Using the Xero API to Get Tax Rates in Python
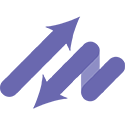
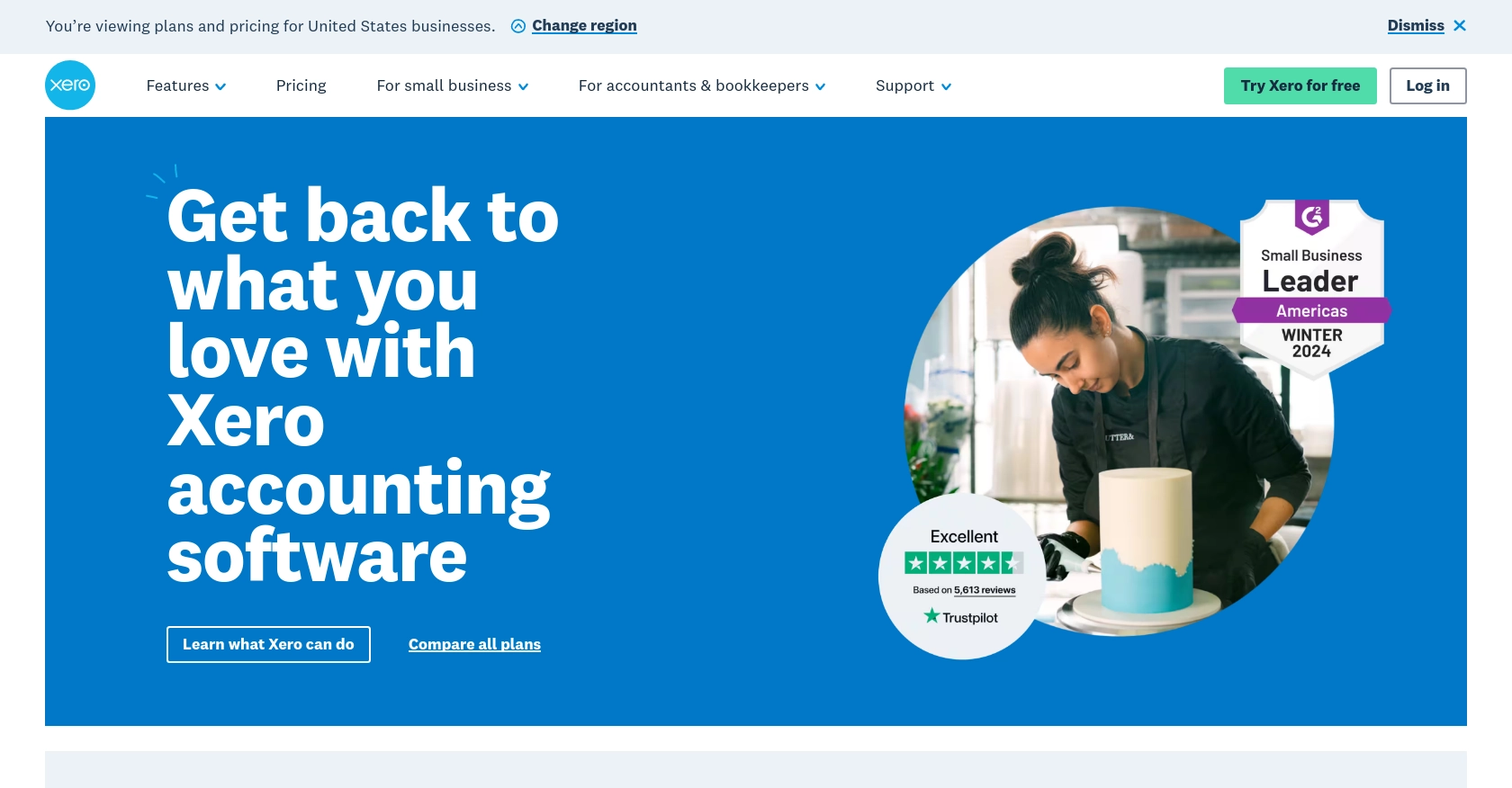
Introduction to Xero API for Tax Rates
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for financial management.
Developers may want to integrate with Xero's API to automate accounting tasks and streamline financial operations. One common use case is retrieving tax rates, which can be essential for calculating taxes on transactions and ensuring compliance with local tax regulations.
In this article, we will explore how to use the Xero API to get tax rates using Python. This integration can help developers automate tax calculations and improve the accuracy of financial reporting.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start using the Xero API to retrieve tax rates, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Follow these steps to create your sandbox account and prepare for API access.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, sign up on the Xero Developer Portal. This account will give you access to the necessary tools and resources for API integration.
Setting Up a Xero Sandbox Organization
Once your developer account is ready, create a sandbox organization:
- Log in to your Xero developer account.
- Navigate to the "My Apps" section and click on "New App."
- Fill in the required details, such as app name and company URL.
- Select "Accounting" as the API you want to use.
- Click "Create App" to generate your sandbox organization.
Configuring OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication. Follow these steps to configure it:
- In your app settings, locate the "OAuth 2.0 Credentials" section.
- Copy the client ID and client secret provided by Xero.
- Set up a redirect URI, which is required for the OAuth flow. This should be a valid URL where users will be redirected after authentication.
For more details on OAuth 2.0 setup, refer to the Xero OAuth 2.0 Overview.
Obtaining Access Tokens
With your OAuth 2.0 credentials, you can now obtain access tokens:
- Use the authorization code flow to request an authorization code from Xero.
- Exchange the authorization code for an access token and a refresh token.
- Store these tokens securely, as they will be used to authenticate API requests.
Refer to the Xero OAuth 2.0 Auth Flow for detailed instructions.
Connecting to Your Xero Sandbox Organization
Finally, connect your app to the sandbox organization:
- Use the access token to make API calls to your sandbox organization.
- Ensure that you have the necessary scopes for accessing tax rates. You can manage scopes in your app settings.
For more information on scopes, visit the Xero OAuth 2.0 Scopes page.
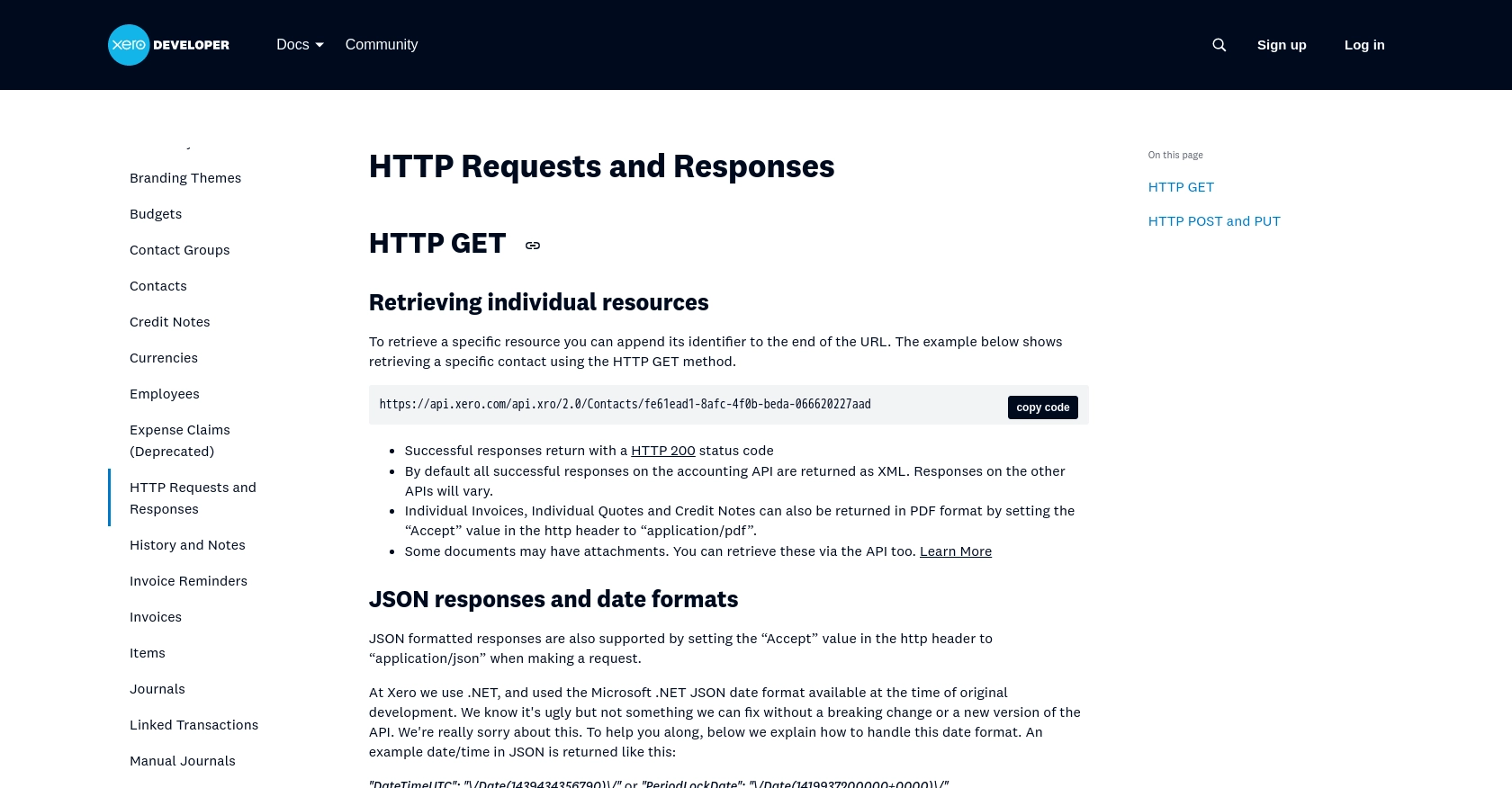
sbb-itb-96038d7
Making API Calls to Retrieve Xero Tax Rates Using Python
Now that you have set up your Xero sandbox account and configured OAuth 2.0 authentication, you can proceed to make API calls to retrieve tax rates. This section will guide you through the process of using Python to interact with the Xero API and obtain tax rate information.
Prerequisites for Using Python with Xero API
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Additionally, you will need the requests
library to make HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Tax Rates from Xero API
Create a new Python file named get_xero_tax_rates.py
and add the following code:
import requests
# Set the API endpoint for tax rates
endpoint = "https://api.xero.com/api.xro/2.0/TaxRates"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the Xero API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
tax_rates = response.json()
print("Tax Rates:", tax_rates)
else:
print("Failed to retrieve tax rates. Status Code:", response.status_code)
Replace Your_Access_Token
with the access token you obtained during the OAuth 2.0 authentication process.
Running the Python Script and Verifying Results
Execute the script from your terminal or command line using the following command:
python get_xero_tax_rates.py
If successful, the script will output the tax rates retrieved from your Xero sandbox organization. Verify the results by checking the tax rates in your sandbox account.
Handling Errors and Understanding Xero API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Xero API may return various HTTP status codes to indicate the outcome of your request:
- 200 OK: The request was successful, and the tax rates are returned.
- 401 Unauthorized: The access token is invalid or expired. Ensure your token is correct and refresh it if necessary.
- 403 Forbidden: The request is not allowed. Check your app's permissions and scopes.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL.
For more information on handling errors, refer to the Xero API Requests and Responses documentation.
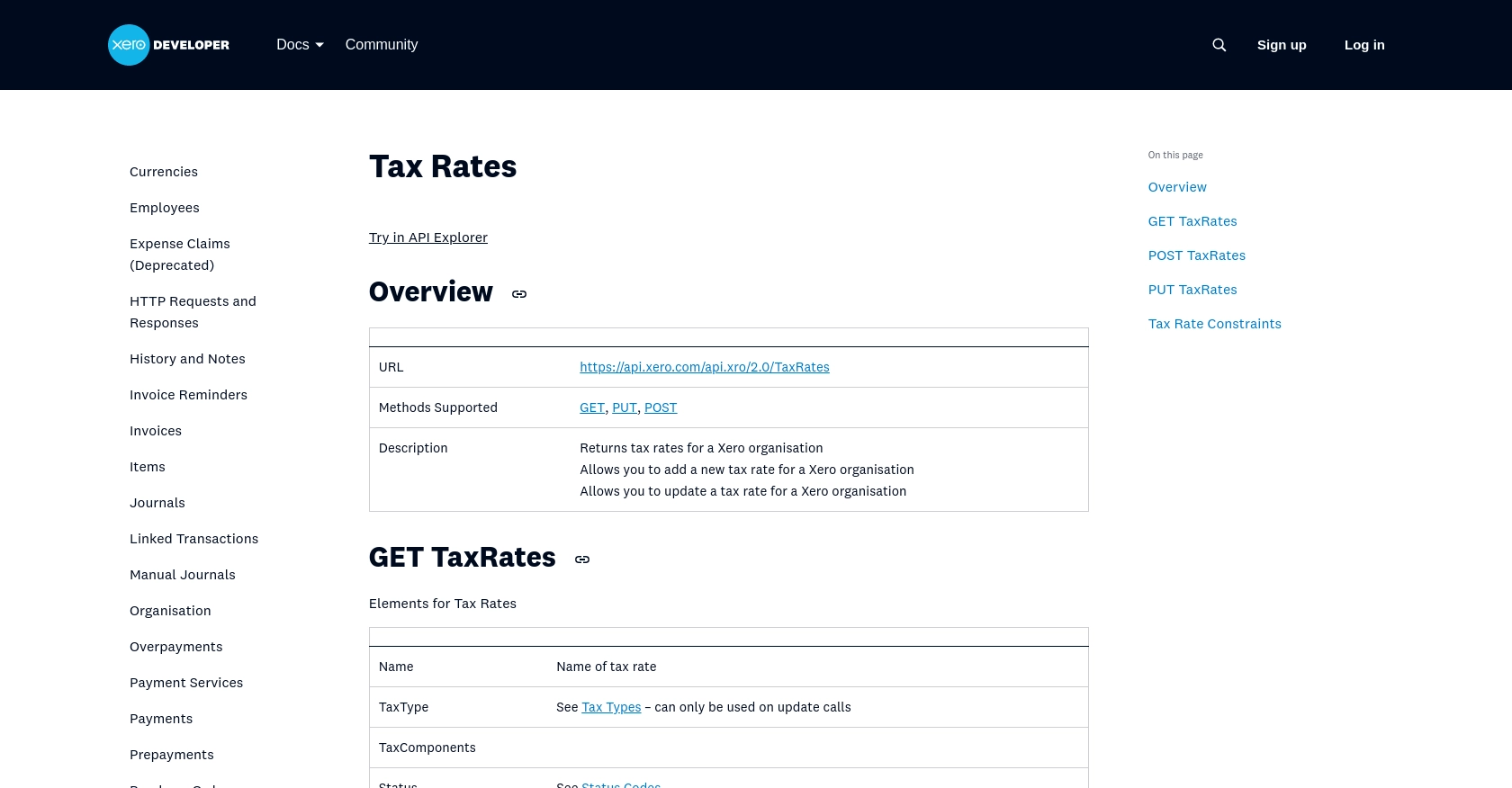
Conclusion and Best Practices for Using Xero API in Python
Integrating with the Xero API to retrieve tax rates using Python can significantly enhance your financial management processes by automating tax calculations and ensuring compliance with local regulations. By following the steps outlined in this article, you can efficiently set up your Xero sandbox account, configure OAuth 2.0 authentication, and make API calls to access tax rate data.
Best Practices for Secure and Efficient Xero API Integration
- Secure Storage of Credentials: Always store your access tokens and client secrets securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handling Rate Limits: Be mindful of Xero's API rate limits to avoid throttling. According to the Xero OAuth 2.0 API limits, ensure your application handles rate limiting gracefully by implementing retry logic with exponential backoff.
- Data Standardization: Standardize and transform data fields as needed to ensure consistency across different systems and applications.
- Error Handling: Implement robust error handling to manage different HTTP status codes and ensure your application can recover from errors smoothly.
Streamlining Integrations with Endgrate
If managing multiple integrations and APIs becomes overwhelming, consider leveraging Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Xero, allowing you to focus on your core product while outsourcing integration complexities. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by streamlining your integration efforts.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/taxrates
Ready to get started?