How to Create Deals with the Zoho CRM API in PHP
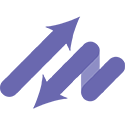
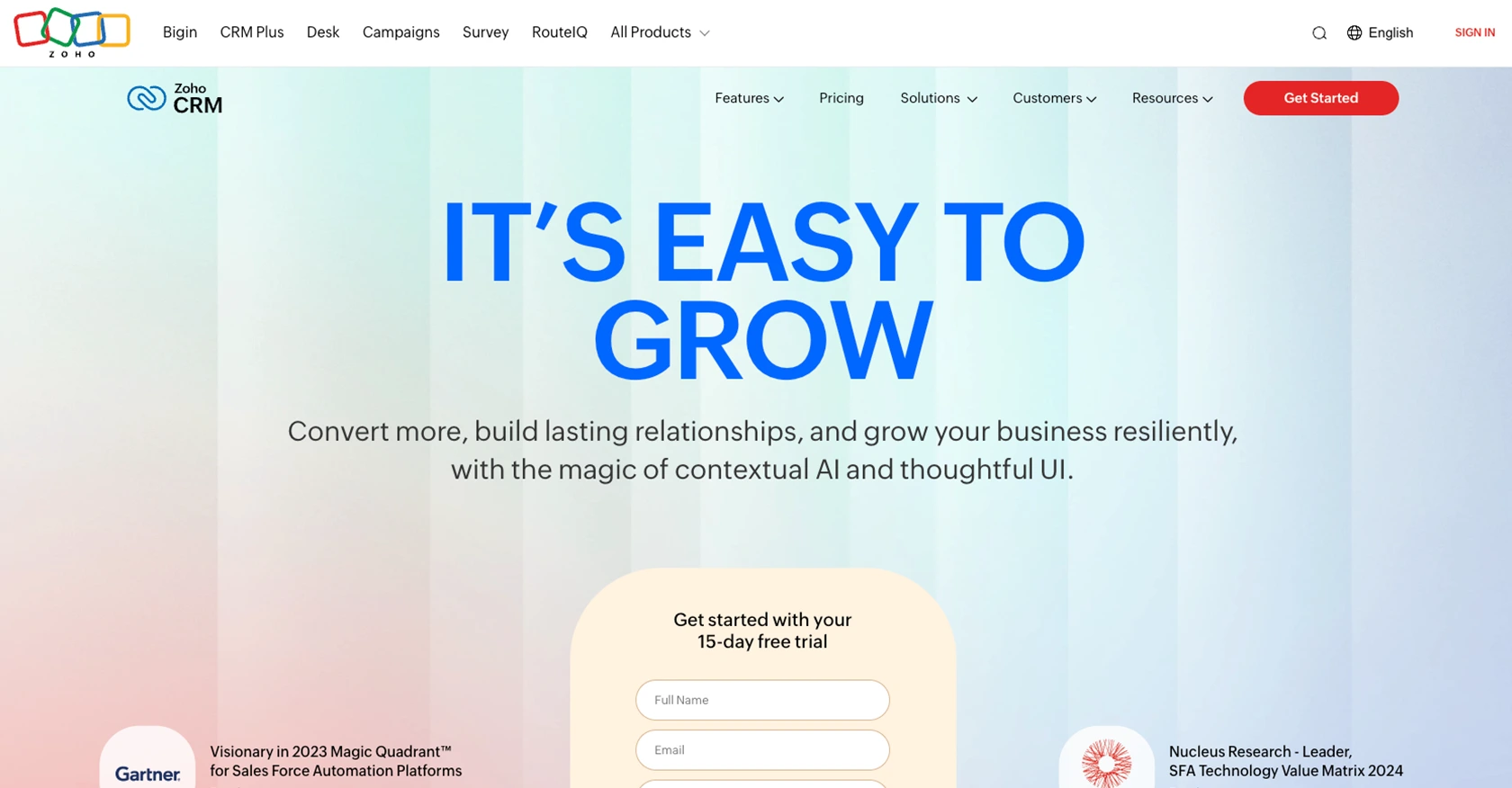
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and scalability, Zoho CRM is a preferred choice for businesses aiming to enhance their customer engagement and streamline operations.
Developers often integrate with Zoho CRM to automate and optimize various business processes. For example, using the Zoho CRM API, developers can create deals programmatically, allowing sales teams to manage opportunities more efficiently and focus on closing deals rather than manual data entry.
This article will guide you through creating deals in Zoho CRM using PHP, providing step-by-step instructions to leverage the API for seamless integration.
Setting Up Your Zoho CRM Sandbox Account
Before you can start creating deals with the Zoho CRM API in PHP, you'll need to set up a sandbox account. This allows you to test your integration without affecting your live data. Zoho CRM offers a developer sandbox environment that replicates your production setup, providing a safe space for testing.
Step 1: Sign Up for a Zoho Developer Account
If you don't already have a Zoho account, you'll need to create one. Visit the Zoho Developer Console and sign up for a free account. If you already have an account, simply log in.
Step 2: Create a Sandbox Environment
Once logged in, navigate to the CRM section and select the option to create a sandbox. Follow the on-screen instructions to set up your sandbox environment. This will mirror your production environment, allowing you to test API calls safely.
Step 3: Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication. To interact with the API, you'll need to register your application:
- Go to the Zoho Developer Console.
- Select "Register Client" and choose the client type that suits your application (e.g., Web-based, Self Client).
- Fill in the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Step 4: Generate OAuth Tokens
With your Client ID and Client Secret, you can now generate OAuth tokens:
- Make an authorization request to Zoho's OAuth server to obtain an authorization code.
- Exchange the authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the OAuth Overview documentation for detailed steps on generating tokens.
Step 5: Set Up API Scopes
Ensure your application has the necessary scopes to create deals in Zoho CRM. Scopes define the level of access your application has. For creating deals, you might need scopes like ZohoCRM.modules.deals.CREATE
.
With your sandbox account and OAuth setup complete, you're ready to start integrating with the Zoho CRM API using PHP.
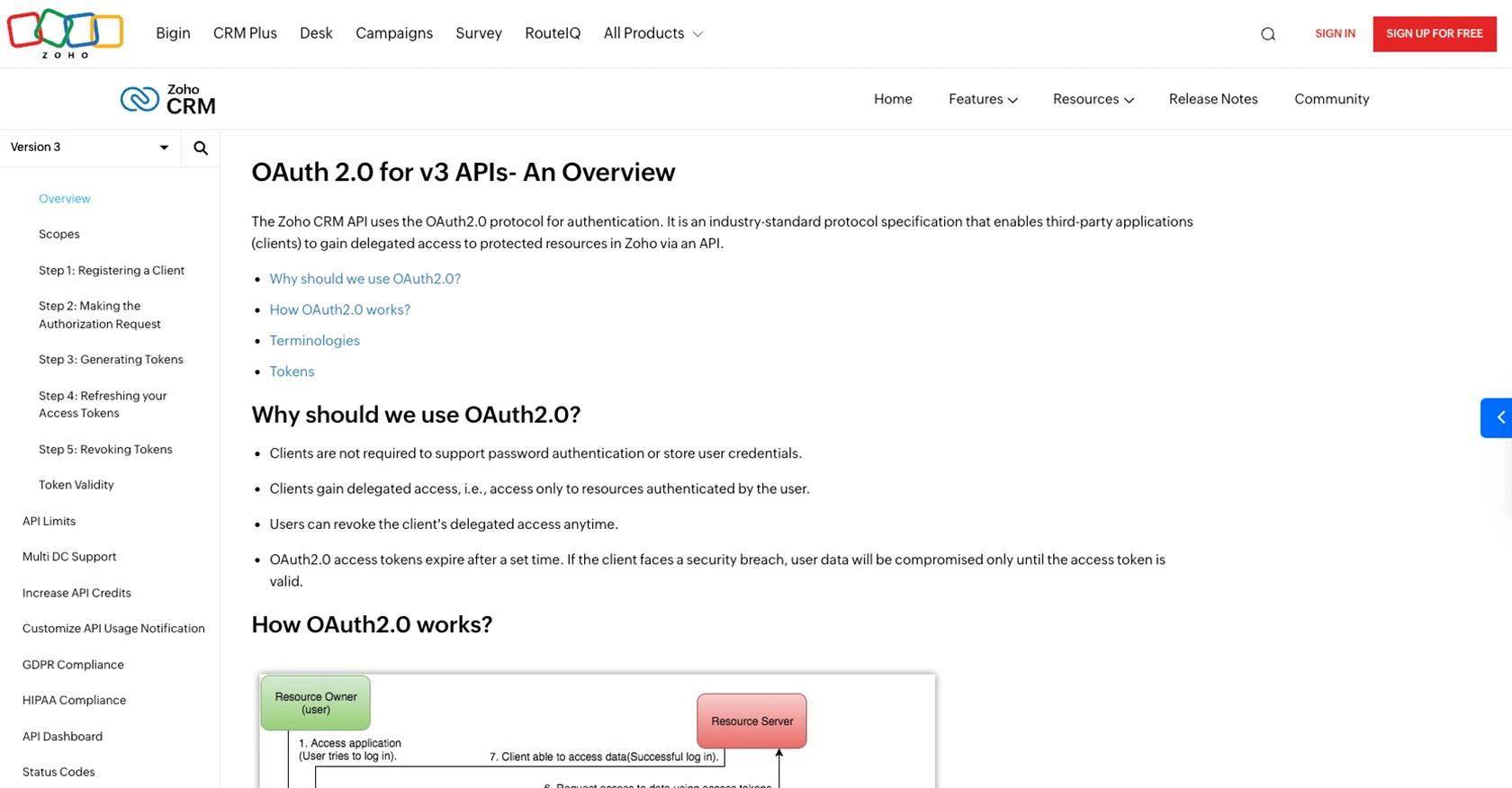
sbb-itb-96038d7
Making API Calls to Create Deals in Zoho CRM Using PHP
To create deals in Zoho CRM using PHP, you'll need to make API calls to the Zoho CRM endpoint. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API requests, and handling responses.
Setting Up Your PHP Environment for Zoho CRM API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP version 7.4 or higher.
- Ensure the
cURL
extension is enabled in yourphp.ini
file, as it is required for making HTTP requests. - Install Composer, a dependency manager for PHP, to manage any additional libraries you might need.
Installing Required PHP Libraries for Zoho CRM API
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it via Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Create Deals in Zoho CRM
Now, let's write the PHP code to create a deal in Zoho CRM:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('POST', 'https://www.zohoapis.com/crm/v3/Deals', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'data' => [
[
'Deal_Name' => 'New Deal',
'Stage' => 'Qualification',
'Amount' => 5000,
'Closing_Date' => '2024-12-31'
]
]
]
]);
echo $response->getBody();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. This code sends a POST request to the Zoho CRM API to create a new deal with specified details.
Handling API Responses and Errors
After making the API call, you should handle the response to ensure the deal was created successfully:
if ($response->getStatusCode() == 201) {
echo "Deal created successfully!";
} else {
echo "Failed to create deal. Error: " . $response->getBody();
}
Check the status code of the response. A status code of 201 indicates success, while other codes may indicate errors. Refer to the Zoho CRM API Status Codes documentation for more details.
Verifying Deal Creation in Zoho CRM Sandbox
To verify that the deal was created, log in to your Zoho CRM sandbox account and navigate to the Deals module. You should see the newly created deal listed there.
Handling Common Errors and Rate Limits
Be aware of common errors such as authentication failures or invalid data. Handle these by checking the response body for error messages. Additionally, Zoho CRM imposes rate limits on API calls. Refer to the API Limits documentation to ensure your application adheres to these limits.
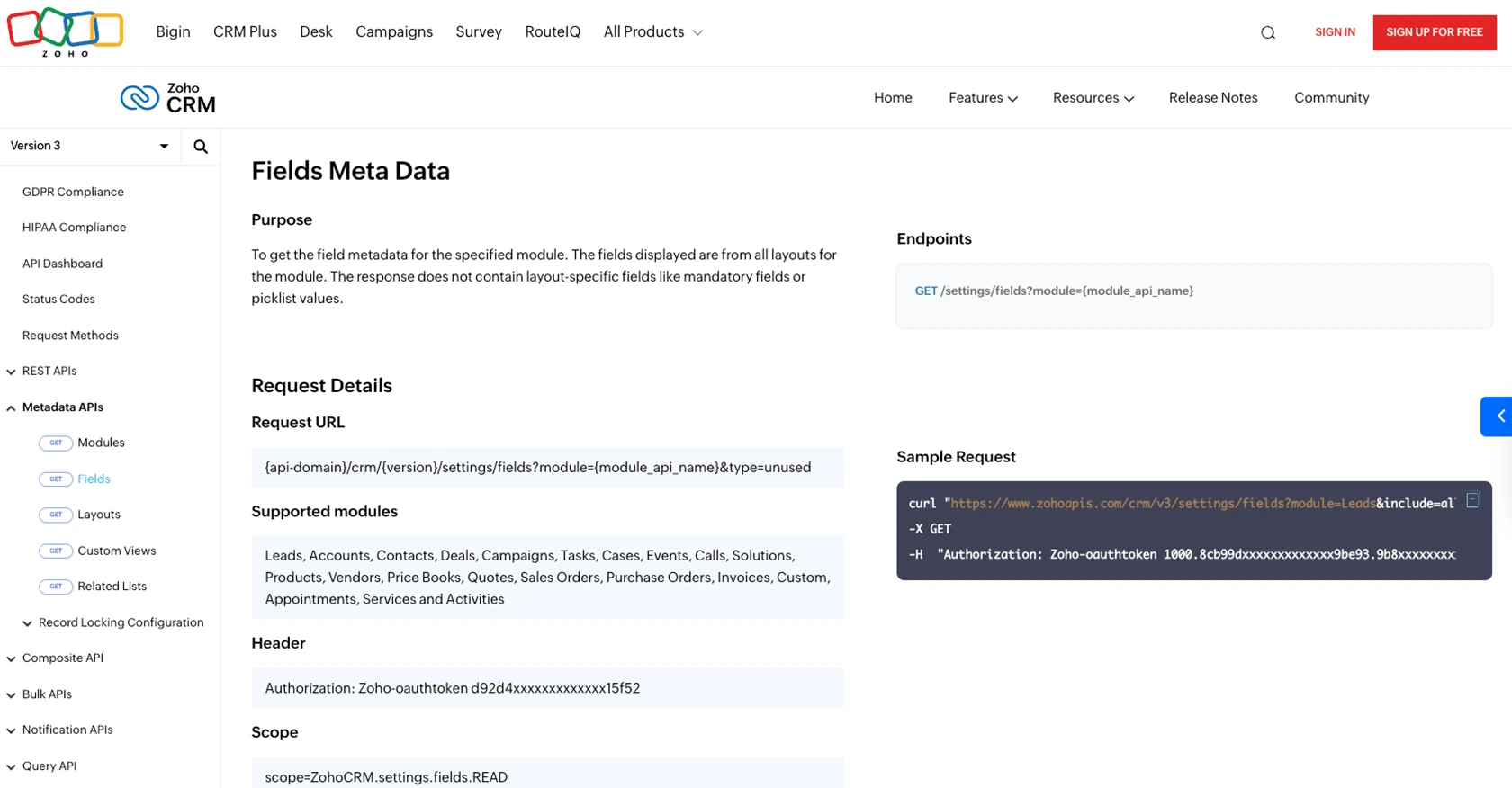
Best Practices for Integrating with Zoho CRM API
When integrating with the Zoho CRM API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho CRM imposes rate limits on API requests. Implement logic to handle rate limit errors gracefully, such as retrying requests after a delay. Refer to the API Limits documentation for specifics.
- Validate API Responses: Always check the status codes and response bodies of API calls. Implement error handling to manage different types of errors, such as authentication failures or invalid data submissions.
- Optimize Data Handling: When dealing with large datasets, consider using pagination to manage data efficiently. This ensures that your application remains responsive and performs well.
- Use Scopes Wisely: Define the minimum necessary scopes for your application to limit access to only what is needed. This enhances security by reducing the potential impact of compromised tokens.
Leveraging Endgrate for Streamlined Integrations
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop integration logic once and apply it across multiple platforms, reducing redundancy.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Conclusion: Mastering Zoho CRM API Integration with PHP
Integrating with the Zoho CRM API using PHP empowers developers to automate and enhance business processes, allowing sales teams to focus on what they do best—closing deals. By following the steps outlined in this guide, you can efficiently create deals in Zoho CRM, ensuring a seamless integration experience.
Remember to adhere to best practices for security and efficiency, and consider leveraging tools like Endgrate to simplify and streamline your integration efforts. With the right approach, you can unlock the full potential of Zoho CRM for your business.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?