How to Get Records with the Sugar Sell API in PHP
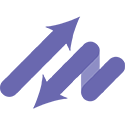
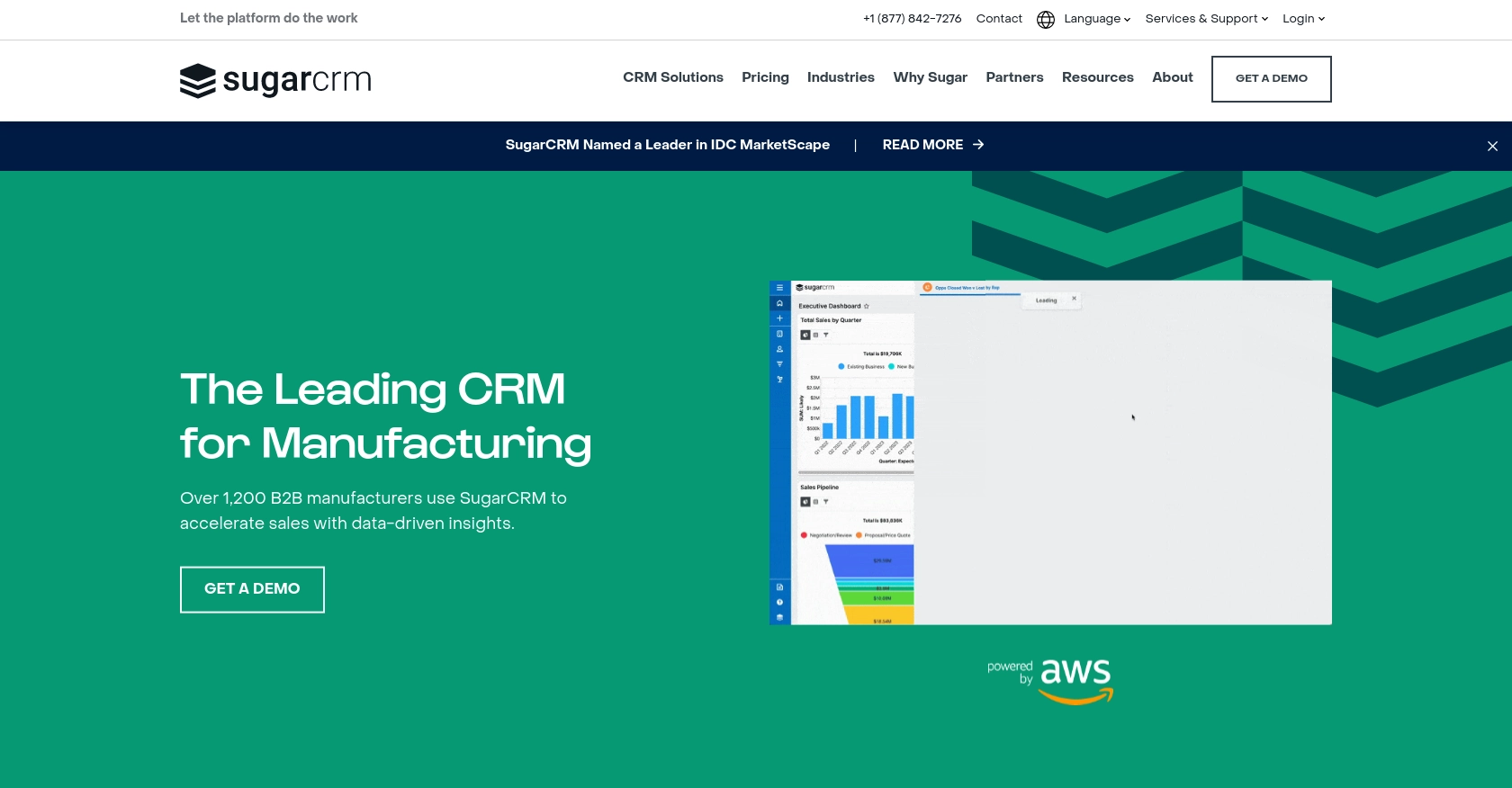
Introduction to Sugar Sell API
Sugar Sell is a robust CRM platform designed to enhance sales processes by providing comprehensive tools for managing customer relationships. Its features include lead management, opportunity tracking, and sales forecasting, making it an ideal choice for businesses looking to streamline their sales operations.
Integrating with the Sugar Sell API allows developers to access and manipulate sales data programmatically, enabling automation and customization of sales workflows. For example, a developer might use the Sugar Sell API to retrieve customer records and integrate them with an external analytics platform, providing deeper insights into sales performance.
Setting Up Your Sugar Sell Test/Sandbox Account
Before you can start interacting with the Sugar Sell API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Sell Sandbox Account
To begin, you'll need to create a Sugar Sell account. If you don't already have one, visit the Sugar Sell website and sign up for a free trial or demo account. This will provide you with access to a sandbox environment where you can test API interactions.
Configuring OAuth2 Authentication for Sugar Sell API
Sugar Sell uses OAuth2 for authentication, which requires setting up an application within your sandbox account. Follow these steps to configure OAuth2:
- Log in to your Sugar Sell sandbox account.
- Navigate to the Admin section and select OAuth Keys.
- Click on Create OAuth Key and fill in the required details.
- Set the Client ID to "sugar" and leave the Client Secret blank for default settings.
- Save the OAuth Key and note down the Client ID and Client Secret.
Generating Access Tokens for API Requests
With your OAuth2 application configured, you can now generate access tokens to authenticate API requests. Use the following PHP code snippet to obtain an access token:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https:///rest/v/oauth2/token");
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode([
"grant_type" => "password",
"client_id" => "sugar",
"client_secret" => "",
"username" => "",
"password" => "",
"platform" => "custom"
]));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$tokenData = json_decode($response, true);
$accessToken = $tokenData['access_token'];
Replace <site_url>
, <version>
, <your_username>
, and <your_password>
with your specific details. This script will return an access_token
that you can use for subsequent API calls.
For more detailed information on authentication, refer to the SugarCRM Authentication Documentation.
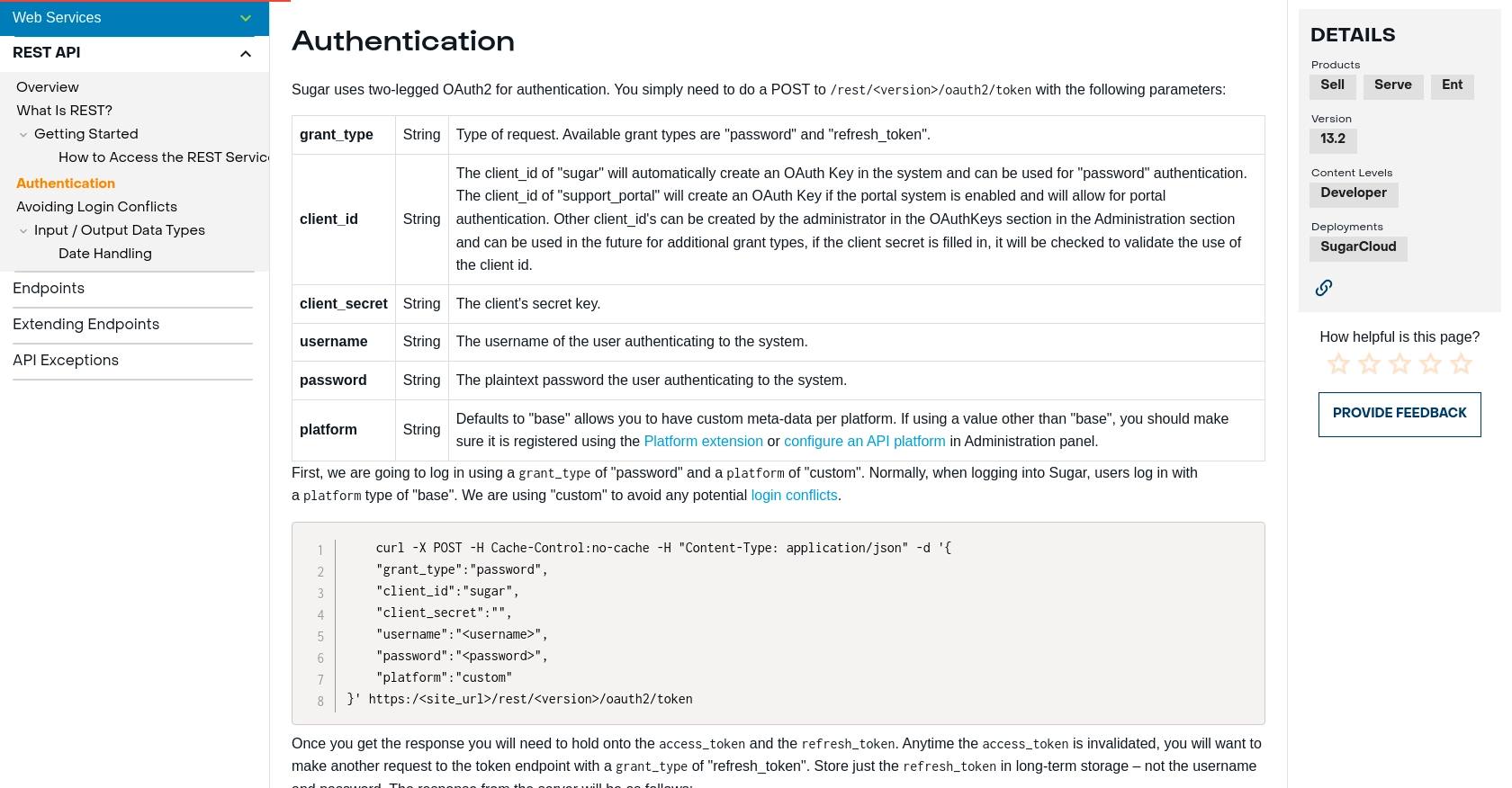
sbb-itb-96038d7
Making API Calls to Retrieve Records from Sugar Sell Using PHP
Once you have your access token, you can start making API calls to interact with Sugar Sell's data. In this section, we'll guide you through the process of retrieving records using PHP.
Prerequisites for PHP API Integration with Sugar Sell
Before proceeding, ensure you have the following installed on your system:
- PHP 7.4 or higher
- cURL extension for PHP
These tools are essential for making HTTP requests and handling JSON responses.
Example Code for Fetching Records from Sugar Sell API
Below is a PHP script to retrieve records from the Sugar Sell API. This example demonstrates how to fetch a list of accounts:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https:///rest/v/Accounts");
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Authorization: Bearer " . $accessToken
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
if (isset($data['records'])) {
foreach ($data['records'] as $record) {
echo "Account Name: " . $record['name'] . "<br>";
}
} else {
echo "Failed to retrieve records.";
}
Replace <site_url>
and <version>
with your specific Sugar Sell instance details. This script will output the names of the accounts retrieved from the API.
Verifying Successful API Requests in Sugar Sell
To ensure your API request was successful, check the response for the expected data. If the request is successful, the data should match the records in your Sugar Sell sandbox account. You can also verify by logging into the Sugar Sell dashboard and checking the records directly.
Handling Errors and Common Error Codes in Sugar Sell API
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 401 Unauthorized: Indicates an issue with authentication. Ensure your access token is valid.
- 404 Not Found: The requested resource does not exist. Verify the endpoint URL.
- 500 Internal Server Error: A server-side error. Check the Sugar Sell status or try again later.
Implement error handling in your code to manage these scenarios gracefully and provide meaningful feedback to users.
Best Practices for Using Sugar Sell API in PHP
When working with the Sugar Sell API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials, such as access tokens, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Sell API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay.
- Standardize Data Fields: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Streamline Your Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API solution that simplifies the process, allowing you to focus on your core product. With Endgrate, you can:
- Save time and resources by outsourcing integration development.
- Build once for each use case, rather than multiple times for different platforms.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sugarsell
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/#Authentication
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/endpoints/
Ready to get started?