Using the Chargebee API to Get Customers (with Javascript examples)
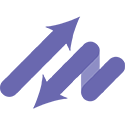
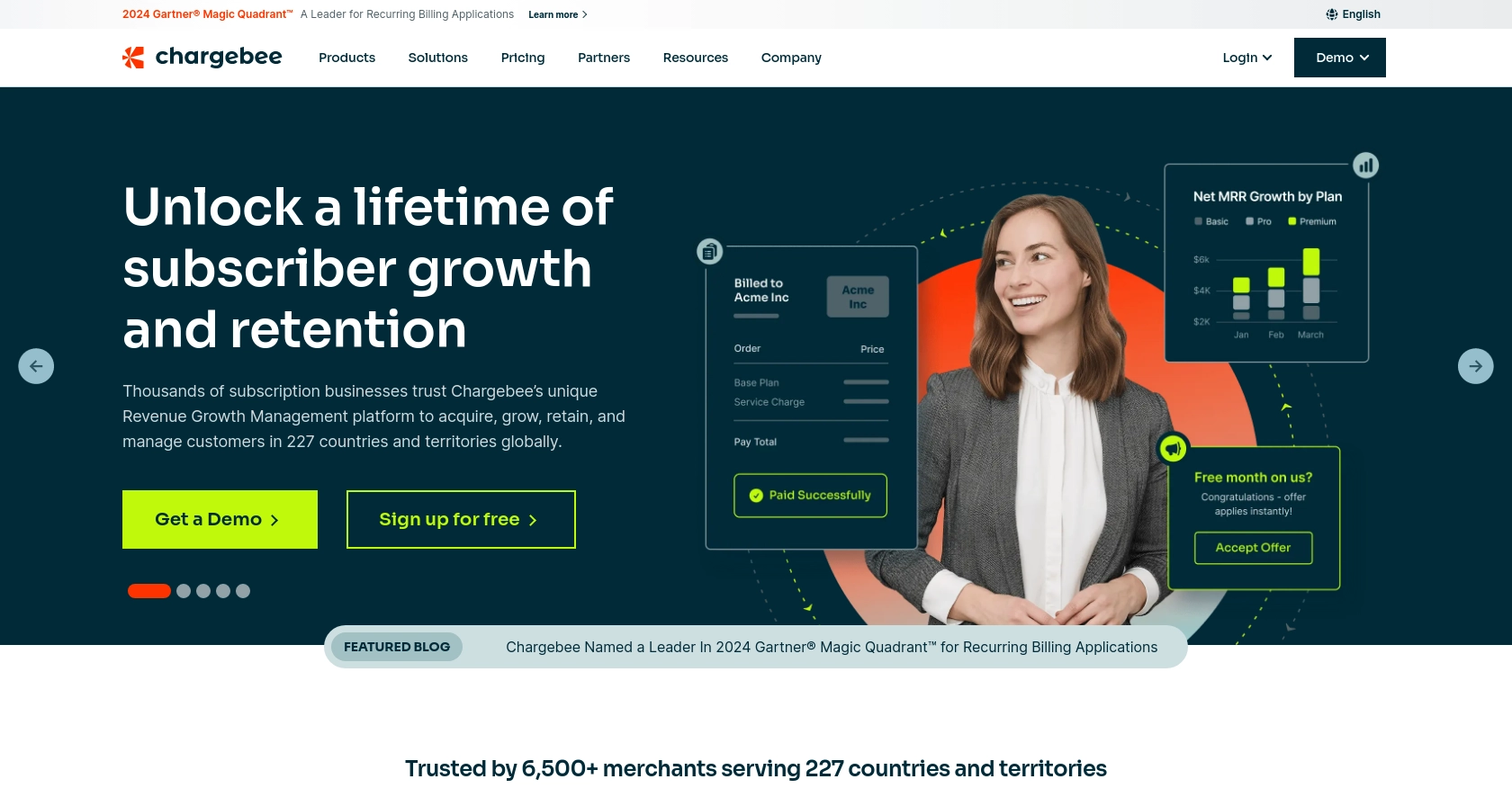
Introduction to Chargebee API Integration
Chargebee is a robust subscription management platform that simplifies billing operations for businesses of all sizes. It offers a comprehensive suite of tools to manage subscriptions, invoicing, and revenue operations, making it a popular choice for SaaS companies.
Developers often seek to integrate with Chargebee's API to streamline customer management processes. For example, you might want to retrieve customer data to analyze subscription trends or automate customer onboarding. By leveraging the Chargebee API, developers can efficiently manage customer data, enhance billing workflows, and improve overall operational efficiency.
Setting Up Your Chargebee Test/Sandbox Account
Before diving into integrating with the Chargebee API, it's essential to set up a test or sandbox account. This allows developers to experiment with API calls without affecting live data, ensuring a safe environment for testing and development.
Creating a Chargebee Test Account
To get started, you'll need to create a Chargebee test account. Follow these steps:
- Visit the Chargebee signup page and register for a free account.
- Once registered, log in to your Chargebee dashboard.
- Navigate to the Sites section and create a new test site. This site will serve as your sandbox environment.
Generating Chargebee API Keys
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key, and the password is left empty. To generate your API keys:
- In your Chargebee dashboard, go to Settings > API Keys.
- Click on Create API Key and provide a name for your key.
- Copy the generated API key and store it securely. You'll use this key to authenticate your API requests.
Configuring API Access and Permissions
Ensure your API key has the necessary permissions to access customer data:
- Under API Keys, click on the key you created.
- Set the required scopes, such as Read and Write permissions for customers.
- Save your changes to update the key permissions.
Testing Chargebee API Calls
With your test account and API key ready, you can start making API calls. Here's a basic example using JavaScript to list customers:
// Example: Fetching customers using Chargebee API
const axios = require('axios');
const site = 'your-test-site';
const apiKey = 'your_api_key';
axios.get(`https://${site}.chargebee.com/api/v2/customers`, {
auth: {
username: apiKey,
password: ''
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching customers:', error);
});
Replace your-test-site
and your_api_key
with your actual Chargebee test site name and API key.
By following these steps, you can effectively set up a Chargebee test account and begin integrating with the API to manage customer data efficiently.
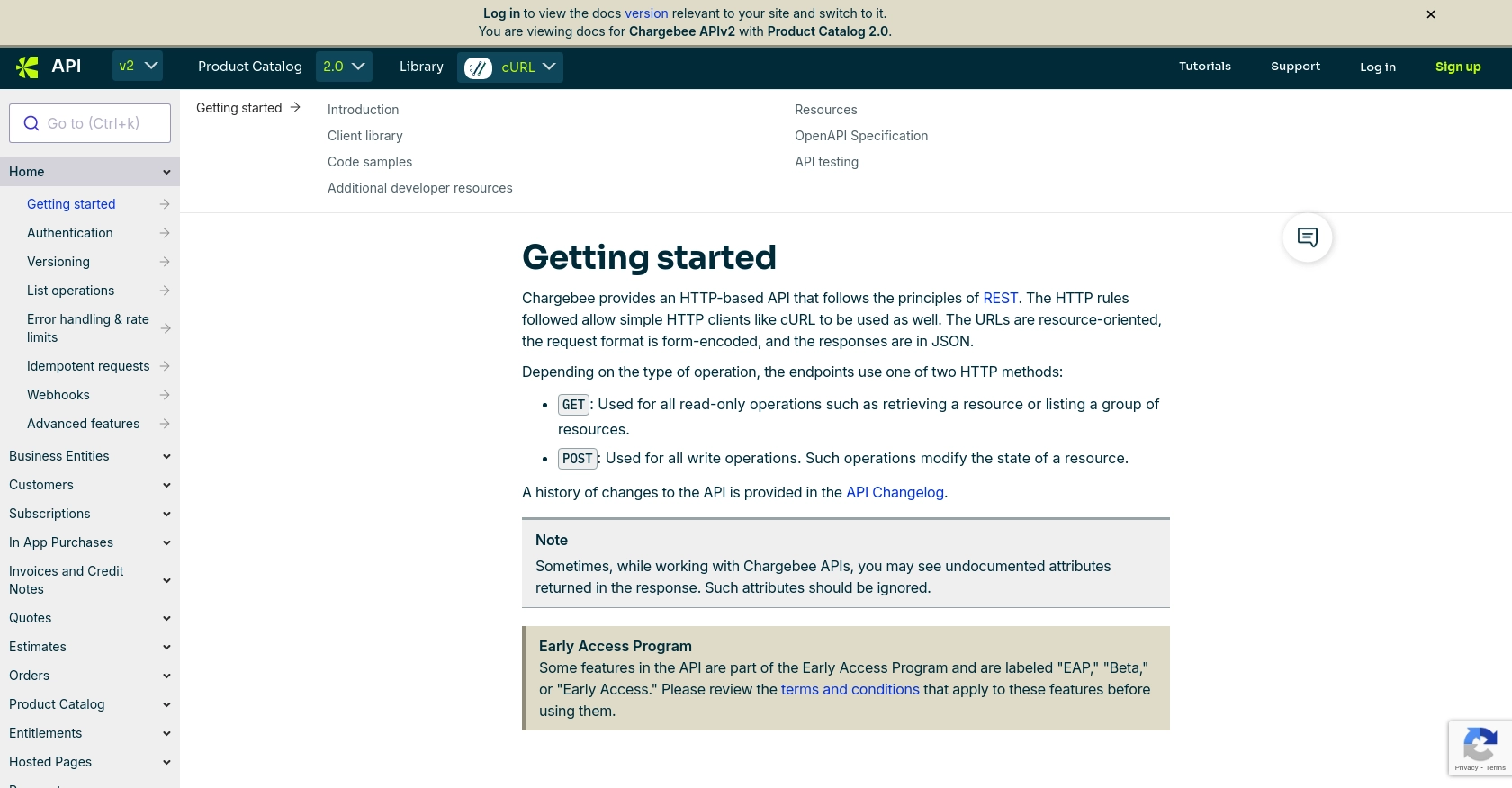
sbb-itb-96038d7
Making API Calls to Retrieve Customers Using Chargebee API with JavaScript
To interact with the Chargebee API and retrieve customer data, you'll need to set up your development environment with the necessary tools and libraries. This section will guide you through the process of making API calls using JavaScript, ensuring you can efficiently manage customer data within your application.
Setting Up Your JavaScript Environment for Chargebee API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside of a browser. Additionally, you'll need the Axios library to handle HTTP requests.
- Install Node.js from the official website.
- Open your terminal and run the following command to install Axios:
npm install axios
Writing JavaScript Code to Fetch Customers from Chargebee
With your environment set up, you can now write the JavaScript code to fetch customer data from Chargebee. The following example demonstrates how to make a GET request to the Chargebee API to retrieve a list of customers:
// Import the Axios library
const axios = require('axios');
// Define your Chargebee site and API key
const site = 'your-test-site';
const apiKey = 'your_api_key';
// Make a GET request to the Chargebee API to list customers
axios.get(`https://${site}.chargebee.com/api/v2/customers`, {
auth: {
username: apiKey,
password: ''
}
})
.then(response => {
// Log the response data to the console
console.log('Customer Data:', response.data);
})
.catch(error => {
// Handle any errors that occur during the request
console.error('Error fetching customers:', error);
});
Replace your-test-site
and your_api_key
with your actual Chargebee test site name and API key.
Understanding the Response and Handling Errors
When the API call is successful, the response will contain customer data in JSON format. You can parse and manipulate this data as needed for your application. If an error occurs, such as an invalid API key or network issue, the error handling block will log the error details to the console.
For more information on error codes and handling, refer to the Chargebee API documentation.
Verifying API Call Success in Chargebee Dashboard
After running your code, verify the success of your API call by checking the Chargebee dashboard. Navigate to the Customers section to ensure the data retrieved matches the customers listed in your test site.
Best Practices for Chargebee API Integration
- Securely store your API keys and avoid hardcoding them in your source files.
- Implement error handling to manage API rate limits and other potential issues.
- Regularly update your API keys and permissions to maintain security.
By following these steps and best practices, you can effectively integrate with the Chargebee API to manage customer data using JavaScript.
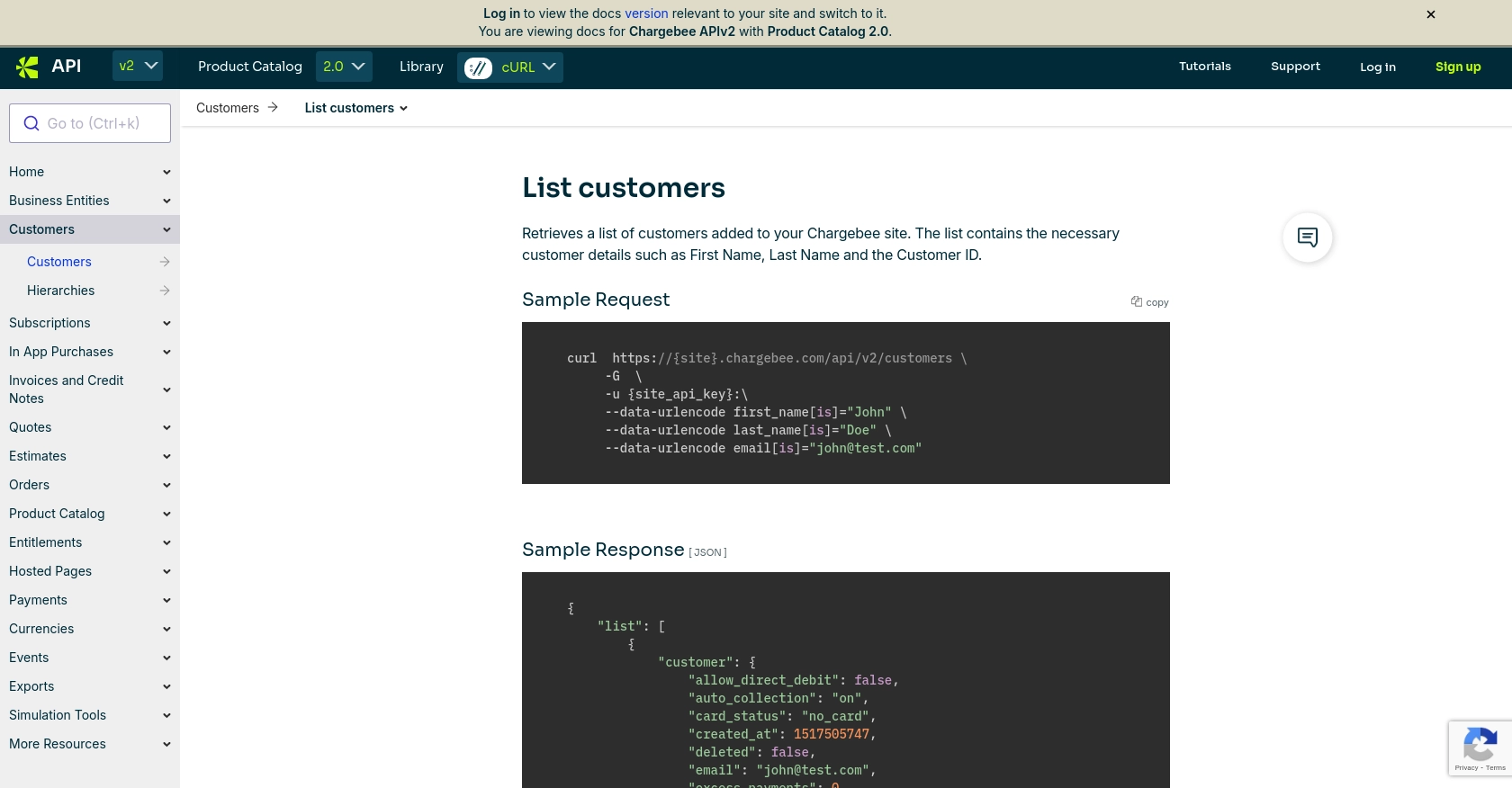
Conclusion: Best Practices for Chargebee API Integration with JavaScript
Integrating with the Chargebee API using JavaScript can significantly enhance your subscription management processes, allowing for seamless customer data retrieval and management. By following the steps outlined in this guide, you can efficiently set up your development environment, authenticate API requests, and handle customer data securely.
Implementing Secure Storage for Chargebee API Keys
One of the critical best practices is to ensure that your Chargebee API keys are stored securely. Avoid hardcoding them in your source files. Instead, use environment variables or secure vaults to manage sensitive information. This approach minimizes the risk of unauthorized access and enhances the security of your application.
Handling Chargebee API Rate Limits and Errors
Chargebee imposes rate limits on API requests to ensure fair usage and system stability. It is essential to implement error handling mechanisms to manage these limits effectively. Consider using exponential backoff strategies for retrying requests and monitoring error codes to address issues promptly. For detailed error handling guidelines, refer to the Chargebee API documentation.
Regularly Updating Chargebee API Keys and Permissions
To maintain the security and functionality of your integration, regularly update your Chargebee API keys and review permissions. This practice ensures that only authorized applications have access to your Chargebee account, reducing the risk of security breaches.
Utilizing Endgrate for Streamlined Chargebee Integrations
For developers seeking to simplify their integration processes, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Chargebee, offering an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration capabilities.
By adhering to these best practices and utilizing tools like Endgrate, you can optimize your Chargebee API integration, ensuring a robust and secure solution for managing customer data and subscription workflows.
Read More
Ready to get started?