How to Get Companies with the Teamwork CRM API in Javascript
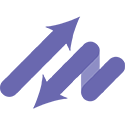
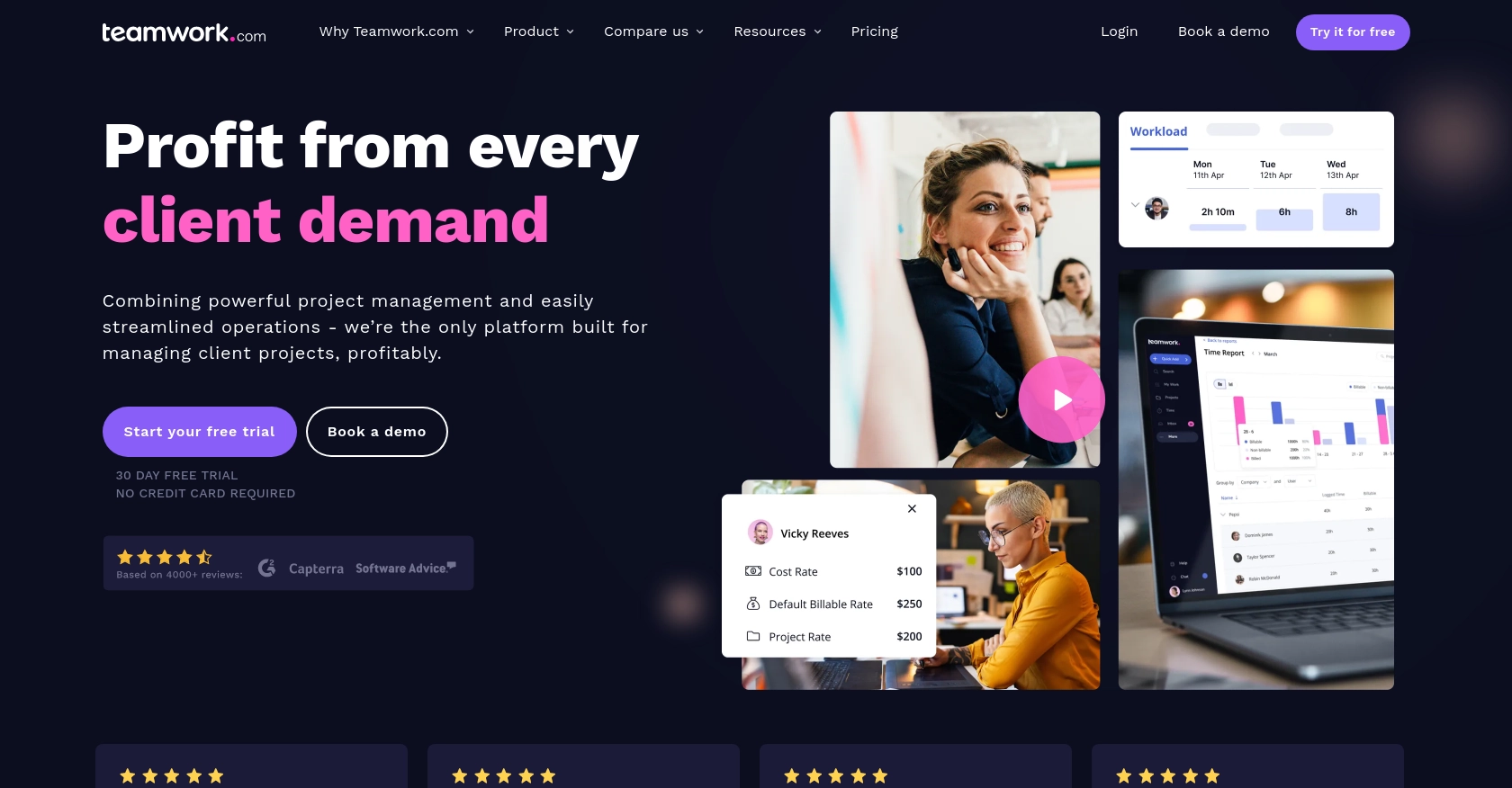
Introduction to Teamwork CRM API
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales processes efficiently. It offers a comprehensive suite of features that enable teams to track leads, manage deals, and nurture customer relationships seamlessly.
Integrating with the Teamwork CRM API allows developers to automate and enhance their sales workflows. For example, you might want to retrieve company data to analyze sales trends or integrate with other business tools for a more cohesive sales strategy.
This article will guide you through the process of using JavaScript to interact with the Teamwork CRM API, specifically focusing on retrieving company information. By the end of this tutorial, you'll be equipped to leverage the API for more efficient data management and integration.
Setting Up Your Teamwork CRM Test Account
Before you can start interacting with the Teamwork CRM API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Create a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. Follow the instructions to create your account and log in.
Generate API Credentials for Teamwork CRM
To authenticate your API requests, you'll need to generate API credentials. Teamwork CRM supports OAuth 2.0 for secure authentication. Follow these steps to set up your credentials:
- Navigate to the Developer Portal within your Teamwork CRM account.
- Register a new app to obtain your client ID and client secret.
- Once registered, you will be able to implement the OAuth 2.0 App Login Flow.
- Use the client ID and secret to generate an access token, which will be used in the Authorization header of your API requests.
For more detailed instructions, refer to the Teamwork CRM Authentication Documentation.
Understanding Custom Authentication
Teamwork CRM also allows for custom authentication methods. Ensure you follow the specific guidelines provided by Teamwork to securely authenticate your API requests.
Verify Your Setup
Once you have your API credentials, test your setup by making a simple API call to ensure everything is working correctly. You can use tools like Postman or cURL to verify your credentials and access token.
// Example using fetch in JavaScript
fetch('https://{yourSiteName}.teamwork.com/crm/v2/companies', {
method: 'GET',
headers: {
'Authorization': 'Bearer {your_access_token}'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace {yourSiteName}
and {your_access_token}
with your actual site name and access token.
If the request is successful, you should see a list of companies in the response. If you encounter any errors, refer to the Teamwork CRM Error Codes Documentation for troubleshooting.
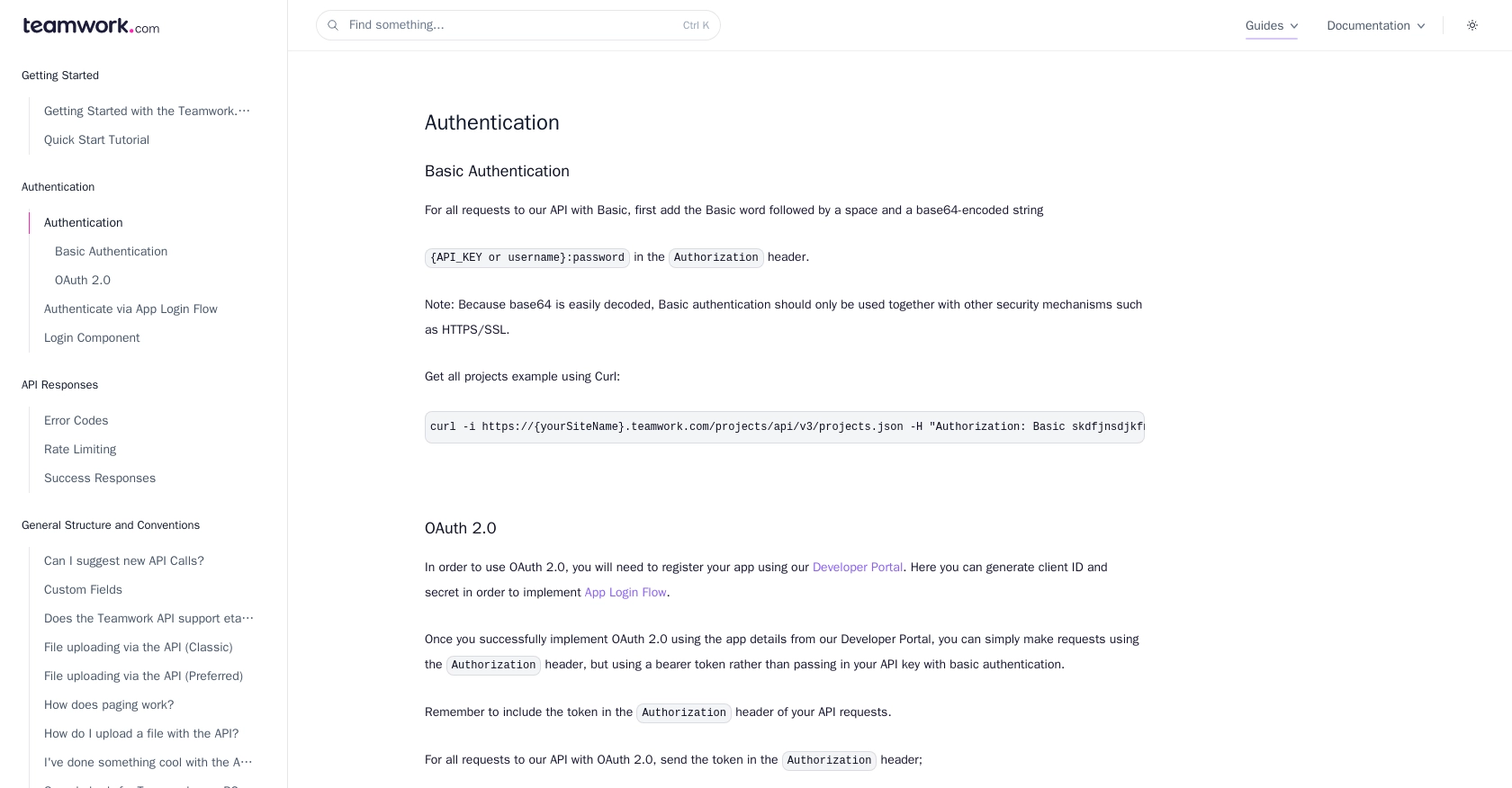
sbb-itb-96038d7
Making API Calls to Retrieve Companies with Teamwork CRM in JavaScript
To interact with the Teamwork CRM API using JavaScript, you'll need to ensure you have the correct environment set up. This section will guide you through the process of making API calls to retrieve company information using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have a modern JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use the Fetch API, which is supported in most modern browsers.
Installing Necessary Dependencies
If you're using Node.js, you might need to install the node-fetch
package to use the Fetch API. Run the following command to install it:
npm install node-fetch
Writing JavaScript Code to Fetch Company Data from Teamwork CRM
Now, let's write the JavaScript code to make an API call to Teamwork CRM to retrieve company data. Use the following code snippet:
const fetch = require('node-fetch'); // Only needed for Node.js
const endpoint = 'https://{yourSiteName}.teamwork.com/crm/v2/companies';
const accessToken = '{your_access_token}';
fetch(endpoint, {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log('Company Data:', data);
})
.catch(error => {
console.error('Error fetching company data:', error);
});
Replace {yourSiteName}
and {your_access_token}
with your actual Teamwork site name and access token.
Understanding the API Response and Error Handling
Upon a successful request, the API will return a JSON object containing the company data. You can log this data to the console or process it as needed for your application.
It's crucial to handle potential errors. The code snippet above checks for HTTP errors and logs them. Refer to the Teamwork CRM Error Codes Documentation for more information on handling specific error codes.
Verifying Successful API Requests in Teamwork CRM
To verify that your API requests are successful, you can cross-check the returned data with the information available in your Teamwork CRM account. Ensure that the data matches and that no errors are present in the console.
By following these steps, you can efficiently retrieve company data from Teamwork CRM using JavaScript, enabling seamless integration and data management within your applications.
Conclusion and Best Practices for Integrating Teamwork CRM API with JavaScript
Integrating with the Teamwork CRM API using JavaScript provides a powerful way to automate and enhance your sales processes. By retrieving company data, you can gain valuable insights and streamline your workflows, making your business operations more efficient.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to keep your access tokens safe.
- Handling Rate Limits: Be mindful of the rate limits imposed by Teamwork CRM. As per the documentation, ensure your application does not exceed 150 requests per minute to avoid disruptions.
- Error Handling: Implement robust error handling to manage potential issues gracefully. Refer to the error codes documentation for guidance on handling specific errors.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Leverage Endgrate for Seamless Integration Management
While integrating with APIs like Teamwork CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Teamwork CRM. This allows you to focus on your core product while outsourcing the complexities of integration management.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product offerings.
Read More
Ready to get started?