Using the Sage Accounting API to Create or Update Item in Python
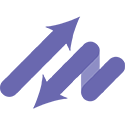
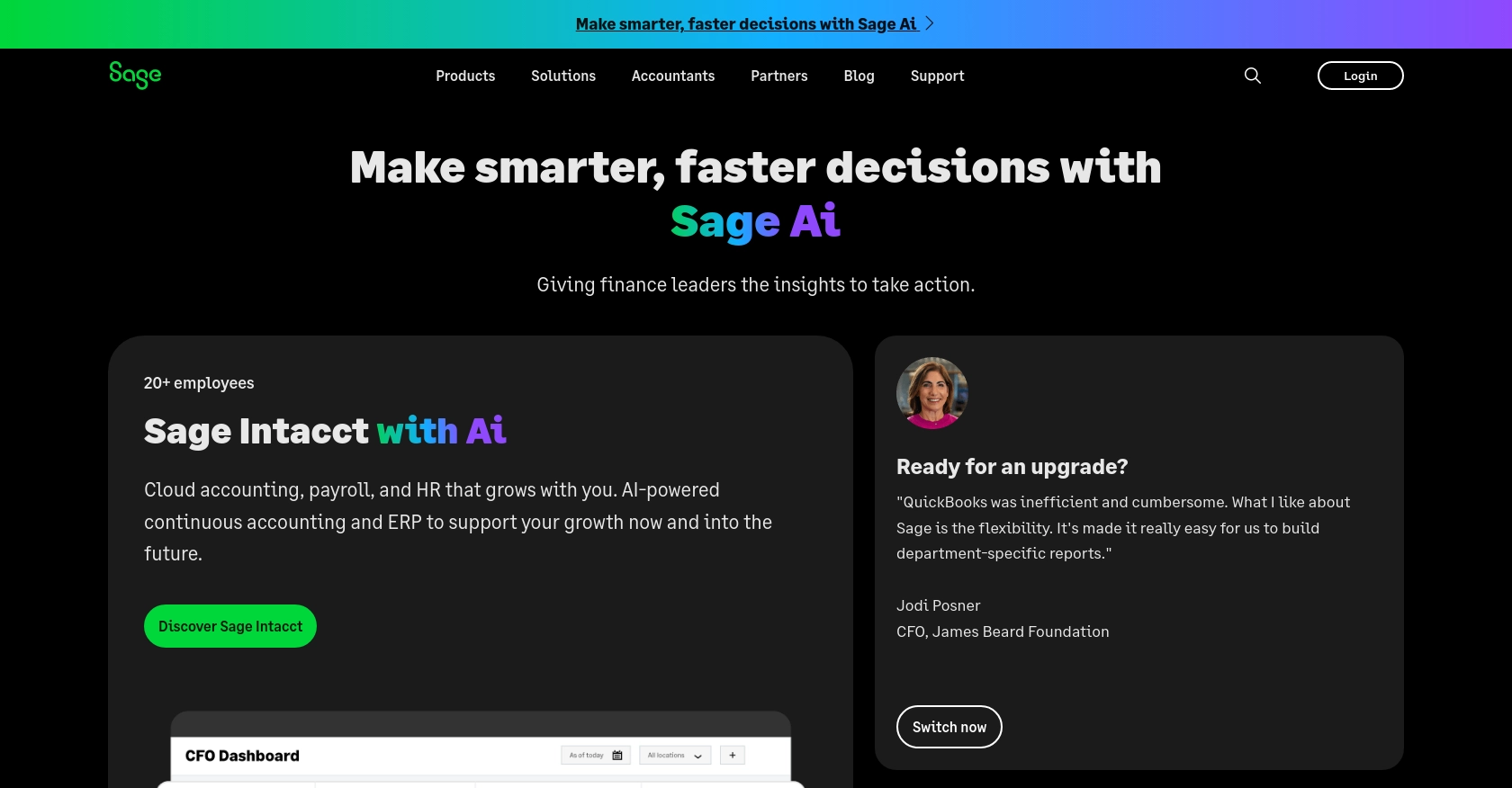
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage finances, track expenses, and streamline accounting processes. With its user-friendly interface and powerful features, Sage Accounting is a popular choice for businesses looking to enhance their financial management capabilities.
Integrating with the Sage Accounting API allows developers to automate and optimize various accounting tasks. For example, you can create or update items in the inventory directly from your application, ensuring that your product data is always up-to-date and accurate. This integration can significantly enhance efficiency by reducing manual data entry and minimizing errors.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting real data, making it an essential step for development and testing.
Step 1: Sign Up for a Sage Developer Account
To begin, you'll need a Sage Developer account. This account provides access to the necessary tools and resources for integrating with Sage Accounting.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the instructions to complete your registration.
Step 2: Create a Trial Business Account
Next, create a trial business account to use as your sandbox environment. This account will allow you to test API interactions without impacting live data.
- Navigate to the Sage Accounting Quick Start Guide.
- Select the appropriate region and subscription tier for your trial account.
- Follow the prompts to set up your trial business.
Step 3: Register a New Application
With your developer account and trial business set up, the next step is to register a new application. This will provide you with the client credentials needed for OAuth authentication.
- Log in to your Sage Developer account.
- Click on "Create App" and enter the required details, including a name and callback URL for your app.
- Save your app to generate a Client ID and Client Secret.
- Refer to the Create an App Guide for more details.
Step 4: Upgrade to a Developer Account
To fully utilize the sandbox environment, upgrade your account to a developer account. This provides extended access for testing purposes.
- Submit a request through the Upgrade Your Account page.
- Provide details such as your name, email, app name, and Client ID.
- Wait for confirmation, which typically takes 3-5 working days.
Once these steps are completed, you'll be ready to start making API calls to the Sage Accounting sandbox environment, allowing you to create or update items with confidence.
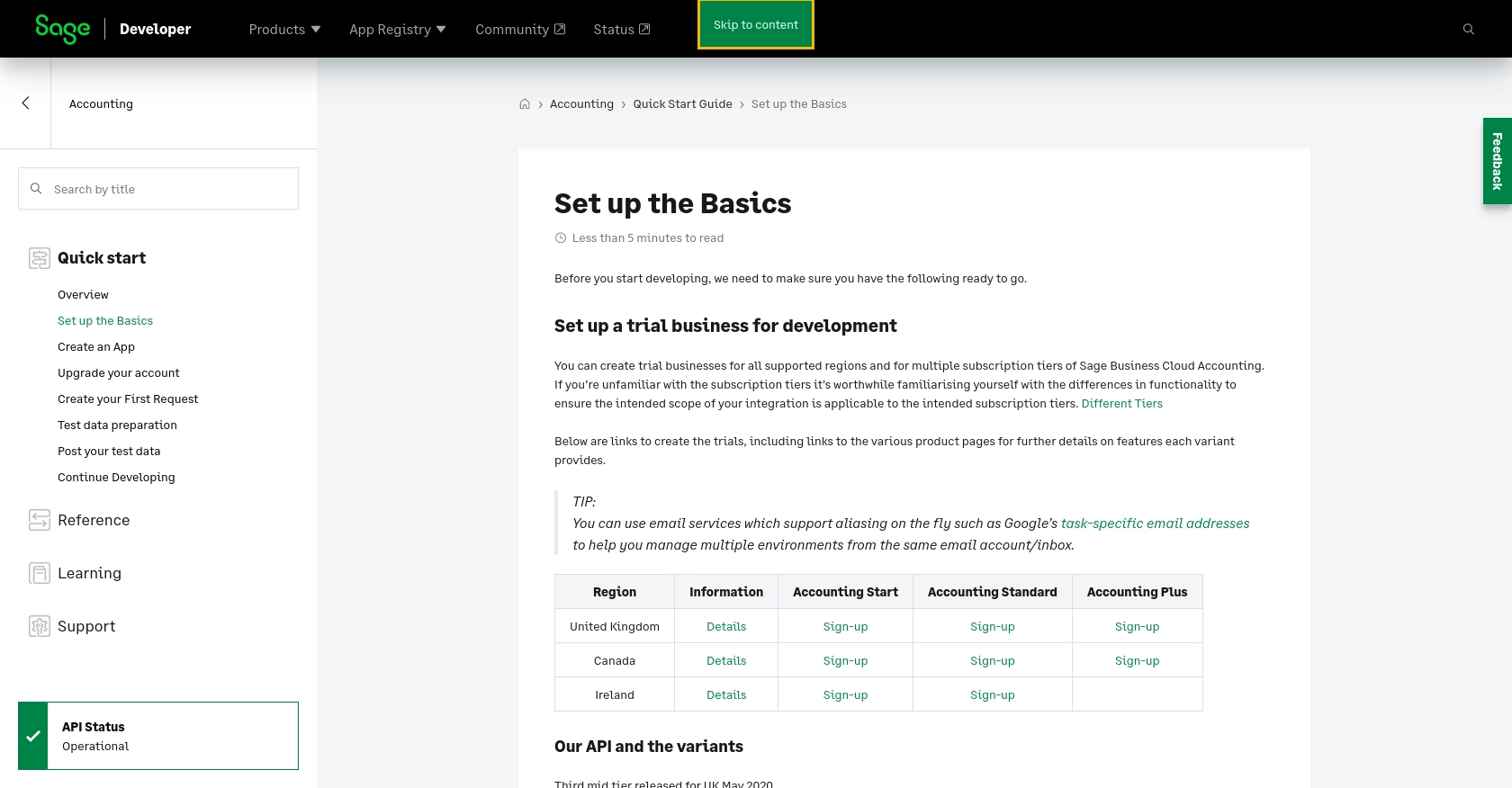
sbb-itb-96038d7
Making API Calls to Create or Update Items in Sage Accounting Using Python
Prerequisites for Using Python with Sage Accounting API
To interact with the Sage Accounting API using Python, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or shell and install the requests
library using the following command:
pip install requests
Creating a New Item in Sage Accounting
To create a new item in Sage Accounting, follow these steps:
- Create a file named
create_item.py
and add the following code:
import requests
# Set the API endpoint
url = "https://api.accounting.sage.com/v3.1/products"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the item data
item_data = {
"product": {
"description": "New Product",
"sales_ledger_account_id": "string",
"purchase_ledger_account_id": "string",
"item_code": "NP001",
"notes": "Sample product",
"sales_tax_rate_id": "string",
"usual_supplier_id": "string",
"purchase_tax_rate_id": "string",
"cost_price": 10.0,
"active": True
}
}
# Make the POST request
response = requests.post(url, json=item_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Item created successfully:", response.json())
else:
print("Failed to create item:", response.status_code, response.text)
Replace Your_Token
with the token obtained from your Sage Developer account.
Run the code using the command:
python create_item.py
Upon success, you should see the details of the newly created item in the output.
Updating an Existing Item in Sage Accounting
To update an existing item, follow these steps:
- Create a file named
update_item.py
and add the following code:
import requests
# Set the API endpoint with the item key
url = "https://api.accounting.sage.com/v3.1/products/{item_key}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the updated item data
updated_data = {
"product": {
"description": "Updated Product",
"cost_price": 12.0,
"active": True
}
}
# Make the PUT request
response = requests.put(url, json=updated_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Item updated successfully:", response.json())
else:
print("Failed to update item:", response.status_code, response.text)
Replace Your_Token
and {item_key}
with your token and the specific item key you wish to update.
Run the code using the command:
python update_item.py
Upon success, you should see the updated details of the item in the output.
Handling Errors and Verifying API Calls
To ensure your API calls are successful, always check the response status codes:
- 201: Indicates successful creation of an item.
- 200: Indicates successful update of an item.
- For other status codes, refer to the Sage Accounting API documentation for error handling guidance.
Verify the changes in your Sage Accounting sandbox environment to ensure the items are created or updated as expected.
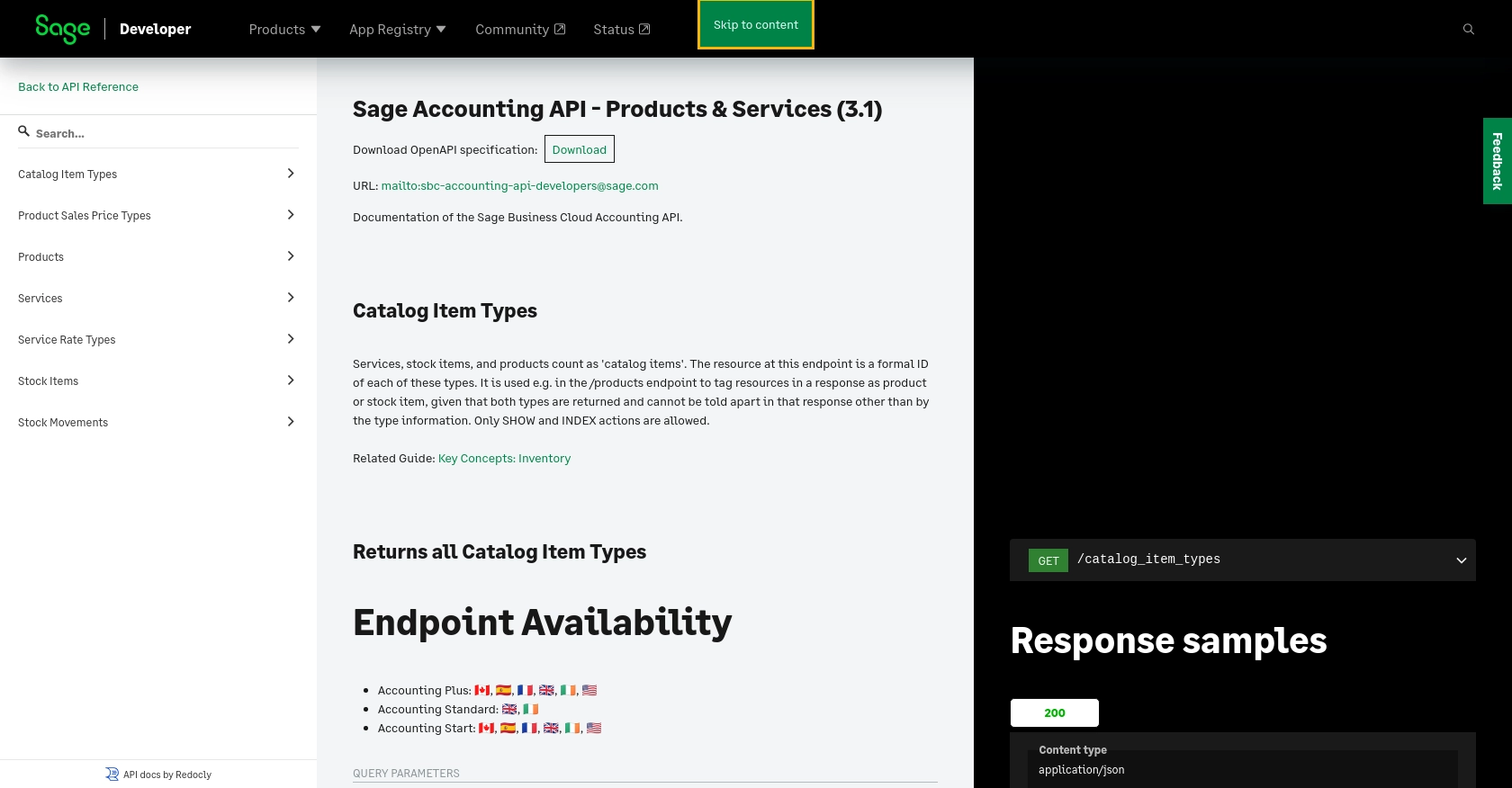
Conclusion and Best Practices for Using Sage Accounting API
Integrating with the Sage Accounting API using Python offers a powerful way to automate and streamline your accounting processes. By creating or updating items directly through the API, you can ensure that your inventory data remains accurate and up-to-date, reducing manual errors and saving valuable time.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure consistency in data fields when creating or updating items. This helps maintain data integrity across your applications.
- Monitor API Responses: Regularly check API responses and logs to identify and resolve any issues promptly. This proactive approach helps maintain seamless integration.
Enhance Your Integration Strategy with Endgrate
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. With Endgrate, you can streamline your integration processes, allowing you to focus on your core product development. Endgrate's unified API endpoint connects to multiple platforms, including Sage Accounting, providing a seamless and intuitive integration experience.
By using Endgrate, you can build once for each use case and apply it across various integrations, saving both time and resources. Visit Endgrate to learn more about how it can transform your integration strategy.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/products-services/
Ready to get started?