Using the Freshsales API to Create or Update Contacts in PHP
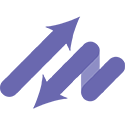
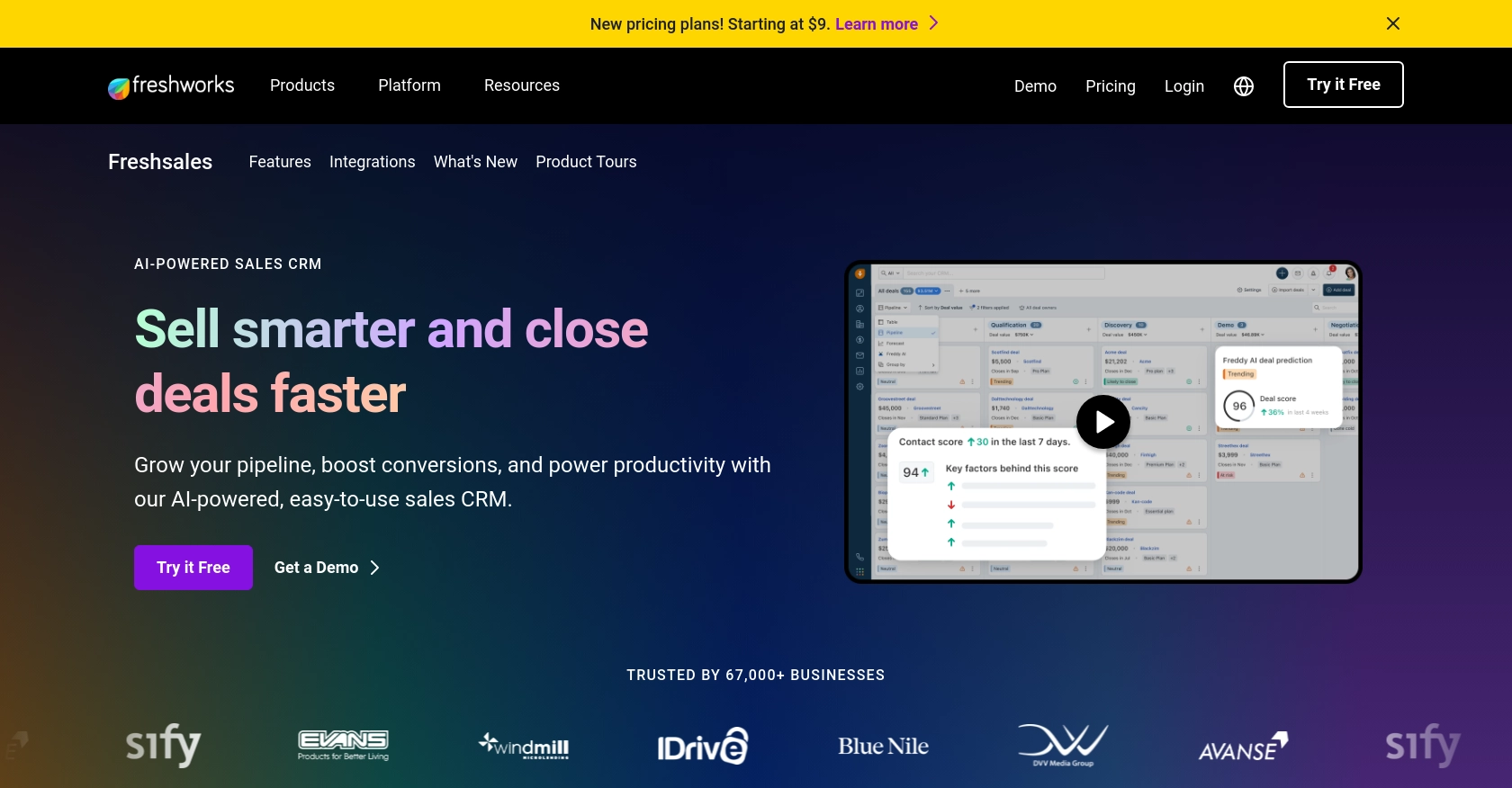
Introduction to Freshsales API
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses streamline their sales processes. With features like lead scoring, email tracking, and built-in phone capabilities, Freshsales enables sales teams to manage their customer interactions more effectively.
Integrating with the Freshsales API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information. For example, a developer might use the Freshsales API to automatically update contact details from an external form submission, ensuring that the sales team always has the most current information.
Setting Up Your Freshsales Test or Sandbox Account
Before you can start integrating with the Freshsales API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Freshsales offers a free trial that you can use to access their API and test your integration.
Creating a Freshsales Account
To begin, visit the Freshsales website and sign up for a free trial. Follow the on-screen instructions to create your account. Once your account is set up, you'll have access to the Freshsales dashboard.
Generating an API Key for Freshsales
Freshsales uses a custom authentication method that requires an API key. Follow these steps to generate your API key:
- Log in to your Freshsales account.
- Navigate to the profile icon in the top right corner and select Settings.
- Under the API Settings section, click on API Key.
- Copy the API key provided. Keep this key secure, as it will be used to authenticate your API requests.
Configuring Your Freshsales App
Once you have your API key, you can configure your application to interact with the Freshsales API. Ensure that your application is set up to include the API key in the headers of your HTTP requests. This will authenticate your requests and allow you to create or update contacts in Freshsales.
With your Freshsales account and API key ready, you can now proceed to make API calls using PHP to create or update contacts. This setup ensures that your integration is both secure and efficient.
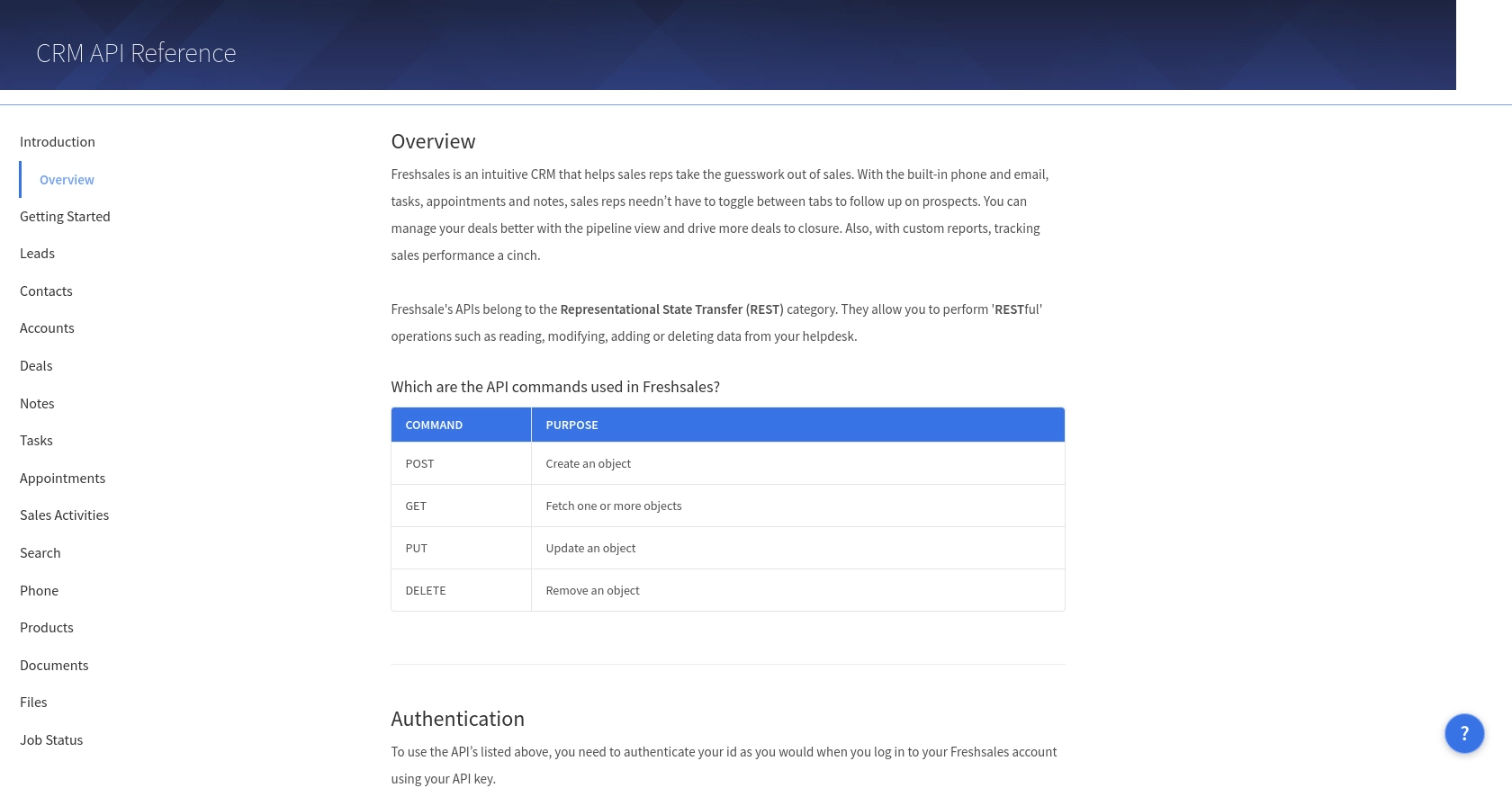
sbb-itb-96038d7
Making API Calls to Freshsales Using PHP
To interact with the Freshsales API and perform operations like creating or updating contacts, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment and making API calls to Freshsales.
Setting Up Your PHP Environment
Before you begin, ensure that you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need the cURL
extension enabled in your PHP configuration, as it will be used to make HTTP requests.
Installing Required PHP Dependencies
To make HTTP requests in PHP, you'll use the cURL
library. Ensure that it's enabled in your php.ini
file:
extension=curl
Restart your web server to apply the changes.
Creating or Updating Contacts with Freshsales API
Now that your environment is set up, you can proceed to make API calls to Freshsales. Below is an example of how to create or update a contact using PHP:
<?php
// Freshsales API endpoint for creating or updating contacts
$url = "https://yourdomain.freshsales.io/api/contacts";
// Your Freshsales API key
$api_key = "Your_API_Key";
// Contact data to be created or updated
$data = [
"contact" => [
"first_name" => "John",
"last_name" => "Doe",
"email" => "john.doe@example.com"
]
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Token token=$api_key",
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display response
$response_data = json_decode($response, true);
echo "Contact ID: " . $response_data['contact']['id'];
}
// Close cURL session
curl_close($ch);
?>
Replace Your_API_Key
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers, and sends a POST request to the Freshsales API with the contact data. If successful, it will return the contact ID.
Verifying API Call Success
After executing the script, you can verify the success of your API call by checking the response for a valid contact ID. Additionally, log in to your Freshsales dashboard to confirm that the contact has been created or updated.
Handling Errors and Error Codes
It's crucial to handle potential errors when making API calls. The Freshsales API may return various HTTP status codes to indicate success or failure. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Implement error handling in your code to manage these responses appropriately.
Best Practices for Using the Freshsales API in PHP
When working with the Freshsales API, it's important to follow best practices to ensure your integration is secure, efficient, and scalable. Here are some key recommendations:
Securely Storing Freshsales API Credentials
Always store your Freshsales API key securely. Avoid hardcoding it directly into your scripts. Instead, use environment variables or a secure vault to manage sensitive information. This helps prevent unauthorized access and protects your data.
Handling Freshsales API Rate Limits
Be mindful of Freshsales API rate limits to avoid service disruptions. Implement logic to handle rate limiting by checking HTTP headers for rate limit information and using exponential backoff strategies to retry requests when limits are reached.
Transforming and Standardizing Data Fields
Ensure that data sent to Freshsales is properly formatted and standardized. This includes validating email addresses, phone numbers, and other contact details before making API calls. Consistent data formatting improves data integrity and usability.
Utilizing Endgrate for Streamlined Integrations
Consider using Endgrate to simplify your integration process. Endgrate allows you to connect with multiple platforms, including Freshsales, through a single API endpoint. This reduces development time and lets you focus on your core product.
By following these best practices, you can create robust and reliable integrations with Freshsales, enhancing your CRM capabilities and improving your team's efficiency.
Read More
Ready to get started?