How to Get Weekly Page Views with the Google Analytics API in Python
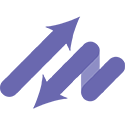
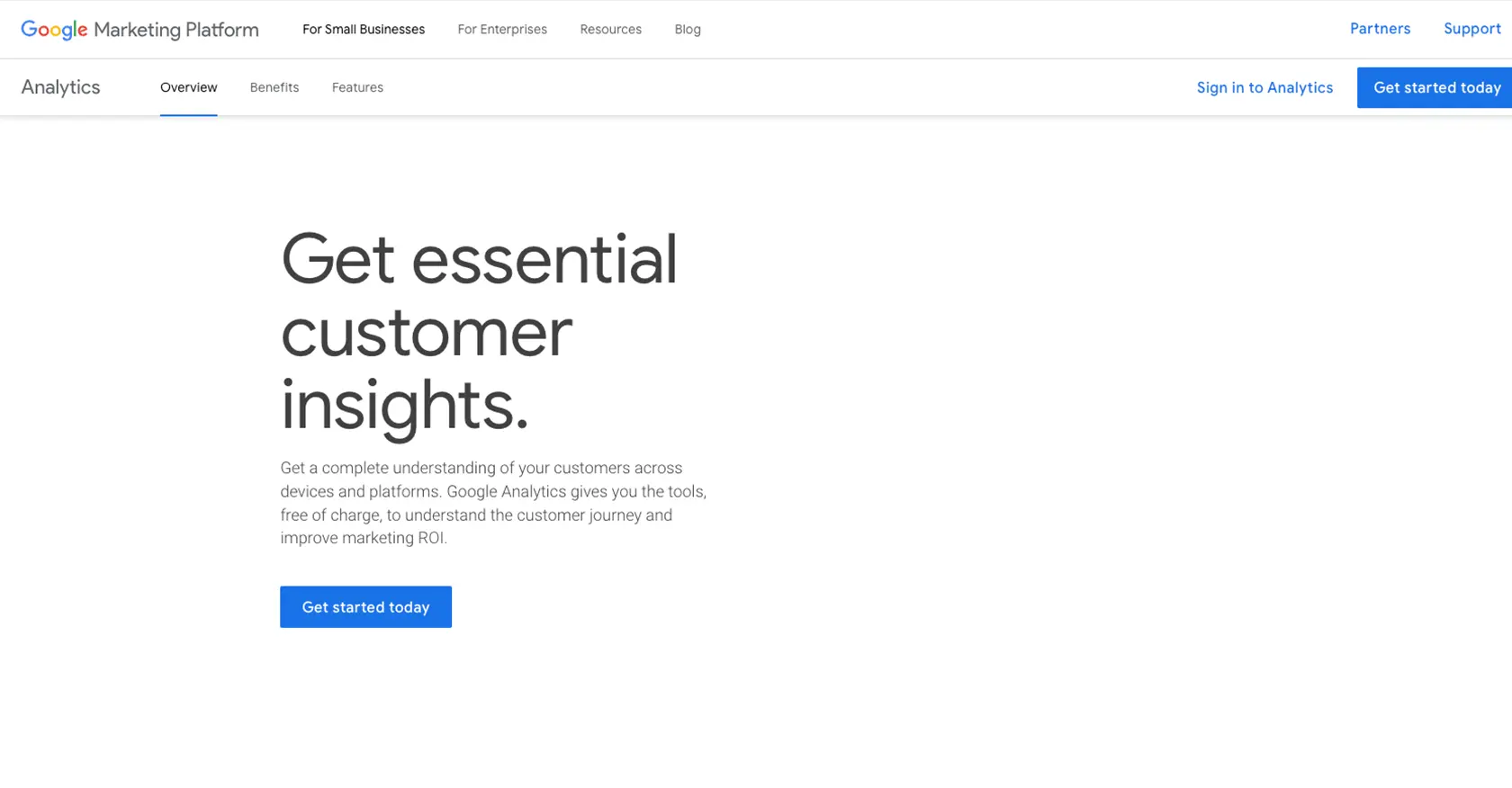
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior, helping businesses make data-driven decisions. It offers a comprehensive suite of features that allow users to track and analyze various metrics, such as page views, user demographics, and conversion rates.
For developers, integrating with the Google Analytics API can automate the retrieval of analytical data, enabling the creation of custom reports and dashboards. For example, a developer might use the API to fetch weekly page views, providing a clear picture of website performance over time.
This article will guide you through using Python to interact with the Google Analytics API, specifically focusing on retrieving weekly page views. By the end of this tutorial, you'll be able to efficiently access and analyze your website's traffic data programmatically.
Setting Up Your Google Analytics Account for API Access
Before you can start using the Google Analytics API to fetch weekly page views, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This process involves creating credentials that allow your Python application to access Google Analytics data securely.
Create a Google Cloud Project
To begin, you'll need a Google Cloud project. If you don't have one, follow these steps:
- Go to the Google Cloud Console.
- Click on the project dropdown and select "New Project."
- Enter a name for your project and click "Create."
Enable Google Analytics API
Once your project is created, you need to enable the Google Analytics API:
- In the Google Cloud Console, navigate to "APIs & Services" and then "Library."
- Search for "Google Analytics API" and click on it.
- Click "Enable" to activate the API for your project.
Configure OAuth Consent Screen
Next, set up the OAuth consent screen to define what users will see when your application requests access:
- Go to "APIs & Services" and select "OAuth consent screen."
- Choose "External" for user type and click "Create."
- Fill out the required fields and click "Save and Continue."
Create OAuth Credentials
Now, create the OAuth 2.0 credentials needed for authentication:
- Navigate to "APIs & Services" and click on "Credentials."
- Select "Create Credentials" and choose "OAuth client ID."
- Choose "Desktop app" as the application type and click "Create."
- Download the JSON file containing your client ID and client secret. Keep this file secure as it contains sensitive information.
With these steps completed, your Google Cloud project is ready to interact with the Google Analytics API using Python. You can now proceed to write code that utilizes these credentials to fetch data.
For more detailed instructions, refer to the official documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, Create Credentials.
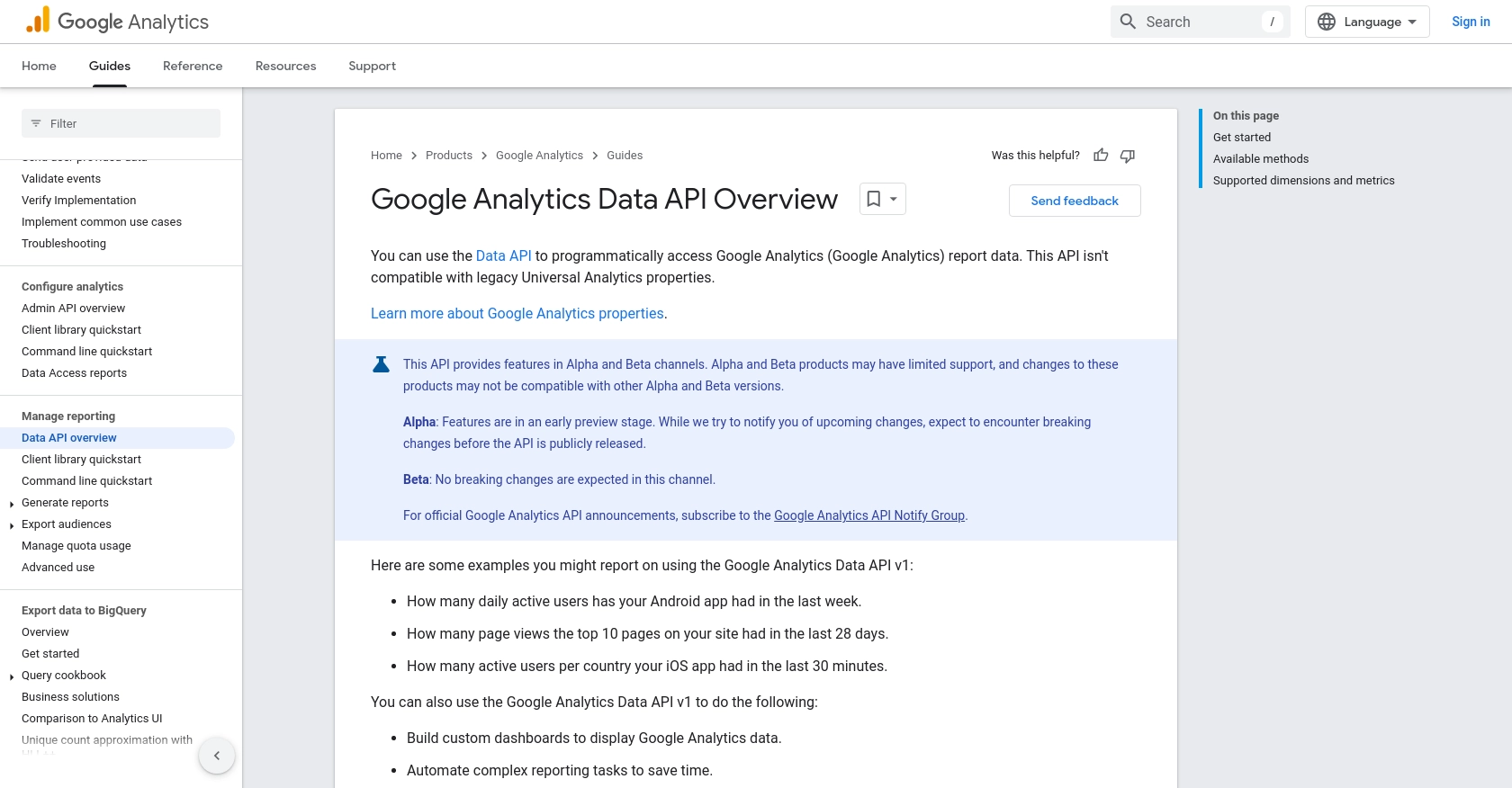
sbb-itb-96038d7
Making the API Call to Retrieve Weekly Page Views with Google Analytics API in Python
Now that you have set up your Google Cloud project and configured OAuth 2.0 authentication, it's time to make the API call to retrieve weekly page views using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Google Analytics API
Before you start coding, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the Google Analytics Data API client library, which simplifies the process of interacting with the API.
- Open your terminal or command prompt.
- Install the Google Analytics Data API client library using pip:
pip install google-analytics-data
Writing the Python Code to Fetch Weekly Page Views
With your environment set up, you can now write the Python code to interact with the Google Analytics API. The following example demonstrates how to retrieve weekly page views:
from google.analytics.data_v1beta import BetaAnalyticsDataClient from google.analytics.data_v1beta.types import DateRange, Dimension, Metric, RunReportRequest def run_report(property_id): """Fetches weekly page views from Google Analytics.""" client = BetaAnalyticsDataClient() request = RunReportRequest( property=f"properties/{property_id}", dimensions=[Dimension(name="date")], metrics=[Metric(name="pageviews")], date_ranges=[DateRange(start_date="7daysAgo", end_date="yesterday")], ) response = client.run_report(request) print_run_report_response(response) def print_run_report_response(response): """Prints the results of the runReport call.""" print(f"{response.row_count} rows received") for dimension_header in response.dimension_headers: print(f"Dimension header name: {dimension_header.name}") for metric_header in response.metric_headers: print(f"Metric header name: {metric_header.name} ({metric_header.type})") print("Report result:") for row in response.rows: print(f"Date: {row.dimension_values[0].value}, Page Views: {row.metric_values[0].value}") # Replace 'YOUR-GA4-PROPERTY-ID' with your actual Google Analytics 4 property ID run_report('YOUR-GA4-PROPERTY-ID')
Replace YOUR-GA4-PROPERTY-ID
with your actual Google Analytics 4 property ID. This code initializes the client, constructs a report request for weekly page views, and prints the results.
Verifying the API Call and Handling Errors
After running the code, you should see the weekly page views printed in your terminal. If the request is successful, the data will match the page views recorded in your Google Analytics account for the specified date range.
In case of errors, the API will return error codes that you can handle in your application. Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your OAuth credentials.
- 403 Forbidden: You do not have permission to access the resource.
Refer to the Google Analytics API documentation for more details on error handling.
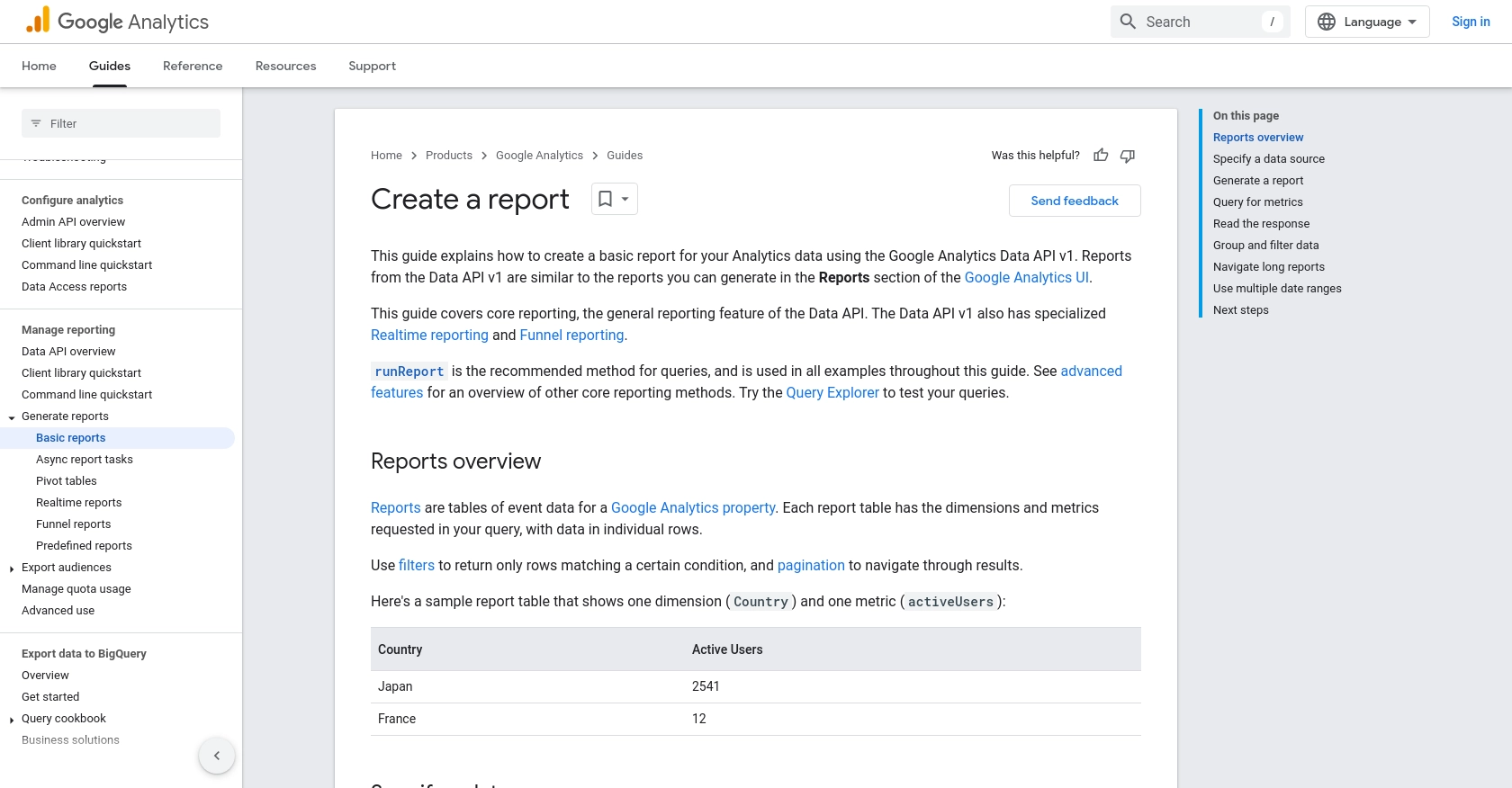
Best Practices for Using Google Analytics API in Python
When working with the Google Analytics API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Keep your OAuth credentials secure. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle API Rate Limits: Google Analytics API has rate limits. To avoid exceeding these limits, implement exponential backoff strategies for retrying requests. For more details, refer to the API documentation.
- Optimize Data Requests: Only request the data you need by specifying precise dimensions and metrics. This reduces the load on the API and speeds up response times.
- Standardize Data Formats: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This will make it easier to integrate with other systems.
Leverage Endgrate for Seamless API Integrations
Building and maintaining integrations with multiple APIs can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Analytics.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of API integrations.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?