Using the Sage 100 API to Get Sales Orders (with Javascript examples)
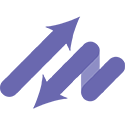
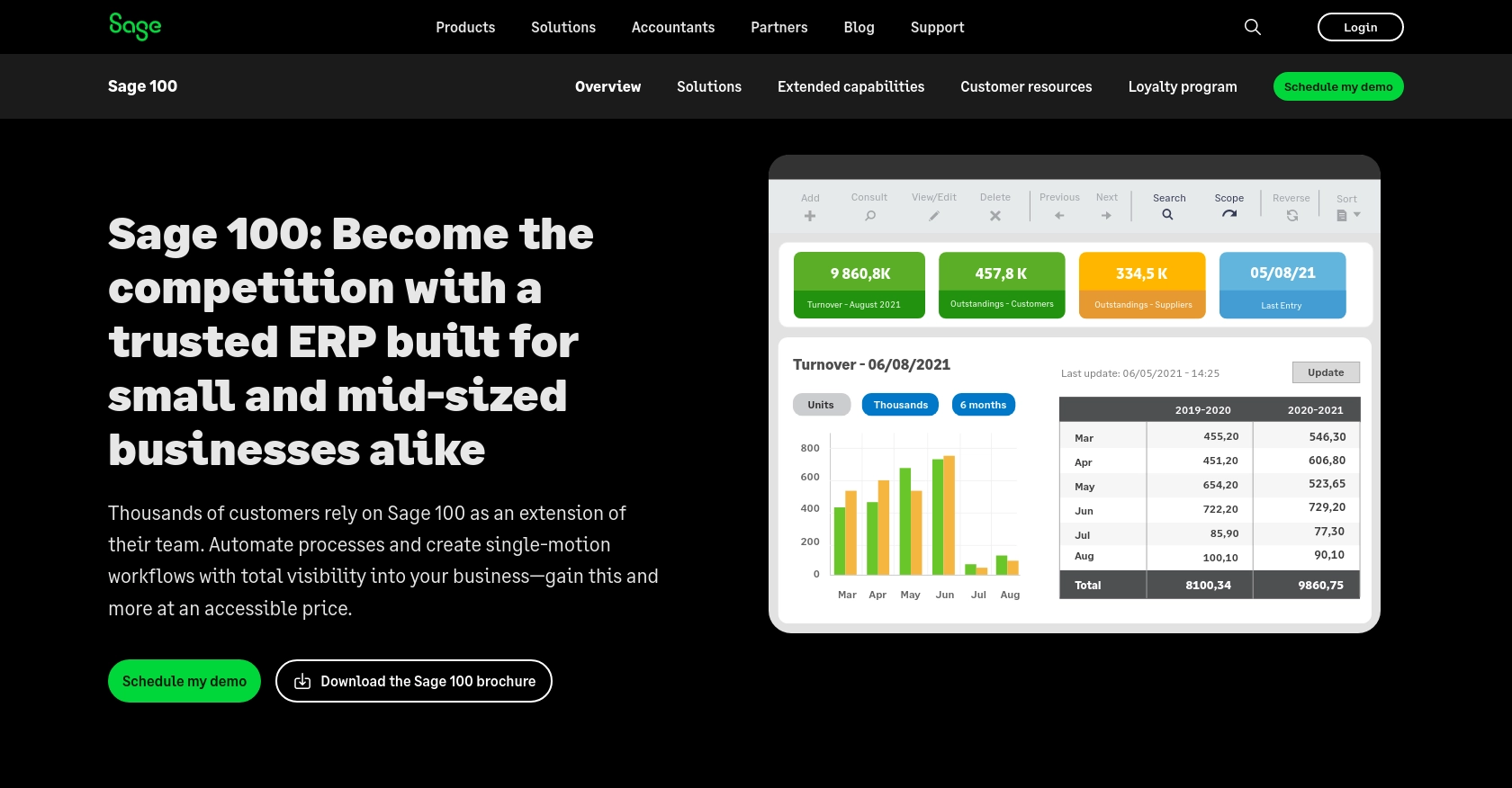
Introduction to Sage 100 API
Sage 100 is a robust enterprise resource planning (ERP) solution designed to help businesses manage their accounting, manufacturing, distribution, and inventory processes. Known for its flexibility and comprehensive features, Sage 100 is a popular choice for small to medium-sized businesses looking to streamline their operations.
Integrating with the Sage 100 API allows developers to access and manipulate data within the Sage 100 system, enabling automation and enhanced data management. For example, a developer might use the Sage 100 API to retrieve sales orders, allowing for real-time updates and analysis of sales data within a custom application or dashboard.
Setting Up a Sage 100 Test/Sandbox Account
Before you can start interacting with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to configure your Sage 100 environment for development:
Install and Configure the Sage 100 ODBC Driver
To connect to the Sage 100 database, ensure that the Sage 100 ODBC driver is installed and properly configured on your system. This involves setting up a Data Source Name (DSN) that connects to the Sage 100 ERP system:
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN that points to the Sage 100 database, using the correct server, database, and authentication settings.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Configure Sage 100 for Client/Server ODBC
Depending on your setup, you may need to configure Sage 100 to run the client/server ODBC driver as an application or service:
- To run as an application, locate and double-click the
pvxiosvr.exe
file in the Sage 100 installation directory. - To run as a service, use the command line to execute
pvxiosvr.exe -i
on the server.
Ensure the service is running correctly by checking the Server Manager and verifying the service status.
Test the ODBC Connection
Once the ODBC driver is configured, test the connection to ensure everything is set up correctly:
- Open the ODBC Data Source Administrator and locate your DSN.
- Use the "Test Connection" feature to verify connectivity.
If successful, your Sage 100 environment is ready for API interactions.
Handle Authentication for Sage 100 API
Sage 100 uses a custom authentication method. Ensure that your application has the necessary credentials to access the API:
- Use the DSN configured earlier to establish a connection via the
System.Data.Odbc
namespace in your application. - Handle exceptions using try-catch blocks to manage connection errors and timeouts.
With these steps completed, you are now ready to start making API calls to Sage 100.
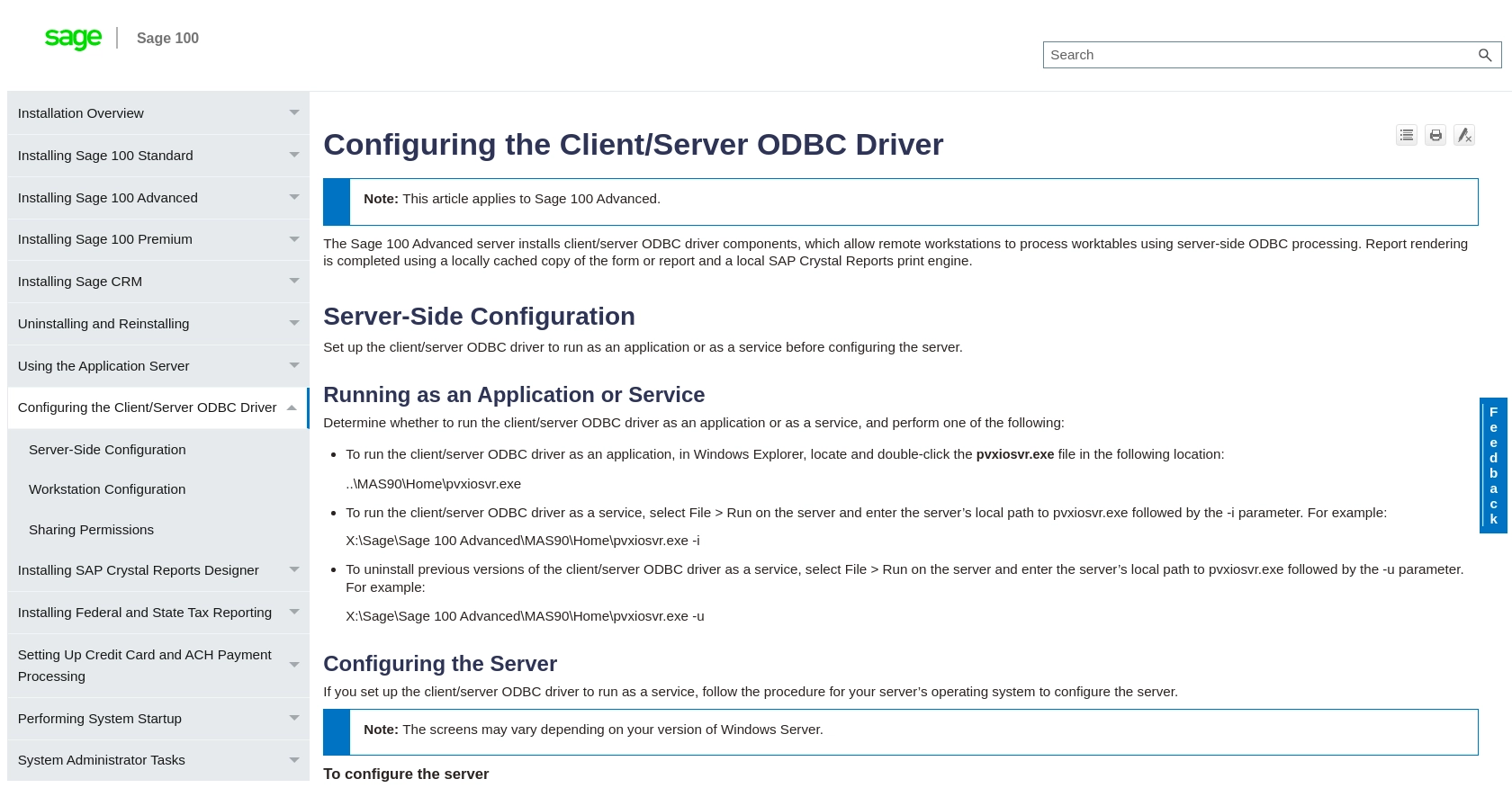
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve sales orders, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Sage 100 API
Before making API calls, ensure you have a JavaScript environment ready. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Retrieve Sales Orders from Sage 100
With your environment set up, you can now write the JavaScript code to interact with the Sage 100 API. The following example demonstrates how to retrieve sales orders using the axios
library.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://your-sage100-api-endpoint/salesorders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Function to get sales orders
async function getSalesOrders() {
try {
const response = await axios.get(endpoint, { headers });
const salesOrders = response.data;
console.log('Sales Orders:', salesOrders);
} catch (error) {
console.error('Error fetching sales orders:', error);
}
}
// Call the function
getSalesOrders();
Replace Your_Token
with the actual token obtained during the authentication setup. The code above defines the API endpoint and headers, then uses axios.get
to make a GET request to the Sage 100 API. If successful, it logs the sales orders to the console.
Verifying Successful API Requests and Handling Errors
After running the code, verify the request's success by checking the console output. You should see a list of sales orders retrieved from the Sage 100 system. If the request fails, the error message will be logged to the console.
- Ensure your API endpoint and token are correct.
- Check for network connectivity issues if the request fails.
- Handle errors gracefully by using try-catch blocks and logging error details.
For more detailed error handling, refer to the Sage 100 API documentation.
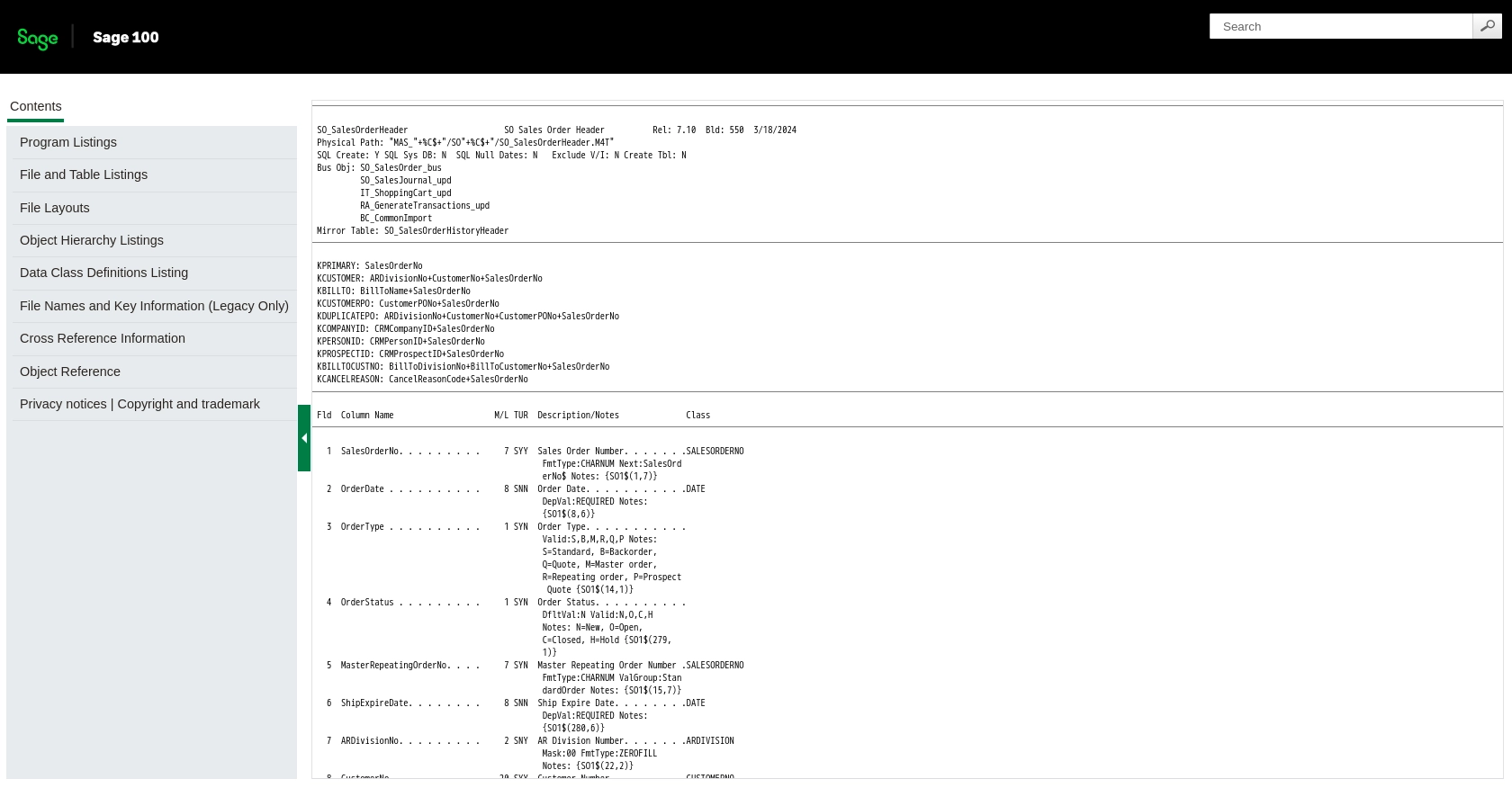
Conclusion and Best Practices for Using Sage 100 API with JavaScript
Integrating with the Sage 100 API to retrieve sales orders using JavaScript can significantly enhance your business operations by providing real-time access to critical sales data. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your application, and make API calls to Sage 100.
Best Practices for Secure and Efficient API Integration with Sage 100
- Securely Store Credentials: Always store your API tokens and credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Sage 100 is transformed and standardized to fit your application's requirements. This will help maintain data consistency across your systems.
- Error Handling: Implement robust error handling to manage exceptions and provide meaningful feedback to users. This includes logging errors and handling network issues effectively.
Streamlining Integration Processes with Endgrate
While integrating with Sage 100 API can be a powerful way to enhance your applications, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sage 100.
By leveraging Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderHeader.htm?Highlight=SO_SalesOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderDetail.htm?Highlight=SO_SalesOrderDetail
Ready to get started?