How to Create Deals with the PipelineCRM API in Python
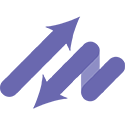
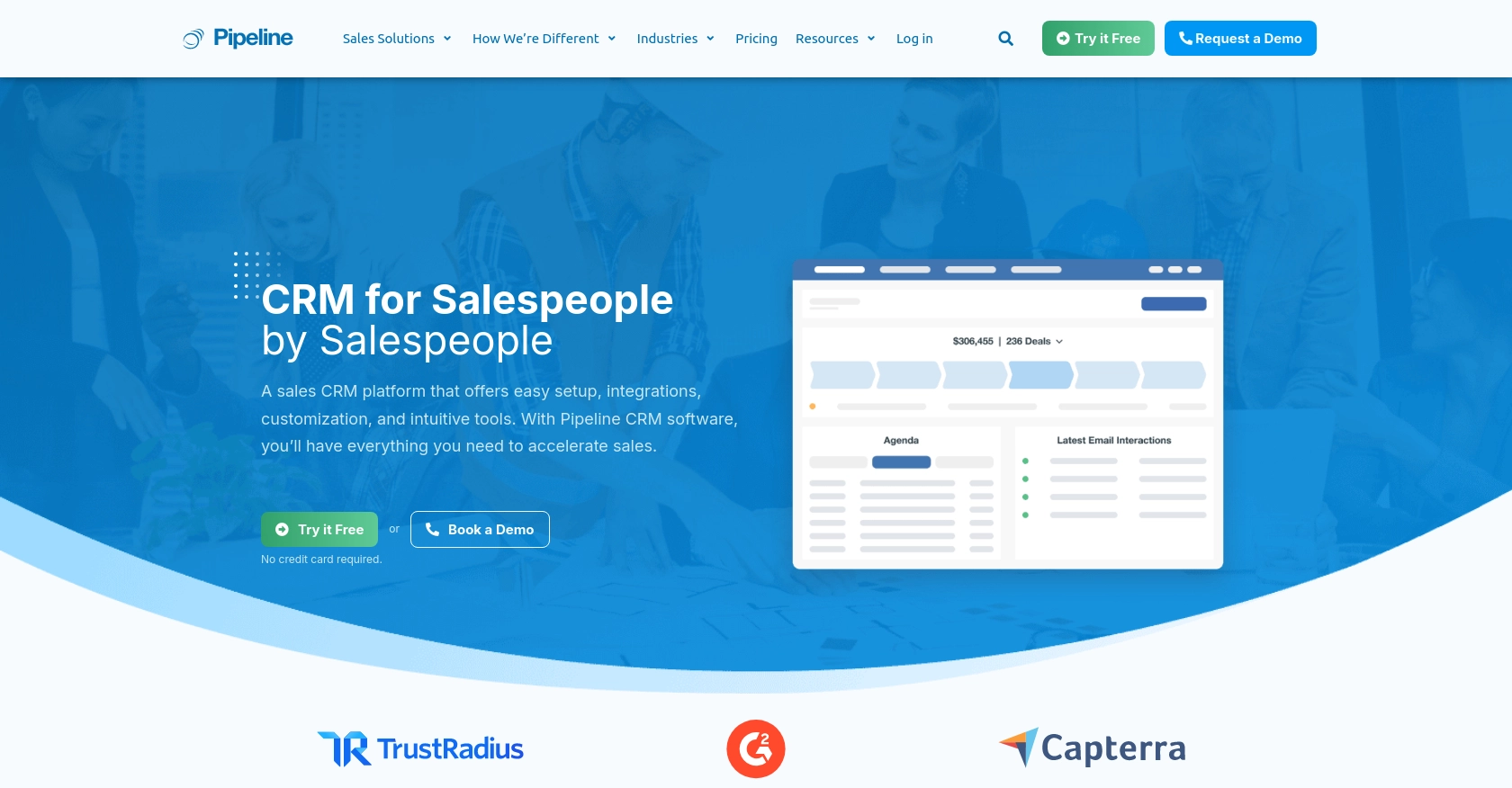
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes more effectively. With features like deal tracking, contact management, and sales forecasting, PipelineCRM provides a comprehensive solution for sales teams looking to streamline their operations.
Integrating with the PipelineCRM API allows developers to automate and enhance their sales workflows. For example, you can create deals programmatically, enabling your application to automatically log new sales opportunities as they arise. This can significantly improve efficiency and ensure that no potential deals are overlooked.
Setting Up Your PipelineCRM Test Account
Before you can start creating deals with the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting any live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial provides access to the features you'll need to test API interactions.
- Visit the PipelineCRM website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process and verify your email address.
Generating Your PipelineCRM API Key
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the "Settings" section from the main dashboard.
- Find the "API Keys" option and click on it.
- Click on "Generate New API Key" and provide a name for your key to help you identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, refer to the PipelineCRM API documentation.
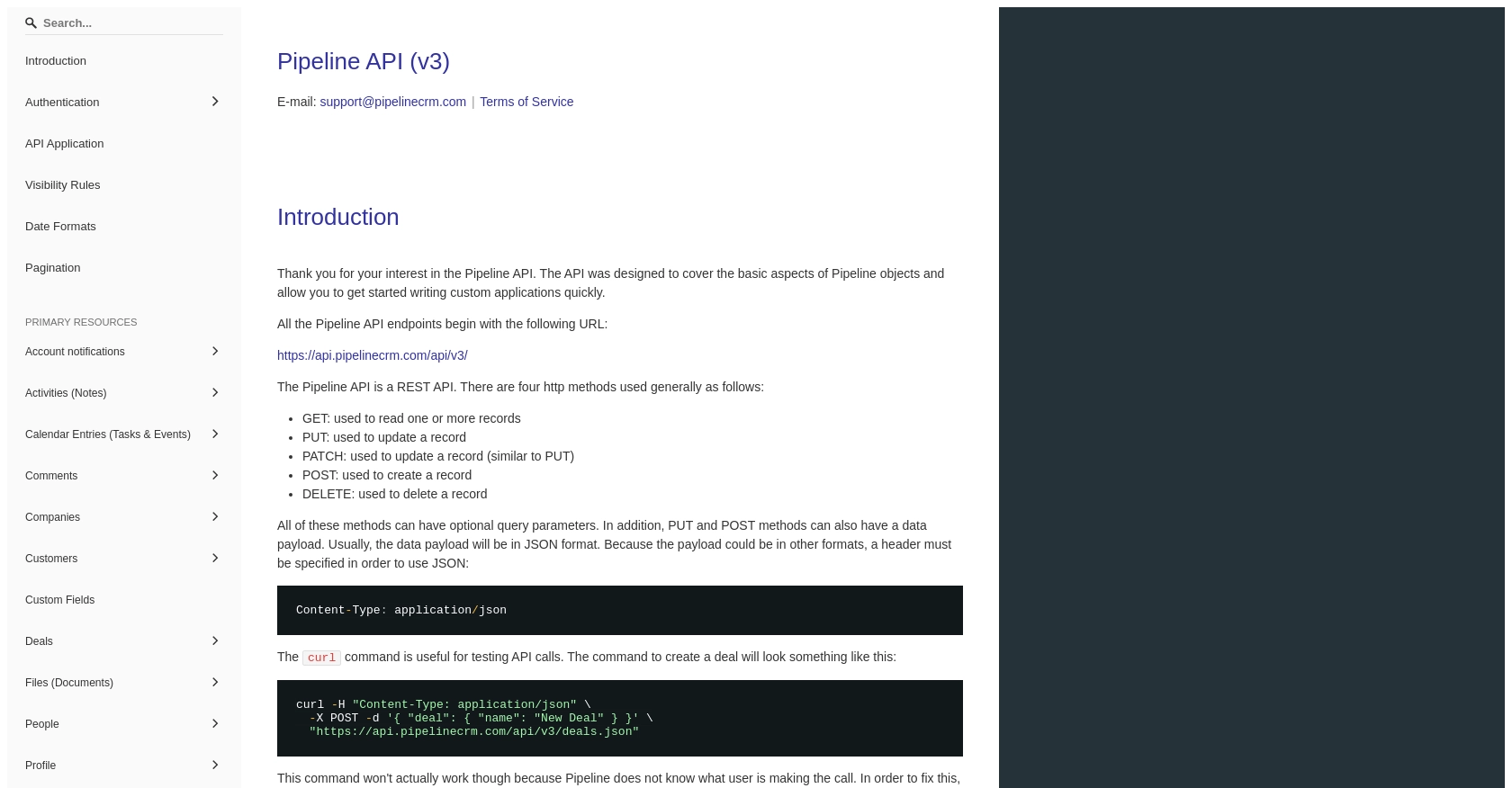
sbb-itb-96038d7
Making API Calls to Create Deals with PipelineCRM in Python
To interact with the PipelineCRM API and create deals programmatically, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for PipelineCRM API Integration
Before you begin coding, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Create Deals with PipelineCRM API
Now that your environment is ready, you can write the Python script to create deals in PipelineCRM. Follow these steps:
import requests
# Define the API endpoint for creating deals
url = "https://api.pipelinecrm.com/api/v3/deals"
# Set your API key
api_key = "Your_API_Key"
# Define the headers, including the API key for authentication
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
# Define the deal data
deal_data = {
"name": "New Deal",
"value": 10000,
"currency": "USD",
"stage_id": 1 # Example stage ID
}
# Make the POST request to create a deal
response = requests.post(url, json=deal_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Deal created successfully:", response.json())
else:
print("Failed to create deal:", response.status_code, response.text)
Replace Your_API_Key
with the API key you generated earlier. This script sets up the necessary headers and deal data, then makes a POST request to the PipelineCRM API to create a new deal. If successful, it will print the details of the created deal.
Handling API Responses and Errors
It's important to handle API responses and potential errors effectively. The above script checks the response status code to determine if the deal creation was successful. Here are some common status codes you might encounter:
- 201 Created: The deal was created successfully.
- 400 Bad Request: The request was malformed. Check your data and headers.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the PipelineCRM API documentation.
Verifying Deal Creation in PipelineCRM
After running your script, log in to your PipelineCRM account and navigate to the deals section to verify that the new deal has been created. This ensures that your API integration is working correctly.
Conclusion and Best Practices for Using PipelineCRM API in Python
Integrating with the PipelineCRM API to create deals programmatically can greatly enhance your sales workflow by automating repetitive tasks and ensuring that no opportunities are missed. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using your API key, and handle API responses effectively.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts. Consider using environment variables or a secure vault service.
- Handle Rate Limiting: Be aware of any rate limits imposed by the PipelineCRM API. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across your application.
- Error Handling: Implement robust error handling to manage different response codes and potential issues with the API.
Streamlining Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including PipelineCRM.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With Endgrate, you build once for each use case, reducing the need for multiple integrations and providing an intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?