How to Get Items with the Sap Business One API in PHP
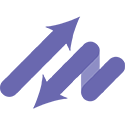
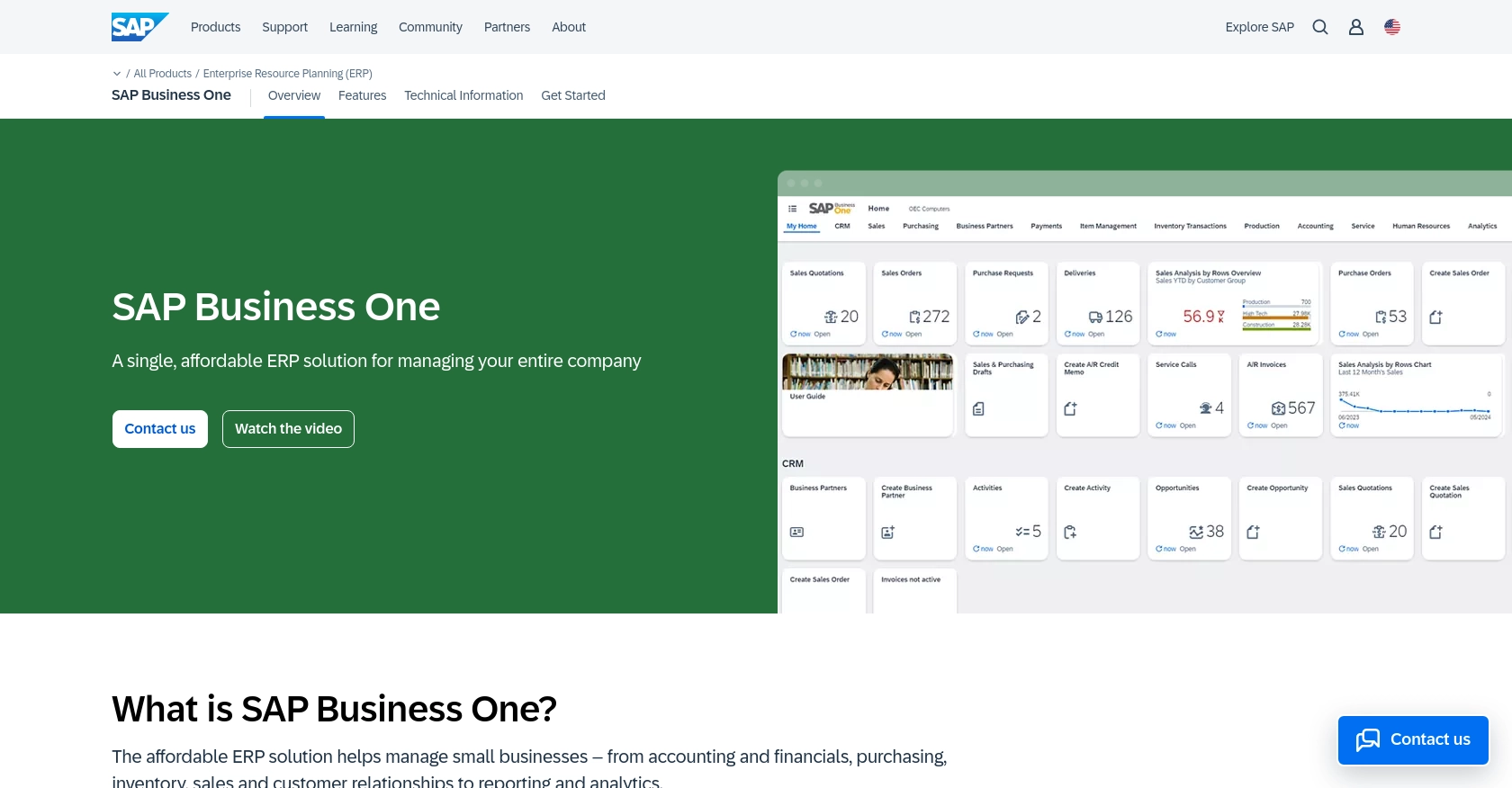
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Integrating with the SAP Business One API allows developers to streamline business operations by automating data retrieval and management tasks. For example, a developer might use the API to fetch item details from the inventory, enabling real-time updates and efficient stock management within a custom application.
Setting Up a Test or Sandbox Account for SAP Business One API Integration
Before you can start interacting with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you will need access to a SAP Business One environment. If your organization already uses SAP Business One, you can request access to a sandbox environment from your system administrator. If not, you may need to contact a SAP partner to set up a trial or demo account.
Configuring API Access in SAP Business One
Once you have access to a sandbox account, follow these steps to configure API access:
- Log in to your SAP Business One sandbox environment.
- Navigate to the Service Layer settings. This is where you will manage API access.
- Create a new API user with the necessary permissions to access the items data. Ensure that this user has read access to the inventory module.
- Generate the API credentials, including the client ID and client secret, which you will use for authentication.
Authenticating with Custom Authentication
SAP Business One uses a custom authentication method for API access. Here’s how to authenticate:
- Use the generated client ID and client secret to authenticate your API requests.
- Refer to the SAP Business One Service Layer documentation for detailed instructions on setting up authentication headers.
With your sandbox account and API access configured, you are now ready to start making API calls to retrieve item data from SAP Business One.
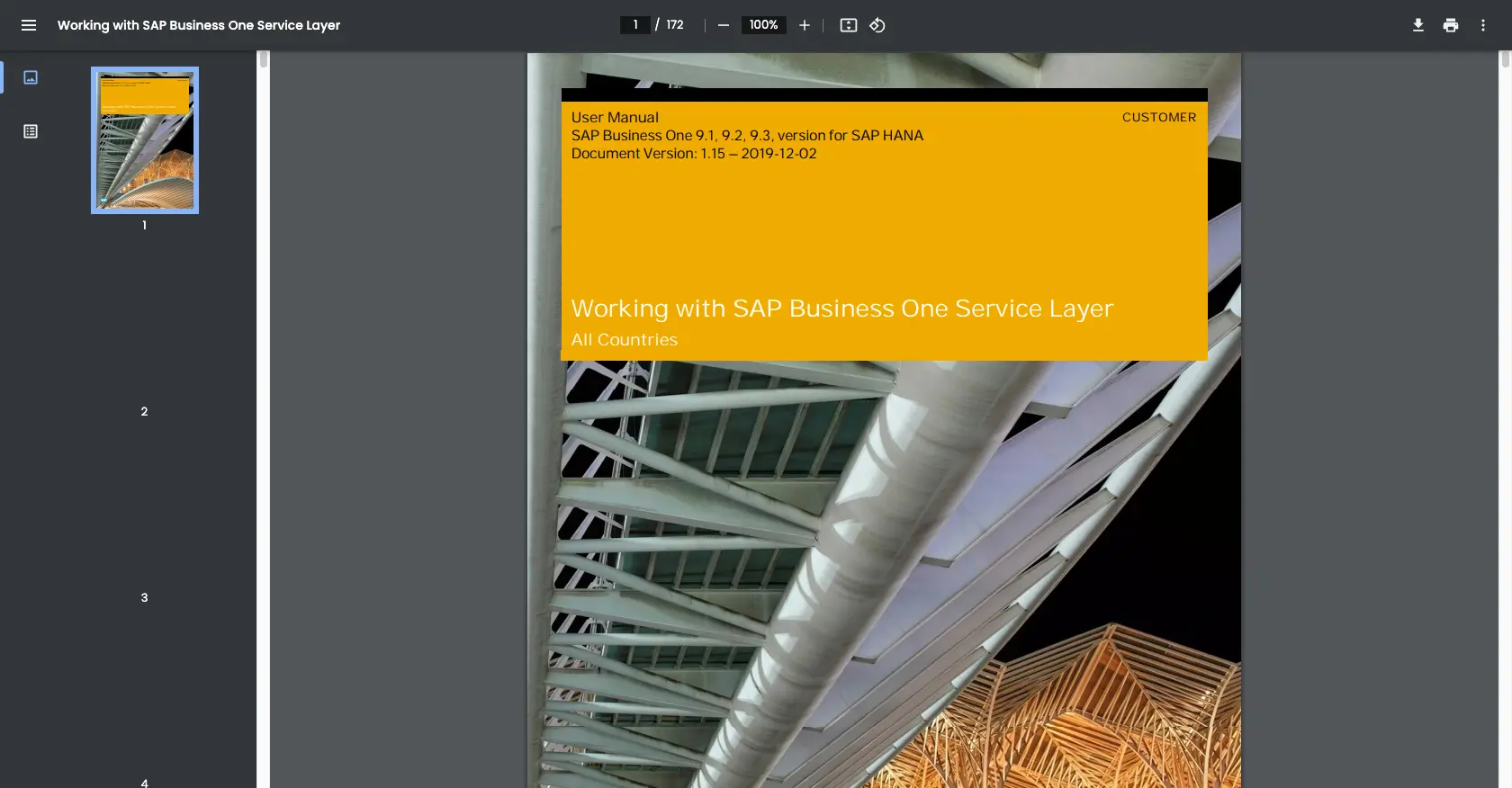
sbb-itb-96038d7
Making API Calls to Retrieve Items from SAP Business One Using PHP
To interact with the SAP Business One API and retrieve item data, you'll need to set up your PHP environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have the right tools and code to successfully fetch items from your SAP Business One sandbox account.
Setting Up Your PHP Environment for SAP Business One API Integration
Before you begin coding, ensure that your development environment is ready:
- Install PHP version 7.4 or higher.
- Ensure you have Composer installed for managing dependencies.
- Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. You can do this by running the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Items from SAP Business One
With your environment set up, you can now write the PHP code to make an API call to SAP Business One and retrieve item data. Create a new PHP file, for example, get_items.php
, and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$endpoint = 'https://your-sap-business-one-url.com/b1s/v1/Items';
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
// Set up the headers for authentication
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Basic ' . base64_encode("$clientId:$clientSecret")
];
try {
// Make the GET request to the SAP Business One API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Check if the request was successful
if ($response->getStatusCode() == 200) {
$items = json_decode($response->getBody(), true);
foreach ($items['value'] as $item) {
echo 'Item Code: ' . $item['ItemCode'] . ' - Item Name: ' . $item['ItemName'] . "<br>";
}
} else {
echo 'Failed to retrieve items. Status code: ' . $response->getStatusCode();
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Client_ID
and Your_Client_Secret
with the credentials you generated during the setup process. This code uses Guzzle to send a GET request to the SAP Business One API, retrieves the items, and prints their details.
Verifying API Call Success in SAP Business One Sandbox
After running your PHP script, you should see a list of items printed on your screen. To verify the success of your API call, log in to your SAP Business One sandbox account and check the inventory module to ensure the items match the data retrieved by your script.
Handling Errors and Troubleshooting
When working with API calls, it's essential to handle potential errors. The code above includes basic error handling, but you can expand it by checking for specific error codes and messages. Refer to the SAP Business One Service Layer documentation for more details on error codes and troubleshooting tips.
Conclusion and Best Practices for Using SAP Business One API with PHP
Integrating with the SAP Business One API using PHP can significantly enhance your business operations by automating data retrieval and management tasks. By following the steps outlined in this guide, you can efficiently fetch item data from your SAP Business One environment and incorporate it into your custom applications.
Best Practices for Secure and Efficient SAP Business One API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to keep your client ID and client secret safe.
- Handle Rate Limiting: Be mindful of any rate limits imposed by SAP Business One. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application’s data structures.
- Error Handling: Implement comprehensive error handling to manage API call failures. Log errors for troubleshooting and refer to the SAP Business One Service Layer documentation for detailed error code information.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including SAP Business One. This allows you to build once for each use case and streamline your integration processes.
By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?