Using the Quickbooks API to Get Customers (with Python examples)
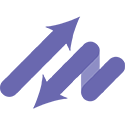
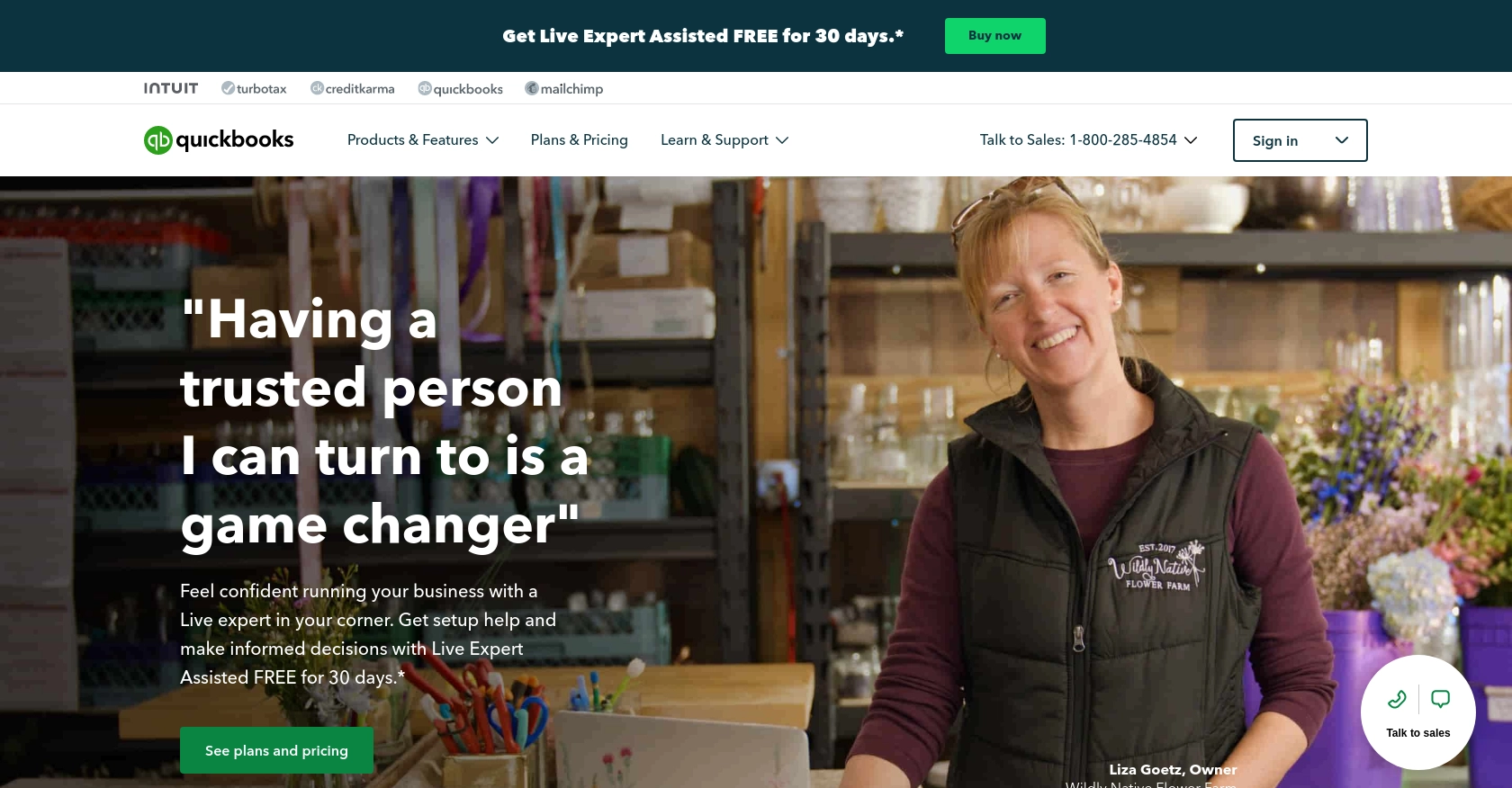
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers comprehensive solutions for managing finances, invoicing, payroll, and more. It is a preferred choice for businesses looking to streamline their financial operations and maintain accurate records.
Integrating with the QuickBooks API allows developers to automate and enhance financial processes by accessing and managing data programmatically. For example, a developer might use the QuickBooks API to retrieve customer information, enabling seamless synchronization of customer data between QuickBooks and other business applications.
This article will guide you through using Python to interact with the QuickBooks API, specifically focusing on retrieving customer data. By following these steps, you can efficiently integrate QuickBooks into your software solutions, enhancing functionality and user experience.
Setting Up Your QuickBooks Test/Sandbox Account
Before you can start interacting with the QuickBooks API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required details to create your developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company to test your API calls:
- Navigate to the "Sandbox" section in your developer dashboard.
- Click on "Create Sandbox" to generate a new sandbox environment.
- Follow the prompts to configure your sandbox company, which will mimic a real QuickBooks account.
Creating a QuickBooks App for OAuth Authentication
QuickBooks uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- Go to the App Management section in your developer dashboard.
- Click on "Create an App" and select "QuickBooks Online and Payments" as the platform.
- Fill in the required app details, such as name and description.
- Once the app is created, navigate to the "Keys & OAuth" tab to find your Client ID and Client Secret.
Configuring OAuth 2.0 Redirect URIs
To complete the OAuth setup, configure the redirect URIs:
- In the "Keys & OAuth" tab, scroll down to the "Redirect URIs" section.
- Add the URIs where you want QuickBooks to redirect users after authentication.
- Ensure these URIs match the ones used in your application to avoid authentication errors.
With your sandbox account and app set up, you're ready to start making API calls to QuickBooks. In the next section, we'll explore how to use Python to retrieve customer data from QuickBooks.
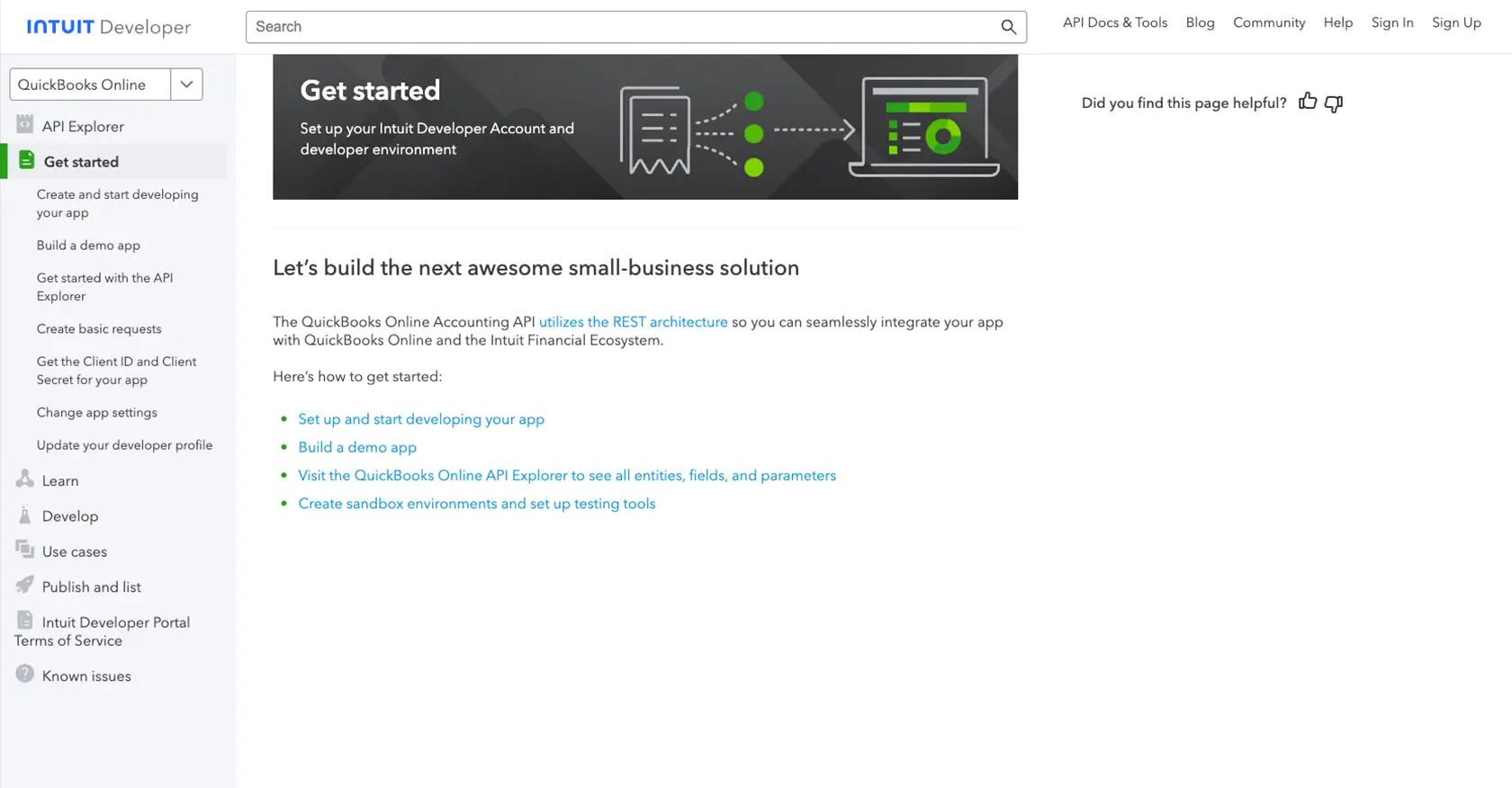
sbb-itb-96038d7
Making API Calls to Retrieve QuickBooks Customer Data Using Python
With your QuickBooks sandbox and app configured, you can now proceed to make API calls to retrieve customer data. This section will guide you through the process using Python, ensuring you have the necessary tools and code to interact with the QuickBooks API effectively.
Setting Up Your Python Environment for QuickBooks API Integration
Before making API calls, ensure your Python environment is set up correctly. You'll need Python 3.11.1 and the necessary libraries to handle HTTP requests and OAuth authentication.
- Ensure Python 3.11.1 is installed on your machine. You can download it from the official Python website.
- Install the required libraries using pip:
pip install requests requests-oauthlib
Authenticating with QuickBooks API Using OAuth 2.0
To authenticate your API requests, you'll use OAuth 2.0. This involves obtaining an access token using your Client ID and Client Secret.
import requests
from requests_oauthlib import OAuth2Session
# Replace with your QuickBooks app credentials
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
redirect_uri = 'Your_Redirect_URI'
# OAuth 2.0 endpoints
authorization_base_url = 'https://appcenter.intuit.com/connect/oauth2'
token_url = 'https://oauth.platform.intuit.com/oauth2/v1/tokens/bearer'
# Create an OAuth2 session
quickbooks = OAuth2Session(client_id, redirect_uri=redirect_uri)
# Redirect user to QuickBooks for authorization
authorization_url, state = quickbooks.authorization_url(authorization_base_url)
print('Please go to {} and authorize access.'.format(authorization_url))
# Get the authorization verifier code from the callback URL
redirect_response = input('Paste the full redirect URL here: ')
quickbooks.fetch_token(token_url, authorization_response=redirect_response, client_secret=client_secret)
# Access token
access_token = quickbooks.token['access_token']
print('Access Token:', access_token)
Retrieving Customer Data from QuickBooks API
Once authenticated, you can make a GET request to the QuickBooks API to retrieve customer data. Here's how you can do it:
# Set the API endpoint for retrieving customers
customer_endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/query'
# Define the query to fetch customers
query = "SELECT * FROM Customer"
# Set the headers with the access token
headers = {
'Authorization': f'Bearer {access_token}',
'Accept': 'application/json'
}
# Make the API request
response = requests.get(customer_endpoint, headers=headers, params={'query': query})
# Check if the request was successful
if response.status_code == 200:
customers = response.json()
print('Customer Data:', customers)
else:
print('Failed to retrieve customers:', response.status_code, response.text)
Replace YOUR_COMPANY_ID
with your actual QuickBooks company ID. The above code sends a query to retrieve all customer data from your QuickBooks sandbox account.
Verifying API Call Success and Handling Errors
After running the code, verify the output to ensure the API call was successful. The customer data should match the records in your QuickBooks sandbox. If the request fails, the response will include an error code and message, which can help diagnose the issue.
For more information on error codes and handling, refer to the QuickBooks API documentation.
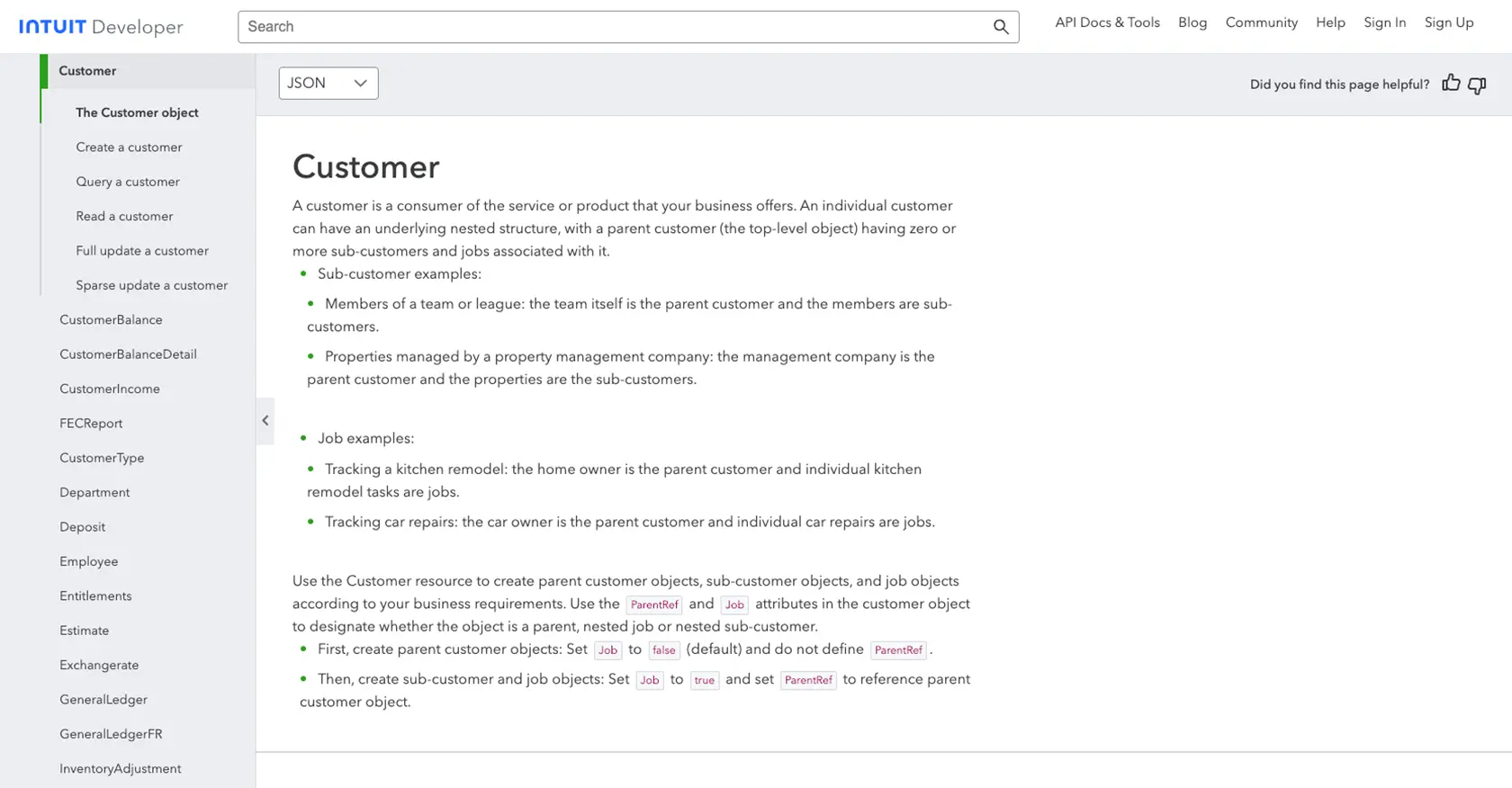
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using Python provides a powerful way to automate and enhance financial processes within your applications. By following the steps outlined in this article, you can efficiently retrieve customer data, enabling seamless synchronization between QuickBooks and other business systems.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to ensure fair usage. Monitor your API calls and implement retry logic to handle rate limit errors gracefully. Refer to the QuickBooks API documentation for specific rate limit details.
- Standardize Data Fields: When integrating customer data, ensure that data fields are standardized across systems to maintain consistency and accuracy.
- Error Handling: Implement robust error handling to manage API errors effectively. Use the error codes and messages provided by QuickBooks to diagnose and resolve issues promptly.
Enhancing Integration Capabilities with Endgrate
While integrating with QuickBooks API can significantly enhance your application's functionality, managing multiple integrations can be complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that connects to multiple platforms, including QuickBooks, allowing you to streamline your integration processes. By leveraging Endgrate, you can focus on your core product development while outsourcing the complexities of integration management.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how it can save you time and resources, providing an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/customer
Ready to get started?