Using the LinkedIn API to Get Ad Form Leads in Javascript
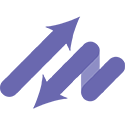
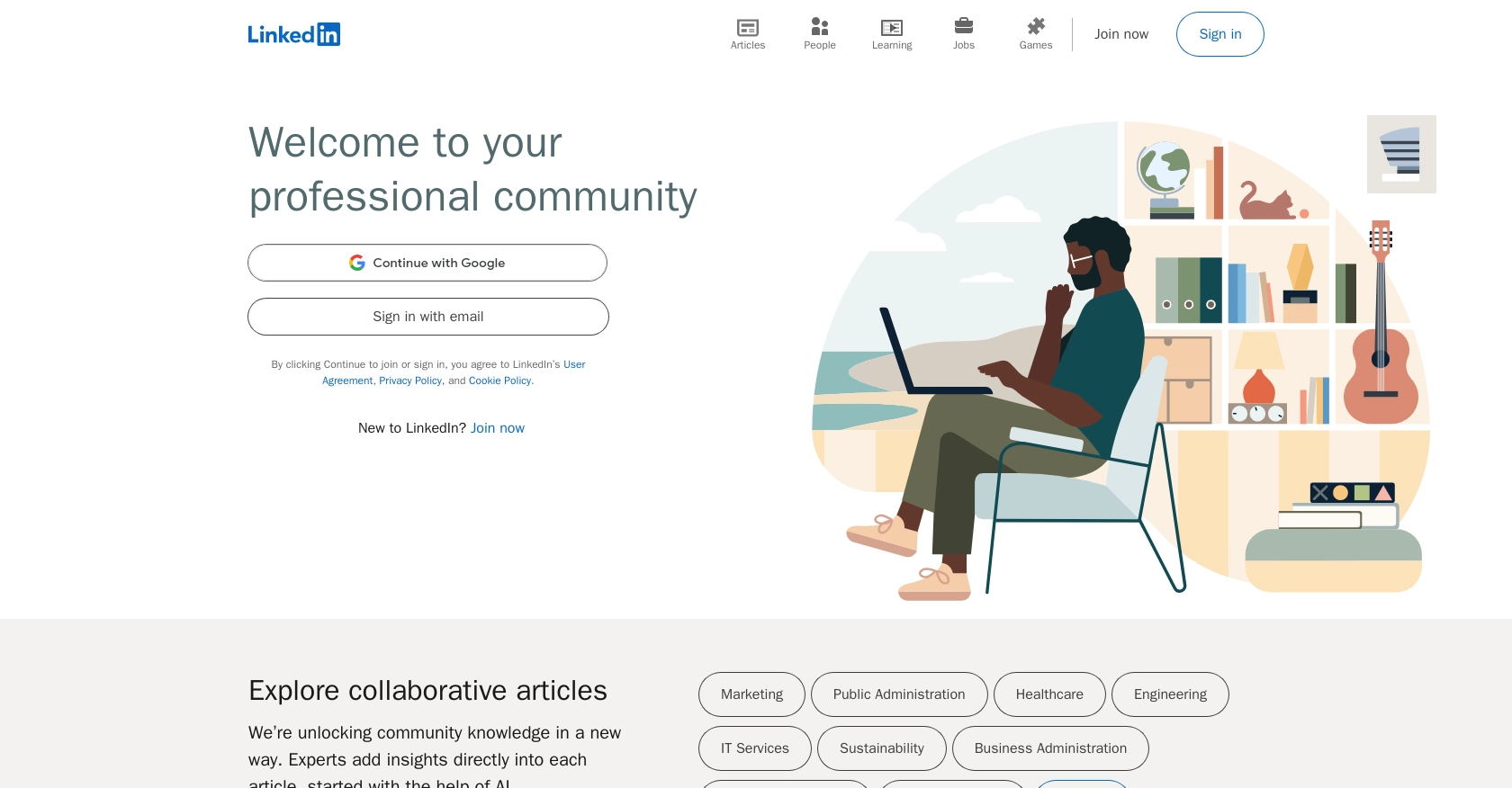
Introduction to LinkedIn API for Ad Form Leads
LinkedIn is a premier professional networking platform that offers a suite of tools for businesses to connect with potential clients and partners. Among its many features, LinkedIn provides robust advertising solutions that allow companies to reach targeted audiences effectively.
Developers may want to integrate with LinkedIn's API to access and manage ad form leads, which can be crucial for marketing automation and CRM systems. For example, using the LinkedIn API, a developer can retrieve lead data from LinkedIn ad forms and seamlessly sync it with their CRM, enabling more personalized and efficient follow-up strategies.
This article will guide you through using JavaScript to interact with LinkedIn's API to fetch ad form leads, providing a step-by-step approach to setting up and executing API calls.
Setting Up Your LinkedIn Developer Account for API Access
Before you can start interacting with LinkedIn's API to fetch ad form leads, you'll need to set up a LinkedIn developer account and create an app. This process will enable you to obtain the necessary credentials for OAuth-based authentication.
Creating a LinkedIn Developer Account
If you don't already have a LinkedIn developer account, follow these steps to create one:
- Visit the LinkedIn Developer Portal.
- Sign in with your LinkedIn credentials or create a new LinkedIn account if you don't have one.
- Once logged in, navigate to the "My Apps" section to start creating a new app.
Creating a LinkedIn App for API Integration
To interact with LinkedIn's API, you need to create an app that will provide you with the client ID and client secret required for OAuth authentication:
- In the "My Apps" section, click on "Create App."
- Fill in the required fields, such as the app name, company, and application description.
- Agree to LinkedIn's API terms and conditions, then click "Create App."
Configuring OAuth Authentication for LinkedIn API
Once your app is created, you need to configure OAuth authentication to securely access LinkedIn's API:
- Navigate to the "Auth" tab within your app settings.
- Note down your client ID and client secret, as these will be used in your API requests.
- Under "OAuth 2.0 Settings," add your redirect URLs. These are the URLs to which LinkedIn will redirect users after they authorize your app.
Requesting Access to LinkedIn's Lead Gen Forms API
To access LinkedIn's Lead Gen Forms API, you must apply for access:
- Go to the "Products" tab in your app settings.
- Add the "Ads Lead Sync API" to your app.
- Complete the access form and submit it for LinkedIn's review.
- Monitor the status of your application in the Developer Portal. If rejected, review the requirements and reapply.
For more detailed information, refer to the LinkedIn Lead Gen API Documentation and the LinkedIn Lead Sync API Access Guide.
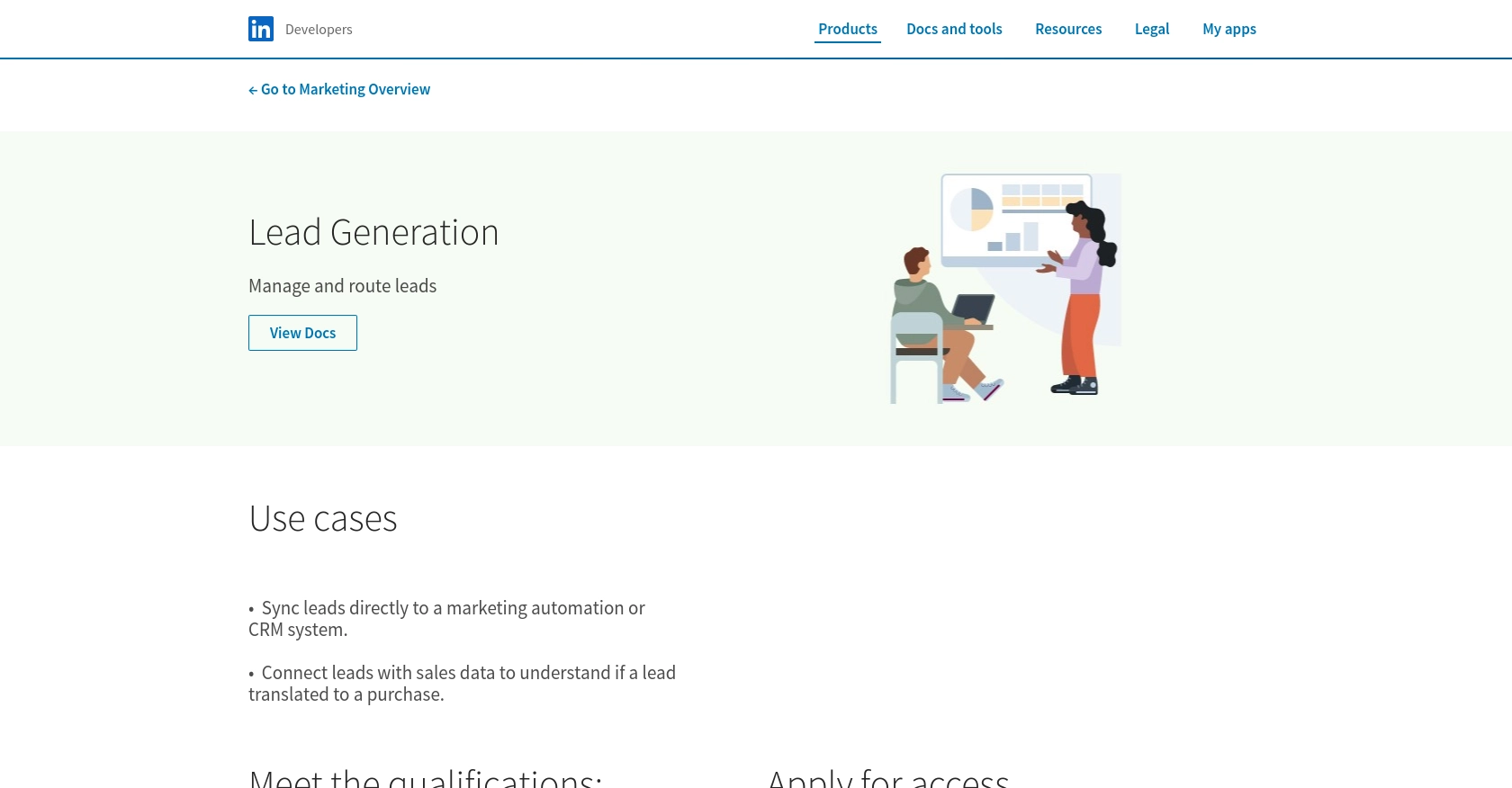
sbb-itb-96038d7
Making API Calls to Fetch LinkedIn Ad Form Leads Using JavaScript
To interact with LinkedIn's API and retrieve ad form leads, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- The
axios
library for making HTTP requests. Install it using the command:
npm install axios
Writing JavaScript Code to Fetch LinkedIn Ad Form Leads
With your environment ready, you can now write the JavaScript code to interact with LinkedIn's API. Here's a sample script to get you started:
const axios = require('axios');
// Set your LinkedIn API credentials
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
const accessToken = 'YOUR_ACCESS_TOKEN';
// Define the API endpoint and headers
const endpoint = 'https://api.linkedin.com/rest/leadFormResponses';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'LinkedIn-Version': '202307',
'X-Restli-Protocol-Version': '2.0.0'
};
// Function to fetch ad form leads
async function fetchAdFormLeads() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Lead Form Responses:', response.data);
} catch (error) {
console.error('Error fetching leads:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchAdFormLeads();
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_ACCESS_TOKEN
with your actual LinkedIn API credentials.
Verifying API Call Success and Handling Errors
After running the script, you should see the lead form responses printed in your console. To verify the success of your API call, check the response data against your LinkedIn sandbox account.
If you encounter errors, LinkedIn's API provides specific error codes. Here are some common ones:
- 401 Unauthorized: Ensure your access token is valid and has the necessary permissions.
- 403 Forbidden: Check if your account has the appropriate roles and permissions.
- 400 Bad Request: Verify that your request parameters and headers are correctly set.
For more detailed error information, refer to the LinkedIn API Error Details documentation.
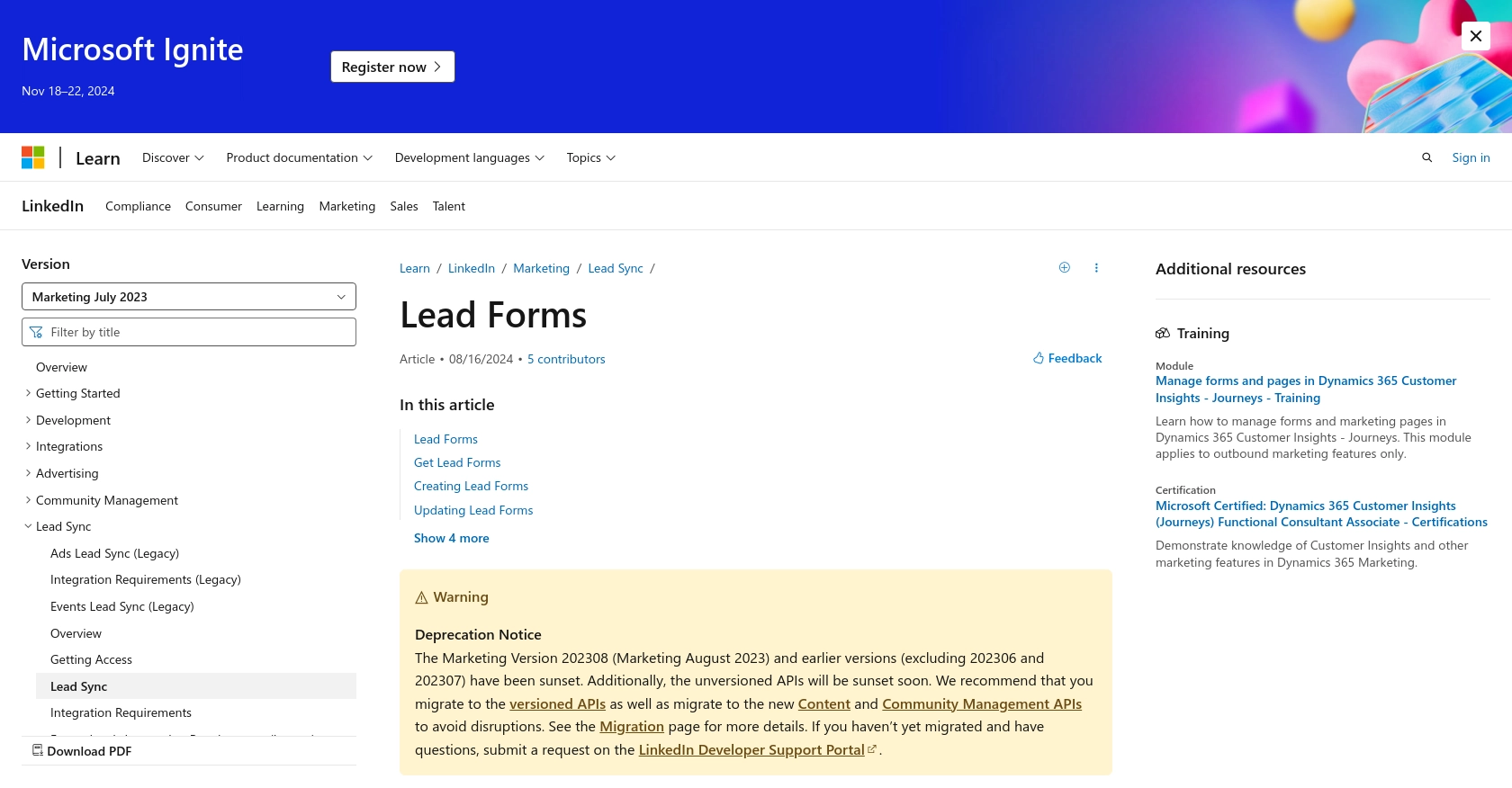
Conclusion and Best Practices for Using LinkedIn API with JavaScript
Integrating with LinkedIn's API to fetch ad form leads using JavaScript can significantly enhance your marketing automation and CRM capabilities. By following the steps outlined in this guide, you can efficiently access and manage lead data, enabling more personalized and effective follow-up strategies.
Best Practices for LinkedIn API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: LinkedIn's API may impose rate limits. Implement logic to handle rate limit responses and retry requests after the specified wait time.
- Data Transformation: Standardize and transform lead data to match your CRM or marketing automation system's schema for seamless integration.
- Monitor API Changes: Stay updated with LinkedIn's API documentation to ensure compatibility with any changes or deprecations.
Enhance Your Integration Strategy with Endgrate
While integrating with LinkedIn's API can be powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including LinkedIn.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development efforts.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a seamless integration experience.
Read More
- https://endgrate.com/provider/linkedin
- https://developer.linkedin.com/product-catalog/marketing/lead-generation
- https://business.linkedin.com/content/dam/me/business/en-us/marketing-solutions/cx/2022/pdf/linkedin-lead-sync-api-access-guide.pdf
- https://learn.microsoft.com/en-us/linkedin/marketing/lead-sync/leadsync?view=li-lms-2023-07&tabs=http
Ready to get started?