How to Get People with the Copper API in Javascript
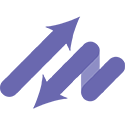
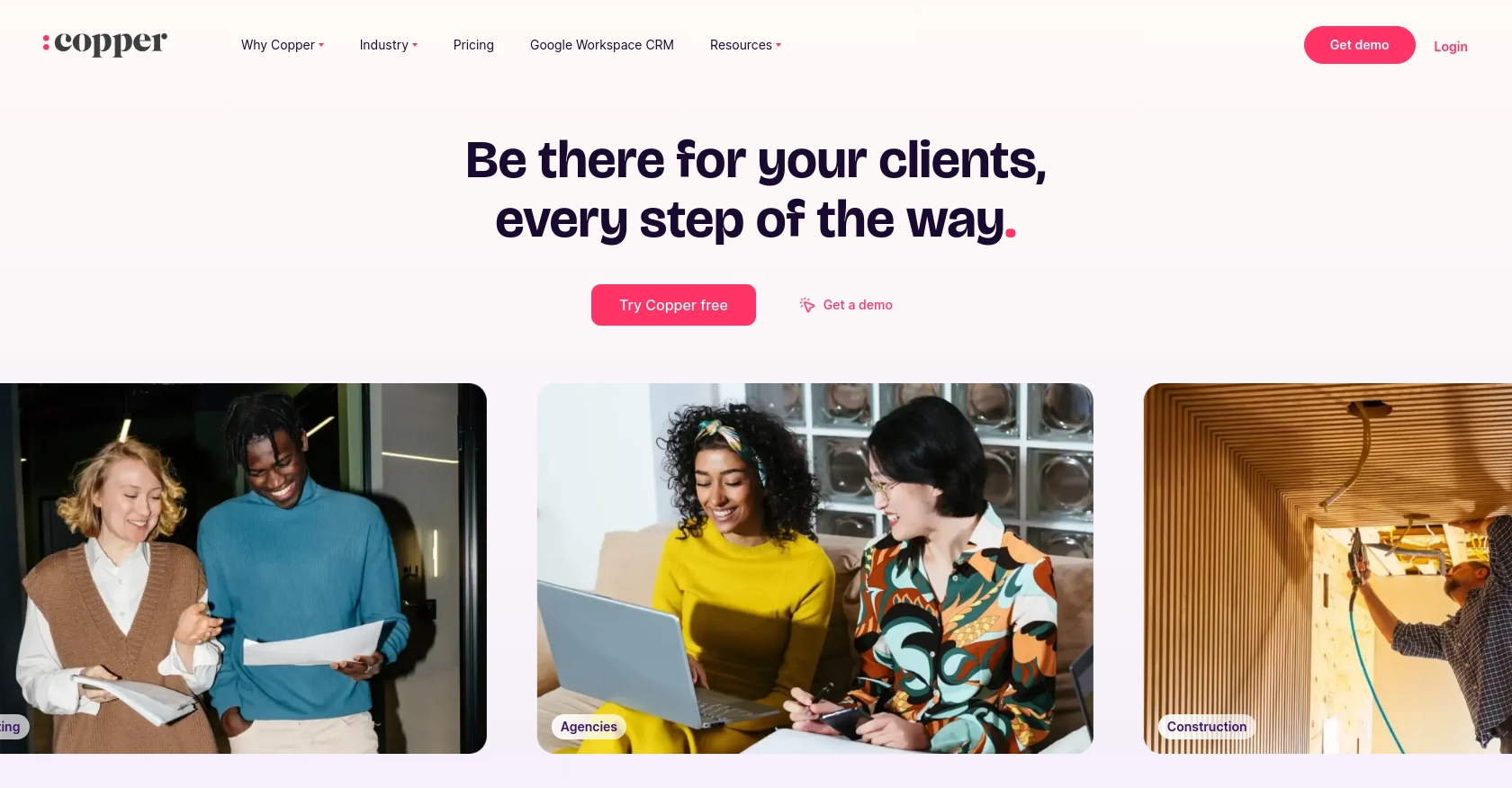
Introduction to Copper API for Seamless Integration
Copper is a robust platform that offers a comprehensive API designed to facilitate seamless integration with various applications. Known for its powerful features, Copper enables businesses to manage their operations efficiently, particularly in the realm of finance and cryptocurrency management.
Developers may want to integrate with the Copper API to access and manage financial data, such as retrieving information about people or entities involved in transactions. For example, a developer could use the Copper API to fetch details about individuals associated with specific financial activities, enabling more personalized financial services or enhanced data analysis.
Setting Up Your Copper API Test or Sandbox Account
Before you can start interacting with the Copper API, it's essential to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data.
Creating a Copper Sandbox Account
To begin, you'll need to create a Copper sandbox account. Follow these steps:
- Visit the Copper website and sign up for a developer account.
- Once registered, navigate to the developer section and select the option to create a sandbox environment.
- Fill in the necessary details and submit your request. You will receive credentials for accessing the sandbox environment.
Generating Copper API Keys for Authentication
The Copper API uses a custom authentication method that requires an API key and secret. Here's how to generate them:
- Log in to your Copper sandbox account.
- Navigate to the API settings section.
- Click on "Create API Key" and provide a name for your key.
- Once generated, note down the API key and secret. These will be used for authenticating your API requests.
Configuring OAuth for Copper API Access
Although Copper primarily uses API keys, you may need to configure OAuth for specific integrations. Follow these steps:
- In your Copper sandbox account, go to the OAuth settings.
- Create a new OAuth application by providing the necessary details such as redirect URI and scopes.
- Once created, you'll receive a client ID and client secret. Keep these credentials secure.
Testing Your Copper API Setup
With your sandbox account and API keys ready, you can start testing API calls. Use tools like Postman or cURL to send requests and verify responses. Ensure that your API key and secret are included in the request headers for successful authentication.
By setting up a Copper sandbox account, you can explore the API's capabilities and integrate its features into your applications with confidence.
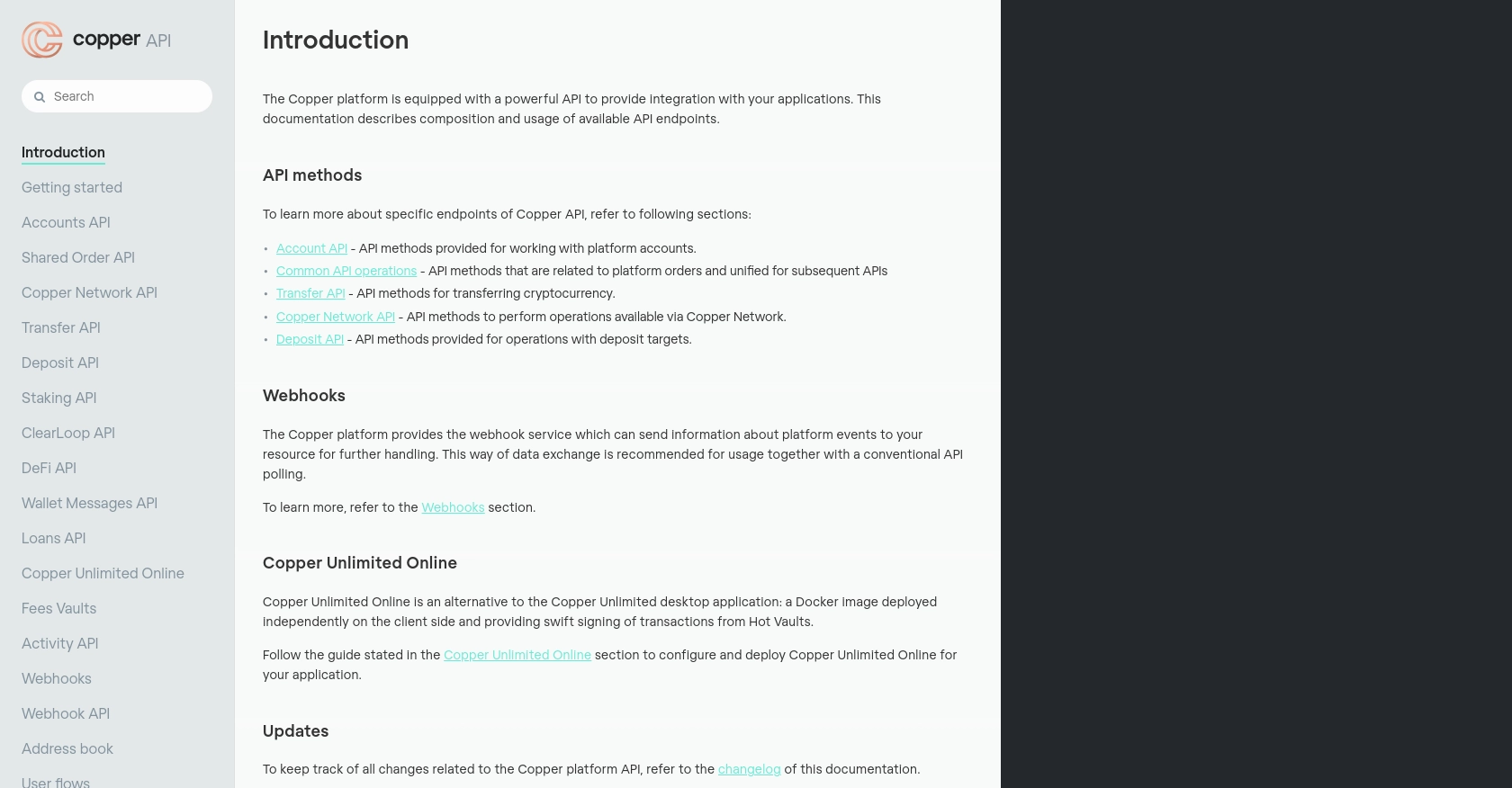
sbb-itb-96038d7
Making API Calls to Retrieve People Data with Copper API in JavaScript
To interact with the Copper API using JavaScript, you need to ensure that your environment is set up correctly. This involves using the right version of Node.js and installing necessary dependencies. Follow these steps to make API calls to retrieve people data from Copper.
Setting Up Your JavaScript Environment for Copper API
Before making API calls, ensure you have Node.js installed. You can download it from the official Node.js website. Once installed, verify the installation by running:
node -v
Next, create a new project directory and initialize it:
mkdir copper-api-integration
cd copper-api-integration
npm init -y
Installing Required Dependencies for Copper API Integration
To make HTTP requests, you'll need the axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch People Data from Copper API
Create a new JavaScript file named getPeople.js
and add the following code:
const axios = require('axios');
// Set your Copper API credentials
const apiKey = 'YOUR_API_KEY';
const apiSecret = 'YOUR_API_SECRET';
// Configure the API endpoint and headers
const endpoint = 'https://api.copper.co/platform/people';
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': 'YOUR_SIGNATURE',
'X-Timestamp': Date.now().toString(),
'Content-Type': 'application/json'
};
// Function to fetch people data
async function getPeople() {
try {
const response = await axios.get(endpoint, { headers });
console.log('People Data:', response.data);
} catch (error) {
console.error('Error fetching people data:', error.response.data);
}
}
// Execute the function
getPeople();
Replace YOUR_API_KEY
, YOUR_API_SECRET
, and YOUR_SIGNATURE
with your actual Copper API credentials.
Running Your JavaScript Code to Retrieve Copper People Data
Execute the script using Node.js:
node getPeople.js
If successful, you should see the people data logged in your console. If there are errors, ensure your API credentials are correct and that your request headers are properly configured.
Handling Errors and Verifying API Call Success
To verify that your request succeeded, check the response status code. A status code of 200 indicates success. If you encounter errors, refer to the Copper API documentation for error codes and troubleshooting tips. Common error codes include:
- 401 Unauthorized: Check your API key and signature.
- 429 Too Many Requests: You have exceeded the rate limit of 30,000 requests per 5 minutes. Wait before retrying.
For more details on error handling, visit the Copper API documentation.
Best Practices for Copper API Integration and Error Handling
Successfully integrating with the Copper API requires adherence to best practices for security and efficiency. Here are some key recommendations:
- Secure Storage of Credentials: Always store your API keys and secrets securely. Use environment variables or secure vaults to prevent unauthorized access.
- Rate Limiting Awareness: Be mindful of Copper's rate limit of 30,000 requests per 5 minutes. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when interacting with the API. This helps in maintaining data integrity across different systems.
- Error Logging and Monitoring: Implement comprehensive logging for API requests and responses. This aids in troubleshooting and ensures quick resolution of issues.
Enhancing Integration Efficiency with Endgrate
While integrating with Copper API can be straightforward, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Copper.
With Endgrate, you can:
- Streamline Integration Processes: Build once for each use case instead of multiple times for different integrations.
- Focus on Core Product Development: Save time and resources by outsourcing integrations, allowing your team to concentrate on core functionalities.
- Deliver a Seamless Experience: Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?