How to Get Accounts with the Salesflare API in Python
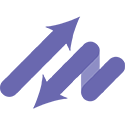
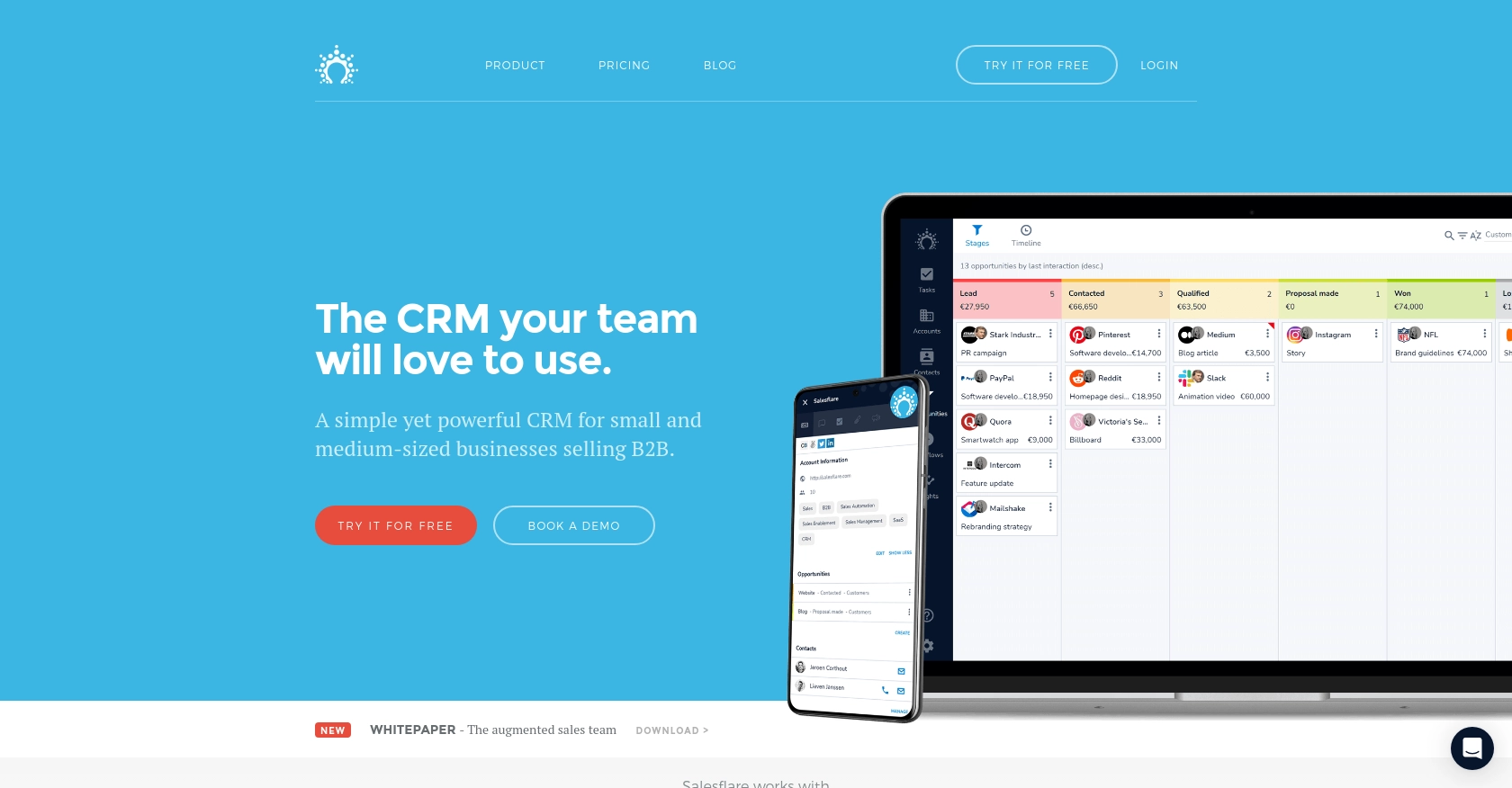
Introduction to Salesflare
Salesflare is a powerful CRM platform designed to simplify and enhance the sales process for small and medium-sized businesses. With its intuitive interface and automation capabilities, Salesflare helps sales teams manage leads, track customer interactions, and streamline their workflows.
Integrating with Salesflare's API allows developers to access and manage account data programmatically, enabling seamless integration with other business tools. For example, a developer might use the Salesflare API to retrieve account information and synchronize it with an internal analytics system, providing valuable insights into customer interactions and sales performance.
Setting Up Your Salesflare Test Account
Before you can start interacting with the Salesflare API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on the Salesflare website. This trial will give you access to all the features you need to test the API.
- Visit the Salesflare website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Salesflare dashboard.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Salesflare account and navigate to the settings page.
- Look for the "API Keys" section and click on it.
- Click on "Generate New API Key" and provide a name for your key to help you identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your Salesflare account and API key ready, you're all set to start making API calls to retrieve account data using Python.
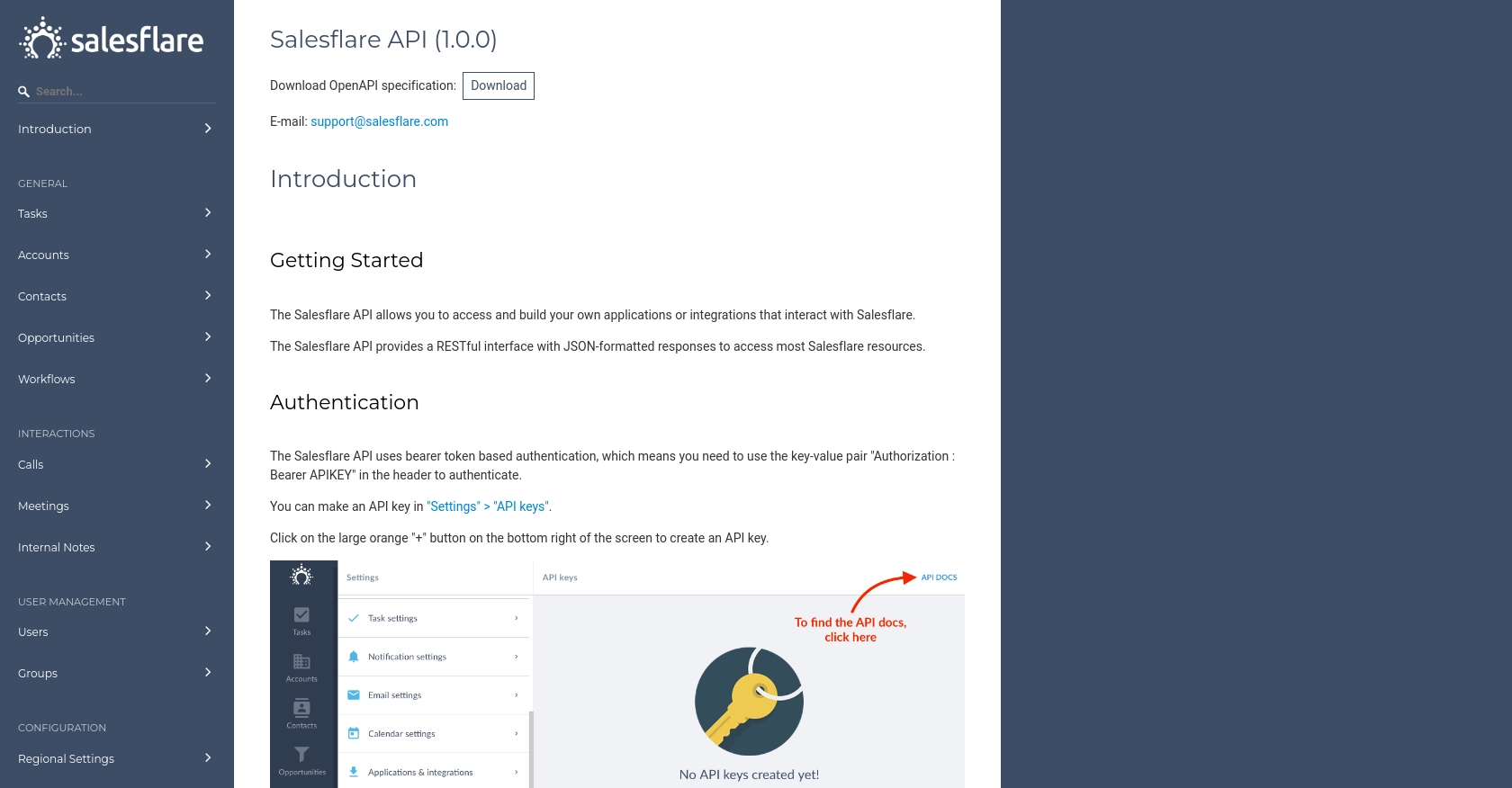
sbb-itb-96038d7
Making API Calls to Retrieve Accounts with Salesflare API in Python
To interact with the Salesflare API using Python, you'll need to ensure you have the right environment set up. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and executing the API call to retrieve account data.
Setting Up Your Python Environment for Salesflare API Integration
Before making any API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1, so make sure you have this version or later. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Accounts from Salesflare
With your environment ready, you can now write the Python code to make an API call to Salesflare and retrieve account data. Create a new Python file named get_salesflare_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.salesflare.com/accounts"
headers = {
"Authorization": "Bearer Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
accounts = response.json()
# Loop through the accounts and print their information
for account in accounts:
print(account)
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated earlier. This script sets up the API endpoint and headers, makes a GET request to the Salesflare API, and processes the response to display account information.
Running Your Python Script and Verifying the Output
To execute your script, run the following command in your terminal or command prompt:
python get_salesflare_accounts.py
If successful, you should see a list of accounts printed in your terminal. This confirms that your API call was successful and that you have retrieved account data from Salesflare.
Handling Errors and Troubleshooting
It's important to handle potential errors when making API calls. The script above checks the HTTP status code to determine if the request was successful. If the status code is not 200, it prints an error message with the status code and response text.
For more detailed error handling, refer to the Salesflare API documentation to understand specific error codes and their meanings.
Conclusion and Best Practices for Using Salesflare API in Python
Integrating with the Salesflare API using Python provides a powerful way to manage and synchronize account data, enhancing your business processes and analytics capabilities. By following the steps outlined in this guide, you can efficiently retrieve account information and integrate it with other tools and systems.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Salesflare API to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data retrieved from Salesflare is transformed and standardized to match the format required by your internal systems for seamless integration.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Salesflare can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Salesflare.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API call, you can interact with multiple services, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration solution.
Read More
Ready to get started?