How to Get Users with the Salesflare API in Python
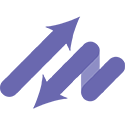
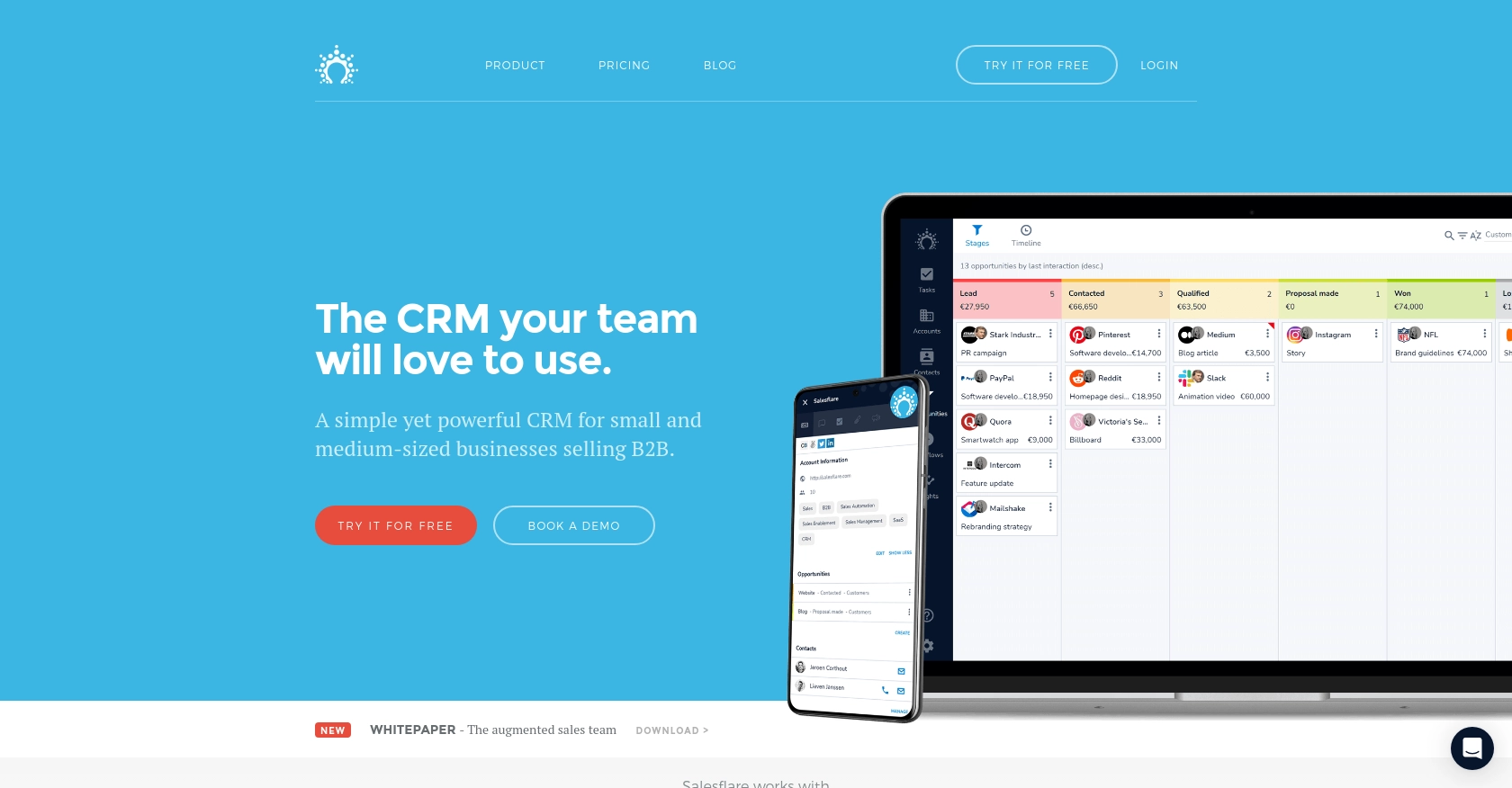
Introduction to Salesflare API
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a range of features, including contact management, email tracking, and sales automation, all aimed at enhancing productivity and efficiency.
Integrating with the Salesflare API allows developers to access and manage user data programmatically, enabling seamless integration with other tools and systems. For example, a developer might use the Salesflare API to retrieve user information and synchronize it with a custom dashboard, providing sales teams with real-time insights and analytics.
Setting Up Your Salesflare Test Account
Before you can start interacting with the Salesflare API, you need to set up a test account. Salesflare offers a free trial that allows developers to explore its features and test API integrations without any initial cost.
Creating a Salesflare Account
To begin, visit the Salesflare website and sign up for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you will have access to the Salesflare dashboard.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Salesflare account.
- Navigate to the "Settings" section from the dashboard.
- Find the "API Keys" tab and click on it.
- Click on "Generate New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
Configuring Your Development Environment
Ensure that you have Python installed on your machine. You will also need the requests
library to make HTTP requests. Install it using the following command:
pip install requests
With your Salesflare account and API key ready, you can now proceed to make API calls and interact with user data programmatically.
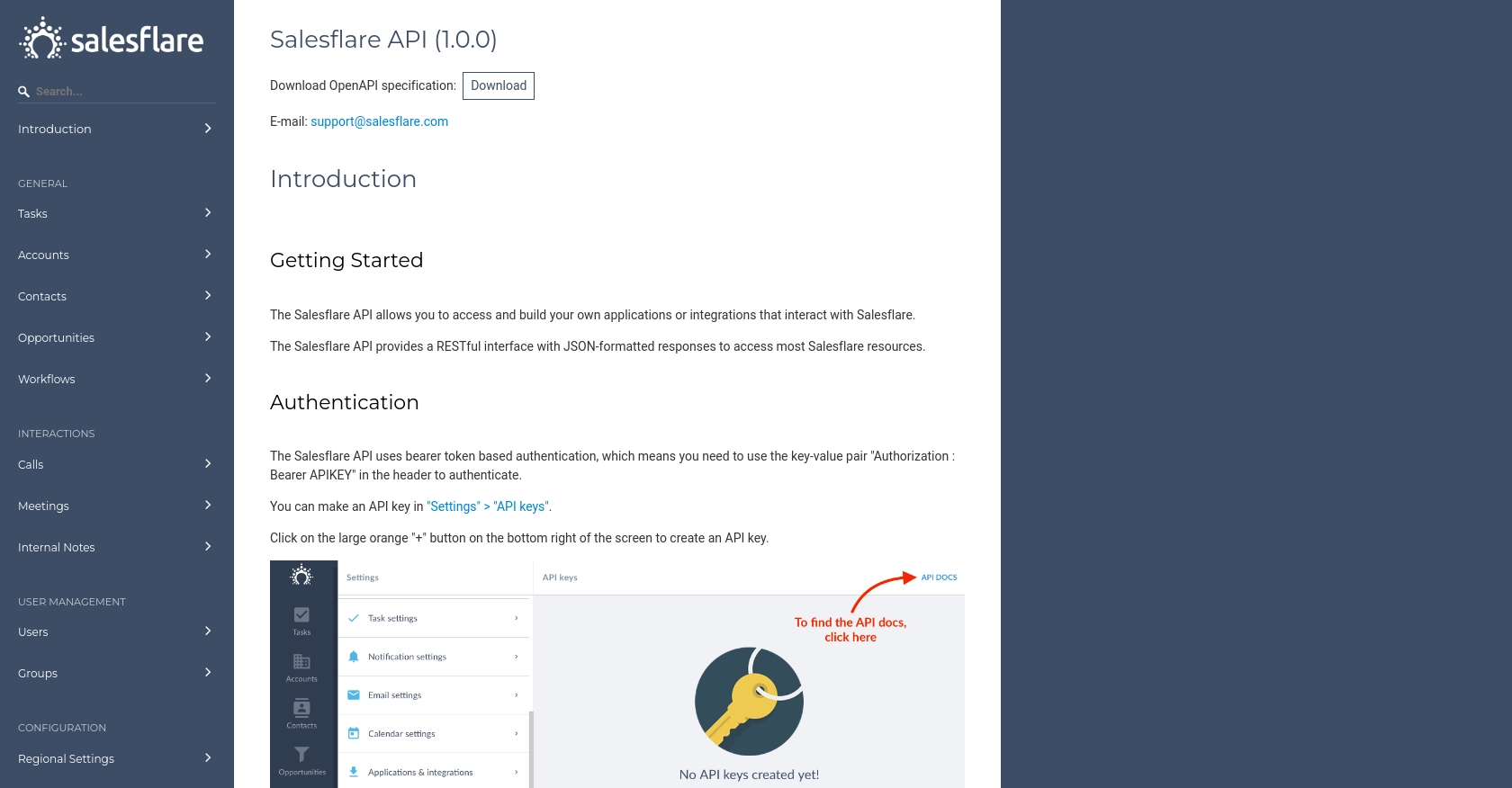
sbb-itb-96038d7
Making API Calls to Retrieve Users with Salesflare API in Python
To interact with the Salesflare API and retrieve user data, you need to set up your development environment and write a Python script to make the necessary API calls. This section will guide you through the process, ensuring you can efficiently access user information from Salesflare.
Setting Up Your Python Environment for Salesflare API Integration
Before making API calls, ensure you have Python installed on your machine. You will also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Users from Salesflare API
With your environment ready, you can now write a Python script to retrieve users from Salesflare. Create a file named get_salesflare_users.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.salesflare.com/users"
headers = {
"Authorization": "Bearer Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the users and print their information
for user in data["users"]:
print(user)
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated from your Salesflare account. This script sends a GET request to the Salesflare API to fetch user data. If successful, it prints the user information; otherwise, it displays an error message.
Running Your Python Script to Access Salesflare User Data
Execute the script from your terminal or command line using the following command:
python get_salesflare_users.py
If the request is successful, you should see a list of users from your Salesflare account displayed in the terminal. This confirms that your API call was successful and that you can access user data programmatically.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The script above checks the response status code to determine if the request was successful. If the status code is not 200, it prints an error message with the status code and response text. Always ensure that your API key is valid and that you have the necessary permissions to access user data.
For more details on handling errors and understanding response codes, refer to the Salesflare API documentation.
Conclusion: Best Practices for Using Salesflare API in Python
Integrating with the Salesflare API using Python can significantly enhance your application's capabilities by providing seamless access to user data. To ensure a smooth integration process, consider the following best practices:
Securely Storing Salesflare API Credentials
Always store your API keys securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information. This practice helps prevent unauthorized access and protects your data.
Handling Salesflare API Rate Limits
Be mindful of the API rate limits imposed by Salesflare to avoid exceeding the allowed number of requests. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more information on rate limits, refer to the Salesflare API documentation.
Transforming and Standardizing Salesflare User Data
When retrieving user data, consider transforming and standardizing the data fields to match your application's requirements. This ensures consistency and simplifies data processing and analysis.
Enhancing Integration with Endgrate
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations and focusing on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience, allowing you to build once for each use case and easily connect with various platforms, including Salesflare.
By following these best practices, you can efficiently integrate with the Salesflare API and enhance your application's functionality, providing valuable insights and automation for your sales processes.
Read More
Ready to get started?