Using the Hubspot API to Create or Update Custom Objects (with PHP examples)
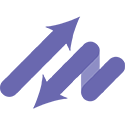
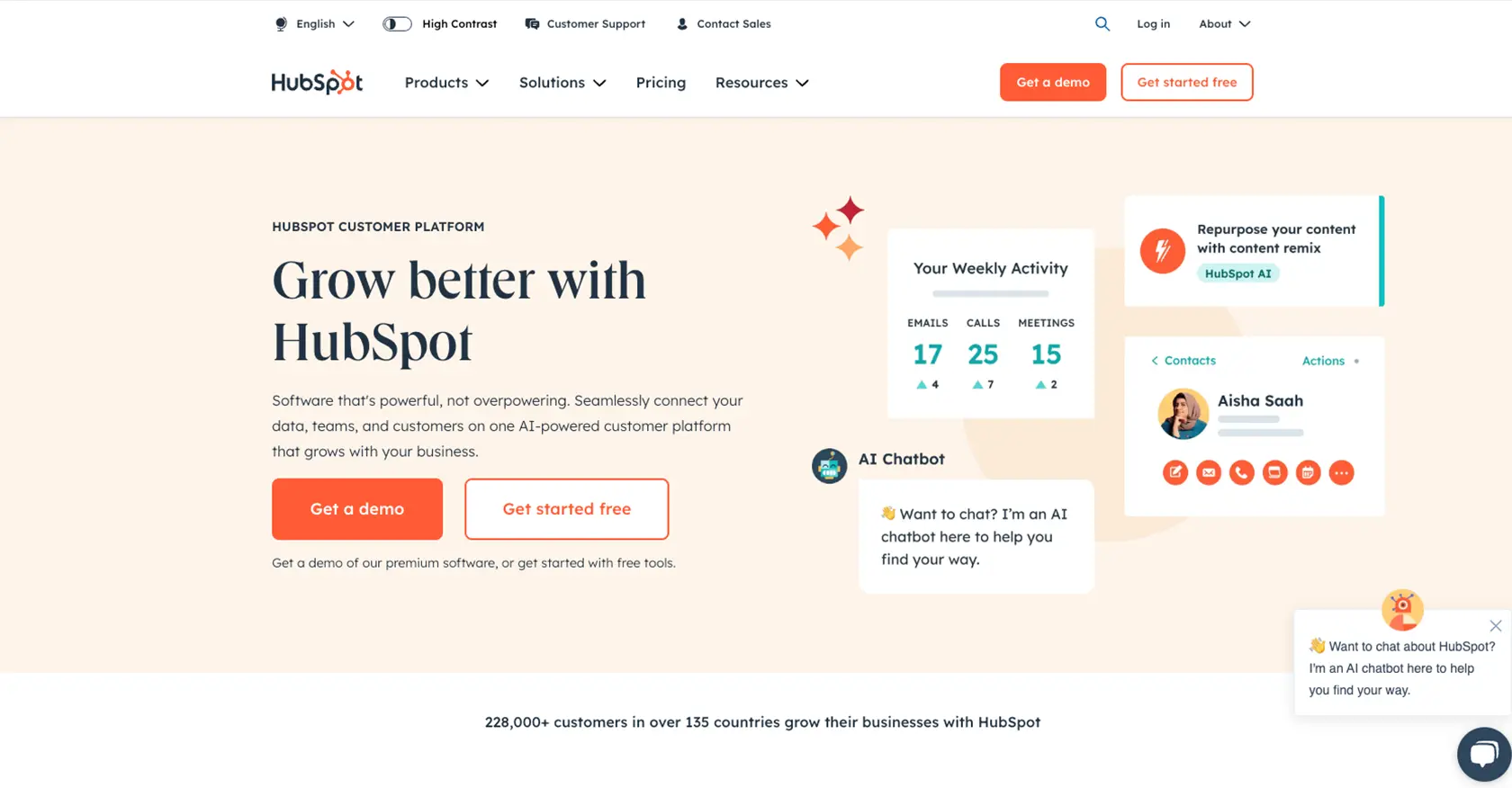
Introduction to HubSpot Custom Objects API
HubSpot is a powerful CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. One of its standout features is the ability to create and manage custom objects, allowing businesses to tailor their CRM data to fit specific needs.
Integrating with HubSpot's Custom Objects API can significantly enhance a developer's ability to manage unique business data. For example, a developer might want to create or update custom objects to track inventory, manage event registrations, or store any other specialized data that doesn't fit into HubSpot's standard objects like contacts or deals.
This article will guide you through using PHP to interact with HubSpot's API, focusing on creating and updating custom objects. By the end of this tutorial, you'll be able to efficiently manage custom data within HubSpot, streamlining your business processes and enhancing your CRM capabilities.
Setting Up Your HubSpot Developer Account for Custom Objects API
Before you can start creating or updating custom objects using HubSpot's API, you'll need to set up a developer account. This will allow you to access HubSpot's sandbox environment, where you can safely test your integrations without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal and click on "Create a developer account."
- Fill in the required information, including your email address and password, then click "Sign up."
- Verify your email address by clicking the link sent to your inbox.
- Log in to your new developer account to access the HubSpot dashboard.
Setting Up a HubSpot Sandbox Environment
Once your developer account is ready, you can set up a sandbox environment to test your API integrations:
- In your HubSpot dashboard, navigate to the "Test Accounts" section.
- Click "Create a test account" to generate a sandbox environment.
- Follow the prompts to configure your test account settings, including selecting the features you want to test.
- Once created, you'll have access to a fully functional HubSpot account for testing purposes.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for secure API access. Follow these steps to configure OAuth for your application:
- In your HubSpot developer account, go to "Apps" and click "Create app."
- Enter the app details, including name and description, then click "Create app."
- Navigate to the "Auth" tab and select "OAuth 2.0" as the authentication method.
- Specify the necessary scopes for accessing custom objects, such as
crm.objects.custom.write
andcrm.objects.custom.read
. - Save your changes and note down the client ID and client secret, which you'll use to authenticate API requests.
For more detailed information on setting up OAuth, refer to the HubSpot OAuth Documentation.
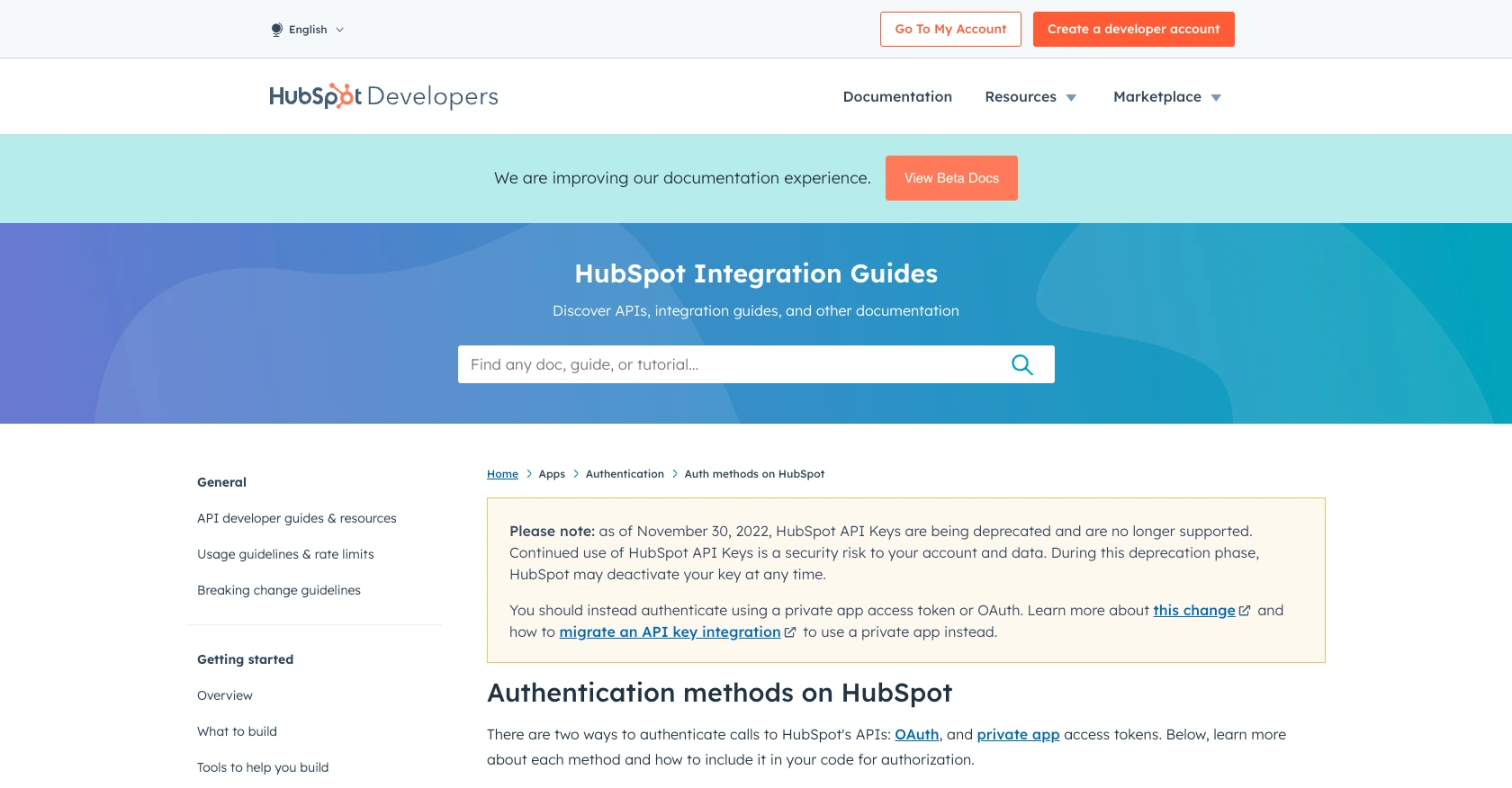
sbb-itb-96038d7
Making API Calls to HubSpot Custom Objects Using PHP
To interact with HubSpot's Custom Objects API using PHP, you'll need to set up your environment and write code to create or update custom objects. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
Installing Required PHP Packages for HubSpot API
You'll need the Guzzle HTTP client to make HTTP requests to the HubSpot API. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update HubSpot Custom Objects
With your environment set up, you can now write PHP code to interact with the HubSpot API. Below is an example of how to create or update a custom object:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_OAuth_Access_Token';
try {
$response = $client->request('POST', 'https://api.hubapi.com/crm/v3/objects/custom_object', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
],
'json' => [
'properties' => [
'property_name' => 'Property Value',
'another_property' => 'Another Value'
]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Custom Object ID: ' . $data['id'];
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_OAuth_Access_Token
with the OAuth token obtained during authentication. This code snippet demonstrates how to send a POST request to create a custom object with specified properties.
Verifying Successful API Requests in HubSpot
After executing the PHP script, verify the creation or update of the custom object in your HubSpot sandbox account. Navigate to the custom objects section to see the new or updated entries.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors. HubSpot API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 429 Too Many Requests: Rate limit exceeded. Refer to HubSpot's rate limit documentation for more details.
Implement error handling in your code to manage these responses effectively.
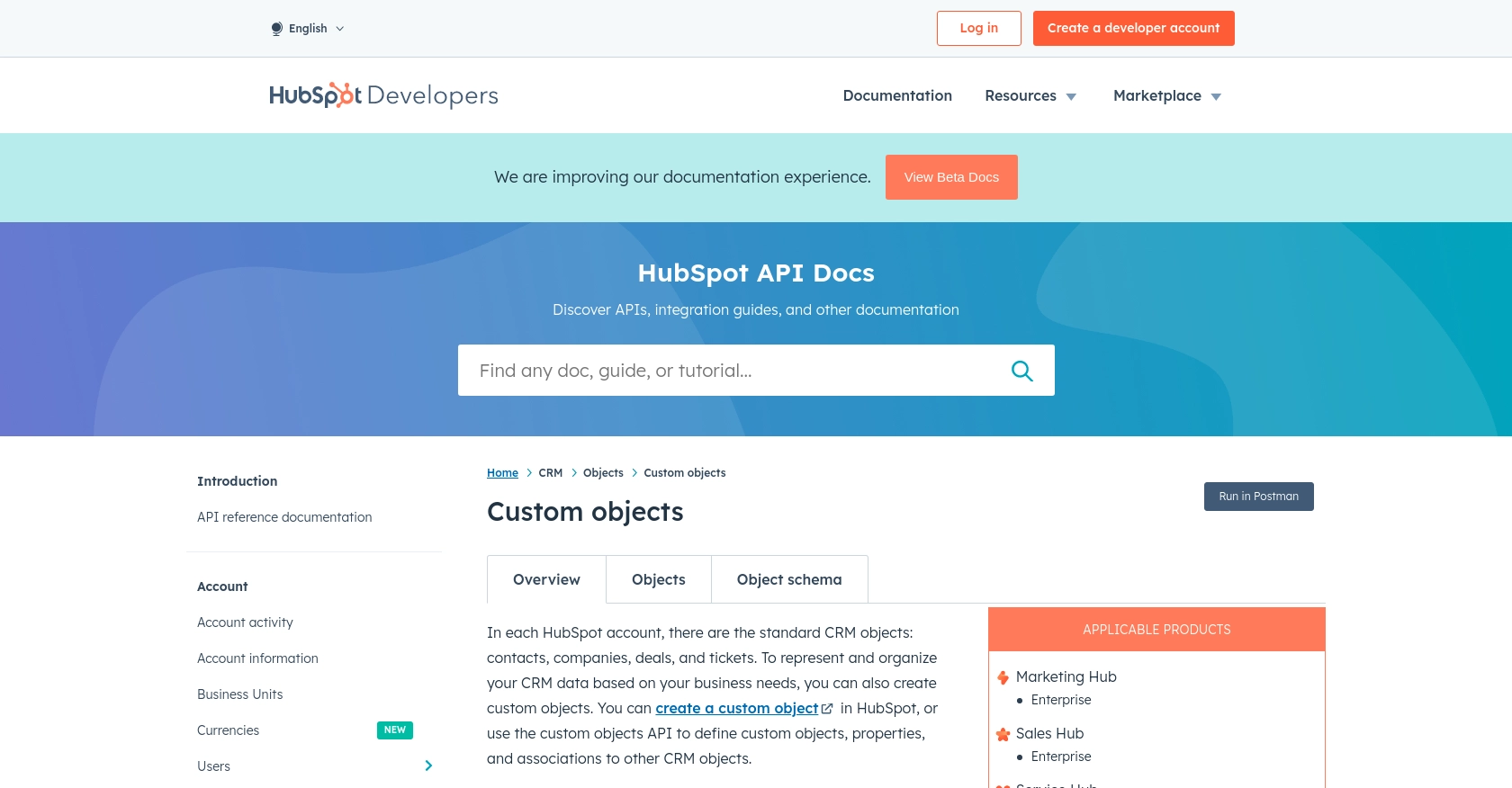
Conclusion and Best Practices for Using HubSpot Custom Objects API with PHP
Integrating with HubSpot's Custom Objects API using PHP can greatly enhance your ability to manage specialized data within your CRM. By following the steps outlined in this guide, you can efficiently create and update custom objects, allowing for more tailored data management solutions.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store OAuth Tokens: Ensure that your OAuth tokens are stored securely and refreshed as needed to maintain access without exposing sensitive information.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which are 100 requests every 10 seconds for OAuth apps. Implement request throttling to avoid exceeding these limits and receiving a 429 error. For more details, refer to the HubSpot rate limit documentation.
- Standardize Data Fields: Consistently format and validate data fields to ensure compatibility with HubSpot's API and maintain data integrity.
- Implement Robust Error Handling: Use comprehensive error handling to manage API responses effectively, ensuring that your application can gracefully handle issues such as authentication failures or invalid requests.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including HubSpot, offering an intuitive integration experience for your customers.
By building once for each use case instead of multiple times for different integrations, you can streamline your development process and enhance your product's capabilities. Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/crm-custom-objects
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?