Using the Quickbooks API to Create or Update Accounts in Javascript
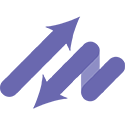
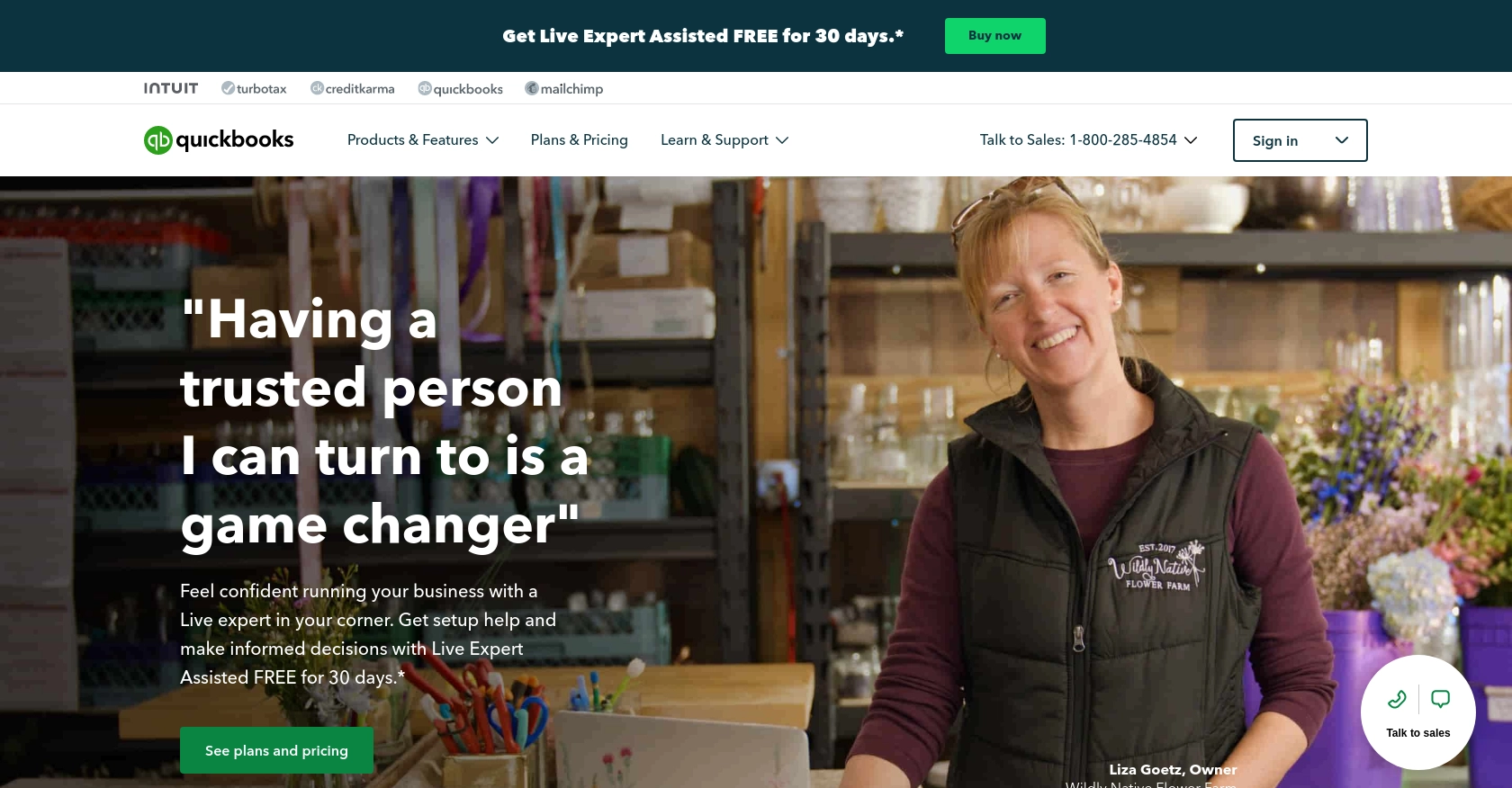
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a comprehensive suite of tools for invoicing, expense tracking, payroll, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with QuickBooks API allows developers to automate and streamline accounting processes, such as creating or updating accounts programmatically. For example, a developer might use the QuickBooks API to automatically update account information when a new transaction occurs, ensuring that financial records are always up-to-date and accurate.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start integrating with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your application in a controlled environment without affecting real financial data. QuickBooks provides a developer-friendly sandbox that mimics the live environment, making it ideal for testing API interactions.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Log In" if you already have one.
- Fill in the required information and complete the registration process.
Creating a QuickBooks App for OAuth Authentication
Once your developer account is set up, the next step is to create an app to obtain the necessary OAuth credentials. Here's how:
- Navigate to the App Management section in your QuickBooks Developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments" as the platform.
- Fill in the app details, such as name and description, and click "Create App."
Obtaining Client ID and Client Secret
After creating your app, you'll need the Client ID and Client Secret for OAuth authentication:
- Go to the "Keys & OAuth" section of your app settings.
- Copy the Client ID and Client Secret. These will be used to authenticate API requests.
Configuring OAuth Redirect URI
To ensure successful authentication, configure the OAuth Redirect URI:
- In the "Keys & OAuth" section, add your application's redirect URI under "Redirect URIs."
- Ensure that the URI matches the one used in your application code.
Testing in the QuickBooks Sandbox Environment
With your app set up, you can now test API calls in the sandbox environment:
- Access the sandbox company from your QuickBooks Developer account dashboard.
- Use the sandbox credentials to authenticate and test API interactions without affecting live data.
For more detailed information, refer to the QuickBooks API Documentation.
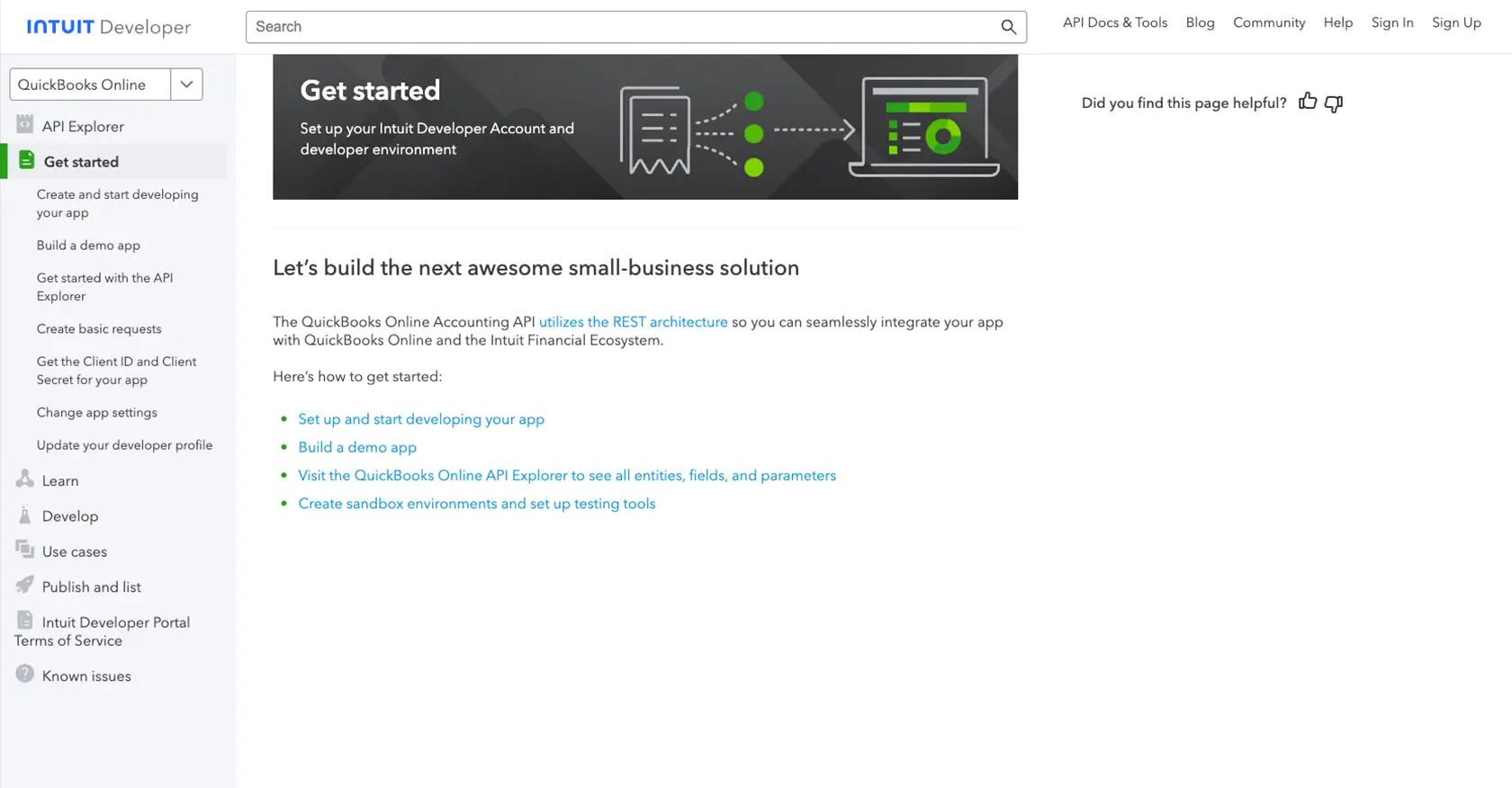
sbb-itb-96038d7
Making API Calls to QuickBooks Using JavaScript
To interact with the QuickBooks API using JavaScript, you'll need to set up your environment and write code to perform the desired API operations. This section will guide you through the process of creating or updating accounts using the QuickBooks API.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm to manage dependencies.
Install the required dependencies by running the following command in your terminal:
npm install axios
The axios
library will be used to make HTTP requests to the QuickBooks API.
Creating or Updating Accounts with QuickBooks API
Now, let's write the JavaScript code to create or update accounts in QuickBooks. Create a file named quickbooks_accounts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/account';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json',
'Accept': 'application/json'
};
// Define the account data
const accountData = {
"Name": "Sample Account",
"AccountType": "Expense"
};
// Function to create or update an account
async function createOrUpdateAccount() {
try {
const response = await axios.post(endpoint, accountData, { headers });
console.log('Account Created/Updated Successfully:', response.data);
} catch (error) {
console.error('Error Creating/Updating Account:', error.response.data);
}
}
createOrUpdateAccount();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and OAuth access token.
Running the JavaScript Code
To execute the code, run the following command in your terminal:
node quickbooks_accounts.js
If successful, you should see a confirmation message indicating that the account was created or updated. You can verify this by checking your QuickBooks sandbox environment.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's essential to handle potential errors. The QuickBooks API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
Refer to the QuickBooks API Documentation for more detailed information on error handling.
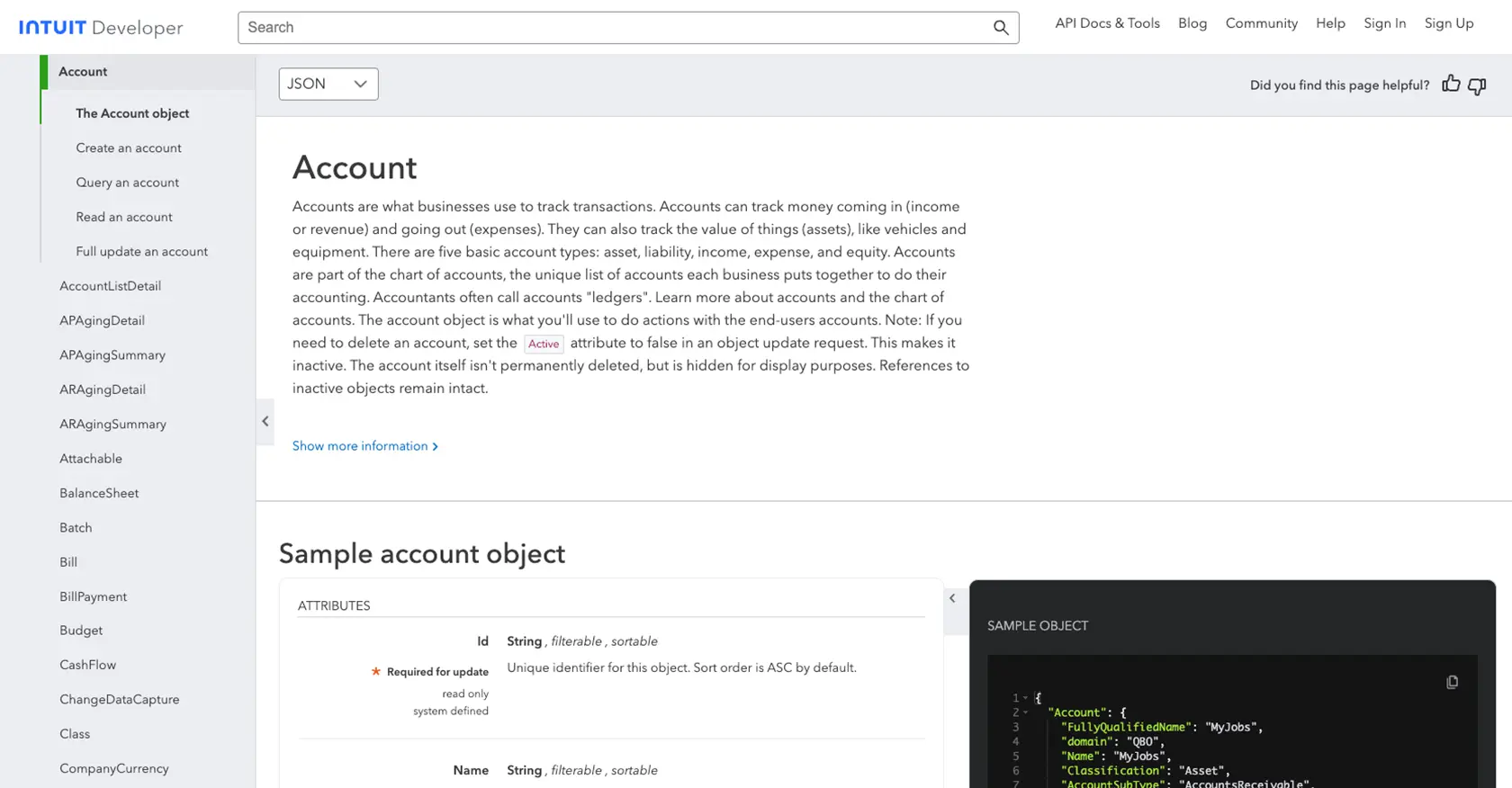
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using JavaScript allows developers to automate and streamline accounting processes, enhancing efficiency and accuracy in financial management. By following the steps outlined in this guide, you can successfully create or update accounts in QuickBooks, ensuring your financial data is always current.
Best Practices for Secure and Efficient QuickBooks API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. Refer to the QuickBooks API Documentation for specific rate limit details.
- Data Standardization: When integrating with QuickBooks, ensure that data fields are standardized and transformed as needed to match QuickBooks' requirements. This will help maintain data consistency across systems.
- Error Handling: Implement robust error handling to manage API response codes effectively. This will help you diagnose issues quickly and maintain a smooth integration experience.
Streamline Your Integrations with Endgrate
While integrating with QuickBooks API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including QuickBooks. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/account
Ready to get started?