How to Export Data with the FTP API in PHP
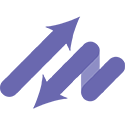
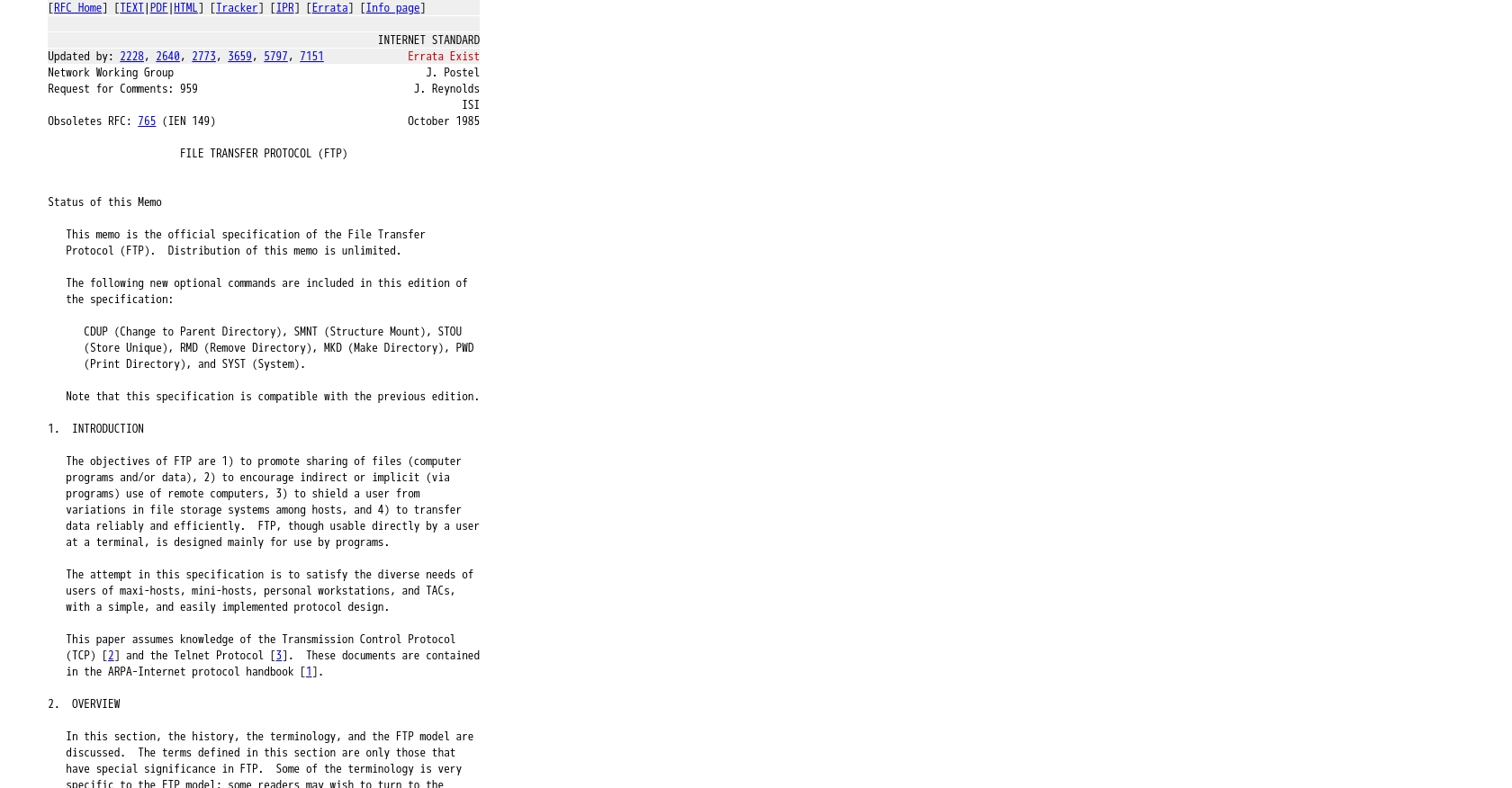
Introduction to FTP API Integration
File Transfer Protocol (FTP) is a standard network protocol used to transfer files between a client and server on a computer network. It is widely used for uploading and downloading files, making it an essential tool for businesses that need to manage large volumes of data efficiently.
Integrating with the FTP API allows developers to automate data export processes, such as transferring daily reports or backups to a remote server. For example, a developer might use the FTP API to export sales data from a local database to a secure server for archiving or further analysis.
This article will guide you through the process of exporting data using the FTP API in PHP, providing detailed steps to set up the connection and perform file transfers seamlessly.
Setting Up Your FTP Test Environment
Before you can start exporting data using the FTP API in PHP, you need to set up a test environment. This involves creating an FTP server account where you can safely test your file transfers without affecting live data.
Creating an FTP Server Account
To begin, you need access to an FTP server. You can either set up a local FTP server on your machine or use a cloud-based service. Many hosting providers offer free FTP accounts as part of their service packages.
- For a local setup, consider using software like FileZilla Server or XAMPP, which allows you to create an FTP server on your computer.
- If you prefer a cloud-based solution, platforms like AWS, Google Cloud, or DigitalOcean provide FTP services that you can configure easily.
Configuring FTP Server Credentials
Once your FTP server is ready, you need to configure the credentials that PHP will use to connect to the server. This typically includes:
- FTP Host: The server address, which could be an IP address or a domain name.
- FTP Username: The username for accessing the FTP server.
- FTP Password: The password associated with the FTP username.
- FTP Port: The port number, usually 21 for FTP connections.
Using PHP to Connect to the FTP Server
To interact with the FTP server using PHP, you'll need to use a language-specific library. PHP's built-in ftp_connect
function is commonly used for this purpose. Here's a basic example of how to establish a connection:
$ftp_server = "ftp.example.com";
$ftp_username = "your_username";
$ftp_userpass = "your_password";
// Establishing connection
$conn_id = ftp_connect($ftp_server);
// Logging in
$login_result = ftp_login($conn_id, $ftp_username, $ftp_userpass);
// Check connection
if ((!$conn_id) || (!$login_result)) {
echo "FTP connection has failed!";
exit;
} else {
echo "Connected to $ftp_server, for user $ftp_username";
}
Replace ftp.example.com
, your_username
, and your_password
with your actual FTP server details.
With your test environment set up, you are now ready to proceed with exporting data using the FTP API in PHP.
Executing FTP API Calls in PHP for Data Export
To effectively export data using the FTP API in PHP, you'll need to ensure that your development environment is properly configured. This section will guide you through the necessary steps to perform FTP operations, including uploading files to the server.
Setting Up PHP Environment for FTP Operations
Before making any FTP API calls, ensure that your PHP environment is ready. You should have PHP installed on your machine, preferably version 7.4 or later, to take advantage of the latest features and security updates. Additionally, make sure the FTP extension is enabled in your php.ini
file.
- Check your PHP version by running
php -v
in the terminal. - Ensure the FTP extension is enabled by looking for
extension=ftp
in yourphp.ini
file.
Uploading Files to FTP Server Using PHP
Once your environment is set up, you can proceed with uploading files to the FTP server. Below is a sample code snippet demonstrating how to upload a file using PHP's FTP functions:
$ftp_server = "ftp.example.com";
$ftp_username = "your_username";
$ftp_userpass = "your_password";
$file_to_upload = "local_file.txt";
$remote_file = "remote_file.txt";
// Establishing connection
$conn_id = ftp_connect($ftp_server);
// Logging in
$login_result = ftp_login($conn_id, $ftp_username, $ftp_userpass);
// Check connection
if ((!$conn_id) || (!$login_result)) {
echo "FTP connection has failed!";
exit;
} else {
echo "Connected to $ftp_server, for user $ftp_username";
}
// Uploading the file
if (ftp_put($conn_id, $remote_file, $file_to_upload, FTP_ASCII)) {
echo "Successfully uploaded $file_to_upload.";
} else {
echo "There was a problem uploading $file_to_upload.";
}
// Closing the connection
ftp_close($conn_id);
Replace ftp.example.com
, your_username
, your_password
, and local_file.txt
with your actual FTP server details and file paths.
Verifying Successful FTP Data Export
After executing the FTP upload, it's crucial to verify that the file has been successfully transferred to the server. You can do this by checking the remote server directory for the presence of the uploaded file. Additionally, ensure that the file size and content match the original file.
Handling FTP Errors and Exceptions in PHP
While performing FTP operations, you may encounter errors such as connection failures or permission issues. It's important to handle these errors gracefully to ensure a robust integration. Consider implementing error handling mechanisms using PHP's built-in functions:
- Check the return values of FTP functions and use conditional statements to handle errors.
- Log error messages for debugging purposes and provide user-friendly feedback.
By following these steps, you can efficiently export data using the FTP API in PHP, ensuring a seamless and automated file transfer process.
Conclusion and Best Practices for FTP API Integration in PHP
Exporting data using the FTP API in PHP can significantly streamline your data management processes, allowing for automated and efficient file transfers. By following the steps outlined in this guide, you can set up a robust FTP integration that meets your business needs.
Best Practices for Secure and Efficient FTP API Usage
- Secure Credentials: Always store FTP credentials securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by your FTP server provider. Implement retry logic and backoff strategies to handle rate limiting gracefully.
- Data Validation: Ensure that data is validated before transfer to prevent errors and maintain data integrity.
- Error Handling: Implement comprehensive error handling to manage connection issues and file transfer errors effectively.
Enhancing Your Integration Strategy with Endgrate
While integrating with the FTP API in PHP is a powerful solution, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including FTP.
By leveraging Endgrate, you can focus on your core product development while outsourcing the intricacies of integration management. This approach not only saves time and resources but also provides a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?