Using the Sugar Market API to Get Contacts in Javascript
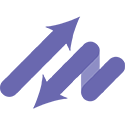
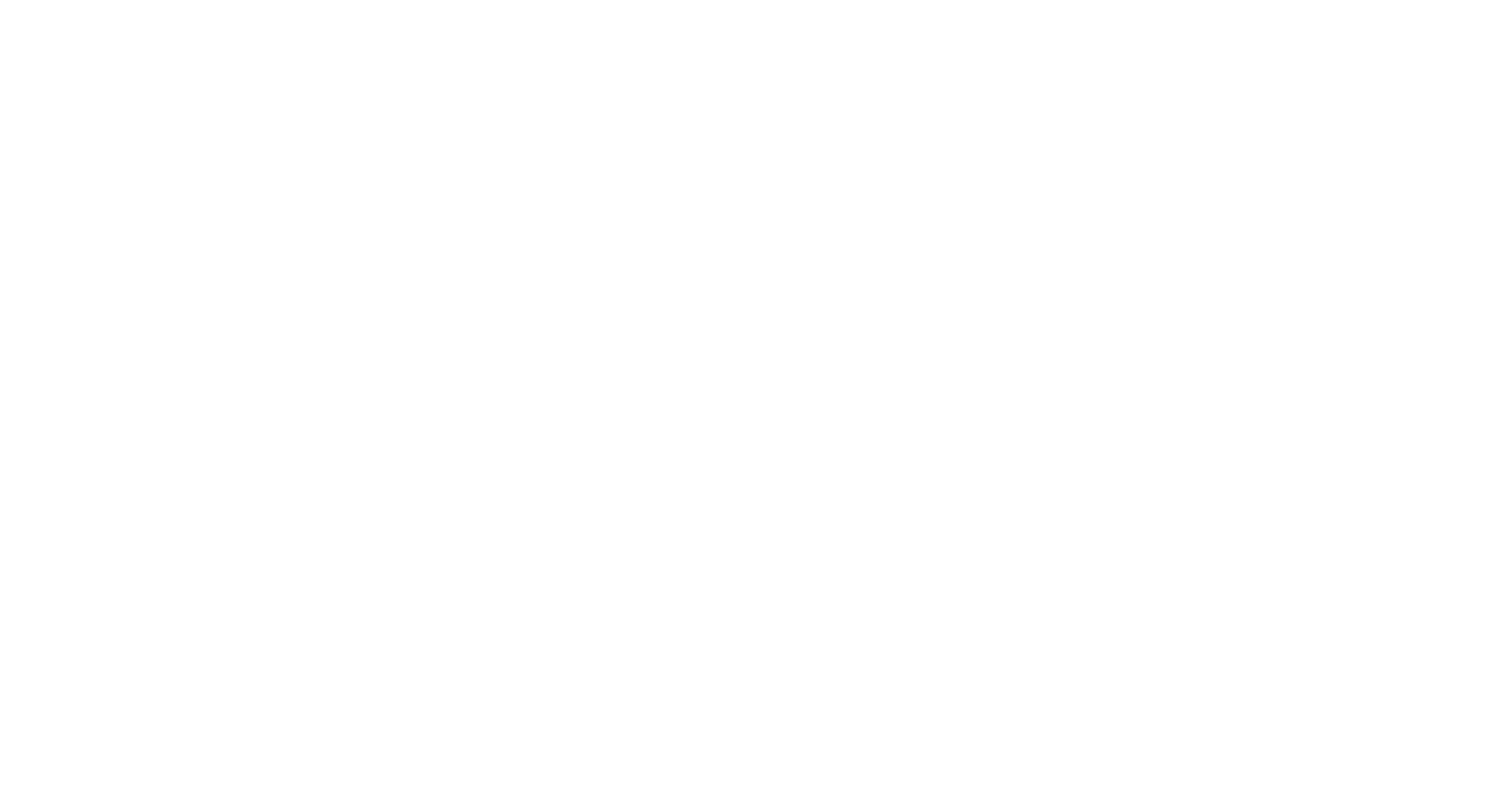
Introduction to Sugar Market API
Sugar Market, a part of the SugarCRM suite, is a powerful marketing automation platform designed to enhance the marketing efforts of businesses. It offers a range of features including email marketing, lead nurturing, and analytics, making it a popular choice for companies looking to streamline their marketing processes.
Integrating with the Sugar Market API allows developers to access and manage marketing data programmatically. For example, a developer might use the Sugar Market API to retrieve contact information, enabling the automation of personalized marketing campaigns based on customer interactions and preferences.
Setting Up a Sugar Market Test/Sandbox Account
Before you can start interacting with the Sugar Market API, you need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the necessary features to test API interactions.
- Visit the Sugar Market website and navigate to the sign-up page.
- Fill out the required information to create your account.
- Once your account is created, log in to access the dashboard.
Generating API Credentials for Sugar Market
To authenticate API requests, you'll need to generate API credentials. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account and navigate to the API settings section.
- Locate the option to create a new API application or token.
- Fill in the necessary details, such as the application name and permissions required.
- Once created, note down the client ID and client secret provided.
These credentials will be used to authenticate your API requests, allowing you to interact with Sugar Market programmatically.
For more detailed information on authentication, refer to the Sugar Market API documentation.
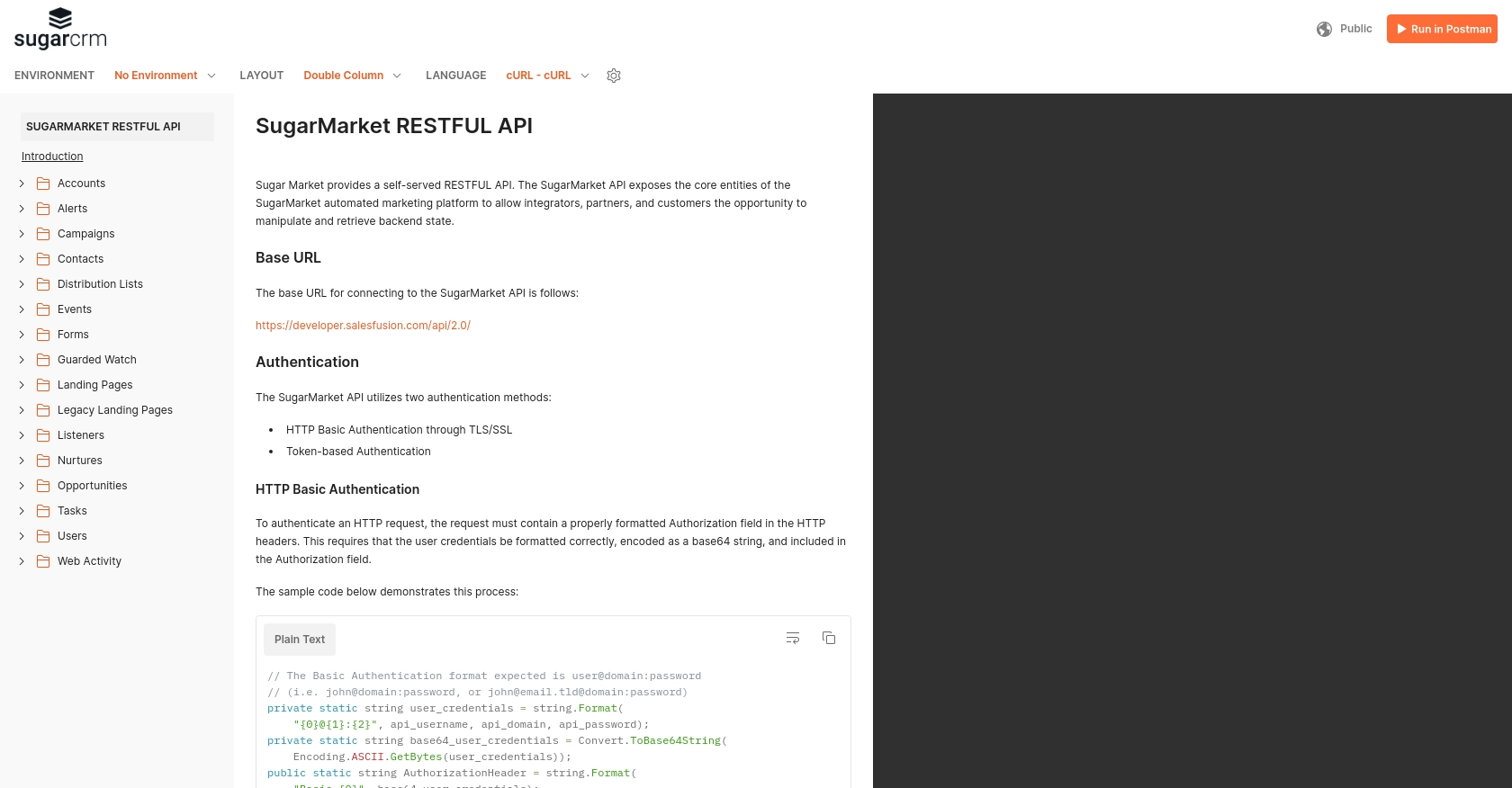
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Sugar Market Using JavaScript
To interact with the Sugar Market API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing the API call.
Setting Up Your JavaScript Environment for Sugar Market API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- Familiarity with JavaScript and asynchronous programming.
Once you have Node.js installed, you can use npm to install the axios
library, which simplifies making HTTP requests:
npm install axios
Executing the Sugar Market API Call to Get Contacts
With your environment set up, you can now write the JavaScript code to make the API call. Create a new file named getSugarMarketContacts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.sugarmarket.com/contacts';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.contacts;
// Display the contacts
contacts.forEach(contact => {
console.log(`Name: ${contact.firstName} ${contact.lastName}, Email: ${contact.email}`);
});
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace YOUR_ACCESS_TOKEN
with the token you obtained from the Sugar Market API credentials.
Running the JavaScript Code and Verifying the API Response
To execute the code, run the following command in your terminal:
node getSugarMarketContacts.js
If successful, you should see a list of contacts printed in the console. Verify the data by checking your Sugar Market sandbox account to ensure it matches the returned information.
Handling Errors and Troubleshooting
When making API calls, it's crucial to handle potential errors. The example code includes a try-catch
block to catch and log errors. Common issues might include:
- Invalid API credentials: Ensure your access token is correct and has the necessary permissions.
- Network issues: Check your internet connection and API endpoint URL.
- Rate limiting: Be aware of any rate limits imposed by Sugar Market and implement retry logic if needed.
For more detailed error information, refer to the Sugar Market API documentation.
Conclusion and Best Practices for Using Sugar Market API with JavaScript
Integrating with the Sugar Market API using JavaScript can significantly enhance your marketing automation efforts by allowing seamless access to contact data. By following the steps outlined in this guide, you can efficiently retrieve and manage contacts, enabling personalized marketing strategies.
Best Practices for Secure and Efficient API Integration
- Securely Store API Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of any rate limits imposed by Sugar Market. Implement logic to handle rate limit responses gracefully, such as exponential backoff or retry mechanisms.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This can help maintain consistency and improve data quality.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Sugar Market can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Sugar Market.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With its intuitive integration experience, you can build once for each use case and easily extend your integration capabilities as needed.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?