Using the ZoomInfo API to Get Contacts in Javascript
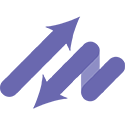
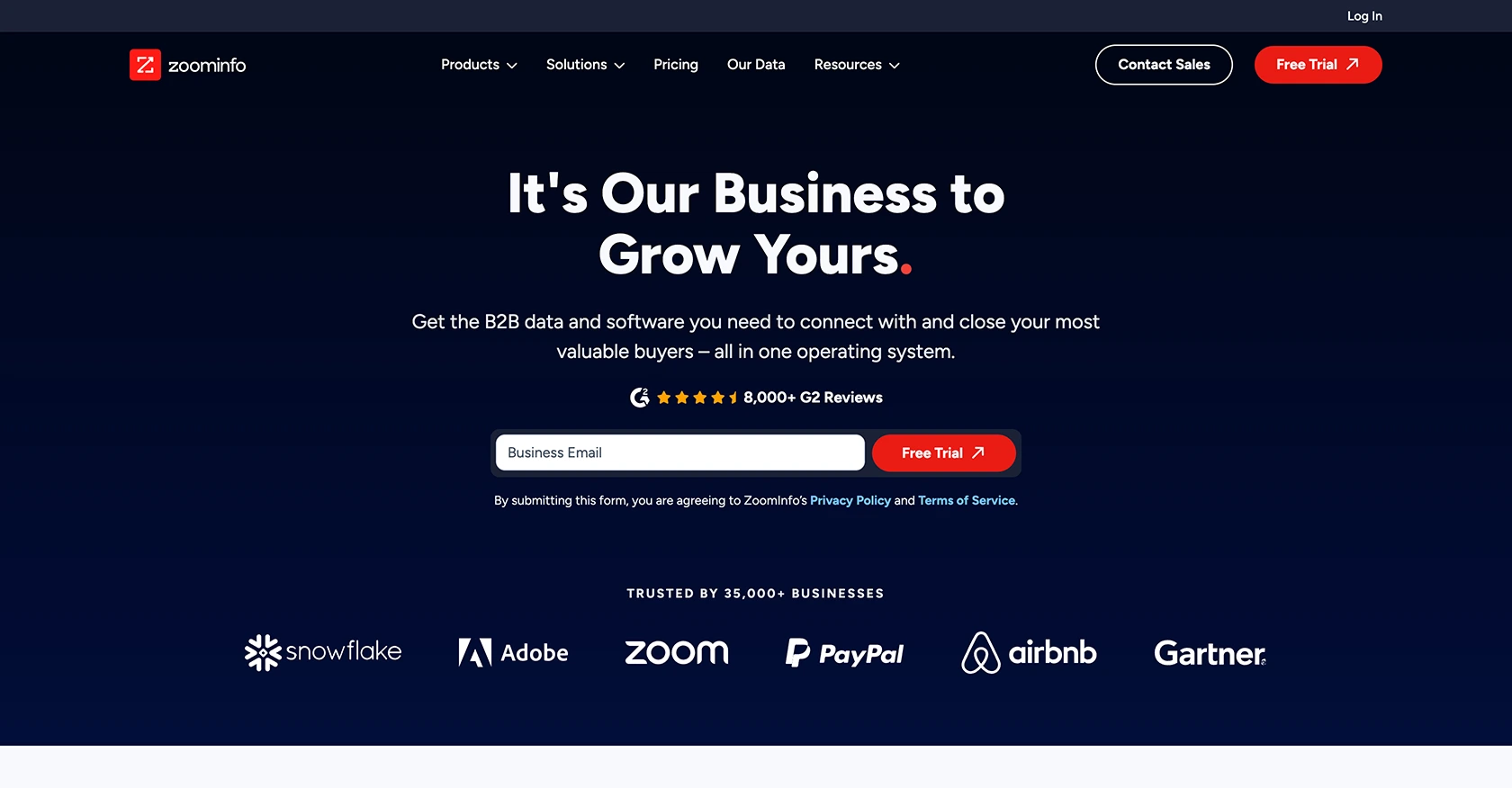
Introduction to ZoomInfo API for Contact Retrieval
ZoomInfo is a powerful business intelligence platform that provides comprehensive data on companies and professionals. It offers a vast database of contact information, company profiles, and industry insights, making it an invaluable tool for sales and marketing teams looking to enhance their outreach strategies.
For developers, integrating with the ZoomInfo API can streamline the process of accessing and managing contact data. By leveraging this API, developers can automate the retrieval of contact information, enabling seamless integration with CRM systems or other business applications. For example, a developer might use the ZoomInfo API to fetch contact details of potential leads and automatically populate a sales pipeline, enhancing the efficiency of lead generation efforts.
Setting Up a ZoomInfo Test or Sandbox Account for API Integration
Before you can start using the ZoomInfo API to retrieve contact information, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. Follow these steps to get started:
Creating a ZoomInfo Developer Account
To access the ZoomInfo API, you must first create a developer account. Visit the ZoomInfo Developer Portal and sign up for an account. This will give you access to the necessary credentials and documentation.
Generating API Credentials for ZoomInfo
Once your developer account is set up, you need to generate API credentials. These credentials typically include a client ID and client secret, which are essential for authenticating your API requests.
- Log in to your ZoomInfo developer account.
- Navigate to the API credentials section.
- Click on "Create New Credentials" and follow the prompts to generate your client ID and client secret.
- Store these credentials securely, as you'll need them to authenticate your API calls.
Understanding ZoomInfo's Custom Authentication
The ZoomInfo API uses a custom authentication method. Ensure you understand how to implement this authentication in your JavaScript code. Refer to the ZoomInfo Authentication Documentation for detailed instructions.
Testing Your ZoomInfo API Setup
With your credentials in hand, you can now test your setup by making a simple API call. This will verify that your authentication is working correctly and that you can access the data you need.
// Example JavaScript code to test ZoomInfo API authentication
const axios = require('axios');
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
async function testZoomInfoAPI() {
try {
const response = await axios.post('https://api.zoominfo.com/authenticate', {
clientId: clientId,
clientSecret: clientSecret
});
console.log('Authentication successful:', response.data);
} catch (error) {
console.error('Error authenticating with ZoomInfo API:', error);
}
}
testZoomInfoAPI();
Replace YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with your actual credentials. Run this script to ensure that you receive a successful authentication response.
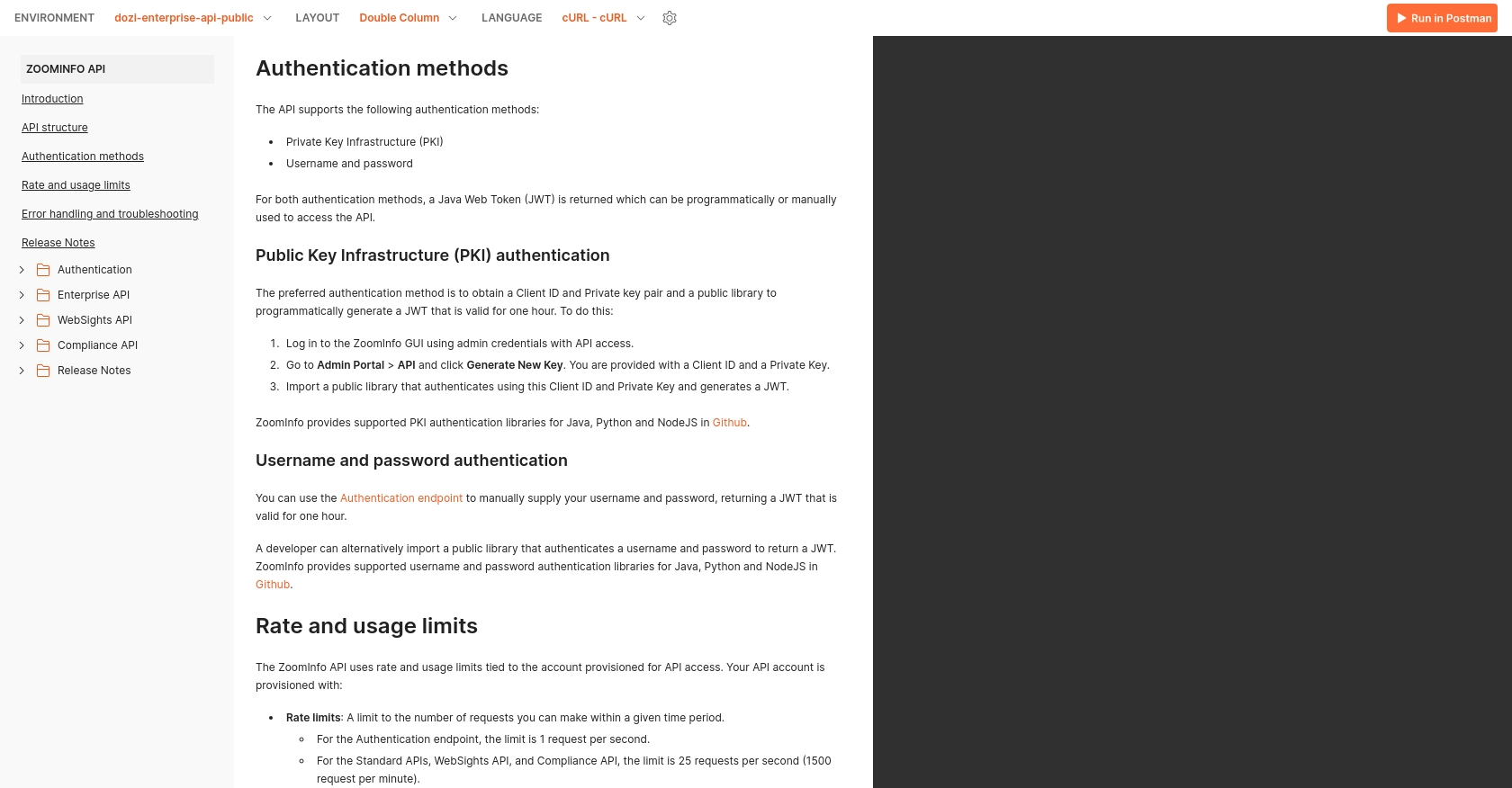
sbb-itb-96038d7
Making API Calls to ZoomInfo for Contact Retrieval Using JavaScript
Once you've set up your ZoomInfo developer account and obtained your API credentials, you're ready to make API calls to retrieve contact information. This section will guide you through the process of setting up your JavaScript environment and making API requests to ZoomInfo.
Setting Up Your JavaScript Environment for ZoomInfo API Integration
To interact with the ZoomInfo API using JavaScript, you'll need to ensure your environment is properly configured. Follow these steps to get started:
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Use npm (Node Package Manager) to install the Axios library, which will help you make HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Retrieve Contacts from ZoomInfo
With your environment set up, you can now write the JavaScript code to make API calls to ZoomInfo and retrieve contact information. Below is an example of how to achieve this:
const axios = require('axios');
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
async function getZoomInfoContacts() {
try {
// Authenticate and get the access token
const authResponse = await axios.post('https://api.zoominfo.com/authenticate', {
clientId: clientId,
clientSecret: clientSecret
});
const accessToken = authResponse.data.accessToken;
// Set the API endpoint and headers
const endpoint = 'https://api.zoominfo.com/contacts';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Make a GET request to the API
const response = await axios.get(endpoint, { headers: headers });
// Log the retrieved contacts
console.log('Contacts retrieved:', response.data);
} catch (error) {
console.error('Error retrieving contacts from ZoomInfo API:', error);
}
}
getZoomInfoContacts();
Replace YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with your actual credentials. This script first authenticates with the ZoomInfo API to obtain an access token, then uses that token to make a GET request to retrieve contact information.
Verifying Successful API Requests and Handling Errors
After running your script, you should see the retrieved contacts printed in your console. To verify the success of your API requests, ensure that the data matches the contacts available in your ZoomInfo sandbox account.
If you encounter errors, check the error message for details. Common issues might include incorrect credentials or network problems. Refer to the ZoomInfo Authentication Documentation for troubleshooting tips.
Best Practices for Using the ZoomInfo API in JavaScript
When working with the ZoomInfo API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to keep these credentials safe from unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the ZoomInfo API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay. Refer to the ZoomInfo API documentation for specific rate limit details.
- Data Transformation and Standardization: When retrieving contact data, ensure that you transform and standardize the data fields to match your application's requirements. This will facilitate seamless integration with your existing systems.
Streamlining Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and resource-intensive. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including ZoomInfo. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?