Using the Google Docs API to Get Document Texts (with Javascript examples)
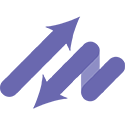
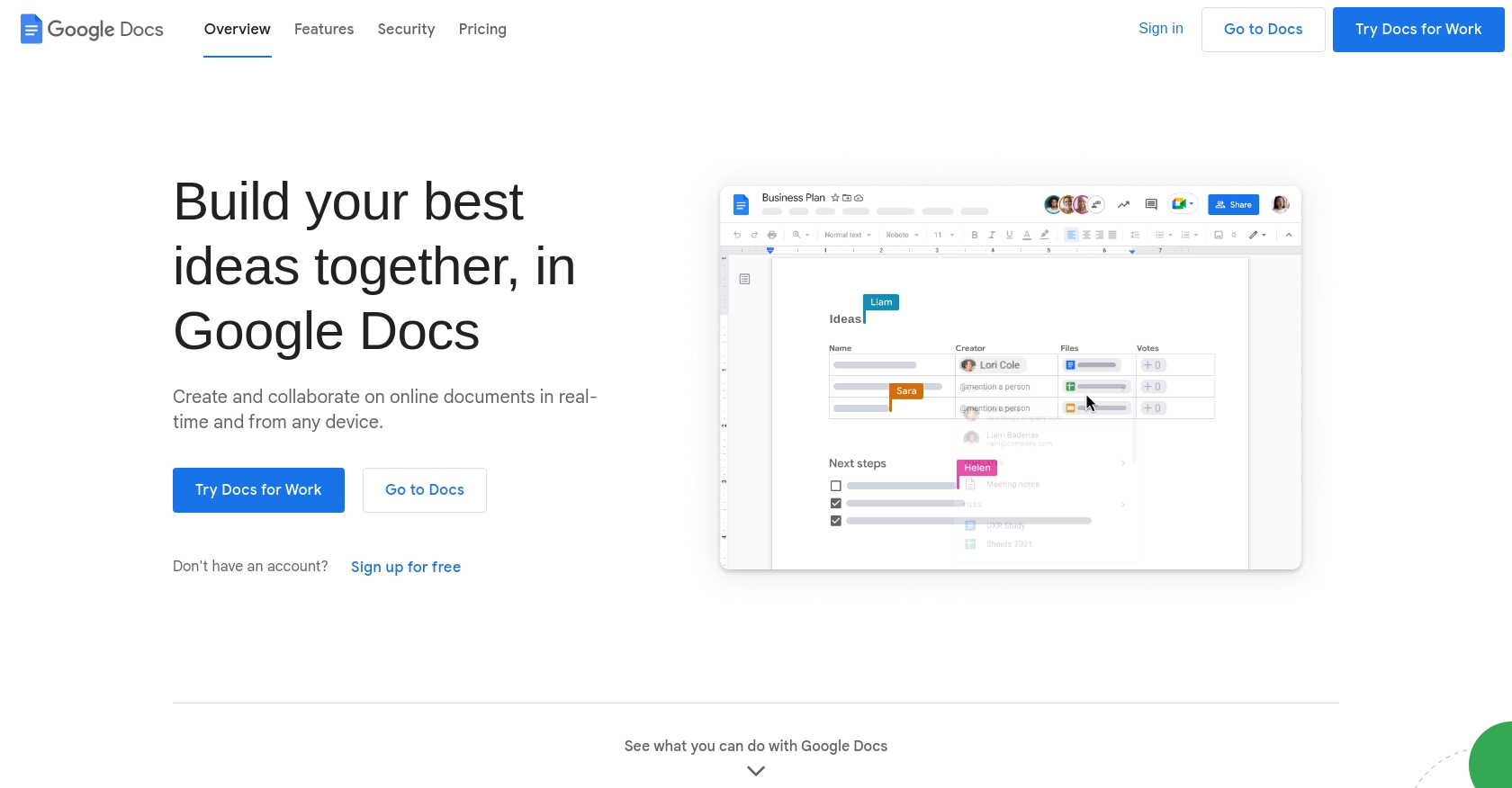
Introduction to Google Docs API
Google Docs is a widely used cloud-based word processing application that allows users to create, edit, and collaborate on documents in real-time. Its seamless integration with other Google Workspace tools makes it an essential part of many businesses' workflows.
For developers, integrating with the Google Docs API opens up a world of possibilities for automating document management tasks. By accessing document content programmatically, developers can create applications that enhance productivity and streamline operations. For example, a developer might use the Google Docs API to extract text from documents for data analysis or to automate the generation of reports.
Setting Up Your Google Docs API Test Environment
Before you can start interacting with the Google Docs API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access and manage documents within your Google Workspace environment.
Create a Google Cloud Project for Google Docs API
- Go to the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Docs API in Your Project
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Docs API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Docs API
- In the Google Cloud Console, navigate to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
Create OAuth 2.0 Credentials for Google Docs API
- Go to Menu > APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for the credential and add your authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the following Google documentation links: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
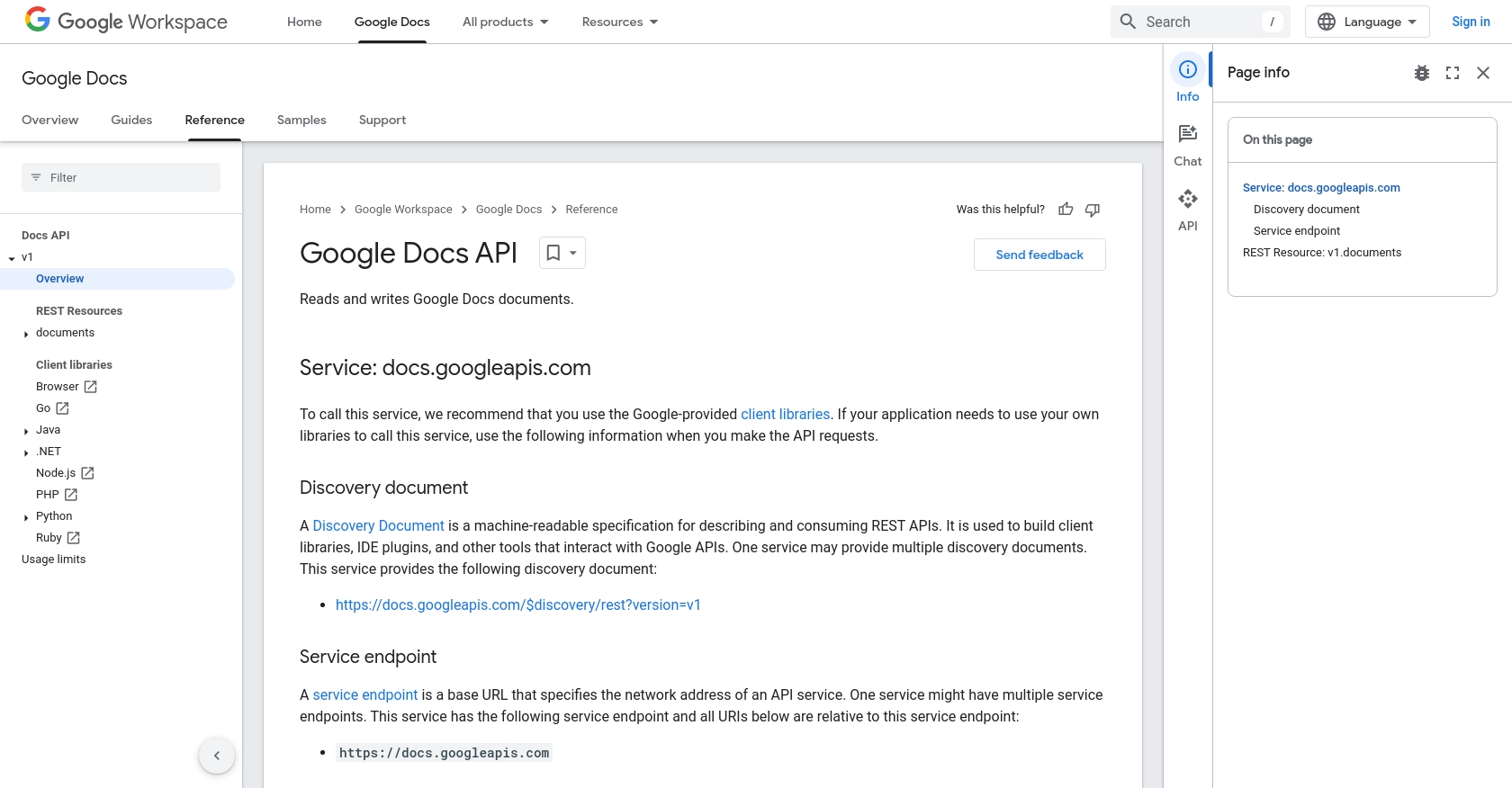
sbb-itb-96038d7
Making API Calls to Retrieve Document Texts Using Google Docs API with JavaScript
To interact with the Google Docs API and retrieve document texts using JavaScript, you'll need to set up your environment and write code that makes HTTP requests to the API. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for Google Docs API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside a browser.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
googleapis
package, which provides access to Google APIs, by runningnpm install googleapis
.
Writing JavaScript Code to Get Document Texts from Google Docs API
Now that your environment is set up, you can write the JavaScript code to retrieve document texts. The following example demonstrates how to use the Google Docs API to get the text content of a document.
const { google } = require('googleapis');
const docs = google.docs('v1');
const { OAuth2 } = google.auth;
// Initialize OAuth2 client
const oauth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set the access token
oauth2Client.setCredentials({
access_token: 'YOUR_ACCESS_TOKEN',
});
// Function to get document text
async function getDocumentText(documentId) {
try {
const response = await docs.documents.get({
documentId: documentId,
auth: oauth2Client,
});
const content = response.data.body.content;
content.forEach(element => {
if (element.paragraph) {
element.paragraph.elements.forEach(textRun => {
if (textRun.textRun) {
console.log(textRun.textRun.content);
}
});
}
});
} catch (error) {
console.error('Error retrieving document:', error);
}
}
// Replace 'YOUR_DOCUMENT_ID' with your actual document ID
getDocumentText('YOUR_DOCUMENT_ID');
In this code:
- We import the
googleapis
library and initialize the Google Docs API client. - We set up OAuth2 authentication using your client ID, client secret, and redirect URI.
- The
getDocumentText
function retrieves the document content and logs the text to the console.
Verifying API Call Success and Handling Errors
After running the code, check the console output to verify that the document texts are retrieved successfully. If the API call fails, the error message will be logged to the console. Common errors include invalid credentials or incorrect document IDs.
For more details on handling errors and understanding error codes, refer to the Google Docs API documentation.
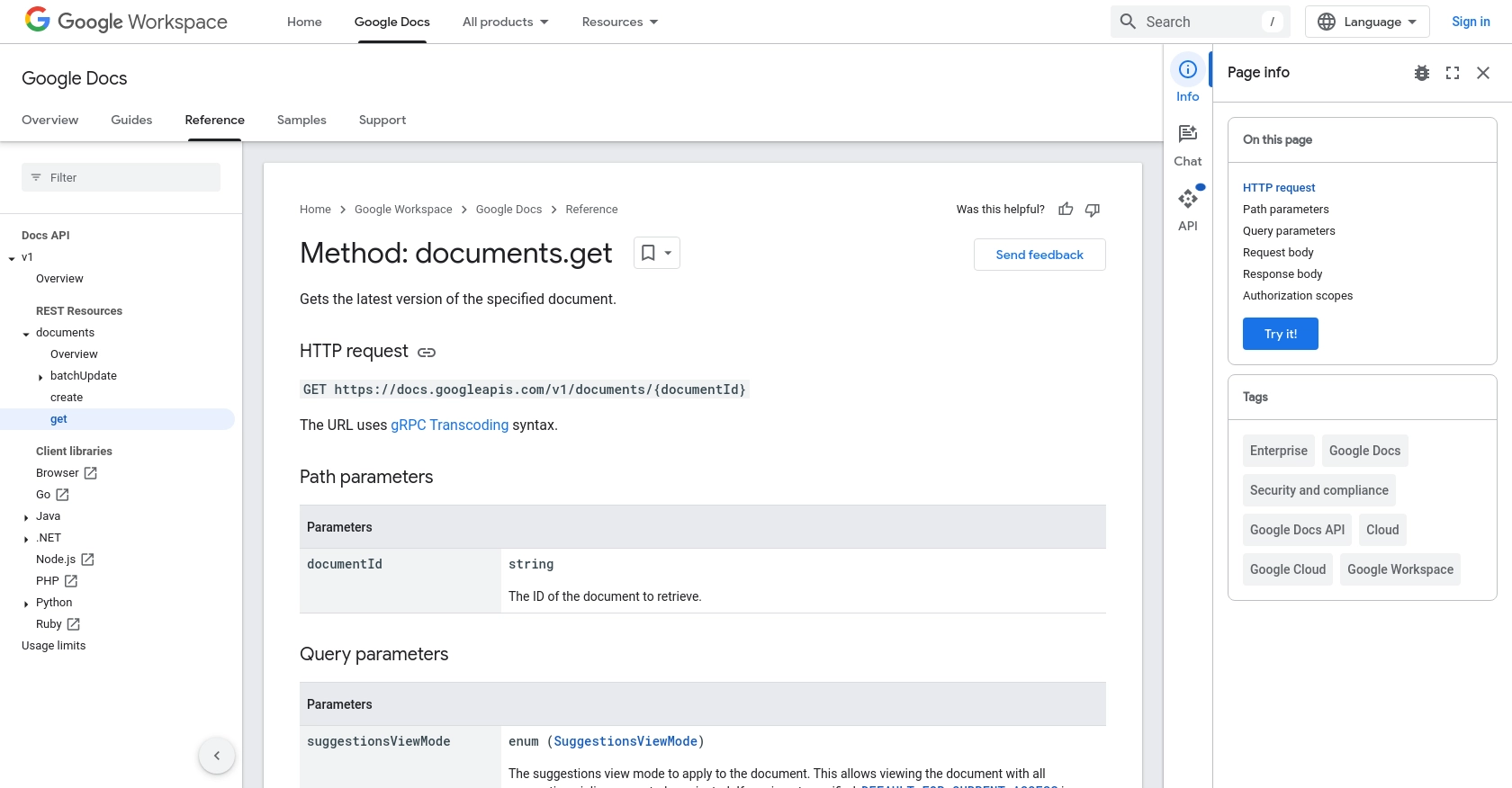
Conclusion and Best Practices for Using Google Docs API with JavaScript
Integrating with the Google Docs API using JavaScript provides developers with powerful tools to automate and enhance document management tasks. By following the steps outlined in this guide, you can efficiently retrieve document texts and incorporate them into your applications.
Best Practices for Secure and Efficient Google Docs API Integration
- Securely Store Credentials: Always keep your client ID, client secret, and access tokens secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's usage limits to avoid exceeding the quota. Implement exponential backoff strategies to handle rate limiting gracefully.
- Optimize Data Processing: When processing large documents, consider fetching only the necessary data to improve performance and reduce API calls.
- Regularly Update Access Tokens: OAuth tokens expire, so ensure your application can refresh tokens automatically to maintain uninterrupted access.
Enhancing Your Integration Experience with Endgrate
For developers looking to streamline their integration processes further, consider using Endgrate. Endgrate simplifies the integration experience by providing a unified API endpoint that connects to multiple platforms, including Google Docs. This allows you to focus on your core product while outsourcing complex integrations.
Visit Endgrate to learn more about how you can save time and resources by leveraging their comprehensive integration solutions.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/reference/rest/v1/documents/get
Ready to get started?