documentation.
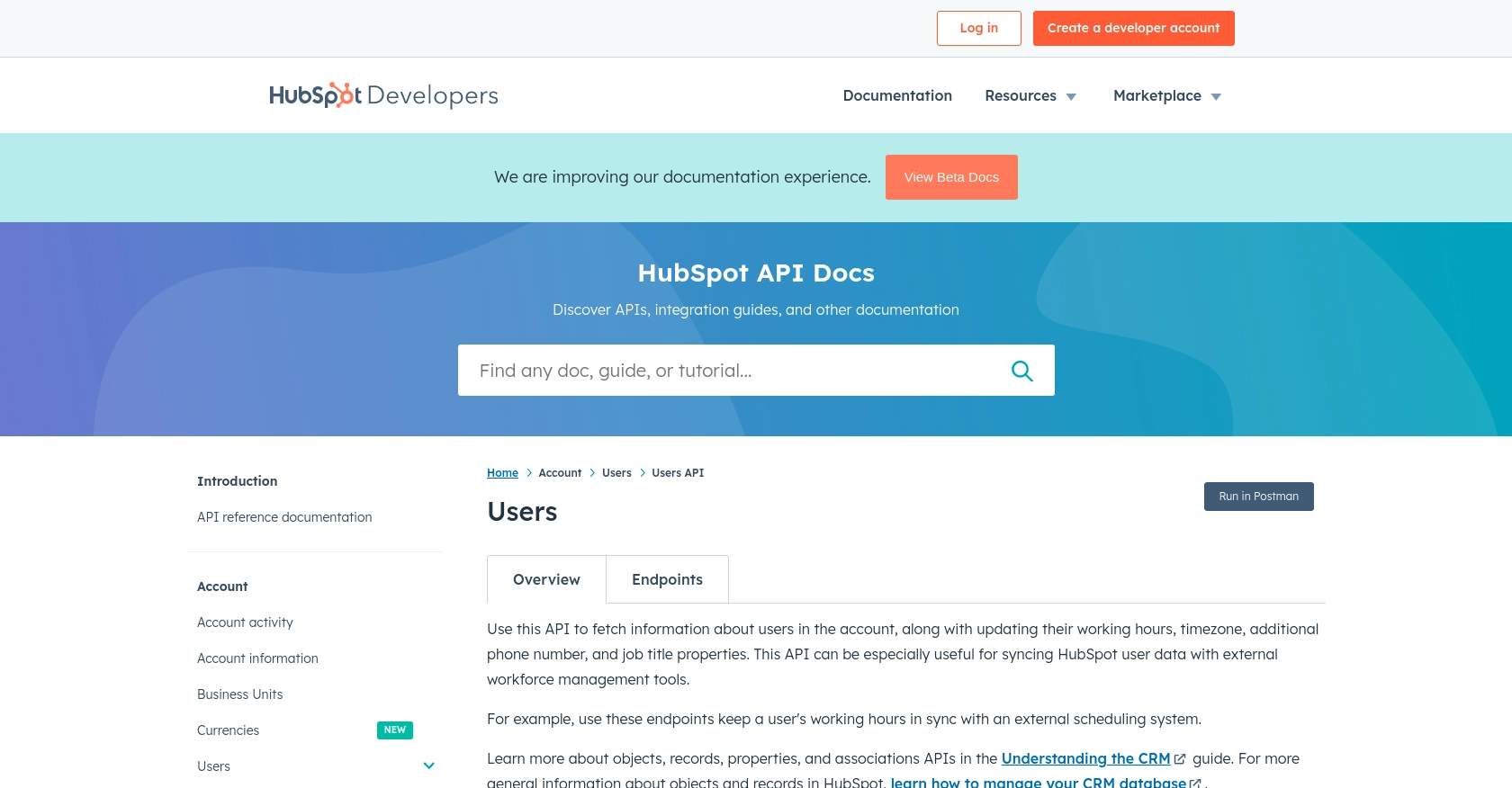
Conclusion and Best Practices for HubSpot API Integration in PHP
Integrating with the HubSpot API using PHP provides a powerful way to enhance your applications with robust CRM functionalities. By following the steps outlined in this guide, you can efficiently retrieve user data and synchronize it with external systems, ensuring data consistency and improving operational efficiency.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store Credentials: Always store your OAuth access tokens and client secrets securely. Consider using environment variables or a secure vault to protect sensitive information.
- Implement Rate Limiting: To avoid hitting HubSpot's rate limits, implement request throttling. HubSpot allows 100 requests every 10 seconds for OAuth apps. Monitor your API usage and adjust your request frequency accordingly.
- Handle Errors Gracefully: Implement comprehensive error handling to manage common issues such as authentication errors or rate limit exceedances. Use the status codes provided by the API to diagnose and resolve issues promptly.
- Standardize Data Fields: When integrating with multiple systems, standardize data fields to ensure consistency across platforms. This practice simplifies data management and reduces the risk of errors.
Enhancing Your Integration Strategy with Endgrate
While integrating with HubSpot's API can significantly enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including HubSpot.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your integration strategy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?