Using the Younium API to Get Products in Javascript
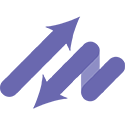
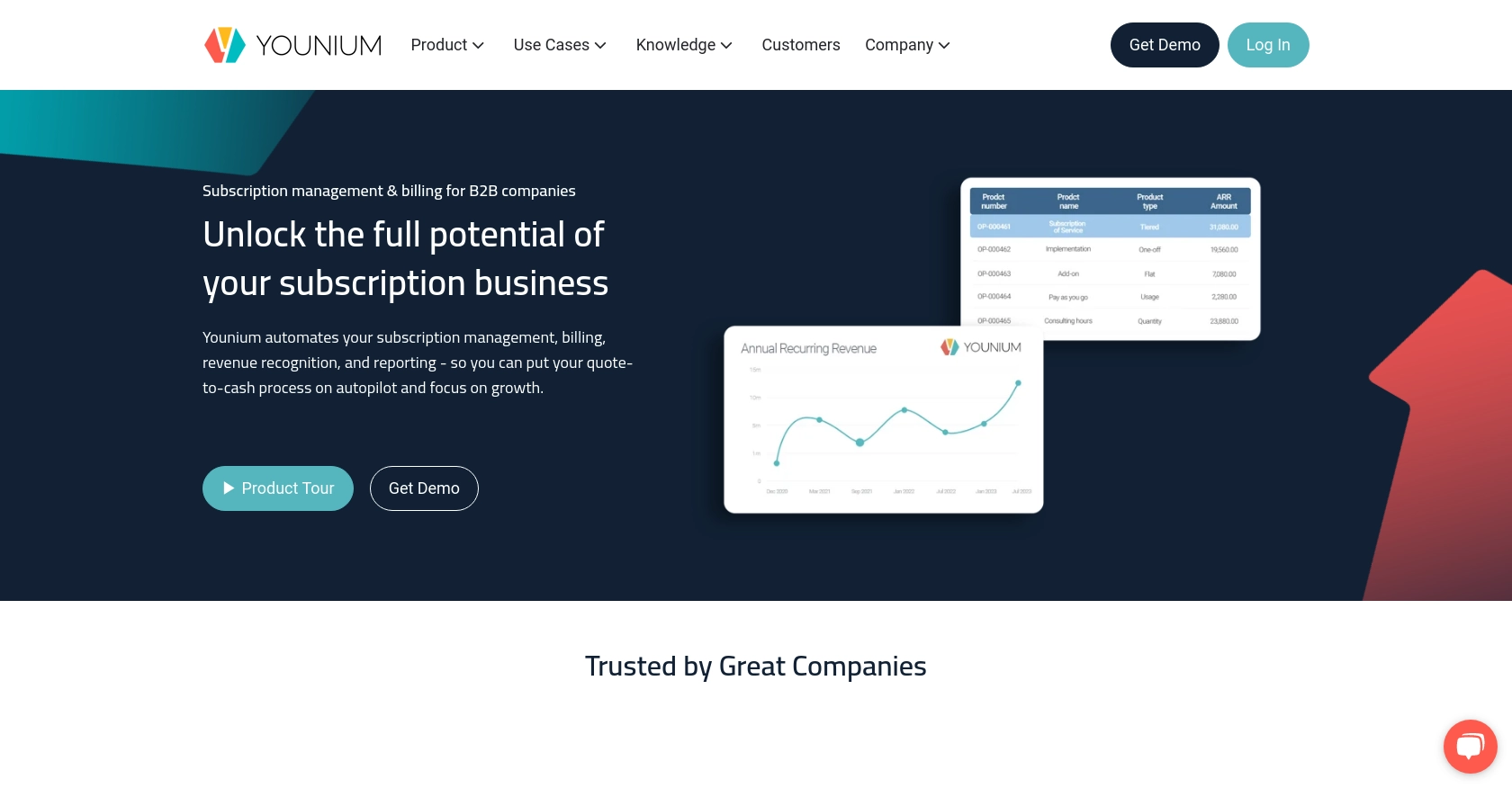
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform tailored for B2B companies. It offers a robust suite of tools for managing billing, invoicing, and financial reporting, making it an essential solution for businesses looking to streamline their subscription processes.
Integrating with the Younium API allows developers to automate and enhance their subscription management workflows. For example, by using the Younium API, developers can efficiently retrieve product information, enabling seamless synchronization of product catalogs between Younium and other systems.
This article will guide you through the process of using JavaScript to interact with the Younium API, specifically focusing on retrieving product data. By following this tutorial, you'll learn how to set up authentication and make API calls to access product information, empowering you to build more integrated and efficient solutions.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start interacting with the Younium API using JavaScript, you'll need to set up a sandbox account. This allows you to test API calls without affecting your production data. Younium provides a sandbox environment that mimics the production environment, enabling developers to experiment and validate their integrations safely.
Creating a Younium Sandbox Account
- Visit the Younium Developer Portal and sign up for a sandbox account if you haven't already.
- Once registered, log in to your Younium sandbox account.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate an API token and client credentials. Follow these steps:
- Click on your profile name in the top right corner and select “Privacy & Security”.
- Navigate to “Personal Tokens” and click on “Generate Token”.
- Provide a relevant description for your token and click “Create”.
- Copy the generated Client ID and Secret Key. These credentials will be used to acquire the JWT access token.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token. This token is necessary for authenticating your API requests:
// Example POST request to acquire JWT token
const fetch = require('node-fetch');
const url = 'https://api.sandbox.younium.com/auth/token';
const headers = {
'Content-Type': 'application/json'
};
const body = JSON.stringify({
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
});
fetch(url, {
method: 'POST',
headers: headers,
body: body
})
.then(response => response.json())
.then(data => console.log('Access Token:', data.accessToken))
.catch(error => console.error('Error:', error));
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. If successful, the response will include an accessToken
that you will use for subsequent API calls.
Handling Authentication Errors
If you encounter any errors during authentication, check the following:
- A 400 Bad Request or 401 Unauthorized response indicates invalid credentials or an expired token. Ensure your client ID and secret are correct.
- Refer to the Younium API documentation for more details on handling authentication errors.
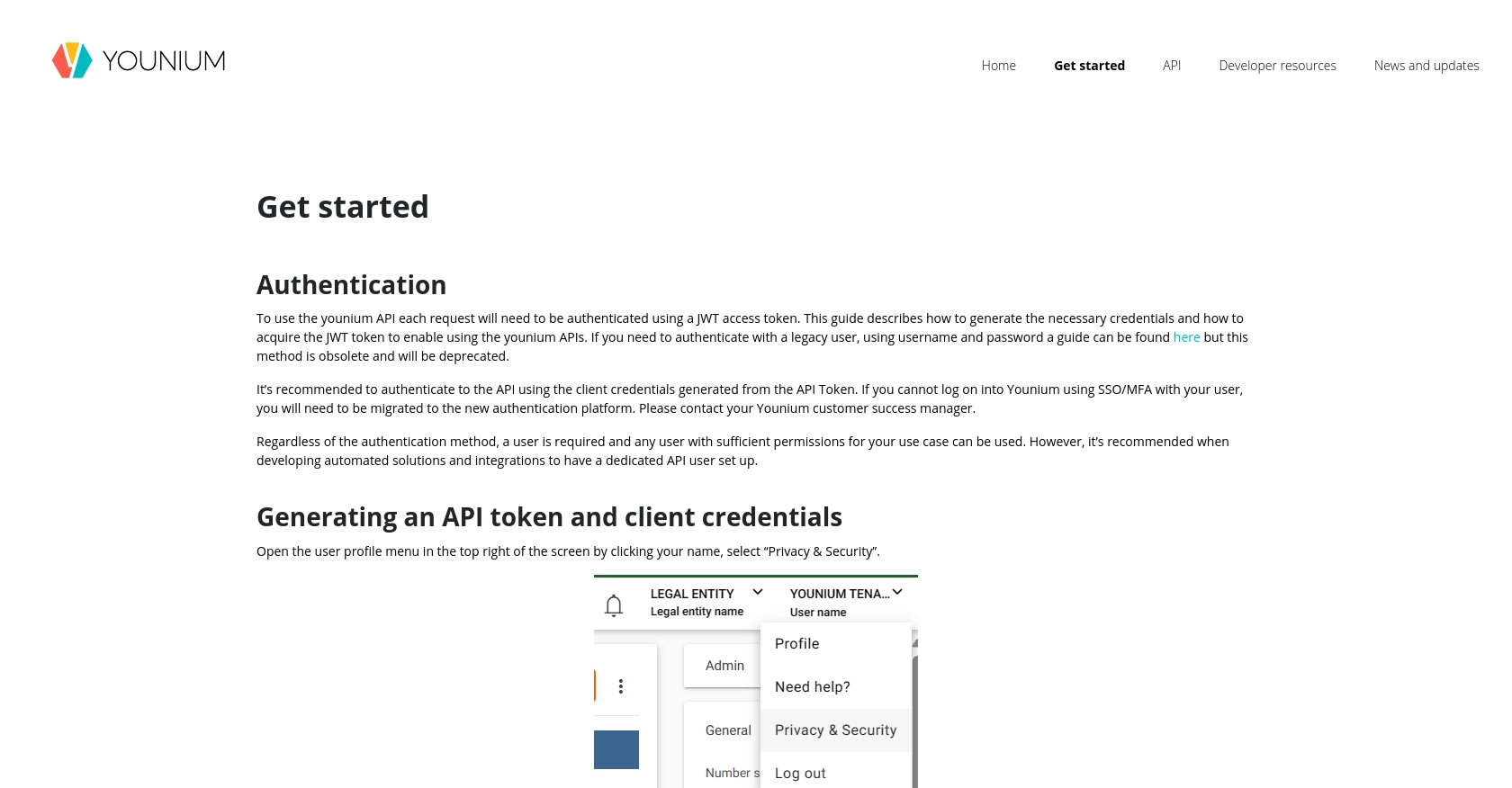
sbb-itb-96038d7
Making API Calls to Retrieve Products from Younium Using JavaScript
Once you have successfully acquired the JWT access token, you can proceed to make API calls to the Younium API to retrieve product information. This section will guide you through the process of setting up your JavaScript environment and executing the necessary code to access product data.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure that your JavaScript environment is properly configured. You will need Node.js installed on your machine to run the JavaScript code. Additionally, you will use the node-fetch
library to handle HTTP requests.
- Ensure Node.js is installed. You can download it from the official Node.js website.
- Install the
node-fetch
library by running the following command in your terminal:
npm install node-fetch
Executing the API Call to Get Products from Younium
With your environment set up, you can now write the JavaScript code to make an API call to Younium and retrieve product data. Use the following code as a template:
const fetch = require('node-fetch');
const url = 'https://api.sandbox.younium.com/products';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json',
'api-version': '2.1'
};
fetch(url, {
method: 'GET',
headers: headers
})
.then(response => response.json())
.then(data => console.log('Products:', data))
.catch(error => console.error('Error:', error));
Replace Your_Access_Token
with the JWT token you obtained earlier. This code sends a GET request to the Younium API to fetch product data.
Verifying Successful API Requests and Handling Errors
After running the code, you should see the product data output in your console. If the request is successful, the data will include details about the products available in your Younium sandbox account.
In case of errors, consider the following:
- A 401 Unauthorized response indicates an expired or incorrect access token. Ensure your token is valid and correctly included in the headers.
- A 403 Forbidden response may occur if the specified legal entity is incorrect or if permissions are insufficient. Verify the legal entity and user permissions.
- Refer to the Younium API documentation for more details on handling API errors.
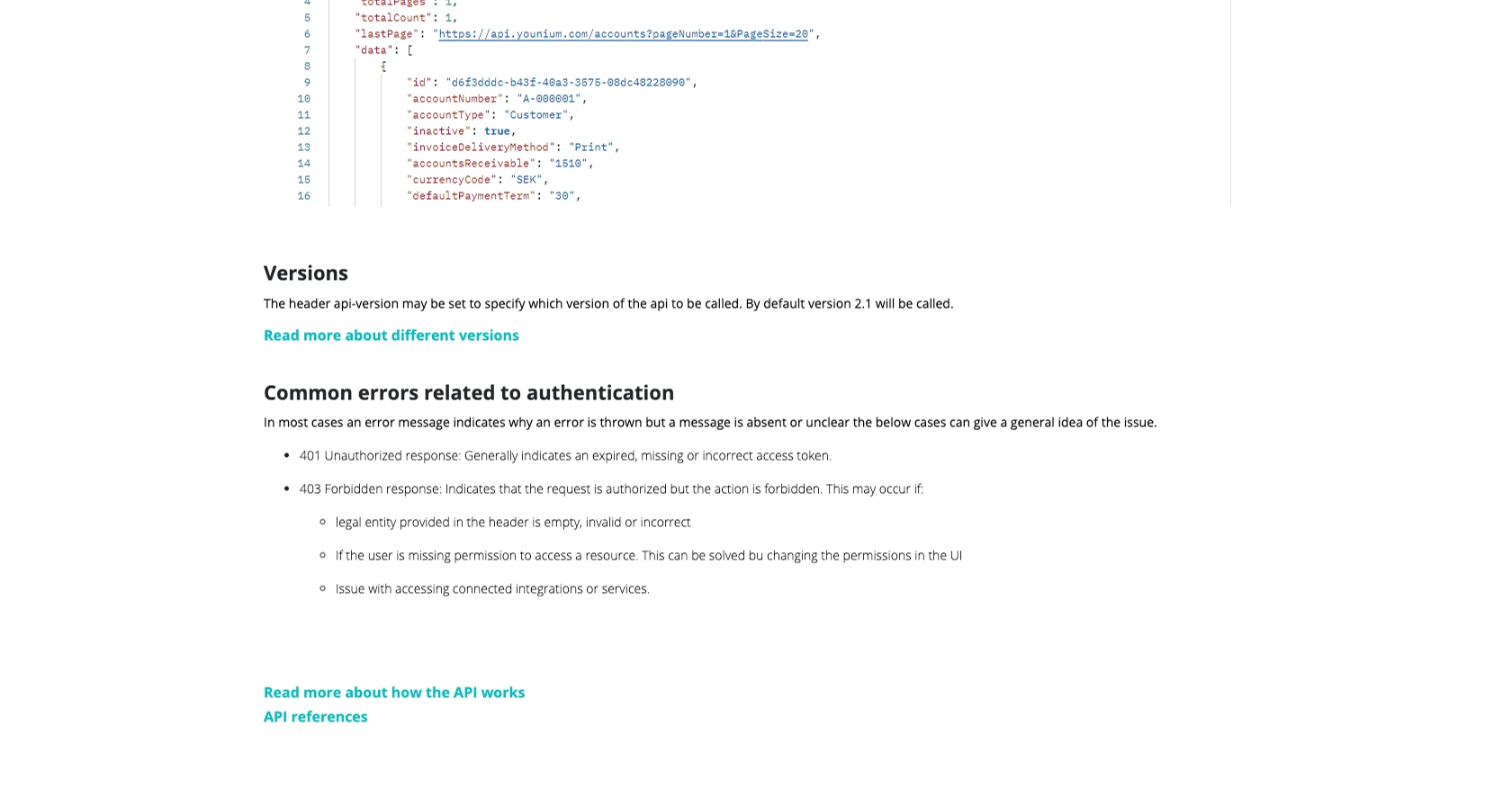
Conclusion and Best Practices for Younium API Integration with JavaScript
Integrating with the Younium API using JavaScript provides a powerful way to automate and streamline your subscription management processes. By following the steps outlined in this guide, you can efficiently retrieve product data and enhance your system's capabilities.
Best Practices for Secure and Efficient Younium API Usage
- Securely Store Credentials: Always store your client credentials and JWT tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that the data retrieved from Younium is standardized and transformed as needed to fit your application's requirements.
- Regularly Refresh Tokens: Remember that JWT tokens are valid for 24 hours. Implement a mechanism to refresh tokens automatically to maintain uninterrupted access.
Enhance Your Integration Experience with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Younium. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can streamline your integration efforts and provide a seamless experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration strategy and enhance your product offerings.
Read More
Ready to get started?