How to Create Leads with the Zoho CRM API in Python
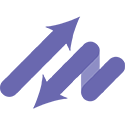
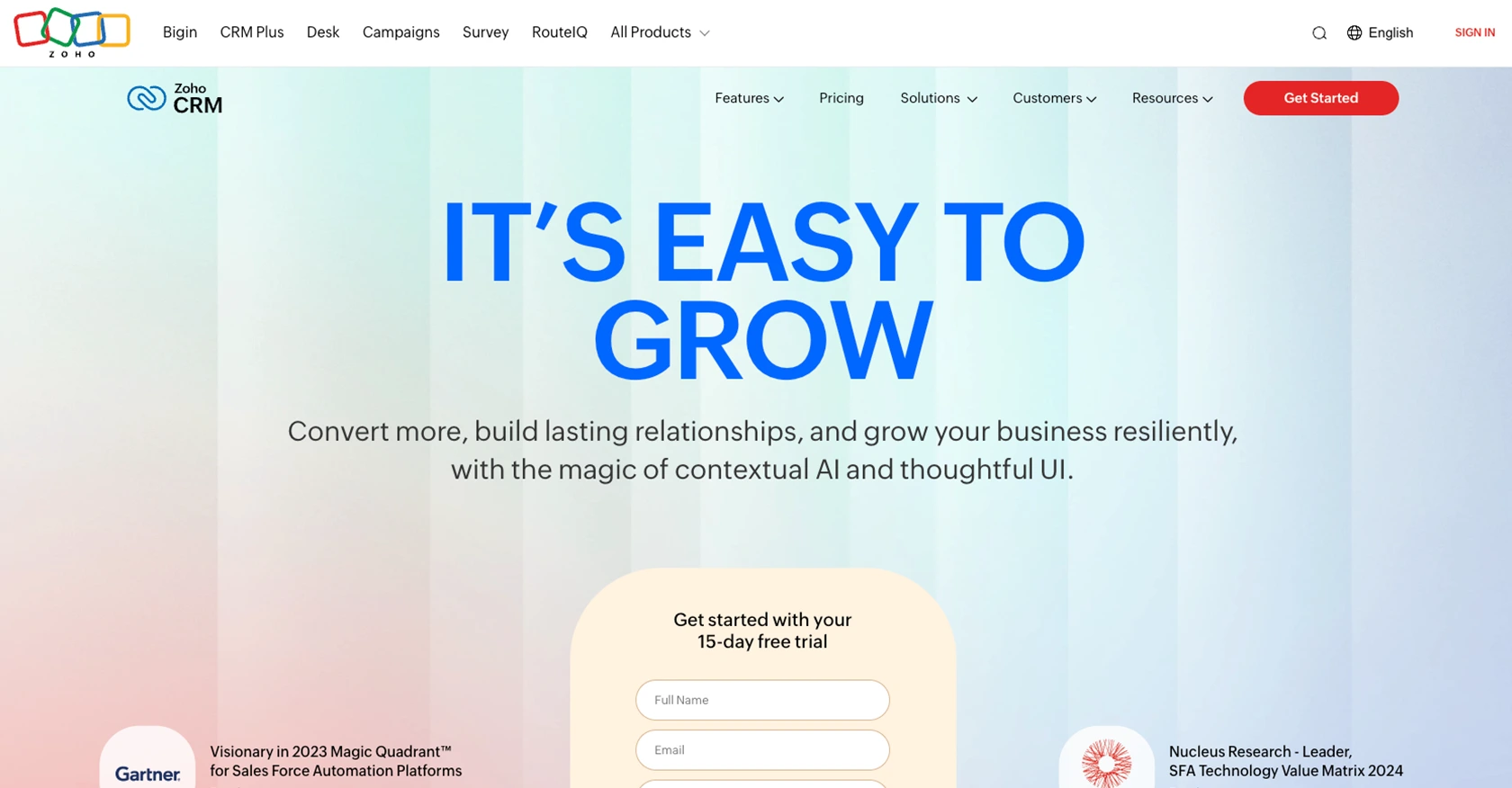
Introduction to Zoho CRM API
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and user-friendly interface, Zoho CRM is a popular choice for businesses looking to streamline their customer interactions and improve efficiency.
Developers often integrate with Zoho CRM to automate and enhance business processes. By leveraging the Zoho CRM API, developers can create, update, and manage leads, contacts, and other CRM entities programmatically. For example, you can automate the creation of new leads from a web form submission, ensuring that your sales team can follow up promptly and efficiently.
This article will guide you through the process of creating leads using the Zoho CRM API with Python, providing detailed steps and code examples to help you integrate seamlessly with Zoho CRM.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start creating leads with the Zoho CRM API, you'll need to set up a test or sandbox account. This allows you to safely test your integration without affecting live data. Zoho CRM offers a developer sandbox environment that mirrors your production setup, enabling you to test API interactions effectively.
Step 1: Register for a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, you can sign up for a free developer account. Visit the Zoho Developer Console and follow the instructions to create your account. This will give you access to the necessary tools and resources for API integration.
Step 2: Create a Zoho CRM Sandbox
Once your developer account is set up, you can create a sandbox environment:
- Log in to your Zoho CRM account.
- Navigate to the Setup section.
- Under Developer Space, select Sandbox.
- Click on Create Sandbox and follow the prompts to set up your sandbox environment.
Step 3: Set Up OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, ensuring secure access to your CRM data. Follow these steps to configure OAuth:
- In the Zoho Developer Console, register your application.
- Choose the appropriate client type (e.g., Web Based, Self Client).
- Enter the required details, including Client Name, Homepage URL, and Authorized Redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Step 4: Generate Access and Refresh Tokens
With your Client ID and Client Secret, you can now generate access tokens:
- Make an authorization request to Zoho's OAuth server.
- Exchange the authorization code for access and refresh tokens.
- Store these tokens securely, as they will be used to authenticate API requests.
For detailed steps, refer to the Zoho CRM OAuth documentation.
With your sandbox environment and OAuth authentication set up, you're ready to start integrating with the Zoho CRM API using Python.
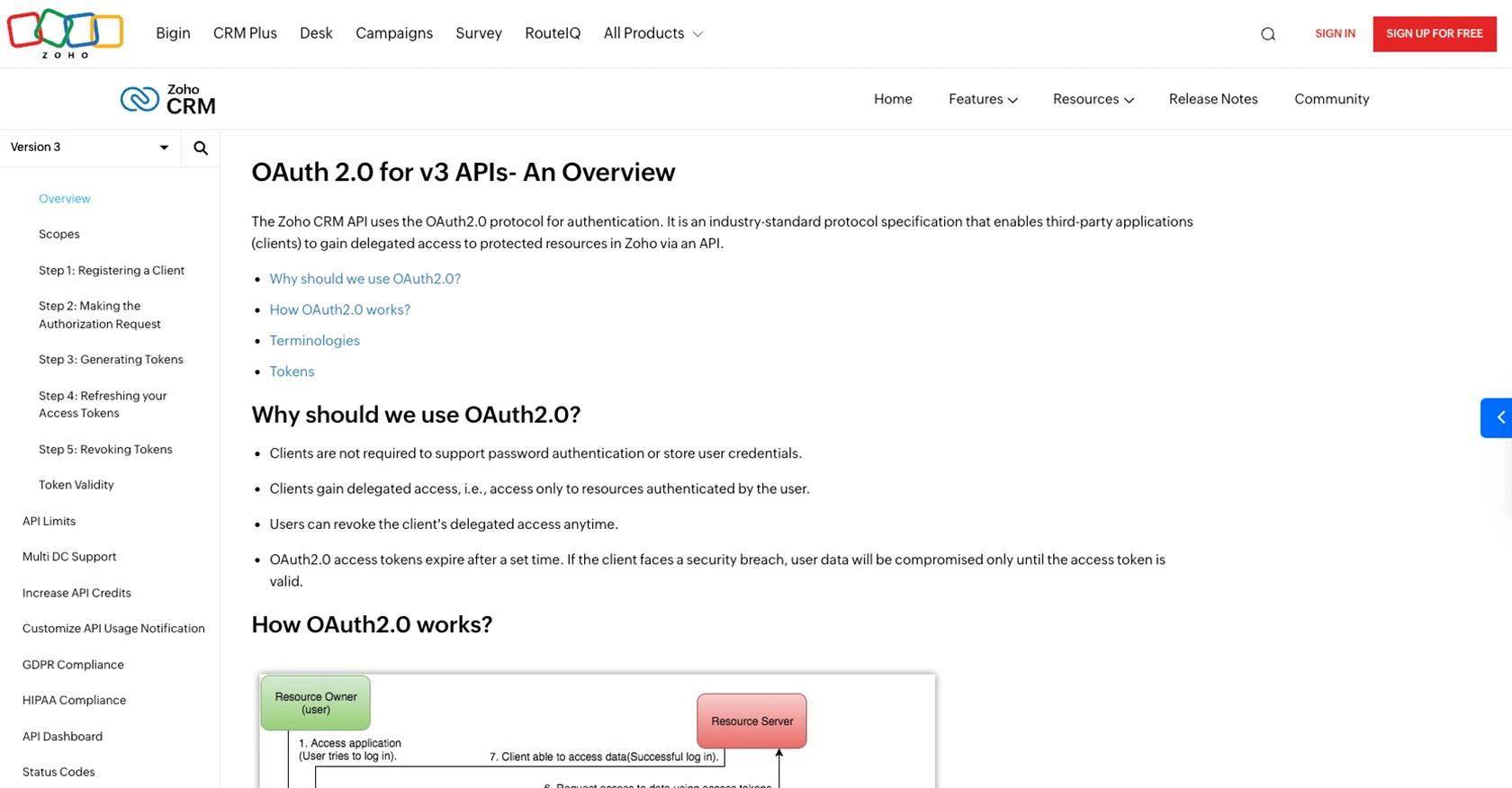
sbb-itb-96038d7
Making API Calls to Create Leads in Zoho CRM Using Python
To interact with the Zoho CRM API and create leads using Python, you'll need to ensure your development environment is properly set up. This involves installing the necessary dependencies and writing the code to make API requests.
Setting Up Your Python Environment for Zoho CRM API Integration
Before you begin coding, make sure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Ensure Python 3.11.1 is installed. You can download it from the official Python website.
- Install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Create Leads in Zoho CRM
With your environment set up, you can now write the Python code to create leads in Zoho CRM. The following example demonstrates how to make a POST request to the Zoho CRM API to add a new lead.
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/crm/v3/Leads"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token",
"Content-Type": "application/json"
}
# Define the lead data
lead_data = {
"data": [
{
"Last_Name": "Doe",
"First_Name": "John",
"Email": "john.doe@example.com",
"Company": "Example Corp"
}
]
}
# Make the POST request to create a lead
response = requests.post(url, json=lead_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Lead created successfully:", response.json())
else:
print("Failed to create lead:", response.status_code, response.json())
Replace Your_Access_Token
with the access token you obtained during the OAuth setup. The lead_data
dictionary contains the information for the lead you want to create, such as the last name, first name, email, and company.
Verifying Successful Lead Creation in Zoho CRM
After running the script, you should verify that the lead was successfully created in your Zoho CRM sandbox environment:
- Log in to your Zoho CRM account.
- Navigate to the Leads module.
- Check for the newly created lead with the details you provided.
Handling Errors and Zoho CRM API Response Codes
When making API calls, it's important to handle potential errors. The Zoho CRM API may return various status codes indicating the success or failure of your request. Common error codes include:
- 400 Bad Request: The request was invalid. Check your input data.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You do not have permission to perform this action.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For a complete list of status codes and their meanings, refer to the Zoho CRM API documentation.
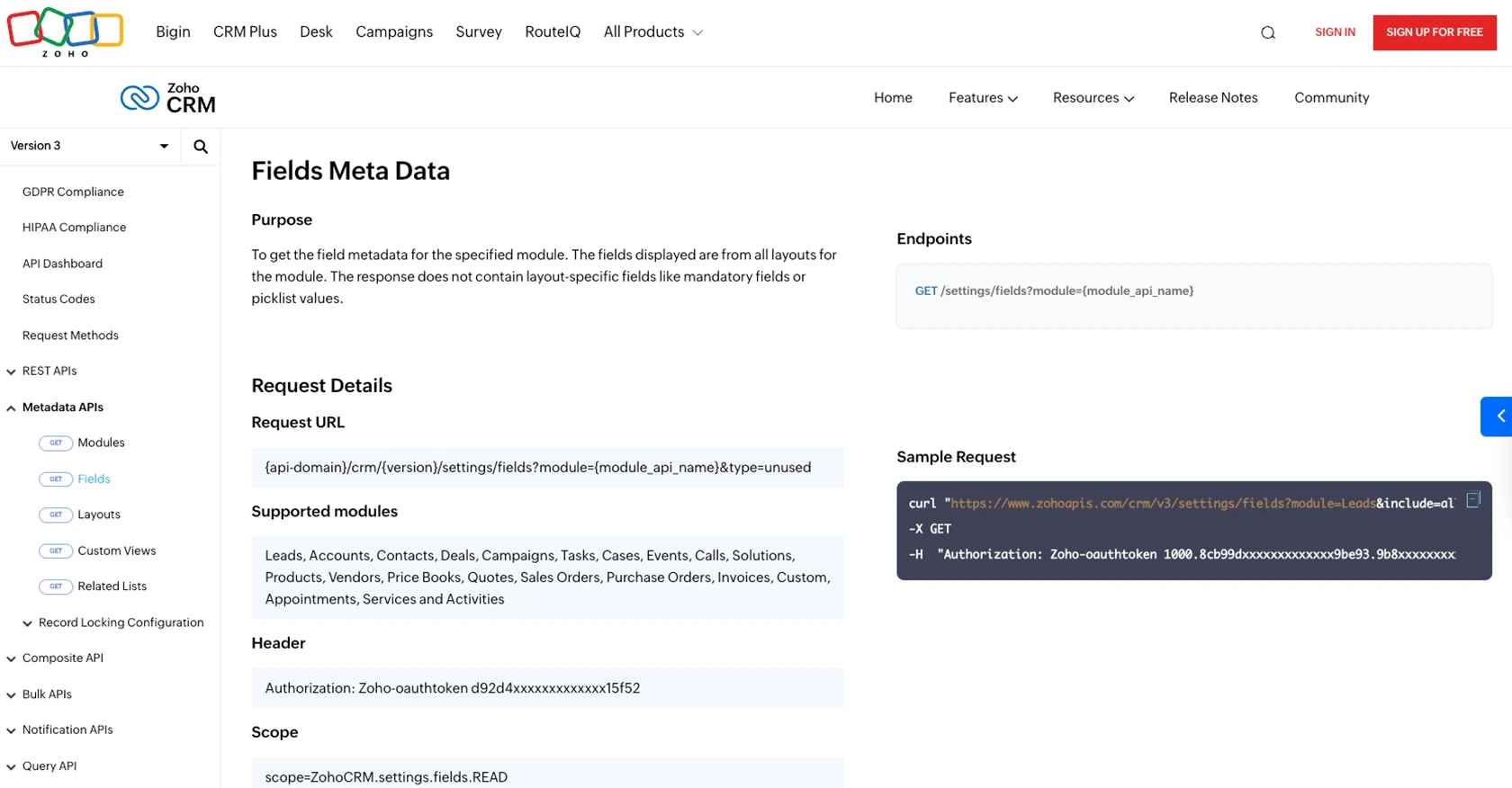
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using Python allows developers to automate lead management and streamline business processes. By following the steps outlined in this guide, you can efficiently create leads and enhance your CRM capabilities.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Token Storage: Always store your OAuth tokens securely. Avoid exposing them in public repositories or client-side code to prevent unauthorized access.
- Handle Rate Limits: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic to handle rate limit errors gracefully. For more details, refer to the API limits documentation.
- Data Standardization: Ensure that the data you send to Zoho CRM is standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling in your code to manage API response codes effectively and provide meaningful feedback to users.
Enhance Your Integration Strategy with Endgrate
While integrating with Zoho CRM directly is powerful, managing multiple integrations can be complex and time-consuming. Consider using Endgrate to simplify your integration strategy. With Endgrate, you can:
- Outsource integrations and focus on your core product development.
- Build once for each use case and apply it across multiple platforms.
- Provide an intuitive integration experience for your customers.
Visit Endgrate to learn how you can streamline your integration processes and enhance your product offerings.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?