How to Create Task with the Wrike API in Javascript
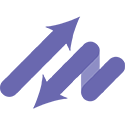
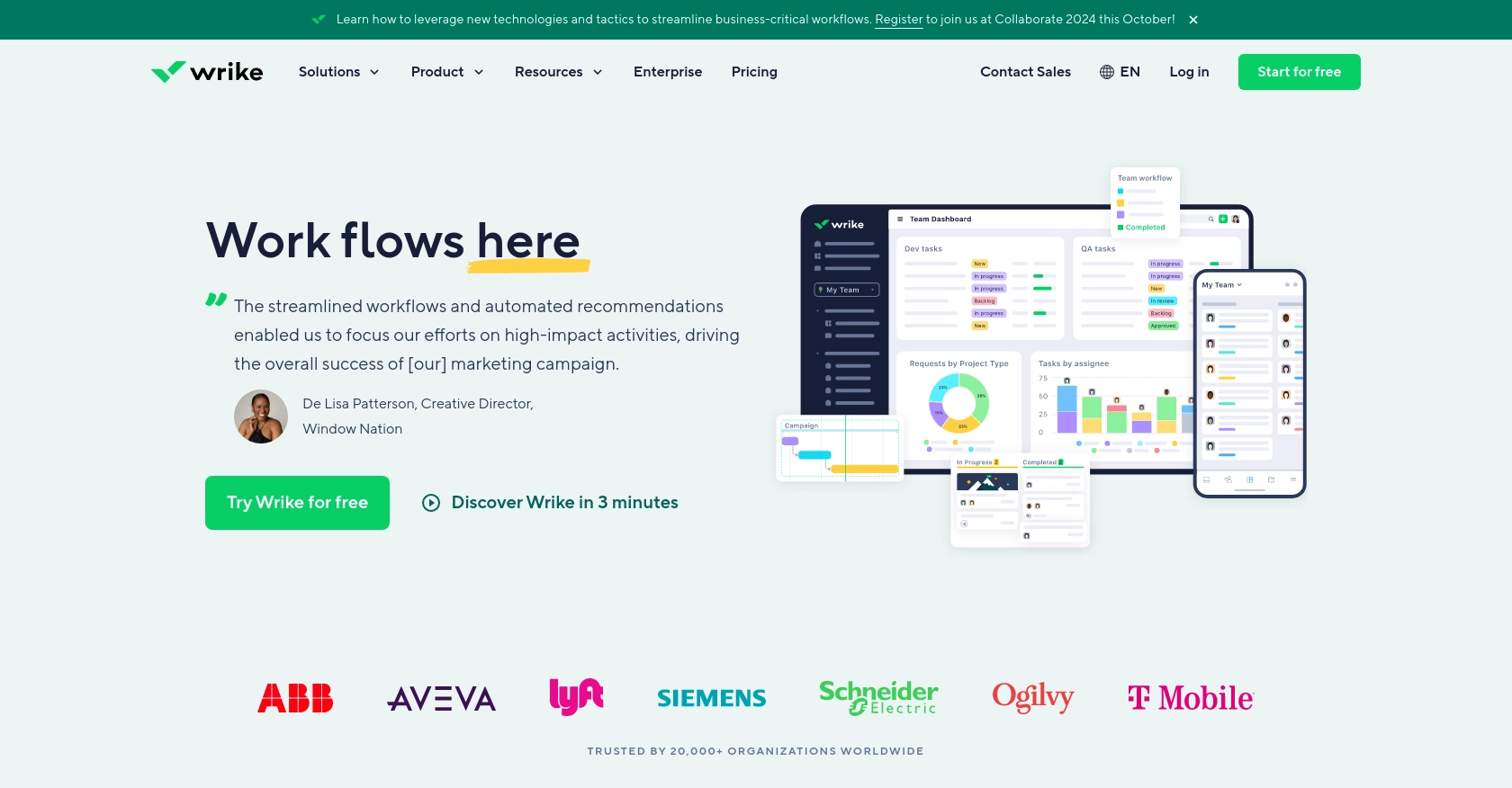
Introduction to Wrike API Integration
Wrike is a powerful project management and collaboration platform that helps teams streamline their workflows and enhance productivity. With features like task management, time tracking, and real-time collaboration, Wrike is a popular choice for businesses looking to optimize their project management processes.
Integrating with Wrike's API allows developers to automate and customize their project management tasks. For example, you can create tasks programmatically within Wrike using JavaScript, enabling seamless integration with other applications and systems. This can be particularly useful for automating task creation based on specific triggers or events in your workflow.
Setting Up Your Wrike Test/Sandbox Account for API Integration
Before you can start creating tasks with the Wrike API using JavaScript, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Wrike Account
If you don't already have a Wrike account, you can sign up for a free trial on the Wrike website. Follow the instructions to create your account. If you already have an account, simply log in.
Setting Up a Wrike Sandbox Environment
Wrike offers a sandbox environment for developers to test their integrations. To access the sandbox, you may need to contact Wrike support or your account manager to enable this feature.
Creating a Wrike App for OAuth 2.0 Authentication
Wrike uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials.
- Navigate to the Wrike Developer Portal at developers.wrike.com.
- Log in with your Wrike account credentials.
- Go to the "Apps" section and click on "Create New App."
- Fill in the required details such as the app name and description.
- Set the redirect URI, which is the URL where users will be redirected after they authorize your app.
- Once your app is created, note down the Client ID and Client Secret. You'll need these for OAuth 2.0 authentication.
Obtaining OAuth 2.0 Access Token
With your Client ID and Client Secret, you can now obtain an access token to authenticate API requests.
- Direct users to Wrike's authorization URL, including your Client ID and redirect URI.
- After users authorize your app, they'll be redirected to your specified URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Wrike's token endpoint.
For detailed instructions, refer to the Wrike OAuth 2.0 documentation.
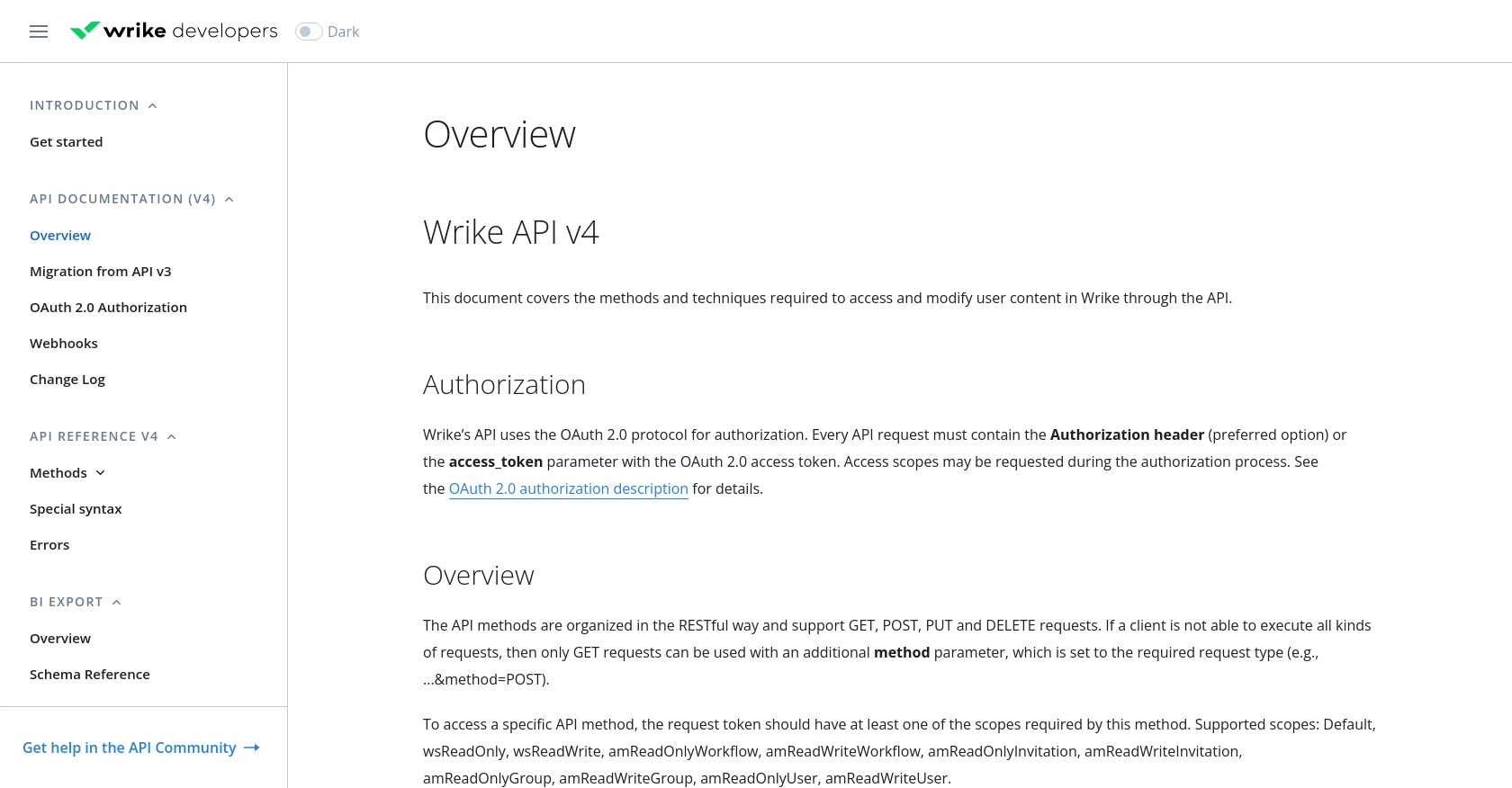
sbb-itb-96038d7
Making API Calls to Create Tasks in Wrike Using JavaScript
To create tasks in Wrike using JavaScript, you'll need to make API calls to the Wrike API. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Wrike API Integration
Before making API calls, ensure you have a suitable JavaScript environment. You'll need Node.js installed on your machine, along with the axios
library for making HTTP requests.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library by runningnpm install axios
.
Writing JavaScript Code to Create a Task in Wrike
Now that your environment is set up, you can write the JavaScript code to create a task in Wrike. The following example demonstrates how to make a POST request to the Wrike API to create a task in a specified folder.
const axios = require('axios');
// Replace with your access token and folder ID
const accessToken = 'YOUR_ACCESS_TOKEN';
const folderId = 'YOUR_FOLDER_ID';
// Define the task details
const taskData = {
title: 'New Task Title',
description: 'Description of the new task',
status: 'Active'
};
// Set the API endpoint and headers
const endpoint = `https://www.wrike.com/api/v4/folders/${folderId}/tasks`;
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Make the POST request to create the task
axios.post(endpoint, taskData, { headers })
.then(response => {
console.log('Task Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Task:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
and YOUR_FOLDER_ID
with your actual access token and folder ID. The taskData
object contains the task details, such as the title and description.
Verifying Task Creation in Wrike
After running the code, you can verify that the task was created successfully by checking your Wrike account. Navigate to the specified folder and confirm that the new task appears with the correct details.
Handling Errors and Wrike API Error Codes
When making API calls, it's important to handle potential errors. The Wrike API provides specific error codes that can help you diagnose issues. For more information on error codes, refer to the Wrike API error documentation.
Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
Ensure your access token is valid and that you have the necessary permissions to create tasks in the specified folder.
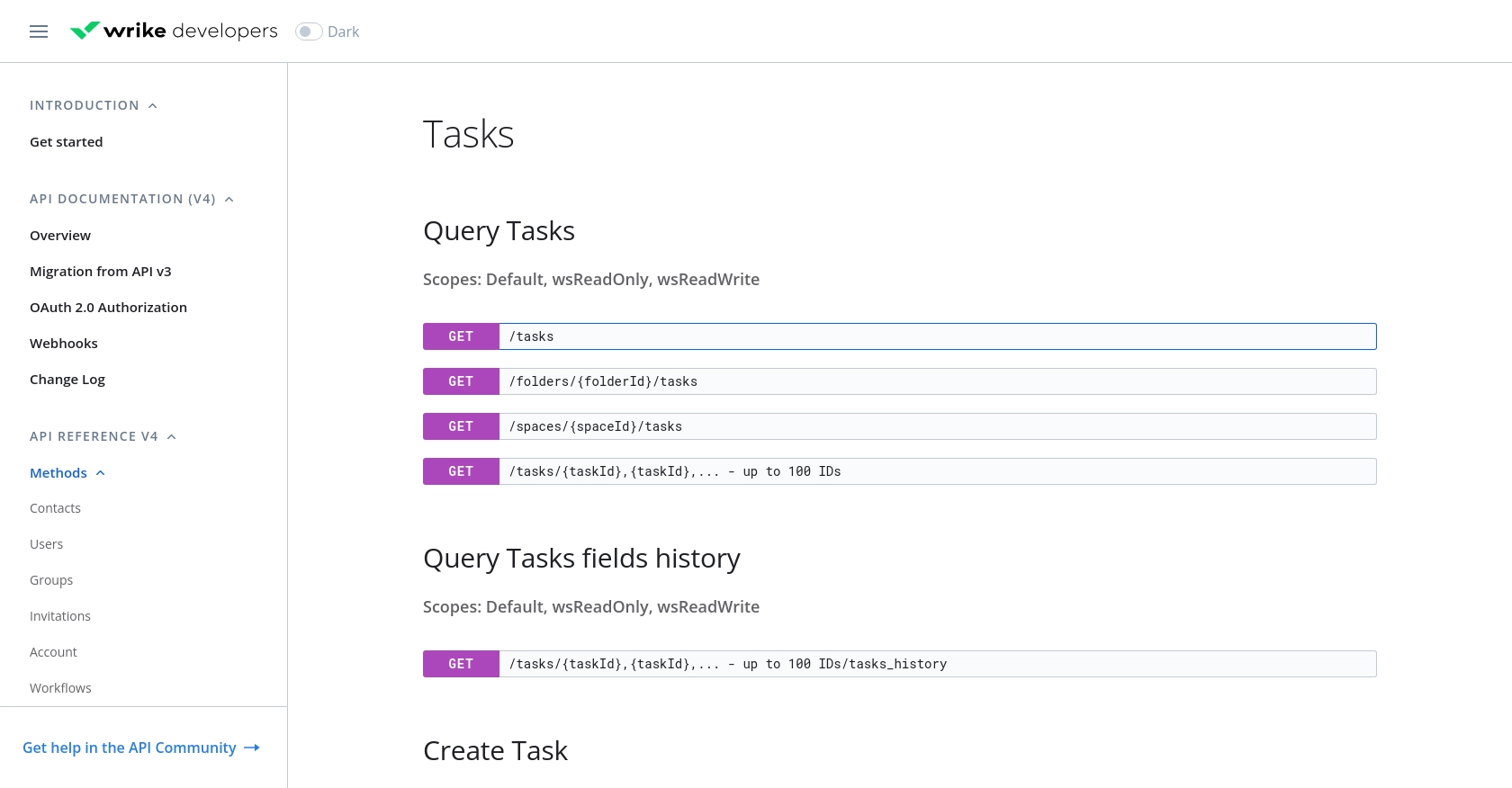
Conclusion and Best Practices for Wrike API Integration
Integrating with the Wrike API using JavaScript allows developers to automate task management and enhance productivity by seamlessly connecting Wrike with other systems. By following the steps outlined in this guide, you can efficiently create tasks in Wrike and ensure smooth integration.
Best Practices for Secure and Efficient Wrike API Usage
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Wrike's API rate limits to prevent your application from being throttled. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your applications to maintain consistency and avoid data discrepancies when integrating with Wrike.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes, Endgrate offers a unified API solution that simplifies connecting with multiple platforms, including Wrike. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover how to build once for each use case instead of multiple times for different integrations.
Read More
Ready to get started?