Using the CSV API to Export Data as CSV (with Python examples)
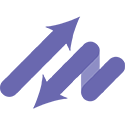
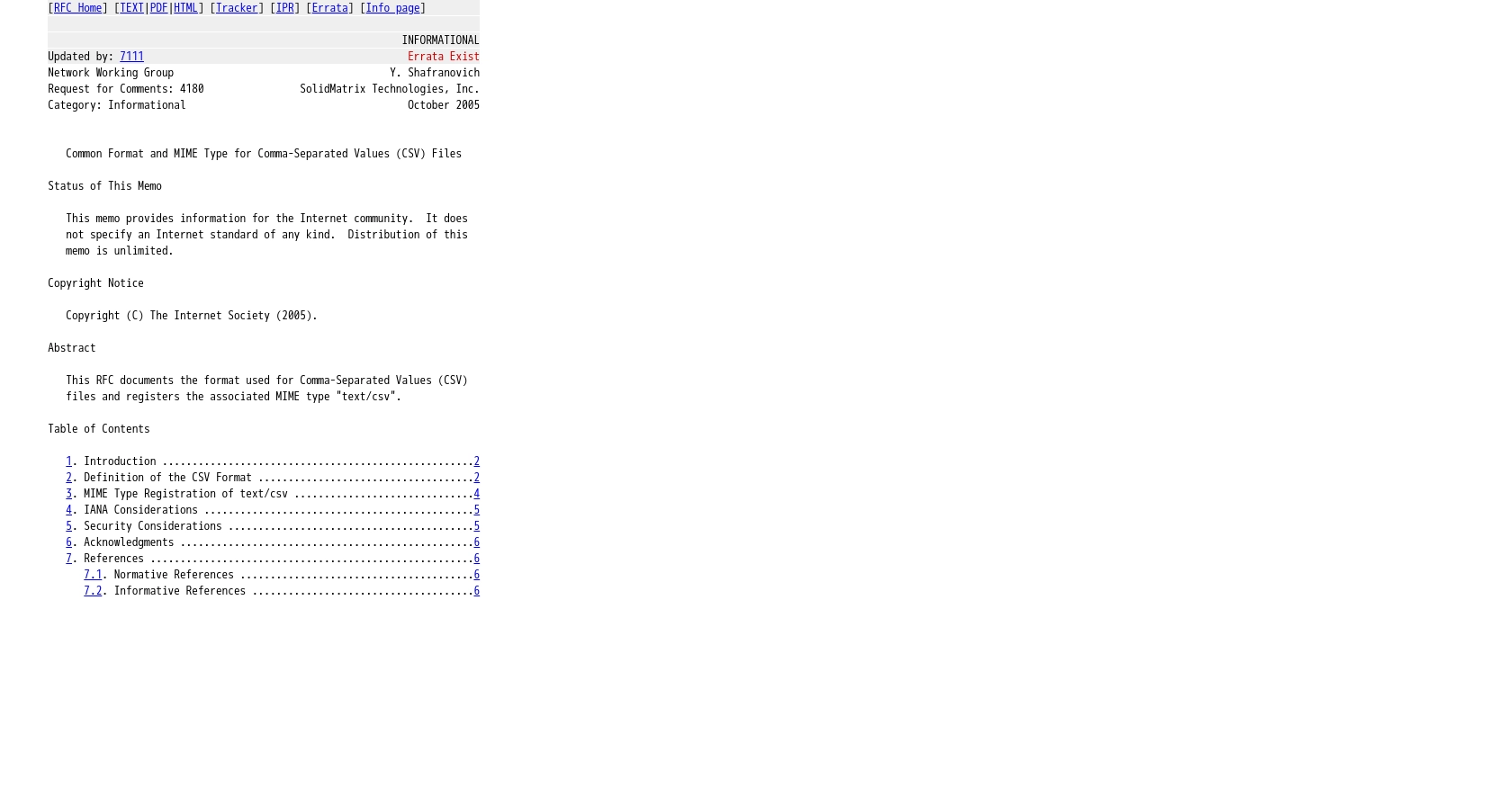
Introduction to CSV Integration
CSV (Comma-Separated Values) is a widely used format for data exchange, allowing for easy import and export of data across various platforms. It is particularly popular in business environments for handling large datasets due to its simplicity and compatibility with numerous applications.
Developers may want to integrate with CSV APIs to automate data export processes, enabling seamless data transfer between systems. For example, a developer could use a CSV API to export customer data from a SaaS application into a CSV file, which can then be used for reporting or data analysis.
This article will guide you through using Python to interact with a CSV API, demonstrating how to export data as a CSV file efficiently. By following the steps outlined, developers can streamline their data handling processes and enhance productivity.
Setting Up Your CSV API Test Environment
Before you begin exporting data using the CSV API, it's essential to set up a test environment. This allows you to safely experiment with API calls without affecting live data. Follow these steps to configure your CSV API test environment effectively.
Create a CSV API Sandbox Account
To start, you'll need to create a sandbox account. This account provides a controlled environment where you can test CSV data exports. Visit the CSV API provider's website and sign up for a free trial or demo account. This will give you access to the necessary tools and resources for testing.
Generate API Credentials for CSV Integration
Once your sandbox account is set up, you'll need to generate API credentials. These credentials are crucial for authenticating your API requests. Follow these steps to obtain your API key:
- Log in to your sandbox account.
- Navigate to the API settings or developer section.
- Locate the option to generate a new API key.
- Copy the API key and store it securely, as you'll need it for authentication in your Python scripts.
Understanding CSV API Authentication
The CSV API uses a custom integration approach, which involves uploading and downloading CSV files in chunks. Ensure you understand the authentication process by reviewing the API documentation provided by the CSV integration provider. This will help you correctly implement the authentication in your code.
Prepare Your Development Environment
Before making API calls, ensure your development environment is ready. You'll need Python installed on your machine, along with the necessary libraries for handling CSV files. Use the following command to install the required library:
pip install pandas
With your environment set up, you're ready to start making API calls to export data as CSV files using Python.
Making API Calls to Export Data as CSV Using Python
To effectively interact with the CSV API and export data, you'll need to set up your Python environment and write code that handles the API requests. This section will guide you through the process of making API calls to export data as CSV files using Python.
Setting Up Your Python Environment for CSV API Integration
Ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the pandas
library to handle CSV files. Install it using the following command:
pip install pandas
Writing Python Code to Interact with the CSV API
Once your environment is ready, you can write Python code to interact with the CSV API. The following example demonstrates how to export data as a CSV file:
import requests
import pandas as pd
# Define the API endpoint and headers
api_endpoint = "https://api.example.com/export"
headers = {
"Authorization": "Bearer Your_API_Key",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(api_endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Load the data into a pandas DataFrame
data = pd.DataFrame(response.json())
# Export the data to a CSV file
data.to_csv("exported_data.csv", index=False)
print("Data exported successfully as exported_data.csv")
else:
print(f"Failed to export data. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you generated earlier. This script makes a GET request to the CSV API, retrieves the data, and exports it to a CSV file named exported_data.csv
.
Verifying Successful Data Export in the CSV API Sandbox
After running the script, verify that the data export was successful by checking the exported_data.csv
file in your working directory. The file should contain the data retrieved from the CSV API.
Handling Errors and Common Error Codes in CSV API Integration
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check your request parameters.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 500 Internal Server Error: The server encountered an error. Try again later.
Implement error handling in your code to manage these scenarios effectively.
Conclusion and Best Practices for CSV API Integration with Python
Integrating with a CSV API using Python can significantly enhance your data management capabilities, allowing for seamless data export and analysis. By following the steps outlined in this article, you can efficiently automate data export processes, saving time and reducing manual errors.
Best Practices for Secure and Efficient CSV API Integration
- Securely Store API Credentials: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Implement Rate Limiting: Be mindful of the API provider's rate limits to avoid throttling. Implement retry logic with exponential backoff for handling rate limit errors.
- Data Transformation and Standardization: Use libraries like
pandas
to transform and standardize data fields, ensuring consistency across different datasets. - Error Handling: Implement robust error handling to manage common API errors, such as authentication failures or server issues, ensuring your application remains resilient.
Streamlining Integrations with Endgrate
While integrating with a CSV API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including CSV APIs. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integration efforts.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover a more efficient way to manage your API integrations.
Read More
Ready to get started?