Using the Re:amaze API to Get Conversations in Javascript
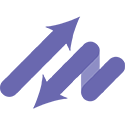
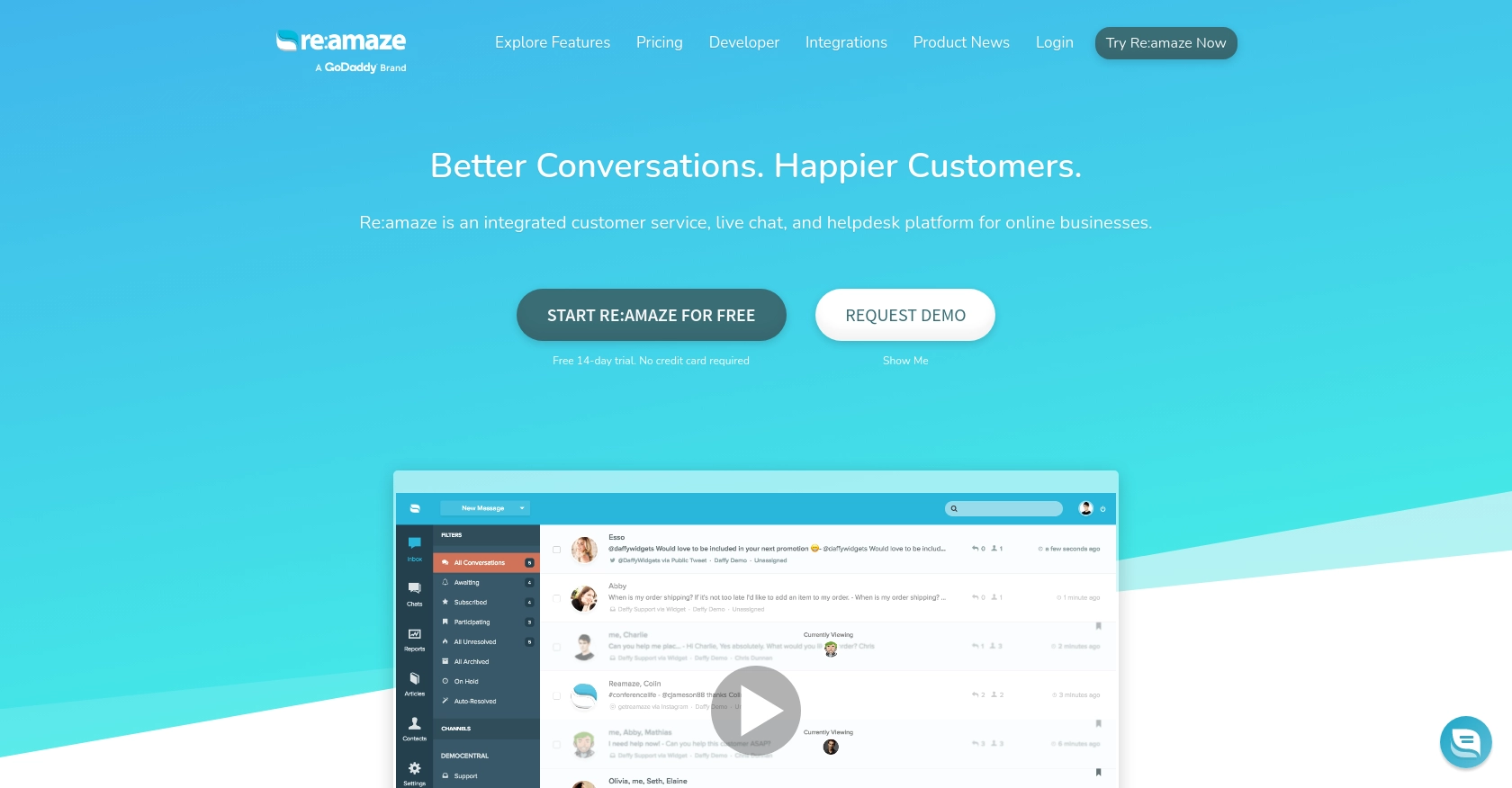
Introduction to Re:amaze API Integration
Re:amaze is a versatile customer support platform that offers a unified inbox for managing communications across various channels. It provides tools for live chat, automated messaging, and customer engagement, making it an ideal choice for businesses looking to enhance their customer service experience.
Integrating with the Re:amaze API allows developers to programmatically access and manage conversations, enabling seamless automation of customer interactions. For example, a developer might want to retrieve conversations to analyze customer queries and improve response strategies.
Setting Up Your Re:amaze Test Account for API Access
Before you can start interacting with the Re:amaze API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Free Re:amaze Account
To begin, sign up for a free Re:amaze account:
- Visit the Re:amaze website and click on "Try Re:amaze Now."
- Follow the prompts to create your account using your email, Google, or Microsoft credentials.
- Once your account is created, log in to access the Re:amaze dashboard.
Generating Your Re:amaze API Token
With your account set up, you'll need to generate an API token to authenticate your API requests:
- Navigate to the "Settings" section within your Re:amaze account.
- Under the "Developer" menu, click on "API Token."
- Select "Generate New Token" to create a unique API token for your account.
- Make sure to copy and securely store this token, as it will be required for API authentication.
For more details, refer to the Re:amaze API documentation.
Understanding Re:amaze API Authentication
The Re:amaze API uses HTTP Basic Auth for authentication. Ensure that all requests are made over HTTPS to maintain security:
- Include your login email and API token in the request header.
- Set the "Accept" header to "application/json" to specify the response format.
Here's an example of how to set up a basic request using curl:
curl 'https://{brand}.reamaze.io/api/v1/conversations' \
-u {login-email}:{api-token} \
-H 'Accept: application/json'
Replace {brand}
, {login-email}
, and {api-token}
with your specific details.
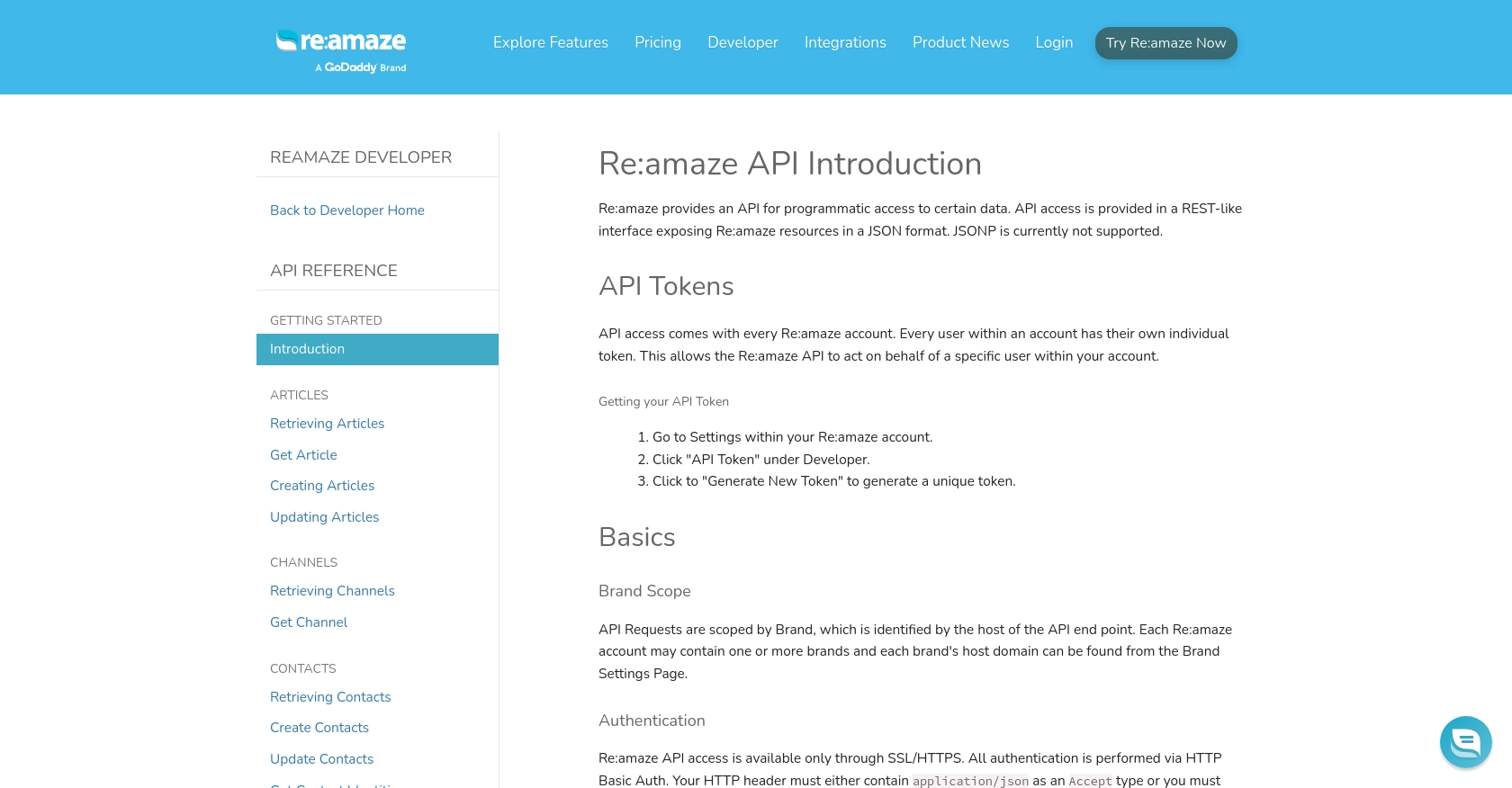
sbb-itb-96038d7
Making API Calls to Retrieve Conversations from Re:amaze Using JavaScript
To interact with the Re:amaze API and retrieve conversations, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment and writing the necessary code.
Setting Up Your JavaScript Environment for Re:amaze API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js for server-side scripting or a browser console for client-side scripting. For this tutorial, we'll use Node.js.
- Ensure you have Node.js installed. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library to simplify HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Conversations from Re:amaze
Now that your environment is set up, you can write the JavaScript code to fetch conversations from Re:amaze.
const axios = require('axios');
// Replace with your Re:amaze brand, login email, and API token
const brand = 'your-brand';
const loginEmail = 'your-email@example.com';
const apiToken = 'your-api-token';
// Set the API endpoint
const endpoint = `https://${brand}.reamaze.io/api/v1/conversations`;
// Configure the request headers
const headers = {
'Accept': 'application/json',
'Authorization': `Basic ${Buffer.from(`${loginEmail}:${apiToken}`).toString('base64')}`
};
// Function to fetch conversations
async function fetchConversations() {
try {
const response = await axios.get(endpoint, { headers });
const conversations = response.data.conversations;
console.log('Conversations:', conversations);
} catch (error) {
console.error('Error fetching conversations:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchConversations();
In this code, we use the axios
library to make a GET request to the Re:amaze API. We configure the request with the necessary headers, including the Authorization
header for HTTP Basic Auth. The fetchConversations
function retrieves and logs the conversations.
Verifying Successful API Requests in Re:amaze
After running the code, you should see the list of conversations printed in your terminal. To verify the request's success, you can log in to your Re:amaze dashboard and check the conversations section to ensure they match the retrieved data.
Handling Errors and Understanding Re:amaze API Error Codes
When making API calls, it's crucial to handle potential errors. The Re:amaze API may return various HTTP status codes to indicate issues:
- 401 Unauthorized: Check your API token and login credentials.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before retrying.
- 500 Internal Server Error: An issue occurred on the server. Try again later.
For more details on error handling, refer to the Re:amaze API documentation.
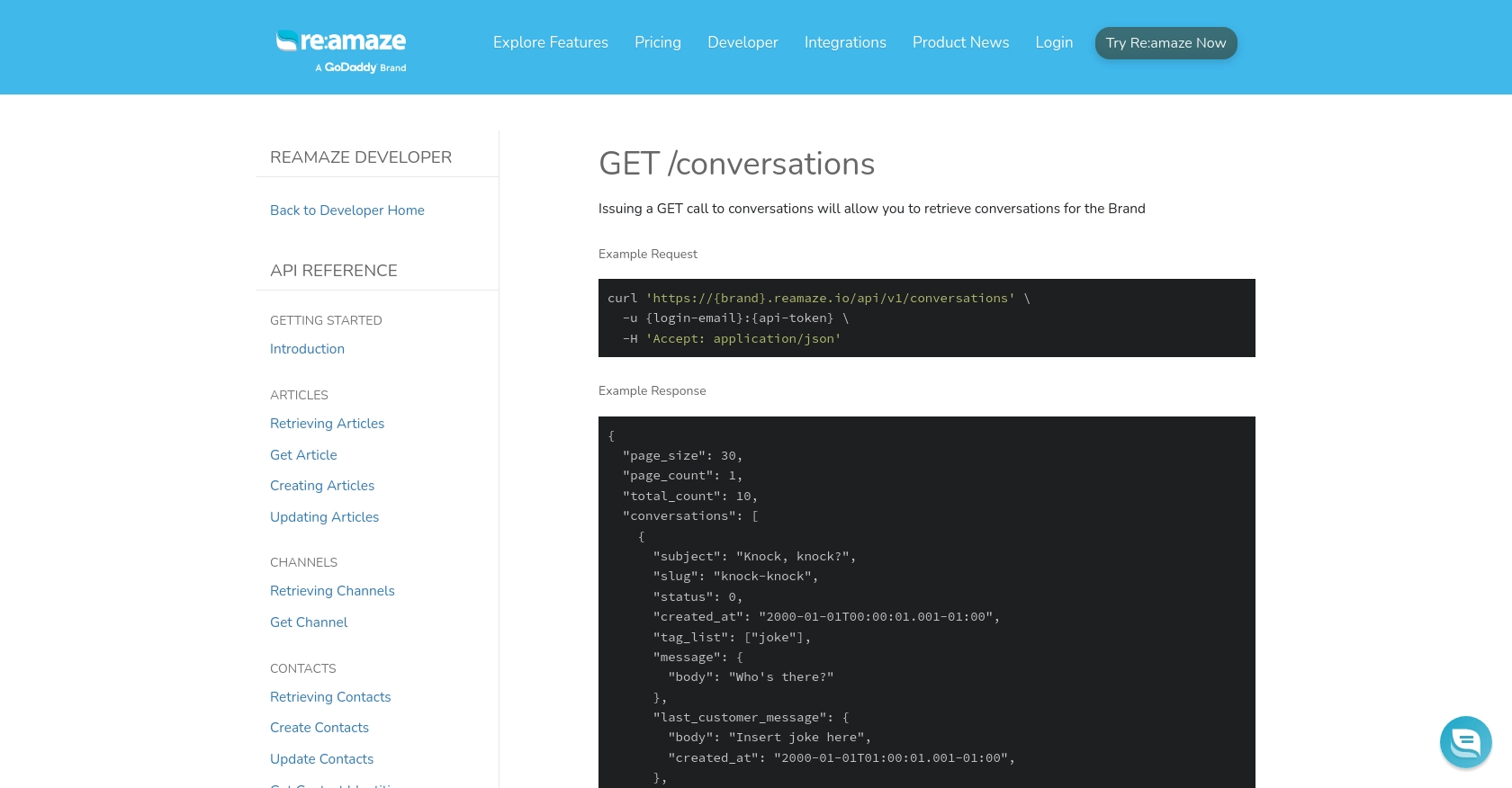
Conclusion and Best Practices for Re:amaze API Integration in JavaScript
Integrating with the Re:amaze API using JavaScript provides developers with powerful tools to automate and enhance customer support interactions. By following the steps outlined in this guide, you can efficiently retrieve and manage conversations, helping your team respond to customer queries more effectively.
Best Practices for Secure and Efficient Re:amaze API Usage
- Secure Storage of API Credentials: Always store your API tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handling Rate Limits: Be mindful of the Re:amaze API rate limits. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to fit your application's requirements, improving data consistency.
Enhancing Integration Capabilities with Endgrate
While integrating with Re:amaze directly can be effective, using a tool like Endgrate can streamline the process further. Endgrate allows you to manage multiple integrations through a single API endpoint, saving time and resources. By outsourcing integrations to Endgrate, you can focus on your core product development and provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how it can help you build once for each use case instead of multiple times for different integrations.
Read More
Ready to get started?