Using the Zoho Books API to Get Accounts in Python
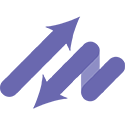
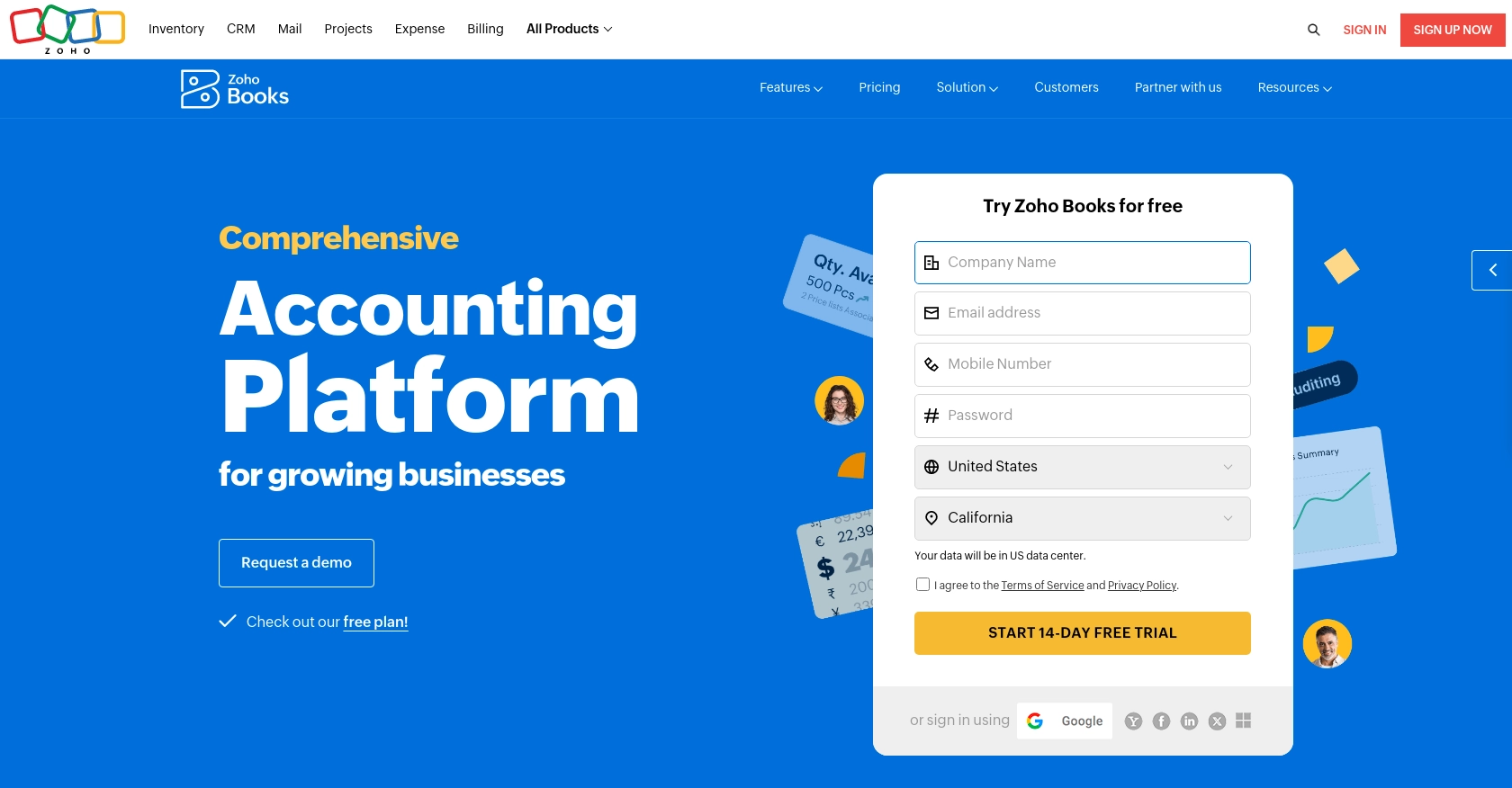
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with Zoho Books API allows developers to automate and streamline accounting processes. For example, you can use the API to retrieve account details, enabling seamless financial data integration with other business applications. This can be particularly useful for generating financial reports or syncing data with CRM systems.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start using the Zoho Books API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Zoho Books offers a free trial that you can use to access the API and test its features.
Creating a Zoho Books Account
To begin, visit the Zoho Books signup page and create a free account. Follow the instructions to complete the registration process. Once your account is set up, you will have access to the Zoho Books dashboard.
Registering a New Client in Zoho's Developer Console
Zoho Books uses OAuth 2.0 for authentication, which requires you to register your application to obtain a Client ID and Client Secret. Follow these steps to register your application:
- Go to the Zoho Developer Console.
- Click on Add Client ID.
- Fill in the required details, such as the client name, redirect URI, and authorized domains.
- Submit the form to receive your Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Generating OAuth Tokens for Zoho Books API
With your Client ID and Client Secret, you can now generate OAuth tokens to authenticate API requests. Follow these steps:
- Redirect users to the following authorization URL with the necessary parameters:
- Upon user consent, Zoho will redirect to your specified URI with a
code
parameter. - Exchange this code for an access token by making a POST request:
- Store the
access_token
andrefresh_token
securely for future API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
"code": "YOUR_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"redirect_uri": "YOUR_REDIRECT_URI",
"grant_type": "authorization_code"
}
response = requests.post(url, data=payload)
tokens = response.json()
print(tokens)
For more details on OAuth setup, refer to the Zoho Books OAuth documentation.
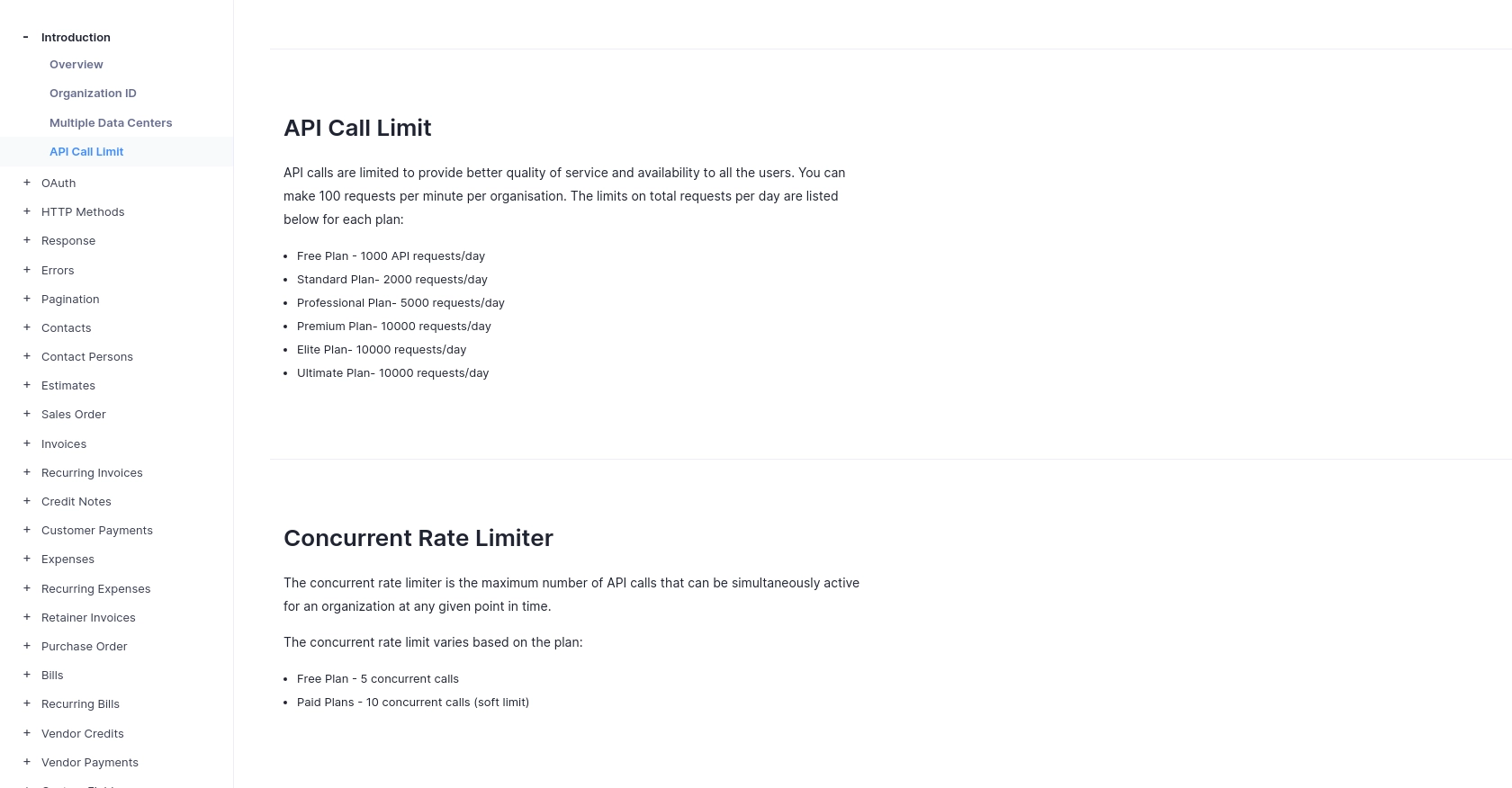
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Zoho Books Using Python
To interact with the Zoho Books API and retrieve account details, you'll need to make HTTP requests using Python. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for Zoho Books API
Ensure you have Python 3.11.1 installed on your machine. You'll also need the requests
library to make HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Accounts from Zoho Books
Create a new Python file named get_zoho_books_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://www.zohoapis.com/books/v3/chartofaccounts?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the accounts and print their information
for account in data.get("chartofaccounts", []):
print(f"Account Name: {account['account_name']}, Account Type: {account['account_type']}")
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token.
Understanding the API Response and Error Handling
When you run the script, it will output the list of accounts from Zoho Books. If the request fails, it will print an error message. Common HTTP status codes you might encounter include:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid access token. Ensure your token is correct.
- 429 Rate Limit Exceeded: You've exceeded the API call limit. Zoho Books allows 100 requests per minute per organization. For more details, refer to the API call limit documentation.
Verifying the Retrieved Accounts in Zoho Books
To verify the accounts retrieved by your script, log in to your Zoho Books account and navigate to the Chart of Accounts section. The accounts listed in your script's output should match those in the Zoho Books dashboard.
For more information on error codes, refer to the Zoho Books error documentation.
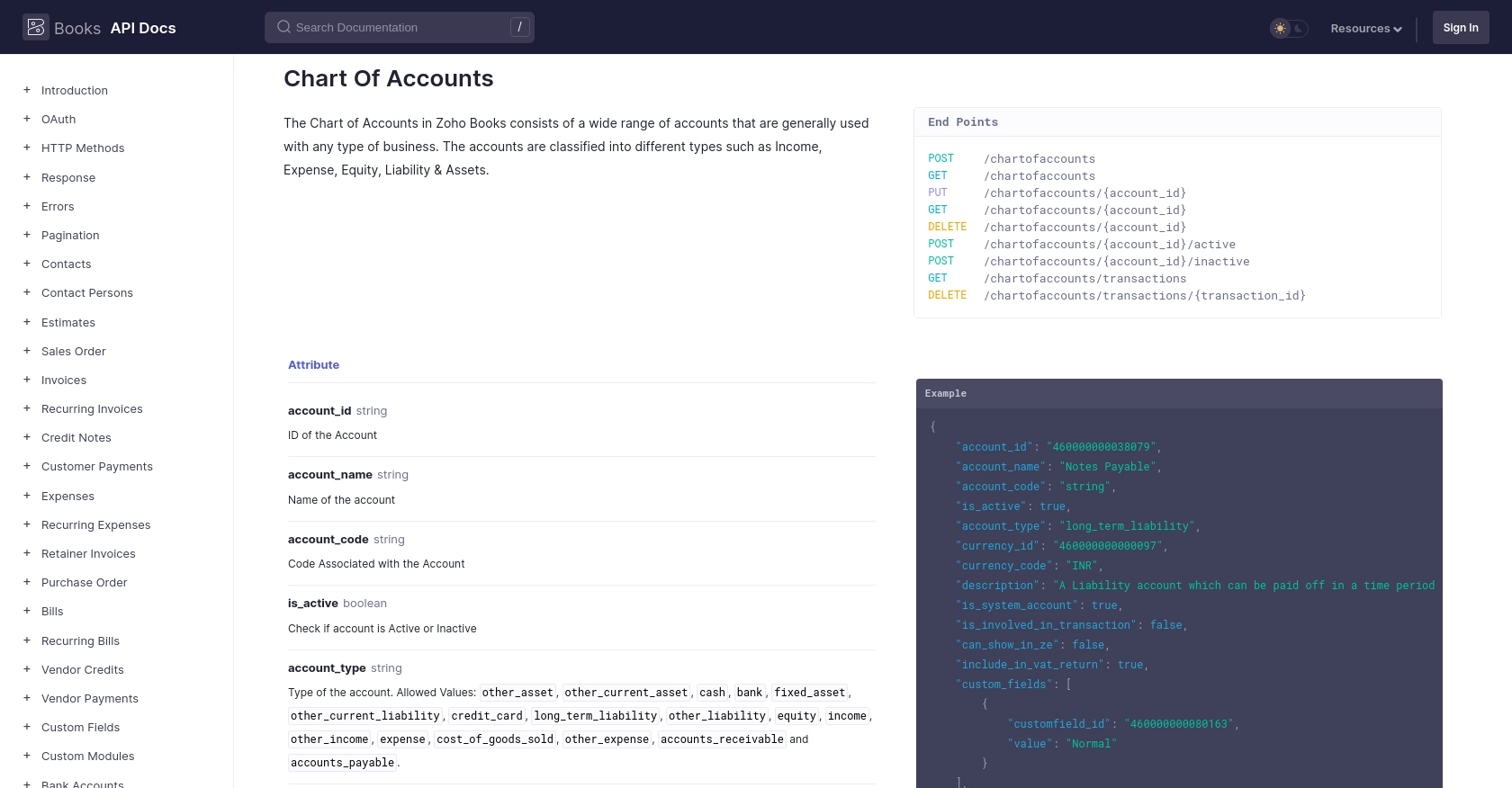
Conclusion and Best Practices for Using Zoho Books API in Python
Integrating with the Zoho Books API using Python can significantly enhance your financial data management by automating tasks and ensuring seamless data flow between your accounting software and other business applications. By following the steps outlined in this guide, you can efficiently retrieve account details and handle API responses.
Best Practices for Secure and Efficient API Integration with Zoho Books
- Secure Storage of Credentials: Always store your OAuth tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handling Rate Limits: Zoho Books enforces a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors and retry requests after a delay. For more details, refer to the API call limit documentation.
- Error Handling: Implement robust error handling to manage different HTTP status codes. This ensures your application can gracefully handle issues like expired tokens or network errors.
- Data Transformation and Standardization: When integrating data from Zoho Books with other systems, ensure consistent data formats and structures to avoid discrepancies.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Zoho Books. By using Endgrate, you can save time and resources, focusing on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can help you build efficient, scalable integrations by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?