Using the Chargebee API to Get Payments in Javascript
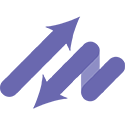
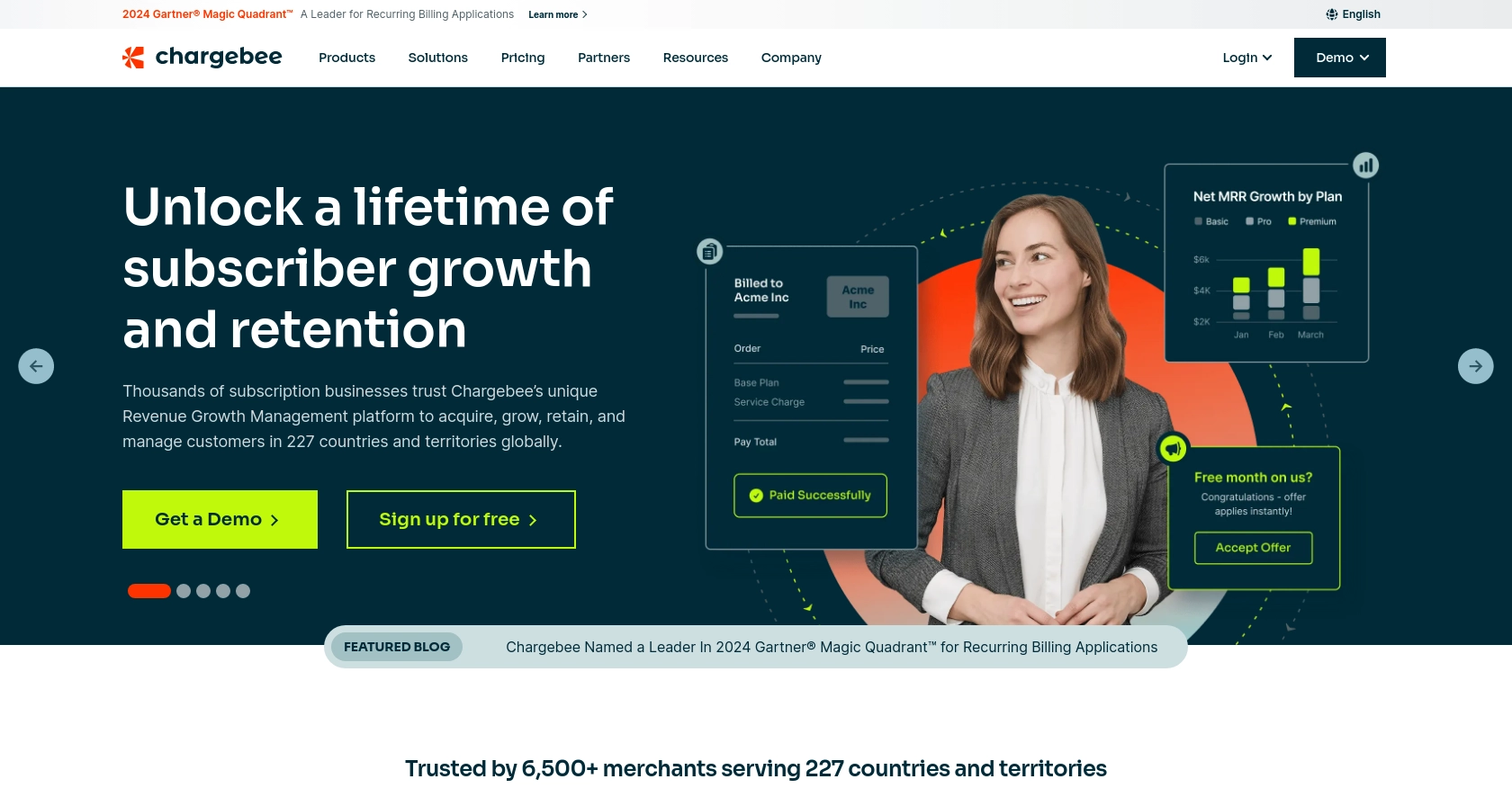
Introduction to Chargebee API for Payment Transactions
Chargebee is a robust subscription billing and revenue management platform designed to streamline billing processes for SaaS businesses. It offers a comprehensive suite of tools to manage subscriptions, invoicing, payments, and more, making it a popular choice for businesses looking to automate their billing workflows.
Integrating with Chargebee's API allows developers to efficiently manage payment transactions, providing seamless access to transaction data. For example, a developer might use the Chargebee API to retrieve payment details and integrate them into a custom dashboard for real-time financial insights.
This article will guide you through using JavaScript to interact with the Chargebee API, focusing on retrieving payment transactions. By the end of this tutorial, you'll be equipped to access and manage payment data, enhancing your application's billing capabilities.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before you can start interacting with the Chargebee API using JavaScript, you'll need to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting live data, ensuring a safe space for development and testing.
Creating a Chargebee Test Account
If you don't already have a Chargebee account, follow these steps to create a test account:
- Visit the Chargebee signup page and register for a free trial.
- Fill in the required details, such as your email address and company information.
- Once registered, you'll receive a confirmation email. Follow the link to verify your account and log in to the Chargebee dashboard.
Generating API Keys for Chargebee
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key, and the password is left empty. Here's how to generate your API keys:
- Log in to your Chargebee account and navigate to the Settings section.
- Under API & Webhooks, select API Keys.
- Click on Create a Key and choose the appropriate permissions for your integration needs.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Note: Ensure you use the API key specific to your test site to avoid any unintended interactions with live data.
Configuring OAuth for Chargebee API Access
For enhanced security and user-specific access, you can configure OAuth for Chargebee API access:
- In the Chargebee dashboard, navigate to Settings > API & Webhooks > OAuth Apps.
- Click on Create an OAuth App and provide the necessary details, such as the app name and redirect URL.
- Once created, you'll receive a client ID and client secret. Use these credentials to authenticate users via OAuth.
With your Chargebee test account and API keys set up, you're now ready to start making API calls to retrieve payment transactions using JavaScript. In the next section, we'll dive into the specifics of making these calls and handling the responses.
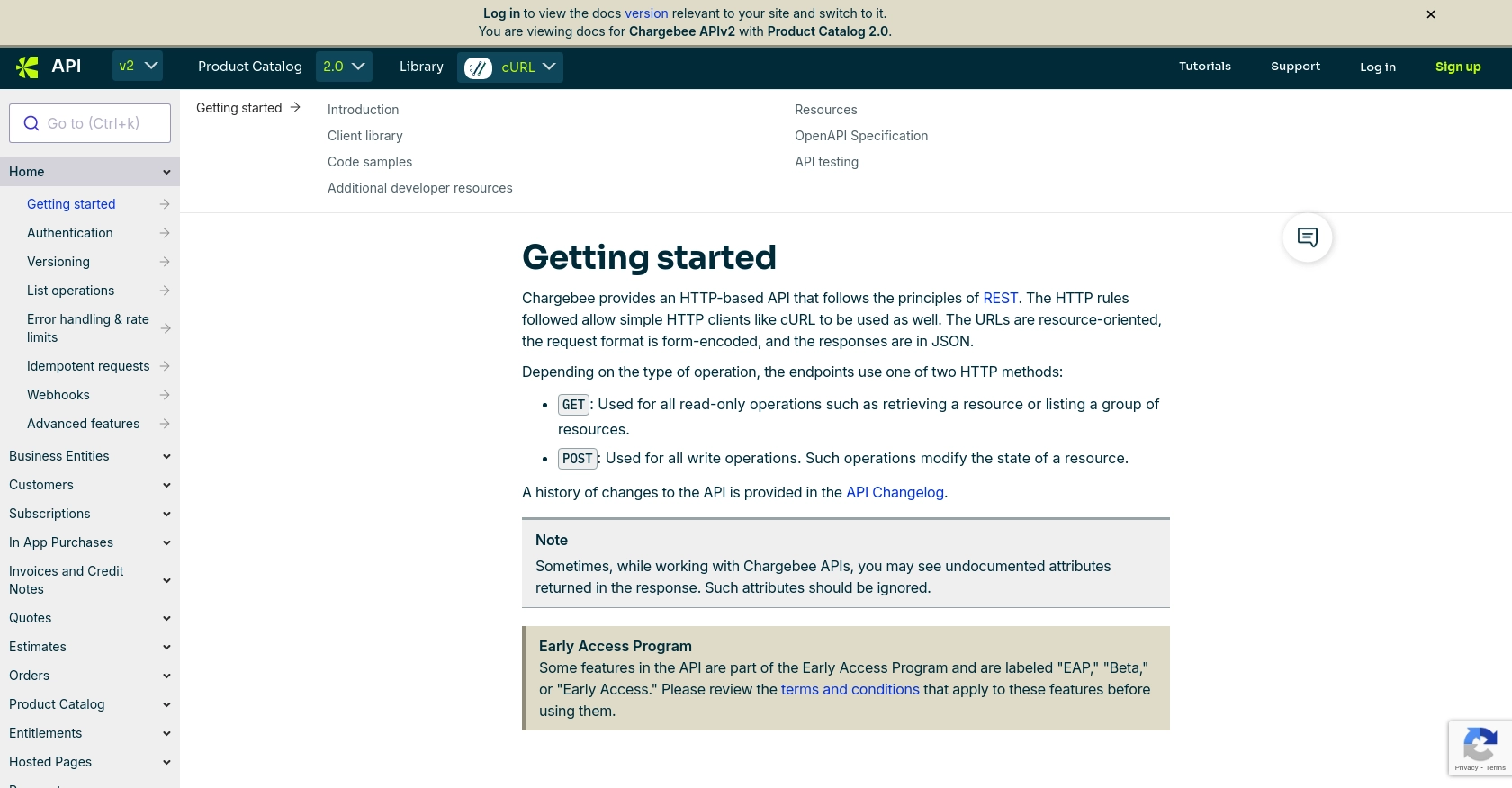
sbb-itb-96038d7
Making API Calls to Retrieve Payment Transactions Using Chargebee API in JavaScript
To interact with the Chargebee API and retrieve payment transactions using JavaScript, you'll need to set up your development environment and write code that makes HTTP requests to the Chargebee API endpoints. This section will guide you through the process, including setting up your JavaScript environment, writing the code to make API calls, and handling the responses.
Setting Up Your JavaScript Environment for Chargebee API Integration
Before making API calls, ensure you have the necessary tools and libraries installed:
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Use npm (Node Package Manager) to install the Axios library, which simplifies making HTTP requests:
npm install axios
Writing JavaScript Code to Retrieve Payment Transactions from Chargebee
With your environment set up, you can now write the JavaScript code to interact with the Chargebee API. The following example demonstrates how to retrieve payment transactions:
const axios = require('axios');
// Set the Chargebee API endpoint and authentication details
const site = 'your-site';
const apiKey = 'your-api-key';
const endpoint = `https://${site}.chargebee.com/api/v2/transactions`;
// Function to retrieve payment transactions
async function getPaymentTransactions() {
try {
const response = await axios.get(endpoint, {
auth: {
username: apiKey,
password: ''
},
params: {
limit: 10,
status: 'success'
}
});
console.log('Payment Transactions:', response.data.list);
} catch (error) {
console.error('Error retrieving transactions:', error.response.data);
}
}
// Call the function to retrieve transactions
getPaymentTransactions();
In this code, we use Axios to make a GET request to the Chargebee API's transactions endpoint. We authenticate using the API key and specify parameters to filter the transactions. The response is logged to the console, displaying the list of payment transactions.
Handling API Responses and Errors When Using Chargebee API
After making the API call, it's essential to handle the responses and any potential errors effectively. The Chargebee API returns data in JSON format, which you can parse and use in your application. If an error occurs, the API will provide an error code and message, which you should log and handle appropriately.
For example, if you encounter a 429 error code, it indicates that you've exceeded the API rate limits. In such cases, implement a retry mechanism with exponential backoff to manage the request load efficiently. For more details on error handling, refer to the Chargebee API error handling documentation.
By following these steps, you can successfully retrieve payment transactions from Chargebee using JavaScript, enabling you to integrate this data into your applications and enhance your billing management capabilities.
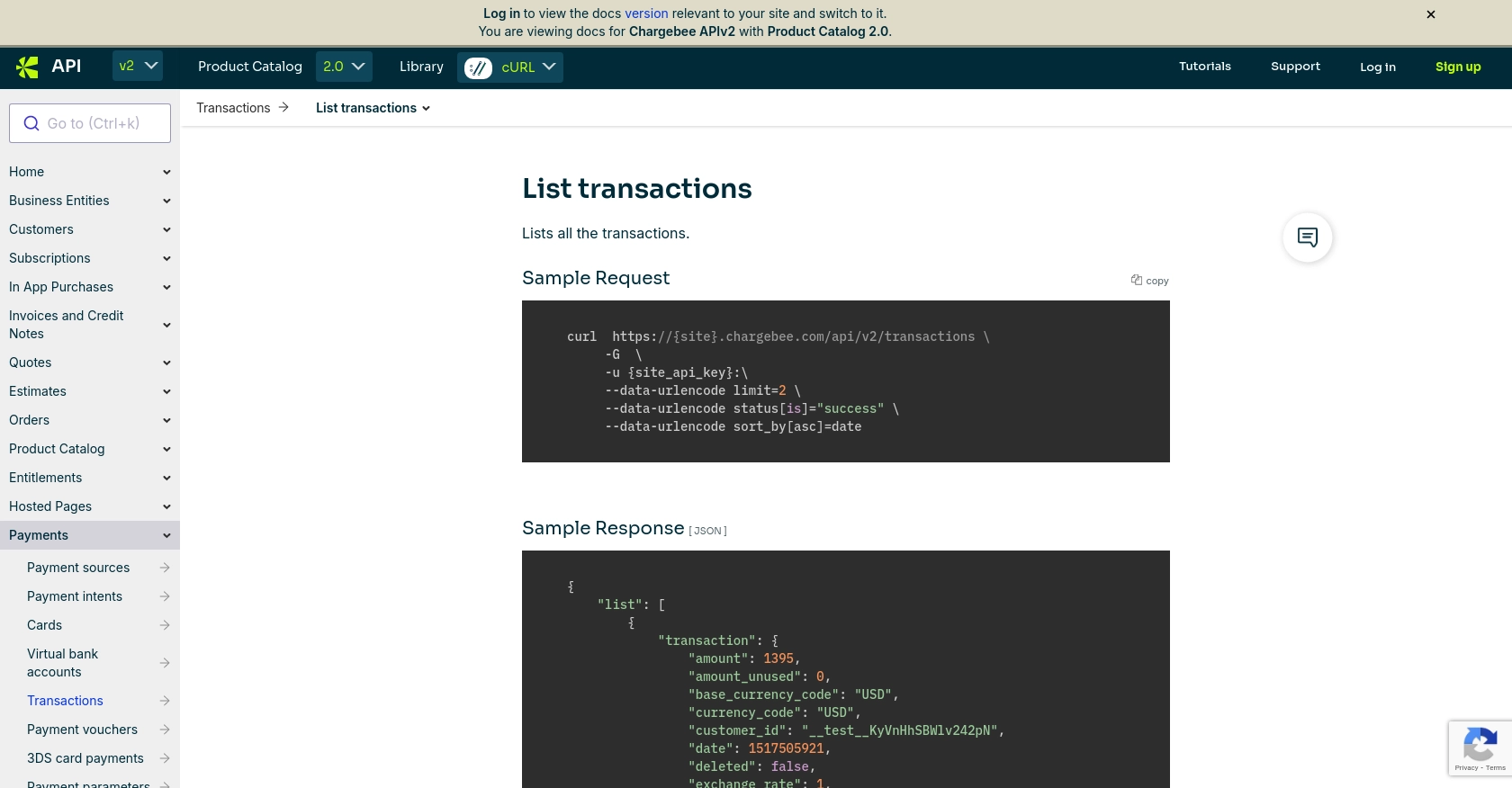
Conclusion and Best Practices for Using Chargebee API in JavaScript
Integrating Chargebee's API into your JavaScript applications can significantly enhance your billing management capabilities by providing seamless access to payment transaction data. By following the steps outlined in this guide, you can efficiently retrieve and manage payment transactions, enabling real-time financial insights and improved workflow automation.
Best Practices for Secure and Efficient Chargebee API Integration
- Secure API Key Management: Store your API keys securely and avoid hardcoding them in your application code. Use environment variables or secure vaults to manage sensitive information.
- Handle API Rate Limits: Chargebee imposes rate limits on API requests. Implement a retry mechanism with exponential backoff to manage request load efficiently and avoid 429 errors. For more details, refer to the Chargebee API rate limits documentation.
- Data Transformation and Standardization: Ensure that the data retrieved from Chargebee is transformed and standardized to match your application's data structures, facilitating seamless integration.
- Monitor API Usage: Regularly monitor your API usage and adjust your integration as needed to optimize performance and avoid exceeding rate limits.
Enhance Your Integration Strategy with Endgrate
While integrating with Chargebee's API offers numerous benefits, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and retention.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a seamless integration experience.
Read More
Ready to get started?